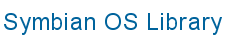
![]() |
![]() |
|
Location:
cchfdatasupplier.h
Link against: chf.lib
class CCHFDataSupplier : public CActive;
Base class for all Content Handling Framework Data Suppliers.
Data Suppliers provides the content of a URI-identified resource to a client.
The class is derived from CActive
to provide derived classes the mechanism for using their component in asynchronous transactions if required. Derived CCHFDataSupplier
class designers and users take full responsibility for managing the addition of their component to an active scheduler. Most
data suppliers will exhibit asynchronous behaviour and the API is designed to reflect this.
After creation of a data supplier component, the user of the component follows this recommended order of operation;
1. User
optionally calls SetReadPosition(TUint32 aReadPositionInBytes, TUint32 aReadLengthInBytes)
to specify range
2. User
calls Start(..) on the component to supplier an observer interface and to commence reading
3. Data Supplier calls ReceivedCompleteL() on the observer to indicate availability of content
4. User
calls ContentType(..) to retrieve the content type.
5. User
calls Read(..) to retrieve the content
6. User
calls RxReady()
to indicate readiness to receive more data
7. Data Supplier calls ReceivedCompleteL() on the observer to indicate availability of more data
8. Steps 5 to 6 is repeated until KErrEof is reported by either RxReady()
or ReceivedCompleteL()
CBase
- Base class for all classes to be instantiated on the heap
CActive
- The core class of the active object abstraction
CCHFDataSupplier
- Base class for all Content Handling Framework Data Suppliers
Defined in CCHFDataSupplier
:
BaseConstructL()
, CCHFDataSupplier()
, CCHFDataSupplier_Reserved_3()
, CCHFDataSupplier_Reserved_4()
, ContentType()
, EContentTypeKnown
, EContentTypeUnknown
, File()
, GetContentAccessIntent()
, GetContentAccessIntent()
, GetFile()
, NewFromFileHandleL()
, NewFromFileHandleL()
, Observer()
, Read()
, RecognizeDataL()
, RecognizeDataL()
, Reset()
, ResourceSize()
, Resume()
, RxReady()
, SetContentAccessIntent()
, SetContentTypeL()
, SetObserver()
, SetReadPosition()
, SetReadPosition()
, Start()
, Suspend()
, TContentTypeStatus
, Uri()
, iContentStatus
, ~CCHFDataSupplier()
Inherited from CActive
:
Cancel()
,
Deque()
,
DoCancel()
,
EPriorityHigh
,
EPriorityIdle
,
EPriorityLow
,
EPriorityStandard
,
EPriorityUserInput
,
Extension_()
,
IsActive()
,
IsAdded()
,
Priority()
,
RunError()
,
RunL()
,
SetActive()
,
SetPriority()
,
TPriority
,
iStatus
Inherited from CBase
:
Delete()
,
operator new()
IMPORT_C ~CCHFDataSupplier();
Destructor.
This delete the resources allocated in this base class and cancels the Active Object.
protected: IMPORT_C CCHFDataSupplier(TInt aPriority);
Constructor.
|
virtual TInt Start(MCHFDataSupplierObserver &aObserver)=0;
Starts the Data Supplier call to supply an observer interface and to request the Data Supplier to start supplying data.
|
|
virtual TInt Read(TPtrC8 &aReadBuffer)=0;
Clients should only call this method when they have been notified of a successful receive using the ReceivedCompleteL() method on the observer interface.
Reads the portion of data received.
The data is not destroyed when the call returns and may be read repeatedly before the next call to RxReady()
.
|
|
virtual TInt RxReady()=0;
Called by the client to indicate readiness to receive more data.
The last data is destroyed
|
virtual TInt SetReadPosition(TUint32 aReadPositionInBytes, TUint32 aReadLengthInBytes)=0;
Sets the range of data to be read.
|
|
IMPORT_C TInt SetReadPosition(TUint32 aReadPositionInBytes);
Sets the data supplier read position.
Use this method to navigate the resource content by moving the read position. To set the range, instead of just the position,
see CCHFDataSupplier::SetReadPosition(TUint32 aReadPositionInBytes, TUint32 aReadLengthInBytes)
|
|
virtual IMPORT_C void SetObserver(MCHFDataSupplierObserver &aObserver);
Replaces the current Data Supplier Observer.
Call this method to replace the observer of the Data Supplier. The new observer becomes the target of all subsequent call backs.
|
virtual IMPORT_C TInt Suspend();
Suspends activity on the Data Supplier.
|
virtual IMPORT_C TInt Resume();
Resumes the Data Supplier from a suspended state.
|
virtual IMPORT_C TContentTypeStatus ContentType(TPtrC8 &aContentType) const;
Gets the content type of the resource.
|
|
virtual IMPORT_C void SetContentTypeL(const TDesC8 &aNewContentType);
Sets the content type to a client-specified value.
Call this method to override the content type of the resource for subsequent use by a content handler.
|
|
virtual IMPORT_C const TDesC8 &Uri() const;
Gets the URI of the resource.
|
IMPORT_C RFile *File();
The public calling API for getting the file-handle of the resource, if the content is being supplied by file-handle rather than by URI.
|
virtual IMPORT_C TUint32 ResourceSize() const;
Gets the resource's total content size.
|
IMPORT_C MCHFDataSupplierObserver &Observer() const;
Gets the data supplier's observer.
|
|
virtual IMPORT_C TInt Reset();
Called by the client to reinitialise the data connection.
The data supplier is in its initial state. Start()
must be called again to reuse the resource.
|
IMPORT_C TInt GetContentAccessIntent(ContentAccess::TIntent &aContentAccessIntent) const;
The public calling API for getting the content-access intent.
|
|
IMPORT_C TInt SetContentAccessIntent(ContentAccess::TIntent aContentAccessIntent);
The public calling API for setting the content-access intent.
|
|
static IMPORT_C CCHFDataSupplier *NewFromFileHandleL(RFile &aFile, TUint aBufferSizeInBytes);
Creates a CCHFDataSupplier object given a file-handle (rather than a URI).
|
|
static IMPORT_C CCHFDataSupplier *NewFromFileHandleL(RFile &aFile, TUint aBufferSizeInBytes, ContentAccess::TIntent aContentAccessIntent);
Creates a CCHFDataSupplier object given a file-handle (rather than a URI).
|
|
protected: IMPORT_C void RecognizeDataL(const TDesC &aFileName, const TDesC8 &aDataBuffer);
Attempts recognition of the content MIME type.
This attempts to recognise the content MIME type given a portion of the content and its filename. It defaults the value of the content type to 'application/octet-stream' if there is no possible recognition, otherwise the content-type is set to the best guess of the content-type.
Sets the content type status indicator, iContentStatus, to EContentTypeKnown
|
|
protected: IMPORT_C void RecognizeDataL(RFile &aFile);
Attempts recognition of the content MIME type.
This attempts to recognise the content MIME type given a file-handle. It defaults the value of the content type to 'application/octet-stream' if there is no possible recognition, otherwise the content-type is set to the best guess of the content-type.
Sets the content type status indicator, iContentStatus, to EContentTypeKnown
|
|
protected: IMPORT_C void BaseConstructL(const TDesC8 &aUri);
Initialises the Data Supplier base class. Call this method in your derived class' ConstructL() to set the URI of the data supplier.
|
|
private: virtual IMPORT_C void GetFile(RFile *&aFile);
The private virtual function for getting the file-handle of the resource, if the content is being supplied by file-handle rather than by URI.
|
private: virtual IMPORT_C void GetContentAccessIntent(ContentAccess::TIntent *&aContentAccessIntent);
The private virtual function for getting the content-access intent value by non-const pointer, so that it can be read and modified.
|
TContentTypeStatus
Describes the status of the content type buffer; whether known, i.e. set, or not.
|
protected: TContentTypeStatus iContentStatus;
Holds the status of the content type buffer.