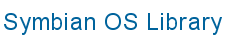
![]() |
![]() |
|
Here is a list of line and call information items that are available
through the Telephony ISV API, CTelephony
.
Only a simple status is available for the data and fax lines.
More detailed information is available for the voice line. Voice call control describes the Telephony ISV API's model of the voice line, which may help you understand the information available.
Call-in-progress indicator: are any calls in progress on the fax, data or voice line?
Voice line information:
Voice line status: overall status of the voice line. Use this to tell whether a voice call is in progress and to detect incoming calls
Status of voice calls you own: status of individual voice calls dialled or answered by you. Use this to detect when the remote party terminates your call
Detailed line and call information: use this to find out about any voice call currently in progress. It does not matter whether it is owned by you or not
Remote party information: use this to find out about a voice call's remote party. It does not matter whether it is owned by you or not. The remote party does not always allow this information to be available
Dynamic call capabilities: can a call be held, resumed or and swapped? This is only available for calls that you dialled or answered yourself
Note: With the exception of the Call-in-progress indicator, none of this information is available when the phone is in Flight Mode
You can get the current value of all these items. Each item's description gives you an example of this. Some of the methods to get information are asynchronous; the operation can be cancelled before it completes.
For some of these items, CTelephony
can notify you when the
information changes. Each item's description tells you whether notification is
available. If so, then this document tells you the notification
event and information class that you need.
How to request notification when information changes explains how to use them and provides some examples.
Notification can also be cancelled.
You can read a number of flags that indicate several conditions. Two of them answer the questions below:
Can CTelephony
detect when a call is in progress?
If so, is a call currently in progress? This is true if
any call is in progress: it can be a voice,
data or fax call, it can be active or on-hold, and it
does not matter whether the call is handled with CTelephony
or
not.
CTelephony::GetIndicator()
writes the value to
a packaged CTelephony::TIndicatorV1
.
This is an asynchronous call; use
CTelephony::EGetIndicatorCancel
to cancel it.
For example:
#include <e32base.h>
#include <Etel3rdParty.h>
class CClientApp : public CActive
{
private:
CTelephony* iTelephony;
CTelephony::TIndicatorV1 iIndicatorV1;
CTelephony::TIndicatorV1Pckg iIndicatorV1Pckg;
public:
CClientApp(CTelephony* aTelephony);
void SomeFunction();
private:
/*
These are the pure virtual methods from CActive that
MUST be implemented by all active objects
*/
void RunL();
void DoCancel();
};
CClientApp::CClientApp(CTelephony* aTelephony)
: CActive(EPriorityStandard),
iTelephony(aTelephony),
iIndicatorV1Pckg(iIndicatorV1)
{
//default constructor
}
void CClientApp::SomeFunction()
{
iTelephony->GetIndicator(iStatus, iIndicatorV1Pckg);
SetActive();
}
void CClientApp::RunL()
{
if(iStatus==KErrNone)
{
if(iIndicatorV1.iCapabilities & CTelephony::KIndCallInProgress)
{
// We can detect when a call is in progress
if(iIndicatorV1.iIndicator & CTelephony::KIndCallInProgress)
{} // A call is in progress
else
{} // A call is not in progress
}
else
{} // We do not know whether a call is in progress
}
}
void CClientApp::DoCancel()
{
iTelephony->CancelAsync(CTelephony::EGetIndicatorCancel);
}
Remember to link to the Etel3rdParty.lib
and
euser.lib
libraries.
The CTelephony::EIndicatorChange
notification
event indicates when any of the flags change. Use the
CTelephony::TIndicatorV1Pckg
information class and the
CTelephony::EIndicatorChangeCancel
cancellation code; see
Get notification when information changes.
A simple status is avilable for the phone's fax line.
The status returned in a packaged
CTelephony::TCallStatusV1
. It represents states such as
Idle, Dialling, Ringing, Answering, On-Hold etc.
Note: In the past, CTelephony
could
be used for data calls. This functionality has been deprecated and may be
removed in future version of Symbian OS.
CTelephony::GetLineStatus()
writes the
information to a packaged CTelephony::TCallStatusV1
. Pass
the method a CTelephony::TPhoneLine
set to
CTelephony::EFaxLine
.
This is a synchronous call and cannot be cancelled.
For example:
CTelephony* telephony = CTelephony::NewLC();
CTelephony::TCallStatusV1 callStatusV1;
CTelephony::TCallStatusV1Pckg callStatusV1Pckg( callStatusV1 );
CTelephony::TPhoneLine line = CTelephony::EFaxLine;
telephony->GetLineStatus( line, callStatusV1Pckg );
CTelephony::TCallStatus faxLineStatus = callStatusV1.iStatus;
CleanupStack::PopAndDestroy( telephony );
You cannot receive notification when the data line's status changes.
A simple status is avilable for the phone's data line.
The status returned in a packaged
CTelephony::TCallStatusV1
. It represents states such as
Idle, Dialling, Ringing, Answering, On-Hold etc.
Note: In the past, CTelephony
could
be used for data calls. This functionality has been deprecated and may be
removed in future version of Symbian OS.
CTelephony::GetLineStatus()
writes the
information to a packaged CTelephony::TCallStatusV1
. Pass
the method a CTelephony::TPhoneLine
set to
CTelephony::EDataLine
.
This is a synchronous call and cannot be cancelled.
For example:
CTelephony* telephony = CTelephony::NewLC();
CTelephony::TCallStatusV1 callStatusV1;
CTelephony::TCallStatusV1Pckg callStatusV1Pckg( callStatusV1 );
CTelephony::TPhoneLine line = CTelephony::EDataLine;
telephony->GetLineStatus( line, callStatusV1Pckg );
CTelephony::TCallStatus dataLineStatus = callStatusV1.iStatus;
CleanupStack::PopAndDestroy( telephony );
Remember to link to the Etel3rdParty.lib
library.
You cannot receive notification when the data line's status changes.
A simple status is avilable for the phone's voice line. Use this to tell whether a voice call is in progress and to detect incoming calls.
The status returned in a packaged
CTelephony::TCallStatusV1
. It represents states such as
Idle, Dialling, Ringing, Answering, On-Hold:
|
CTelephony::GetLineStatus()
writes the
information to a packaged CTelephony::TCallStatusV1
. Pass
the method a CTelephony::TPhoneLine
set to
CTelephony::EVoiceLine
.
This is a synchronous call and cannot be cancelled.
For example:
CTelephony* telephony = CTelephony::NewLC();
CTelephony::TCallStatusV1 callStatusV1;
CTelephony::TCallStatusV1Pckg callStatusV1Pckg( callStatusV1 );
CTelephony::TPhoneLine line = CTelephony::EVoiceLine;
telephony->GetLineStatus( line, callStatusV1Pckg );
CTelephony::TCallStatus voiceLineStatus = callStatusV1.iStatus;
CleanupStack::PopAndDestroy( telephony );
Remember to link to the Etel3rdParty.lib
library.
Use the CTelephony::EVoiceLineStatusChange
notification event, the CTelephony::TCallStatusV1Pckg
information class and the
CTelephony::EVoiceLineStatusChangeCancel
cancellation
code; see Get notification when information changes.
A simple status is available for each call that you own. It is
only available for calls the were dialled or answered by you using
CTelephony
; see
Voice call control.
This is useful to detect when the remote paarty terminates on of
your calls. Request notification when a call's status changes. If the new
status is CTelephony::EStatusIdle
then the remote party
has terminated the call.
The status is returned in a packaged
CTelephony::TCallStatusV1
. Inside this, the status is a
CTelephony::TCallStatus
set to one of:
|
CTelephony::GetCallStatus()
writes the
information to a packaged CTelephony::TCallStatusV1
. Pass
the method a CTelephony::TCallId
.
This is a synchronous call and cannot be cancelled.
This example reads the status of a call. When you dialled or
answered the call, you will have been given a
CTelephony::TCallId
that identifies it. Later, this can be
passed to CTelephony::GetCallStatus()
to read the call's
status:
CTelephony::TCallStatusV1 callStatusV1;
CTelephony::TCallStatusV1Pckg callStatusV1Pckg( callStatusV1 );
telephony->GetCallStatus( callId, callStatusV1Pckg );
CTelephony::TCallStatus callStatus = callStatusV1.iStatus;
Remember to link to the Etel3rdParty.lib
library.
This is an asynchronous call; use
CTelephony::EGetNetworkRegistrationStatusCancel
to cancel
it.
Use the CTelephony::EOwnedCall1StatusChange
or CTelephony::EOwnedCall2StatusChange
notification event
for lock 1 or 2 respectively.
Use the CTelephony::TCallStatusV1Pckg
information class.
Use the
CTelephony::EOwnedCall1StatusChangeCancel
or
CTelephony::EOwnedCall2StatusChangeCancel
cancellation
codes.
There is an example of this in Detect remote party terminating a call. See also Get notification when information changes.
The following detailed information is available for voice calls. It is not available for the fax or data calls. You do not need to have dialled or the answered the calls yourself in order to read this information.
The information is returned in a packaged
CTelephony::TCallInfoV1
. This contains several member
variables:
A CTelephony::TCallStatus
that
represents statuses such as Idle, Dialling, Ringing, Answering,
On-Hold etc
A TTimeIntervalSeconds
, measured in
seconds
A CTelephony::TTelAddress
. It is only
valid for outgoing calls. It includes any DTMF tones that have been sent.
A CTelephony::TPhoneNetworkSecurity
that
is either disabled, GSM ciphering enabled or WCDMA ciphering
enabled. It is only valid on GSM & WCDMA networks.
A TInt
. If the call is one that you own
then this is the call ID as returned by the
CTelephony::DialNewCall()
and
CTelephony::AnswerIncomingCall()
methods. Otherwise this
field has a value of '-1'.
Note:
TCallInfoV1
contains a
field called iExitCode
. This field is unused at present; it is
reserved for future expansion.
CTelephony::GetCallInfo()
writes the
information to a packaged CTelephony::TCallInfoV1
.
When you read information, you must select a call to read. You can select the currently active call, the call that's currently on hold, or a call that is in-progress - i.e. in the process of dialling/terminating/ringing etc.
An active call has the
CTelephony::EStatusConnected
status, a call on hold has
the CTelephony::EStatusHold
status. An
in-progress call refers to states such as ringing, dialling,
disconnecting etc. The line and call status can be read as described in
Voice line status
and Status of voice calls you own.
Pass the method a packaged
CTelephony::TCallSelectionV1
to select the information for
the desired call:
The iLine member must be set to
CTelephony::EVoiceLine
The iSelect member is a
CTelephony::TCallSelect
that selects the
CTelephony::EActiveCall
,
CTelephony::EHeldCall
or
CTelephony::EInProgressCall
call.
KErrNotFound
is returned if your selection is not
avilable. For instance, you could request information when no calls are being
made, or you could request information for an on-hold call when there is no
call on hold.
This is a synchronous call and cannot be cancelled.
This example reads both the detailed call infornation and the remote party information for the currently active call:
CTelephony* telephony = CTelephony::NewLC();
CTelephony::TCallInfoV1 callInfoV1;
CTelephony::TCallInfoV1Pckg callInfoV1Pckg( callInfoV1 );
CTelephony::TCallSelectionV1 callSelectionV1;
CTelephony::TCallSelectionV1Pckg callSelectionV1Pckg( callSelectionV1 );
CTelephony::TRemotePartyInfoV1 remotePartyInfoV1;
CTelephony::TRemotePartyInfoV1Pckg remotePartyInfoV1Pckg( remotePartyInfoV1 );
callSelectionV1.iLine = CTelephony::EVoiceLine;
callSelectionV1.iSelect = CTelephony::EActiveCall;
telephony->GetCallInfo( callSelectionV1Pckg, callInfoV1Pckg, remotePartyInfoV1Pckg );
CleanupStack::PopAndDestroy( telephony );
Remember to link to the Etel3rdParty.lib
library.
You cannot receive notification when this item changes. You can only be notified when the voice line's status changes; see Voice line status and Status of voice calls you own above.
The remote party may make their details available to you. If so, then the following information is available for the phone's voice line. It is not available for the fax or data lines. When the voice line is making more than one call (such as when one call is active and another is on hold) then you can read this information for individual calls.
You do not need to have dialled or the answered the call yourself in order to read this information. However, the remote party may not allow you to know this information.
The information is returned in a packaged
CTelephony::TRemotePartyInfoV1
. This contains several
member variables. It is important to check the remote identity
status before reading any other remote party information
:
A CTelephony::TCallRemoteIdentityStatus
that is either available, supressed or unknown.
Available indicates that the rest of the remote party information
is valid. Otherwise calling the party name, remote party's number and call
direction are not valid.
A descriptor of up to 80 characters describing the calling party. This field is only valid if the remote identity status field is set to available.
A CTelephony::TTelAddress
. This field is
only valid if the remote identity status field is set to
available.
A CTelephony::TCallDirection
. The call
is either mobile-originated (your phone dialled) or mobile-terminated (your
phone received the call). It is only valid if the remote identity
status field is set to available.
CTelephony::GetCallInfo()
writes the
information to a packaged CTelephony::TRemotePartyInfoV1
.
Pass the method a CTelephony::TCallSelectionV1
to select
the information for the desired call; see the description of
TCallSelectionV1
in
Detailed line and call information.
This is a synchronous call and cannot be cancelled.
See Detailed line and call information for an example of reading remote party information.
Use the
CTelephony::EOwnedCall1RemotePartyInfoChange
or
CTelephony::EOwnedCall2RemotePartyInfoChange
notification
event for call 1 or 2 respectively.
Use the CTelephony::TRemotePartyInfoV1Pckg
information class.
Use the
CTelephony::EOwnedCall1RemotePartyInfoChangeCancel
or
CTelephony::EOwnedCall2RemotePartyInfoChangeCancel
cancellation codes; see Get notification when information changes.
You can read flags that tell you the following for each call that you own:
Can the call be put on hold? This implies that the call is currently active and that there is no other call on-hold.
Can the call be resumed? This implies that the call is currently on hold and that there is no other active call.
Can the call be swapped? You can swap a call when you have two calls in progress, one active and one on hold. Swapping will put the active call on hold and activate the on-hold call.
You should check this information before putting a call on hold, and before resuming or swapping a held call.
CTelephony::GetCallDynamicCaps()
writes the
information to a packaged CTelephony::TCallCapsV1
.
This is a synchronous call and cannot be cancelled.
This example reads the capabilities of a call. When you dialled
or answered the call, you will have been given a
CTelephony::TCallId
that identifies it. Later, this can be
passed to CTelephony::GetCallDynamicCaps()
to read the
call's capabilities:
CTelephony::TCallCapsV1 callCapsV1;
CTelephony::TCallCapsV1Pckg callCapsV1Pckg( callCapsV1 );
telephony->GetCallDynamicCaps( callId, callCapsV1Pckg );
TUint32 caps = callCapsV1.iControlCaps;
if( caps & CTelephony::KCapsHold )
{} // The call represented by 'callId' can be put on hold
Remember to link to the Etel3rdParty.lib
library.
You cannot receive notification when this item changes.