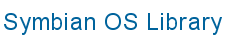
![]() |
![]() |
|
Location:
CDBPREFTABLE.H
Link against: commdb.lib
class CCommsDbConnectionPrefTableView : public CBase;
Implements actions on records of the Connection Preferences table, to allow clients to set the preferred order in which connections are attempted.
The class is similiar to CCommsDbTableView
, but only allows access to connection preferences as a set and not to individual fields in the table. This prevents inappropriate
updates of the records. Like CCommsDbTableView
, the class has a group of functions for navigating through the records in the view, and another group for setting fields
in the current record.
Clients do not create this type of object, but get an instance through CCommsDatabase::OpenConnectionPrefTableLC()
, CCommsDatabase::OpenConnectionPrefTableInRankOrderLC()
, or CCommsDatabase::OpenConnectionPrefTableViewOnRankLC()
.
In addition to the leave codes documented, all leaving functions can leave with any error returned by DBMS during database manipulation.
CBase
- Base class for all classes to be instantiated on the heap
CCommsDbConnectionPrefTableView
- Implements actions on records of the Connection Preferences table, to allow clients to set the preferred order in which
connections are attempted
Defined in CCommsDbConnectionPrefTableView
:
ChangeConnectionPreferenceRankL()
, ColNum()
, DeleteConnectionPreferenceL()
, GotoFirstRecord()
, GotoNextRecord()
, GotoPreviousRecord()
, InsertConnectionPreferenceL()
, InsertConnectionPreferenceL()
, ReadConnectionPreferenceL()
, ReadConnectionPreferenceL()
, SwapConnectionPreferencesL()
, TCommDbIapBearer
, TCommDbIapConnectionPref
, TCommDbIspBearer
, TCommDbIspConnectionPref
, UpdateBearerL()
, UpdateBearerL()
, UpdateDialogPrefL()
, iDb
, iTableExt
, iTableView
, ~CCommsDbConnectionPrefTableView()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C void InsertConnectionPreferenceL(const TCommDbIapConnectionPref &aPref, TBool aReadOnly=EFalse);
Inserts a new IAP connection preference into the connetion preference table. The supplied `aPref` is checked to ensure that the rank and direction do not conflict with existing records. The dialog option, bearer set and IAP are checked for consistancy. Deprecated in 7.0 but re-instated to accommodate 6.1 BC
|
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C void ReadConnectionPreferenceL(TCommDbIapConnectionPref &aPref);
Gets the IAP connection preferences record currently selected in the view.
|
|
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C void UpdateBearerL(const TCommDbIapBearer &aUpdate, TBool aReadOnly=EFalse);
Update the bearer set and IAP for the currently selected IAP connection preference record. The dialog option, bearer set and IAP are checked for consistancy. Deprecated in 7.0 but re-instated to accomodate 6.1 BC. Update the bearer set and iap for the currently selected connection preference. The dialog option, bearer set and IAP are checked for consistancy. The function sets the bearer set (CONNECT_PREF_BEARER_SET) and IAP (CONNECT_PREF_IAP) fields. The direction field (CONNECTION_PREF_DIRECTION) cannot be altered after it has been written to the record.
|
|
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C void UpdateDialogPrefL(const TCommDbDialogPref &aUpdate);
Updates the dialog preference field (CONNECT_PREF_DIALOG_PREF) in the currently selected connection preferences IAP record in the view.
|
|
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C void ChangeConnectionPreferenceRankL(TUint32 aNewRank);
Sets the rank field (CONNECT_PREF_RANKING) in the currently selected connection preference record in the view.
Note that if the new rank is not zero, and there is already a record with this rank and the same direction, then the existing record's rank is set to zero. This means that record is not used when the system finds the preferred connections.
|
|
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C void DeleteConnectionPreferenceL();
Deletes the currently selected connection preference record in the view.
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C void SwapConnectionPreferencesL(TCommDbConnectionDirection aDirection, TUint32 aFirstRank, TUint32 aSecondRank);
Swaps the bearer (CONNECT_PREF_BEARER_SET) and the IAP (CONNECT_PREF_IAP) fields between two connection preferences records.
The records to use are specified by their rank (CONNECT_PREF_RANKING) and direction (CONNECTION_PREF_DIRECTION).
Note that the dialogue option is not altered.
|
|
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C TInt GotoFirstRecord();
Sets the first connection preference record in this view as the current record.
|
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C TInt GotoNextRecord();
Sets the next connection preference record in the view as the current record.
|
Capability: | Dependent | on table, see the guide page referenced below. |
IMPORT_C TInt GotoPreviousRecord();
Sets the previous connection preference record in the view as the current record.
|
IMPORT_C void InsertConnectionPreferenceL(const TCommDbIspConnectionPref &aPref, TBool aReadOnly=EFalse);
Override version of InsertConnectionPreferenceL for BC purposes.
|
IMPORT_C void UpdateBearerL(const TCommDbIspBearer &aUpdate, TBool aReadOnly=EFalse);
Override version of UpdateBearerL for BC purposes.
|
IMPORT_C void ReadConnectionPreferenceL(TCommDbIspConnectionPref &aPref);
Framework left to support BC with v6.1
|
|
protected: inline TDbColNo ColNum(const TDesC &aColumn) const;
Returns the column number of the Column aColumn in the table
Coloumn concept doesn't exist in CommsDat
depNot supported from v9.1
|
|
class TCommDbIapBearer;
Encapsulates the bearer set (CONNECT_PREF_BEARER_SET) and IAP (CONNECT_PREF_IAP) fields. Used in calls to UpdateBearerL()
and as a public member of TCommDbIapConnectionPref
.
Defined in CCommsDbConnectionPrefTableView::TCommDbIapBearer
:
TCommDbIapBearer()
, iBearerSet
, iIapId
TCommDbIapBearer()
IMPORT_C TCommDbIapBearer();
Default constructor. All member data is initialised to zero.
iBearerSet
TUint32 iBearerSet;
Value for the bearer set (CONNECT_PREF_BEARER_SET) field.
iIapId
TUint32 iIapId;
Value for the IAP (CONNECT_PREF_IAP) field.
class TCommDbIapConnectionPref;
Encapsulates the rank (CONNECT_PREF_RANKING), direction (CONNECTION_PREF_DIRECTION), and dialog preference (CONNECT_PREF_DIALOG_PREF)
fields, plus a TCommDbIapBearer
object. A complete connection preference - containing rank, direction, dialogue option, bearers for the dialogue and the
prefered IAP.
Defined in CCommsDbConnectionPrefTableView::TCommDbIapConnectionPref
:
TCommDbIapConnectionPref()
, iBearer
, iDialogPref
, iDirection
, iRanking
, operator==()
TCommDbIapConnectionPref()
IMPORT_C TCommDbIapConnectionPref();
Default constructor. The rank is set to 0, and the direction and dialog preference to unknown.
operator==()
IMPORT_C TBool operator==(const TCommDbIapConnectionPref &aPref) const;
Overloaded equality operator. Checks for equality between 'this' and another TCommDbIapConnectionPref
object passed in.
|
|
iRanking
TUint32 iRanking;
Value for the rank (CONNECT_PREF_BEARER_SET) field.
iDirection
TCommDbConnectionDirection iDirection;
Value for the direction (CONNECTION_PREF_DIRECTION) field.
iDialogPref
TCommDbDialogPref iDialogPref;
Value for the dialog preference (CONNECT_PREF_DIALOG_PREF) field.
iBearer
TCommDbIapBearer iBearer;
Values for the bearer (CONNECT_PREF_BEARER_SET) and IAP (CONNECT_PREF_IAP) fields.
class TCommDbIspBearer;
Frame left in place for BC with 6.1
Defined in CCommsDbConnectionPrefTableView::TCommDbIspBearer
:
TCommDbIspBearer()
TCommDbIspBearer()
IMPORT_C TCommDbIspBearer();
Framework left in place for 6.1BC purposes.
class TCommDbIspConnectionPref;
Defined in CCommsDbConnectionPrefTableView::TCommDbIspConnectionPref
:
TCommDbIspConnectionPref()
, iBearer
, operator==()
TCommDbIspConnectionPref()
IMPORT_C TCommDbIspConnectionPref();
Framework left in place for 6.1BC purposes.
operator==()
IMPORT_C TBool operator==(const TCommDbIspConnectionPref &aPref) const;
Check for equality between 'this' and another TCommDbIspConnectionPref
object passed in. Framework letf in place for 6.1BC purposes. Returns KErrNotSupported only.
|
|
iBearer
TCommDbIspBearer iBearer;
protected: RDbView iTableView;
DBMS view. Variable not used in shim. Not removed because of BC break
protected: CCommsDatabase & iDb;
Comms data base that is being viewed.