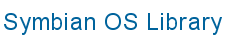
![]() |
![]() |
|
Location:
e32base.h
Link against: euser.lib
class CServer2 : public CActive;
Abstract base class for servers (version 2).
This is an active object. It accepts requests from client threads and forwards them to the relevant server-side client session. It also handles the creation of server-side client sessions as a result of requests from client threads.
A server must define and implement a derived class.
(Note that this class should be used instead of CServer)
CBase
- Base class for all classes to be instantiated on the heap
CActive
- The core class of the active object abstraction
CServer2
- Abstract base class for servers (version 2)
Defined in CServer2
:
CServer2()
, DoCancel()
, DoConnect()
, EGlobalSharableSessions
, ESharableSessions
, EUnsharableSessions
, Extension_()
, Message()
, NewSessionL()
, ReStart()
, RunError()
, RunL()
, Server()
, Start()
, StartL()
, TServerType
, iSessionIter
, ~CServer2()
Inherited from CActive
:
Cancel()
,
Deque()
,
EPriorityHigh
,
EPriorityIdle
,
EPriorityLow
,
EPriorityStandard
,
EPriorityUserInput
,
IsActive()
,
IsAdded()
,
Priority()
,
SetActive()
,
SetPriority()
,
TPriority
,
iStatus
Inherited from CBase
:
Delete()
,
operator new()
virtual IMPORT_C ~CServer2()=0;
Frees resources prior to destruction.
Specifically, it cancels any outstanding request for messages, and deletes all server-side client session objects.
protected: IMPORT_C CServer2(TInt aPriority, TServerType aType=EUnsharableSessions);
Constructs the server object, specifying the server type and the active object priority.
Derived classes must define and implement a constructor through which the priority can be specified. A typical implementation calls this server base class constructor through a constructor initialization list.
|
Capability: | ProtServ | if aName starts with a '!' character |
IMPORT_C TInt Start(const TDesC &aName);
Adds the server with the specified name to the active scheduler, and issues the first request for messages.
|
|
Capability: | ProtServ | if aName starts with a '!' character |
IMPORT_C void StartL(const TDesC &aName);
Adds the server with the specified name to the active scheduler, and issues the first request for messages, and leaves if the operation fails.
|
IMPORT_C void ReStart();
Restarts the server.
The function issues a request for messages.
inline RServer2 Server() const;
Gets a handle to the server.
Note that the RServer2
object is classified as Symbian internal, and its member functions cannot be acessed. However, the handle can be passed to
the RSessionBase::CreateSession()
variants that take a server handle.
|
protected: inline const RMessage2 &Message() const;
Gets a reference to the server's current message.
|
protected: virtual IMPORT_C void DoCancel();
Implements the cancellation of any outstanding request for messages.
protected: virtual IMPORT_C TInt RunError(TInt aError);
Handles the situation where a call to CServer2::RunL()
, leaves.
This is the server active object's implementation of the active object framework's RunError()
function.
In practice, the leave can only be caused by a session's ServiceL() function, which is called from this RunL()
; this error is reflected back to that session by calling its ServiceError() function.
|
|
protected: virtual IMPORT_C void DoConnect(const RMessage2 &aMessage);
Handles the connect request from the client.
|
protected: virtual IMPORT_C TInt Extension_(TUint aExtensionId, TAny *&a0, TAny *a1);
Extension function
|
|
private: virtual IMPORT_C CSession2 *NewSessionL(const TVersion &aVersion, const RMessage2 &aMessage) const=0;
Creates a server-side session object.
The session represents a communication link between a client and a server, and its creation is initiated by the client through
a call to one of the RSessionBase::CreateSession()
variants.
A server must provide an implementation, which as a minimum should:
check that the version of the server is compatible with the client by comparing the client supplied version number against the server's version number; it should leave if there is incompatibility.
construct and return the server side client session object.
|
|
TServerType
Defines the set of session types that the server can create.
A specific session type is specified when the CServer2 object is created.
|
protected: TDblQueIter< CSession2 > iSessionIter;