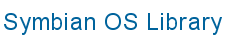
![]() |
![]() |
|
To write a Multimedia Framework (MMF) format plugin, the following needs to be done:
Write the appropriate source .cpp
and .h
files that make up the implementation of the format plugin.
See Creating a Format Decode Plugin and
Creating a Format Encode Plugin for information on how to do this.
Write a resource file defining the formats available in the plugin DLL.
Write the appropriate build files.
For each format plugin DLL one resource file (.RSS
) is required to enable ECom to identify and instantiate the format or formats present in the DLL. It is up to the implementer whether the formats are
to be placed in one or more ECom plugin DLLs.
An example resource file for a format plugin DLL containing one format decode and one format encode is as follows:
// nnnnnnnn.RSS
#include <acmeControllerUIDs.hrh>
#include <mmfPluginInterfaceUIDs.hrh>
#include <mmfdatasourcesink.hrh>
#include "RegistryInfo.rh"
RESOURCE REGISTRY_INFO theInfo
{
dll_uid = KAcmeFormatPluginDLLUid;
interfaces =
{
INTERFACE_INFO
{
interface_uid = KmmfUidPluginInterfaceFormatDecode ;
implementations =
{
IMPLEMENTATION_INFO
{
implementation_uid = KAcmeFormatWavReadUid;
version_no = 1;
display_name = "Acme WAV Read Format ";
default_data = "KUidAcmeControllerPlugin"
opaque_data = "<s>Acme<i>KUidMediaTypeAudio<e>.wav<h>RIFF?????WAVE<m>audio</x-wav">
}
}
},
INTERFACE_INFO
{
interface_uid = KmmfUidPluginInterfaceFormatEncode ;
implementations =
{
IMPLEMENTATION_INFO
{
implementation_uid = KAcmeFormatWavWriteUid;
version_no = 1;
display_name = "Acme WAV Write Format ";
default_data = "KUidAcmeControllerPlugin"
opaque_data = "<s>Acme<i>KUidMediaTypeAudio<e>.wav<h>RIFF?????WAVE<m>audio/wav<m>audio/x-wav"; }
};
}
}
}
Where:
nnnnnnnn
is the UID of the ECom plugin DLL. This is the same as the dll_uid
.
AcmeFormatUids.hrh
is a header file containing the format specific UIDs . These values could also be inserted directly into the resource file.
AcmeControllerUids.hrh
is a header file containing the controller specific UIDs . These values could also be inserted directly into the resource
file. The controller uid is required as the format needs to register which default controller it is expected to be used with
(see default_data
).
version_no
is the version number. Note that although this field can be read by ECom, the format instantiation does not make use of this field.
display_name
contains the display name.
default_data
is the UID used to specify the controller that is used in the controller recognition to identify which formats are supported
by the controller. This controller UID is not exclusive, other controller plugins with a different UID to that specified
in default_data
can also use a particular format plugin. The controller UID is used by the format recognition code (i.e when a client requests
information about the formats supported by the system, the format supported by the format plugin will be reported by the controller
plugin with the specified UID).
opaque_data
:
<s>
is the supplier of the plugin.
<i>
is a media id supported by this plugin. Multiple entries with this tag can be included.
<m>
is a mime type that describes the format. Multiple entries with this tag can be included.
<e>
is a file extension supported by this format. Multiple entries with this tag can be included.
<h>
is a segment of header data that can be matched against the first few bytes of a clip to check whether this format is capable
of handling the clip. Multiple entries with this tag can be included.
A number of audio formats are included as part of the multimedia framework which provide a number of standard common audio
formats. The formats provided are WAV
, au
and RAW
. These can be found in the multimedia component directory under \Mmf\Src\Plugin\Format
. These provide example code for writing a format plugin. There is also a test format in the UnitTest\basecl
sub-directories which could be used as a template for writing a new format plugin.
There are no special requirements for an MMF format plugin mmp
file over and above a conventional ECom plugin.
The main points to note are:
TARGETTYPE
is ECOMIIC
.
TARGETPATH
is \System\Libs\Plugins
.
UID
should be the ECom DLL
.
LIBRARY
needs to include ecom.lib
.
Note that it is not necessary to include mmfserverbaseclasses.lib
.
When implementing a format plugin consider the following:
Error handling. If the format can result in an error condition that needs reporting back to the controller during the playing
state, then the format should implement the MAsyncEventHandlerSendEventToClient()
mixin function. Format plugins should only use the SendEventToClient
methods if the error has ocurred internally (i.e. not due to the controller calling one of the format plugin's methods).
Performance considerations. The size of the buffer created by the format plugin needs some consideration. In general a larger
buffer size will provide better performance as it involves fewer buffer transfers between the source and the sink. The buffer
should always be large enough to support one frame of data, however if the frame size of the format is fairly small i.e.
less than 4K for example, then it is better to define a frame as a multiple of actual frames. Creating a too large frame
will lead to a larger frame time interval, which affects the granularity of positioning, and the time delay before processing
the first buffer. The optimum buffer size should be the maximum size such that the granularity is not too noticeable on repositioning
etc. For example, for pcm
data this gives a buffer size of 4K and a granularity of 1/8 second which is a good compromise. If a source or sink is optimised
for a certain buffer size then the controller may suggest a certain buffer size via the CMMFFormatDecodeClass::SuggestSourceBufferSize()
method. If the format plugin can use the suggested buffer size, it would be advantagous for the format plugin so to do,
since a buffer size will only be suggested if there is some efficiency advantage in using such a buffer size.
UIDs. In addition to the format plugin DLL having its own UID
, each format within the format DLL needs allocating its own UIDs. If more than one format plugin is available that handles
the same format then they must each have their own unique UID.