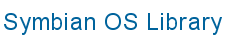
![]() |
![]() |
|
To write a Multimedia Framework (MMF) codec plugin, the following needs to be done:
Write a resource file defining the codecs available in the plugin DLL
Write the appropriate build file
Consider implementation issues
Codecs derived from CMMFCodec
can be audio, or video, codecs.
Codecs that use the Image Conversion Library (ICL) must conform to the
ICL codec API.
Codecs derived from CMMFCodec
are synchronous as it is
expected that the codec will be operating in its own thread of execution, usually via a
CMMFDataPath
or CMMFDataPathProxy
.
The functions in the CMMFCodec
base class, from which a specific
CMMFCodec
must derive, are:
CMMFCodec::NewL()
and
CMMFCodec::ProcessL()
.
Optional additions are CMMFCodec::ConfigureL()
and
CMMFCodec::ResetL()
.
One resource file (.RSS
) is required for each codec plugin DLL. This enables
ECom to identify and instantiate the codec, or codecs, present
in the DLL.
An example resource file follows:
// nnnnnnnn.RSS
//
#include <acmeCodecUIDs.hrh>
#include <mmfPluginInterfaceUIDs.hrh>
#include "RegistryInfo.rh"
RESOURCE REGISTRY_INFO theInfo
{
dll_uid = KAcmeCodecPluginDLLUid;
interfaces =
{
INTERFACE_INFO
{
interface_uid = KMmfUidPluginInterfaceCodec ; // CMMFCodec
implementations =
{
IMPLEMENTATION_INFO
{
implementation_uid = KAcmeCodec1Uid;
version_no = 1;
display_name = "ABCD to WXYZ Acme codec";
default_data = "ABCD, WXYZ" ; // FourCC codes
},
IMPLEMENTATION_INFO
{
implementation_uid = KAcmeCodec2Uid;
version_no = 1;
display_name = "mp3 to PCM16 Acme codec";
default_data = " MP3, P16" ; // FourCC codes.
}
};
}
};
}
Where:
nnnnnnnn
is the UID of the ECom plugin DLL.
AcmeCodecUids.hrh
is a header file containing the codec-specific UIDs.
Dll Uid = KAcmePluginCodecDLLUid
is the same as nnnnnnnn
.
version_no
is the version number. Note that although this field can be read by ECom, the codec instantiation does not make use of it.
display_name
contains display name information and, where used, the preferred supplier name.
default_data
is a pair of 4 character codes that indicate the assumed source data type the codec expects and the data type into which
the codec will decode the source data.
A number of audio codecs are included as part of the MMF which provide a number of standard codec conversions between common
audio data types such as uLaw
, aLaw
, pcm8
, pcm16
and gsm610
. These can be found in the multimedia component directory under \Mmf\Src\Plugin\Codec\Audio
.
Since most of these codecs are relatively simple, they provide a good source of example code for writing a codec plugin. There
are also a number of test codecs in the UnitTest
directory in the \acod
and \basecl
sub-directories which could be used as a template for writing a new CMMFCodec
plugin.
Codec-specific UIDs are defined in a header file. An example is shown below, this contains one plugin only, KExCodecUID
which has a UID of 0x101F81D7
:
#ifndef _MmfExCodecUIDS_H_
#define _MmfExCodecUIDS_H_
#define KExCodecUID 0x101F81D7
#endif
There are no special requirements for an MMF codec plugin mmp
file over and above a conventional ECom plugin.
The main points to note are:
TARGETTYPE
is ECOMIIC
TARGETPATH
is \System\Libs\Plugins
UID should be the ECOM DLL
LIBRARY
needs to include ecom.lib
Note that it is not necessary to include mmfserverbaseclasses.lib
.
When implementing a codec plugin, consider the following:
Memory usage. Codec implementations should avoid reallocating the destination buffer as the sink may require the buffer to be a certain size.
Error handling. If an error occurs during the codec’s data processing then the codec should leave if the error is non-recoverable. If the error may only impair the data rendering then the codec should continue.
Performance considerations. The codec's derived ProcessL()
function should attempt to fill the destination buffer as fully as possible to minimise the number of writes to the data
sink.
UIDs. In addition to the codec plugin DLL having its own UID, each codec within the codec DLL must be allocated its own UID. If more than one codec performs the same data type conversion then they will each require their own UID.