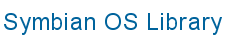
![]() |
![]() |
|
Location:
ecam.h
class TCameraInfo;
Specifies camera device information.
If either zoom or digital zoom are available then the appropriate entries in this class will be set to indicate the range of values that they may take. This should be implemented such that a setting of zero corresponds to no zoom, and a step of one corresponds to the smallest increment available, in a linear fashion.
There are also zoom factors that correspond to the actual zoom factor when at minimum (non-digital only) and maximum zoom. Negative zoom values represent macro functionality.
Image capture, if supported, is simply a case of transferring the current image from the camera to the client via MCameraObserver::ImageReady()
. The camera class must set the iImageFormatsSupported bitfield to indicate the formats available.
Defined in TCameraInfo
:
EBrightnessSupported
, EContrastSupported
, EImageCaptureSupported
, EImageClippingSupported
, EOrientationInwards
, EOrientationMobile
, EOrientationOutwards
, EOrientationUnknown
, EVideoCaptureSupported
, EVideoClippingSupported
, EViewFinderBitmapsSupported
, EViewFinderClippingSupported
, EViewFinderDirectSupported
, EViewFinderMirrorSupported
, TCameraOrientation
, TOptions
, iExposureModesSupported
, iFlashModesSupported
, iHardwareVersion
, iImageFormatsSupported
, iMaxBuffersSupported
, iMaxDigitalZoom
, iMaxDigitalZoomFactor
, iMaxFramesPerBufferSupported
, iMaxZoom
, iMaxZoomFactor
, iMinZoom
, iMinZoomFactor
, iNumImageSizesSupported
, iNumVideoFrameRatesSupported
, iNumVideoFrameSizesSupported
, iOptionsSupported
, iOrientation
, iSoftwareVersion
, iVideoFrameFormatsSupported
, iWhiteBalanceModesSupported
TCameraOrientation
Possible directions in which the camera may point.
|
TOptions
Various flags describing the features available for a particular implementation
|
TUint32 iFlashModesSupported;
The supported flash modes.
This is a bitfield of CCamera::TFlash
values.
If methods CCamera::New2L()
or CCamera::NewDuplicate2L()
are not used to create the CCamera
object, it is assumed that the application may not be able to cope with any future additions to the enum values. So, any
unrecognised enum value passed from the implementation should be filtered by ECAM Implementation. To receive unrecognised/extra
added enum values, application should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. This is an indication to ECAM implementation. In this case, application is assumed to be prepared
to receive unrecognised enum values.
TUint32 iExposureModesSupported;
The supported exposure modes.
This is a bitfield of CCamera::TExposure
values.
TUint32 iWhiteBalanceModesSupported;
The supported white balance settings.
This is a bitfield of of CCamera::TWhiteBalance
values.
If methods CCamera::New2L()
or CCamera::NewDuplicate2L()
are not used to create the CCamera
object, it is assumed that the application may not be able to cope with any future additions to the enum values. So, any
unrecognised enum value passed from the implementation should be filtered by ECAM Implementation. To receive unrecognised/extra
added enum values, application should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. This is an indication to ECAM implementation. In this case, application is assumed to be prepared
to receive unrecognised enum values. Refer to CCamera::CCameraAdvancedSettings::SupportedWhiteBalanceModes()
implementation
TInt iMinZoom;
Minimum zoom value allowed.
Must be negative, or zero if not supported.
This is the minimum value that may be passed to CCamera::SetZoomFactorL()
.
TInt iMaxZoom;
Maximum zoom value allowed.
Must be positive, or zero if not supported.
This is the maximum value that may be passed to CCamera::SetZoomFactorL()
.
TInt iMaxDigitalZoom;
Maximum digital zoom value allowed.
Must be positive, or zero if not supported.
This is the maximum value that may be passed to CCamera::SetDigitalZoomFactorL()
. Digital zoom factor is assumed to be a linear scale from 0 to iMaxDigitalZoom.
TReal32 iMinZoomFactor;
Image size multiplier corresponding to minimum zoom value.
Must be between 0 and 1 inclusive. Both 0 and 1 indicate that macro functionality is not supported.
TReal32 iMaxZoomFactor;
Image size multiplier corresponding to maximum zoom value.
May take the value 0, or values greater than or equal to 1. Both 0 and 1 indicate that zoom functionality is not supported.
TReal32 iMaxDigitalZoomFactor;
Image size multiplier corresponding to maximum digital zoom value.
Implementation recommendation is to use 'appropriate value' for maximum digital zoom which could cover only values given by new digital zoom methods based on image format and capture mode.
Must be greater than or equal to 1.
TInt iNumImageSizesSupported;
Count of still image capture sizes allowed.
Number of different image sizes that CCamera::EnumerateCaptureSizes()
will support, based on the index passed in. Index must be between 0 and iNumImageSizesSupported-1.
TUint32 iImageFormatsSupported;
The supported still image formats.
This is a bitfield of CCamera::TFormat
values.
TInt iNumVideoFrameSizesSupported;
Count of video frame sizes allowed.
This is the number of different video frame sizes that CCamera::EnumerateVideoFrameSizes()
will support, based on the specified index. The index must be between 0 and iNumVideoFrameSizesSupported-1.
TInt iNumVideoFrameRatesSupported;
Count of video frame rates allowed.
This is the number of different video frame rates that CCamera::EnumerateVideoFrameRates()
will support, based on the specified index. The index must be between 0 and iNumVideoFrameRatesSupported-1.
TUint32 iVideoFrameFormatsSupported;
The supported video frame formats.
This is a bitfield of video frame CCamera::TFormat
values.
TInt iMaxFramesPerBufferSupported;
Maximum number of frames per buffer that may be requested.
TInt iMaxBuffersSupported;
Maximum number of buffers allowed