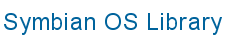
![]() |
![]() |
|
Location:
e32base.h
Link against: euser.lib
class CBase;
Base class for all classes to be instantiated on the heap.
By convention, all classes derived from CBase have a name beginning with the letter 'C'.
The class has two important features:
1. A virtual destructor that allows instances of derived classes to be destroyed and properly cleaned up through a CBase*
pointer. All CBase derived objects can be pushed, as CBase* pointers, onto the cleanup stack, and destroyed through a call
to CleanupStack::PopAndDestroy()
.
2. Initialisation of the CBase derived object to binary zeroes through a specific CBase::operator new()
- this means that members, whose initial value should be zero, do not have to be initialised in the constructor. This allows
safe destruction of a partially-constructed object.
Note that using C++ arrays of CBase-derived types is not recommended, as objects in the array will not be zero-initialised
(as there is no operator new[] member). You should use an array class such as RPointerArray
instead for arrays of CBase-derived types.
Defined in CBase
:
CBase()
, Delete()
, Extension_()
, operator new()
, operator new()
, operator new()
, operator new()
, operator new()
, ~CBase()
virtual IMPORT_C ~CBase();
Virtual destructor.
Enables any derived object to be deleted through a CBase* pointer.
inline TAny *operator new(TUint aSize, TAny *aBase) __NO_THROW;
Initialises the object to binary zeroes.
|
|
inline TAny *operator new(TUint aSize) __NO_THROW;
Allocates the object from the heap and then initialises its contents to binary zeroes.
|
|
inline TAny *operator new(TUint aSize, TLeave);
Allocates the object from the heap and then initialises its contents to binary zeroes.
|
|
inline TAny *operator new(TUint aSize, TUint aExtraSize) __NO_THROW;
Allocates the object from the heap and then initialises its contents to binary zeroes.
Use of this overload is rare.
|
|
inline TAny *operator new(TUint aSize, TLeave, TUint aExtraSize);
Allocates the object from the heap and then initialises its contents to binary zeroes.
Use of this overload is rare.
|
|
static IMPORT_C void Delete(CBase *aPtr);
Deletes the specified object.
|