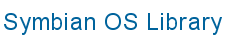
![]() |
![]() |
|
The following example code demonstrates how to use secure sockets.
Open a socket using RSocket::Open()
and connect it with RSocket::Connect()
.
Create a secure socket by calling CSecureSocket::NewL()
, passing the socket and secure protocol name.
In the following example iSocket
is a reference to the already opened and connected socket and
KSSLProtocol
is the descriptor containing the name of a protocol, in this case TLS1.0. The function should return a pointer to the newly
created CSecureSocket
.
Initiate a handshake with the remote server, using the CSecureSocket::StartClientHandshake()
function, to start the application acting as a client. Use CSecureSocket::StartServerHandshake()
to start acting as a server.
If the handshake fails, the call completes with an error code.
...
// Connect the socket server
User::LeaveIfError(iSocketServ.Connect());
// Open the socket
User::LeaveIfError(iSocket.Open(iSocketServ, KAfInet, KSockStream, KProtocolInetTcp));
//Connect the socket
connectInetAddr.SetAddress(KTestAddress);
connectInetAddr.SetPort(KSSLPort); //TLS port
iSocket.Connect(connectInetAddr, iStatus);
...
// Construct the Tls socket
iTlsSocket = CSecureSocket::NewL(iSocket, KSSLProtocol());
// start the handshake
iTlsSocket->StartClientHandshake(iStatus);
Use CSecureSocket::Recv()
and CSecureSocket::RecvOneOrMore()
to receive data from the socket.
Use CSecureSocket::Send()
to send data over the socket.