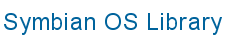
![]() |
![]() |
|
Location:
D32DBMS.H
Link against: edbms.lib
class RDbRowSet;
An abstract base class that provides functionality which is shared between SQL view objects and Table objects. This functionality includes most of the cursor navigation, row retrieval and update behaviour of rowsets.
Rowset objects do not provide the data for the rowset on which they operate. It is the responsibility of the derived classes
RDbView
and RDbTable
to specify the data source.
This class is not intended for user derivation.
Defined in RDbRowSet
:
AtBeginning()
, AtEnd()
, AtRow()
, BeginningL()
, Bookmark()
, Cancel()
, Close()
, ColCount()
, ColDef()
, ColDes()
, ColDes16()
, ColDes8()
, ColInt()
, ColInt16()
, ColInt32()
, ColInt64()
, ColInt8()
, ColLength()
, ColReal()
, ColReal32()
, ColReal64()
, ColSetL()
, ColSize()
, ColTime()
, ColType()
, ColUint()
, ColUint16()
, ColUint32()
, ColUint8()
, CountL()
, DeleteL()
, EBackwards
, EBeginning
, EEnd
, EEnsure
, EFirst
, EForwards
, EInsertOnly
, ELast
, ENext
, EPrevious
, EQuick
, EReadOnly
, EUpdatable
, EndL()
, FindL()
, FirstL()
, GetL()
, GotoL()
, GotoL()
, InsertCopyL()
, InsertL()
, IsColNull()
, IsEmptyL()
, LastL()
, MatchL()
, NextL()
, PreviousL()
, PutL()
, Reset()
, SetColL()
, SetColL()
, SetColL()
, SetColL()
, SetColL()
, SetColL()
, SetColL()
, SetColL()
, SetColL()
, SetColL()
, SetColNullL()
, TAccess
, TAccuracy
, TDirection
, TPosition
, UpdateL()
, iCursor
IMPORT_C void Close();
Closes the rowset and releases any owned resources. It is safe to close a rowset object which is not open.
IMPORT_C void Reset();
Resets the rowset.
For a Table, this just sets the rowset cursor to the beginning position.
For an SQL view, this discards any evaluated rows and returns the cursor to the beginning. The view then requires reevaluation.
The Store Database implementation requires this function to be called in order to recover an open rowset object after the database has been rolled back. The rowset does not need to be closed in this situation.
If a rowset object requires a reset, then all row functions return or leave with KErrNotReady.
IMPORT_C CDbColSet *ColSetL() const;
Returns the entire column set for the rowset. This can be used to discover column ordinals for named columns in this rowset.
The function leaves with KErrNoMemory if there is not enough memory to carry out the operation.
|
IMPORT_C TInt ColCount() const;
Returns the number of columns which are defined in this rowset.
|
IMPORT_C TDbColType ColType(TDbColNo aCol) const;
Returns the type of a column in the rowset.
|
|
IMPORT_C TDbCol ColDef(TDbColNo aCol) const;
Returns the definition of a column in the rowset.
|
|
IMPORT_C TBool AtRow() const;
Tests whether the cursor is on a row.
One of the following is true:
the rowset is currently updating a row
the rowset is currently inserting a row
GetL()
can be called to retrieve the row
|
IMPORT_C TBool AtBeginning() const;
Tests whether the cursor is at the beginning of the rowset.
|
IMPORT_C TBool AtEnd() const;
Tests whether the cursor is at the end of the rowset.
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy either the read or the write access policy for the table. |
IMPORT_C TInt CountL(TAccuracy anAccuracy=EEnsure) const;
Returns the number of rows available in a rowset.
This can take some time to complete, and a parameter can be passed to request a quick but not always thorough count of the rows.
For SQL views, the value returned depends on the evaluation window being used by the view. If there is an evaluation window this function will always return the number of rows available in the window, not the total number which could be returned by the query.
|
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
IMPORT_C TBool IsEmptyL() const;
Tests whether there are any rows in the rowset. This is often faster than testing whether CountL()
==0.
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
IMPORT_C TBool GotoL(TPosition aPosition);
Moves the cursor to a specified position.
This is invoked by Beginning(), End(), FirstL()
, LastL()
, NextL()
and PreviousL()
to navigate the cursor. See those functions for descriptions of how the cursor behaves given different position specifications.
|
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
inline void BeginningL();
Positions the cursor at the beginning of the rowset.
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
inline void EndL();
Positions the cursor at the end of the rowset.
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
inline TBool FirstL();
Positions the cursor on the first row in the rowset. If there are no rows, the cursor is positioned at the end.
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
inline TBool LastL();
Positions the cursor on the last row in the rowset. If there are no rows, the cursor is positioned at the beginning.
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
inline TBool NextL();
Moves the cursor to the next row in the rowset. If there are no more rows, the cursor is positioned to the end.
If the cursor is at the beginning prior to the function, it is equivalent to FirstL()
.
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
inline TBool PreviousL();
Moves the cursor to the previous row in the rowset. If there are no more rows, the cursor is positioned to the beginning.
If the cursor is at the end prior to the function, it is equivalent to LastL()
.
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
IMPORT_C TDbBookmark Bookmark() const;
Gets the bookmark for the current cursor position. Bookmarks cannot be extracted when the rowset is updating or inserting a row.
The Store Database implementation allows bookmarks to be extracted for any cursor position including the beginning and end.
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
IMPORT_C void GotoL(const TDbBookmark &aMark);
Goes to a previously bookmarked position in a rowset.
The Store Database implements bookmarks which are valid in any rowset based on the same table or generated by the same query, and which persist across transaction boundaries.
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
IMPORT_C TBool MatchL(const RDbRowConstraint &aConstraint);
Tests whether the current row in the rowset matches a previously compiled row constraint. The rowset must not currently be updating or inserting a row.
|
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
IMPORT_C TInt FindL(TDirection aDirection, TDbQuery aCriteria);
Searches through a rowset for a row which fulfils an SQL search-condition. The search begins from the current position (which must be a valid row) and proceeds forwards or backwards through the available rows until it finds a match or runs out of rows.
The cursor is positioned to the matching row (or beginning/end of set) on return.
This is a brute-force approach to finding a row using an index for key-based retrieval on a Table rowset is a much faster but less flexible way of finding rows.
|
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the read access policy for the table. |
IMPORT_C void GetL();
Gets the current row data for access using the column extractor functions. The cursor must be positioned at a valid row.
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the write access policy for the table. |
IMPORT_C void InsertL();
Inserts a new row into the rowset. All auto-increment columns will be initialised with their new values, all other columns
will be initialised to NULL values. If no client-begun transaction is in progress, this function begins an automatic transaction,
which is committed by PutL()
.
After the column values have been set using the SetColL()
functions, the row can be written to the database using PutL()
.
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the write access policy for the table. |
IMPORT_C void InsertCopyL();
Inserts a copy of the current row into the rowset. All auto-increment columns will be given a new value (as for InsertL()
), the other columns will copy their values from the cursor's current row. If no client-begun transaction is in progress,
this function begins an automatic transaction, which is committed by PutL()
.
After the column values have been modified using the SetColL()
functions, the row can be written to the database using PutL()
.
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the write access policy for the table. |
IMPORT_C void UpdateL();
Prepares the current row for update. If no client-begun transaction is in progress, this function begins an automatic transaction,
which is committed by PutL()
.
After the column values have been modified using the SetColL()
functions, the row can be written back to the database using PutL()
.
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the write access policy for the table. |
IMPORT_C void PutL();
Completes the update or insertion of a row.
First the new row data is validated:
not-null columns are checked to be not NULL
numerical columns are checked to be in range for their type
variable length columns are checked to not exceed their maximum length
unique index keys are checked to ensure uniqueness is not violated
Note that modifying auto-increment columns is not prevented by DBMS.
Following validation the data is written to the database and any affected indexes are updated. On successful completion of
the write, PutL()
will then commit any automatic transaction.
The cursor is left positioned on the updated or inserted row — where this lies in the rowset is not always well defined. To return to the row which was current prior to the update or insertion, a bookmark can be used.
In the Store Database implementation the written row is located in the rowset as follows:
Tables without an active index will leave updated rows in the same location and append new rows to the end of the rowset.
Tables with an active index place the row according to the active index ordering.
SQL views without an evaluation window will place it according to the rowset ordering. The row may subsequently disappear if it does not match the WHERE clause of the SQL query.
SQL views with a full evaluation window will leave updated rows in the same location and append new rows to the end of the rowset. Re-evaluation may cause the row to disappear if it does not match the WHERE clause of the SQL query.
SQL views with a partial evaluation window will leave updated rows in the same location, new rows are not added to the window and navigation from the new row is undefined. Further navigation and evaluation of the partial window will place the rows in the correct location according to the query.
IMPORT_C void Cancel();
Cancels the update or insertion of a row, or recovers the rowset if PutL()
fails. The cursor will return to the location prior to starting the update or insertion. It is also safe to call this function
when the rowset object is not updating or inserting a row, in which case it does nothing.
In the Store database implementation, if this is called to abort a row update or insertion before PutL()
is called or during row validation in PutL()
, all the changes are discarded without requiring a transaction rollback and the rowset to be Reset()
.
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the write access policy for the table. |
IMPORT_C void DeleteL();
Deletes the current row in a rowset. The rowset must not currently be updating or inserting the row.
The rowset cursor is left positioned at the "hole" left by the deletion of the current row. Navigation to the next or previous row will have the same effect as if this row had not been deleted. Once the cursor has been moved from the "hole" it will disappear from the rowset.
If the client has not begun a transaction, this function will use an automatic transaction to update the rowset.
inline TBool IsColNull(TDbColNo aCol) const;
Tests whether a column has the NULL value.
Columns which have the NULL value can still be extracted with the correct accessor function, in which case numerical columns will return a 0 (or equivalent) value, and text and binary columns will have a zero length.
|
|
IMPORT_C TInt ColSize(TDbColNo aCol) const;
Gets the size in bytes of a column value. This can be used for all column types, including Long columns. NULL columns return a size of 0.
Note:
This may yield unexpected results for small numerical column types as they are stored in memory as 32-bit values.
|
|
IMPORT_C TInt ColLength(TDbColNo aCol) const;
Gets the length of a column value. As compared with ColSize()
, this returns the number of "units" in the column:
NULL columns have a length of 0
non-NULL numerical and date-time columns have a length of 1
for Text columns the length is the character count
for Binary columns the length is the byte count
|
|
IMPORT_C TInt8 ColInt8(TDbColNo aCol) const;
Extracts a TInt8 column value.
|
|
IMPORT_C TInt16 ColInt16(TDbColNo aCol) const;
Extracts a TInt16 or TInt8 column value.
|
|
IMPORT_C TInt32 ColInt32(TDbColNo aCol) const;
Extracts a TInt32, TInt16 or TInt8 column value.
|
|
IMPORT_C TInt64 ColInt64(TDbColNo aCol) const;
Extracts a TInt64 column value.
|
|
inline TInt ColInt(TDbColNo aCol) const;
Extracts a signed integer column value. The type should fit within a TInt.
|
|
IMPORT_C TUint8 ColUint8(TDbColNo aCol) const;
Extracts a Uint8 or Bit column value.
|
|
IMPORT_C TUint16 ColUint16(TDbColNo aCol) const;
Extracts a Uint16, Uint8 or Bit column value.
|
|
IMPORT_C TUint32 ColUint32(TDbColNo aCol) const;
Extracts a Uint32, Uint16, Uint8 or Bit column value.
|
|
inline TUint ColUint(TDbColNo aCol) const;
Extracts an unsigned integer column value.
|
|
IMPORT_C TReal32 ColReal32(TDbColNo aCol) const;
Extracts a TReal32 column value.
|
|
IMPORT_C TReal64 ColReal64(TDbColNo aCol) const;
Extracts a TReal64 column value.
|
|
inline TReal ColReal(TDbColNo aCol) const;
Extracts a TReal64 column value.
|
|
IMPORT_C TTime ColTime(TDbColNo aCol) const;
Extracts a TTime
column value.
|
|
IMPORT_C TPtrC8 ColDes8(TDbColNo aCol) const;
Extracts any column type, except Long columns, as binary data. Can handle any type of non-long column
|
|
IMPORT_C TPtrC16 ColDes16(TDbColNo aCol) const;
Extracts a column as Unicode text.
|
|
IMPORT_C TPtrC ColDes(TDbColNo aCol) const;
Extracts a Text column value. The column type must match the default descriptor type to use this extractor, ie. it must be equal to EDbColText.
|
|
Capability: | Security policy note: | For a secure shared database, the caller must satisfy the write access policy for the table. |
IMPORT_C void SetColNullL(TDbColNo aCol);
Use this function to set the value of a column to NULL.
|
inline void SetColL(TDbColNo aCol, TInt aValue);
Sets a signed integer column value. The type should fit into a TInt.
|
IMPORT_C void SetColL(TDbColNo aCol, TInt32 aValue);
Sets a TInt32, TInt16 or TInt8 column value.
|
IMPORT_C void SetColL(TDbColNo aCol, TInt64 aValue);
Sets a TInt64 column value.
|
inline void SetColL(TDbColNo aCol, TUint aValue);
Sets a signed integer column value. The type should fit into a TInt.
|
IMPORT_C void SetColL(TDbColNo aCol, TUint32 aValue);
Sets a TUint32, TUint16, TUint8 or Bit column value.
|
IMPORT_C void SetColL(TDbColNo aCol, TReal32 aValue);
Sets a TReal32 column value.
|
IMPORT_C void SetColL(TDbColNo aCol, TReal64 aValue);
Sets a TReal64 column value.
|
IMPORT_C void SetColL(TDbColNo aCol, TTime aValue);
Sets a TTime
column value.
TTime
could be either local time or universal time. DBMS doesn't interpret the value of TTime
, it is left up to the user to decide which should be used.
|
IMPORT_C void SetColL(TDbColNo aCol, const TDesC8 &aValue);
Sets a column value from an 8 bit descriptor. This function can also set the value of Long columns.
Usually this is used to set a Text8 or LongText8 column from a non-Unicode text descriptor, but can be used for any column
type: the data content is validated when the row is PutL()
.
|
IMPORT_C void SetColL(TDbColNo aCol, const TDesC16 &aValue);
Set a column value from Unicode text.
|
TPosition
Specifies where the rowset should navigate to in the GotoL()
function. Their use is encapsulated by the respective member functions FirstL()
, NextL()
etc.
|
TAccess
Specifies which operations can be performed on a rowset.
|
TDirection
Specifies the direction to search through the rowset when using the FindL()
function.
|
TAccuracy
Specifies whether the CountL()
function should ensure that it returns the exact value which may be a non-trivial task.
|
protected: RDbHandle< CDbCursor > iCursor;