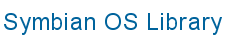
![]() |
![]() |
|
Location:
ecam.h
Link against: ecam.lib
class CCamera : public CBase;
Base class for camera devices.
Provides the interface that an application uses to control, and acquire images from, the camera.
An application must supply an implementation of MCameraObserver2
(or MCameraObserver
).
CBase
- Base class for all classes to be instantiated on the heap
CCamera
- Base class for camera devices
Defined in CCamera
:
Brightness()
, BuffersInUse()
, CCameraAdvancedSettings
, CCameraImageProcessing
, CCameraPresets
, CameraInfo()
, CamerasAvailable()
, CancelCaptureImage()
, CaptureImage()
, Contrast()
, CustomInterface()
, DigitalZoomFactor()
, EBrightnessAuto
, EContrastAuto
, EExposureAperturePriority
, EExposureAuto
, EExposureBacklight
, EExposureBeach
, EExposureCenter
, EExposureInfra
, EExposureManual
, EExposureNight
, EExposureProgram
, EExposureShutterPriority
, EExposureSnow
, EExposureSport
, EExposureSuperNight
, EExposureVeryLong
, EFlashAuto
, EFlashFillIn
, EFlashForced
, EFlashManual
, EFlashNone
, EFlashRedEyeReduce
, EFlashSlowFrontSync
, EFlashSlowRearSync
, EFlashVideoLight
, EFormat16BitRGB565
, EFormat16bitRGB444
, EFormat32BitRGB888
, EFormatExif
, EFormatFbsBitmapColor16M
, EFormatFbsBitmapColor16MU
, EFormatFbsBitmapColor4K
, EFormatFbsBitmapColor64K
, EFormatJpeg
, EFormatMonochrome
, EFormatUserDefined
, EFormatYUV420Interleaved
, EFormatYUV420Planar
, EFormatYUV420SemiPlanar
, EFormatYUV422
, EFormatYUV422Reversed
, EFormatYUV444
, EWBAuto
, EWBBeach
, EWBCloudy
, EWBDaylight
, EWBFlash
, EWBFluorescent
, EWBManual
, EWBShade
, EWBSnow
, EWBTungsten
, EnumerateCaptureSizes()
, EnumerateVideoFrameRates()
, EnumerateVideoFrameSizes()
, Exposure()
, Flash()
, FrameRate()
, FramesPerBuffer()
, GetFrameSize()
, Handle()
, JpegQuality()
, New2L()
, NewDuplicate2L()
, NewDuplicateL()
, NewDuplicateL()
, NewL()
, NewL()
, PowerOff()
, PowerOn()
, PrepareImageCaptureL()
, PrepareImageCaptureL()
, PrepareVideoCaptureL()
, PrepareVideoCaptureL()
, Release()
, Reserve()
, SetBrightnessL()
, SetContrastL()
, SetDigitalZoomFactorL()
, SetExposureL()
, SetFlashL()
, SetJpegQuality()
, SetViewFinderMirrorL()
, SetWhiteBalanceL()
, SetZoomFactorL()
, StartVideoCapture()
, StartViewFinderBitmapsL()
, StartViewFinderBitmapsL()
, StartViewFinderDirectL()
, StartViewFinderDirectL()
, StartViewFinderL()
, StartViewFinderL()
, StopVideoCapture()
, StopViewFinder()
, TBrightness
, TContrast
, TExposure
, TFlash
, TFormat
, TWhiteBalance
, VideoCaptureActive()
, ViewFinderActive()
, ViewFinderMirror()
, WhiteBalance()
, ZoomFactor()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Capability: | MultimediaDD | A process requesting or using this method that has MultimediaDD capability will always have precedence over a process that does not have MultimediaDD. |
Capability: | UserEnvironment | An application that creates a CCamera object must have the UserEnvironment capability. |
static IMPORT_C CCamera *NewL(MCameraObserver2 &aObserver, TInt aCameraIndex, TInt aPriority);
Creates an object representing a camera.
Note: Applications using this method to create camera object may not receive enums/uids added in future( after being baselined). To receive them, they should rather use New2L(..) or NewDuplicate2L(..), in which case, they should prepare themselves to receive unrecognised values.
|
|
|
Capability: | UserEnvironment | An application that creates a CCamera object must have the UserEnvironment capability. |
static IMPORT_C CCamera *NewL(MCameraObserver &aObserver, TInt aCameraIndex);
Creates an object representing a camera.
Note: Applications using this method to create camera object may not receive enums/uids added in future(after being baselined).
To receive them, they should rather use New2L()
or NewDuplicate2L()
, in which case, they should prepare themselves to receive unrecognised values.
|
|
|
static IMPORT_C TInt CamerasAvailable();
Determines the number of cameras on the device.
|
Capability: | MultimediaDD | A process requesting or using this method that has MultimediaDD capability will always have precedence over a process that does not have MultimediaDD. |
Capability: | UserEnvironment | An application that creates a CCamera object must have the UserEnvironment capability. |
static IMPORT_C CCamera *New2L(MCameraObserver2 &aObserver, TInt aCameraIndex, TInt aPriority);
Creates an object representing a camera. Clients prepare themselves to receive unrecognised enum, uids etc.
Note: Clients using this creation method should prepare themselves to receive any unrecognised enum values, uids from 'supported' or 'getter' methods
|
|
|
Capability: | UserEnvironment | An application that creates a CCamera object must have the UserEnvironment capability. |
static IMPORT_C CCamera *NewDuplicateL(MCameraObserver2 &aObserver, TInt aCameraHandle);
Duplicates the original camera object for use by, for example, multimedia systems.
May leave with KErrNoMemory or KErrNotFound if aCameraHandle is not valid.
Note: Applications using this method to create camera object may not receive enums/uids added in future(after being baselined).
To receive them, they should rather use New2L()
or NewDuplicate2L()
, in which case, they should prepare themselves to receive unrecognised values.
|
|
|
Capability: | UserEnvironment | An application that creates a CCamera object must have the UserEnvironment capability. |
static IMPORT_C CCamera *NewDuplicate2L(MCameraObserver2 &aObserver, TInt aCameraHandle);
Duplicates the original camera object for use by, for example, multimedia systems. Clients prepare themselves to receive unrecognised enum, uids etc.
May leave with KErrNoMemory or KErrNotFound if aCameraHandle is not valid.
Note: Clients using this creation method should prepare themselves to receive any unrecognised enum values, uids from 'supported' or 'getter' methods
|
|
|
Capability: | UserEnvironment | An application that creates a CCamera object must have the UserEnvironment capability. |
static IMPORT_C CCamera *NewDuplicateL(MCameraObserver &aObserver, TInt aCameraHandle);
Duplicates the original camera object for use by, for example, multimedia systems.
Note: Applications using this method to create camera object may not receive enums/uids added in future(after being baselined).
To receive them, they should rather use New2L()
or NewDuplicate2L()
, in which case, they should prepare themselves to receive unrecognised values.
|
|
|
virtual void CameraInfo(TCameraInfo &aInfo) const=0;
Gets information about the camera device.
|
virtual void Reserve()=0;
Asynchronous function that performs any required initialisation and reserves the camera for exclusive use.
Calls MCameraObserver
:: ReserveComplete() when complete.
virtual void Release()=0;
De-initialises the camera, allowing it to be used by other clients.
virtual void PowerOn()=0;
Asynchronous method to switch on camera power.
User
must have successfully called Reserve()
prior to calling this function.
Calls MCameraObserver::PowerOnComplete()
when power on is complete.
virtual TInt Handle()=0;
Gets the device-unique handle of this camera object.
|
virtual void SetZoomFactorL(TInt aZoomFactor=0)=0;
Sets the zoom factor.
This must be in the range of TCameraInfo::iMinZoom
to TCameraInfo::iMaxZoom
inclusive. May leave with KErrNotSupported if the specified zoom factor is out of range.
|
virtual TInt ZoomFactor() const=0;
Gets the currently set zoom factor.
|
virtual void SetDigitalZoomFactorL(TInt aDigitalZoomFactor=0)=0;
Sets the digital zoom factor.
This must be in the range of 0 to TCameraInfo::iMaxDigitalZoom
inclusive.
May leave with KErrNotSupported if the zoom factor is out of range.
|
virtual TInt DigitalZoomFactor() const=0;
Gets the currently set digital zoom factor.
|
virtual void SetContrastL(TInt aContrast)=0;
Sets the contrast adjustment of the device.
This must be in the range of -100 to +100 or EContrastAuto. May leave with KErrNotSupported if the specified contrast value is out of range.
|
virtual TInt Contrast() const=0;
Gets the currently set contrast value.
|
virtual void SetBrightnessL(TInt aBrightness)=0;
Sets the brightness adjustment of the device.
No effect if this is not supported, see TCameraInfo::EBrightnessSupported
.
This must be in the range of -100 to +100 or EBrightnessAuto. May leave with KErrNotSupported if the brightness adjustment is out of range.
|
virtual TInt Brightness() const=0;
Gets the currently set brightness adjustment value.
|
virtual void SetFlashL(TFlash aFlash=EFlashNone)=0;
Sets the flash mode.
No effect if this is not supported, see TCameraInfo::iFlashModesSupported
.
May leave with KErrNotSupported if the specified flash mode is invalid.
|
virtual TFlash Flash() const=0;
Gets the currently set flash mode.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera object, it is assumed that application is not prepared to receive extra added enum values (unrecognised).
So, any extra enum value(unrecognised) (set in the ECAM implementation because of sharing clients) should not be returned
from the ECAM implementation. To receive extra added enum values, application should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. ECAM implementation, after verifying this,(by checking version no.) may send new values, if set.
In this case, application is assumed to be prepared to receive unrecognised enum values.
|
virtual void SetExposureL(TExposure aExposure=EExposureAuto)=0;
Sets the exposure adjustment of the device.
No effect if this is not supported, see CameraInfo::iExposureModesSupported.
May leave with KErrNotSupported if the specified exposure adjustment is invalid.
|
virtual TExposure Exposure() const=0;
Gets the currently set exposure setting value.
|
virtual void SetWhiteBalanceL(TWhiteBalance aWhiteBalance=EWBAuto)=0;
Sets the white balance adjustment of the device.
No effect if this is not supported, see TCameraInfo::iWhiteBalanceModesSupported
.
|
|
virtual TWhiteBalance WhiteBalance() const=0;
Gets the currently set white balance adjustment value.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera object, it is assumed that application is not prepared to receive extra added enum values (unrecognised).
So, any extra enum value(unrecognised) (set in the ECAM implementation because of sharing clients) should not be returned
from the ECAM implementation. To receive extra added enum values, application should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. ECAM implementation, after verifying this,(by checking version no.) may send new values, if set.
In this case, application is assumed to be prepared to receive unrecognised enum values. Refer CCamera::CCameraAdvancedSettings::WhiteBalanceMode()
implementation
|
virtual void StartViewFinderDirectL(RWsSession &aWs, CWsScreenDevice &aScreenDevice, RWindowBase &aWindow, TRect &aScreenRect)=0;
Starts transfer of view finder data to the given portion of the screen using direct screen access.
The aScreenRect parameter is in screen co-ordinates and may be modified if, eg, the camera requires the destination to have a certain byte alignment, etc.
|
|
virtual void StartViewFinderDirectL(RWsSession &aWs, CWsScreenDevice &aScreenDevice, RWindowBase &aWindow, TRect &aScreenRect,
TRect &aClipRect)=0;
Starts transfer of view finder data to the given portion of the screen using direct screen access and also clips to the specified portion of the screen.
The view finder has the same size and position as aScreenRect but is only visible in the intersection of aScreenRect and aClipRect.
May leave with KErrNotSupported or KErrNotReady if Reserve()
has not been called, or has not yet completed.
|
|
virtual void StartViewFinderBitmapsL(TSize &aSize)=0;
Starts transfer of view finder data.
Bitmaps are returned by MCameraObserver::ViewFinderFrameReady()
.
|
|
virtual void StartViewFinderBitmapsL(TSize &aSize, TRect &aClipRect)=0;
Starts transfer of view finder data and clips the bitmap to the specified clip rectangle.
The bitmap is the size of the intersection of aSize and aClipRect, not simply aSize padded with white space.
|
|
virtual void StartViewFinderL(TFormat aImageFormat, TSize &aSize)=0;
Starts transfer of view finder data.
Picture data is returned by MCameraObserver2::ViewFinderReady()
.
|
|
virtual void StartViewFinderL(TFormat aImageFormat, TSize &aSize, TRect &aClipRect)=0;
Starts transfer of view finder data and clips the picture to the specified clip rectangle. Picture data is returned by MCameraObserver2::ViewFinderReady()
.
The picture is the size of the intersection of aSize and aClipRect, not simply aSize padded with white space.
|
|
virtual TBool ViewFinderActive() const=0;
Queries whether the view finder is active.
|
virtual void SetViewFinderMirrorL(TBool aMirror)=0;
Sets whether view finder mirroring is on.
Used to switch between what the camera sees and what you would see if the device were a mirror.
|
|
virtual TBool ViewFinderMirror() const=0;
Gets whether view finder mirroring is active.
|
virtual void PrepareImageCaptureL(TFormat aImageFormat, TInt aSizeIndex)=0;
Performs setup and allocation of memory.
Called prior to calling CaptureImage()
to keep the latency of that function to a minimum.
Needs to be called only once for multiple CaptureImage()
calls. May leave with KErrNotSupported or KErrNoMemory or KErrInUse or KErrNotReady.
The specified image format must be one of the formats supported (see TCameraInfo::iImageFormatsSupported
).
The specified size index must be in the range of 0 to TCameraInfo::iNumImageSizesSupported
-1 inclusive.
|
|
virtual void PrepareImageCaptureL(TFormat aImageFormat, TInt aSizeIndex, const TRect &aClipRect)=0;
Performs setup and allocation of memory and clips the image to the specified rectangle.
No effect unless TCameraInfo::iImageClippingSupported is set to ETrue. The image captured is the intersection of aClipRect
and the rectangle from (0,0) to aSize. Needs to be called only once for multiple CaptureImage()
calls. May leave with KErrNotSupported or KErrNoMemory.
The specified image format must be one of the formats supported (see TCameraInfo::iImageFormatsSupported
).
The specified size index must be in the range of 0 to TCameraInfo::iNumImageSizesSupported
-1 inclusive.
|
|
virtual void CaptureImage()=0;
Asynchronously performs still image capture.
Calls MCameraObserver::ImageReady()
when complete.
virtual void EnumerateCaptureSizes(TSize &aSize, TInt aSizeIndex, TFormat aFormat) const=0;
Enumerates through the available image capture sizes, based on the specified size index and format
The size index must be in the range 0 to TCameraInfo::iNumImageSizesSupported
-1 inclusive.
|
virtual void PrepareVideoCaptureL(TFormat aFormat, TInt aSizeIndex, TInt aRateIndex, TInt aBuffersToUse, TInt aFramesPerBuffer)=0;
Prepares for video capture.
Performs setup and allocation of memory prior to calling StartVideoCapture()
to keep the latency of that function to a minimum.
May leave with KErrNotSupported or KErrNoMemory.
|
|
virtual void PrepareVideoCaptureL(TFormat aFormat, TInt aSizeIndex, TInt aRateIndex, TInt aBuffersToUse, TInt aFramesPerBuffer,
const TRect &aClipRect)=0;
Prepares for video capture and clips the frames to the given rectangle.
Performs setup and allocation of memory prior to calling StartVideoCapture()
to keep the latency of that function to a minimum.
May leave with KErrNotSupported or KErrNoMemory.
|
|
virtual void StartVideoCapture()=0;
Starts capturing video.
Calls MCameraObserver::FrameBufferReady()
when each buffer has been filled with the required number of frames, as set by PrepareVideoCaptureL()
.
virtual TBool VideoCaptureActive() const=0;
Tests whether video capture is active.
|
virtual void EnumerateVideoFrameSizes(TSize &aSize, TInt aSizeIndex, TFormat aFormat) const=0;
Enumerates through the available video frame sizes, based on the specified size index and format.
|
virtual void EnumerateVideoFrameRates(TReal32 &aRate, TInt aRateIndex, TFormat aFormat, TInt aSizeIndex, TExposure aExposure=EExposureAuto)
const=0;
Enumerates through the available video frame rates, based on the specified rate index, video frame format, size index and exposure mode.
|
virtual void GetFrameSize(TSize &aSize) const=0;
Gets the frame size currently in use.
|
virtual TReal32 FrameRate() const=0;
Gets the frame rate currently in use.
|
virtual TInt BuffersInUse() const=0;
Gets the number of buffers currently in use.
|
virtual TInt FramesPerBuffer() const=0;
Gets the number of frames per buffer currently in use.
|
virtual void SetJpegQuality(TInt aQuality)=0;
Sets the quality value to use if jpeg is a supported image for video format.
Ignored if jpeg is not a supported image for video format.
|
virtual TInt JpegQuality() const=0;
Gets the currently set jpeg quality value.
Returns 0 if not supported.
|
virtual TAny *CustomInterface(TUid aInterface)=0;
Gets a custom interface. The client has to cast the returned pointer to the appropriate type.
|
|
class CCameraAdvancedSettings : public CBase;
CCamera
advanced settings class exposes an API for controlling individually digital camera advanced settings. These settings directly
relate to the image acquisition phase both for still images and video.
Note: This class is not intended for sub-classing and used to standardise existing varieties of implementations.
CBase
- Base class for all classes to be instantiated on the heap
CCamera::CCameraAdvancedSettings
-
Defined in CCamera::CCameraAdvancedSettings
:
Aperture()
, ApertureExposureLockOn()
, AutoFocusArea()
, AutoFocusLockOn()
, AutoFocusType()
, AutomaticSizeSelectionChangeOn()
, BracketMode()
, BracketParameter()
, BracketParameterAperture
, BracketParameterAutoFocus
, BracketStep()
, BurstImages()
, CameraIndex()
, CameraType()
, CameraType()
, ContinuousAutoFocusTimeout()
, DigitalZoom()
, DriveMode()
, EAutoFocusTypeAuto
, EAutoFocusTypeContinuous
, EAutoFocusTypeMultiAreaCentered
, EAutoFocusTypeOff
, EAutoFocusTypeSingle
, EAutoFocusTypeSingleArea
, EBracketMode3Image
, EBracketMode5Image
, EBracketModeOff
, EBracketParameterColourBalance
, EBracketParameterExposure
, EBracketParameterFlashPower
, EBracketParameterNone
, EBracketStepLarge
, EBracketStepMedium
, EBracketStepNonConfig
, EBracketStepSmall
, ECameraOnBoard
, ECameraPluggable
, ECameraUnknown
, EDriveModeAuto
, EDriveModeBracket
, EDriveModeBracketMerge
, EDriveModeBurst
, EDriveModeContinuous
, EDriveModeSingleShot
, EDriveModeTimeLapse
, EDriveModeTimed
, EEPixelAspect10To11
, EEPixelAspect11To10
, EEPixelAspect16To11
, EEPixelAspect40To33
, EEPixelAspect59To54
, EFocusModeAuto
, EFocusModeFixed
, EFocusModeManual
, EFocusModeUnknown
, EFocusRangeAuto
, EFocusRangeMacro
, EFocusRangeNormal
, EFocusRangePortrait
, EFocusRangeSuperMacro
, EFocusRangeTele
, EMeteringModeAuto
, EMeteringModeCenterWeighted
, EMeteringModeEvaluative
, EMeteringModeSpot
, EPictureOrientationLandscape
, EPictureOrientationPortrait
, EPictureOrientationSquare
, EPictureOrientationUnknown
, EPixelAspect12To11
, EPixelAspect1To1
, EPixelAspectUnknown
, EStabilizationAuto
, EStabilizationComplexityAuto
, EStabilizationComplexityHigh
, EStabilizationComplexityLow
, EStabilizationComplexityMedium
, EStabilizationFine
, EStabilizationMedium
, EStabilizationModeAuto
, EStabilizationModeHorizontal
, EStabilizationModeManual
, EStabilizationModeOff
, EStabilizationModeRotation
, EStabilizationModeVertical
, EStabilizationOff
, EStabilizationStrong
, EWBColorTemperature
, EWBRgb
, EWBUnknown
, EYuvRangeFull
, EYuvRangeUnknown
, EYuvRangeVideoCropped
, ExposureCompensation()
, ExposureCompensationStep()
, ExposureLockOn()
, ExposureMode()
, FlashCompensation()
, FlashCompensationStep()
, FlashMode()
, FocusDistance()
, FocusMode()
, FocusRange()
, GetActiveSettingsL()
, GetAperturesL()
, GetBracketMerge()
, GetCurrentFocusModeStepsL()
, GetDigitalZoomStepsL()
, GetDisabledSettingsL()
, GetExposureCompensationRangeInSteps()
, GetExposureCompensationStepsL()
, GetFlashCompensationRangeInSteps()
, GetFlashCompensationStepsL()
, GetManualFlashPowerLevelsL()
, GetMinFocalLength()
, GetOpticalZoomStepsL()
, GetShutterSpeedsL()
, GetSupportedContinuousAutoFocusTimeoutsL()
, GetSupportedIsoRatesL()
, GetSupportedSettingsL()
, GetTimeLapse()
, GetTimeLapsePeriodRange()
, GetTimerIntervalsL()
, GetWBRgbValue()
, GetWBSupportedColorTemperaturesL()
, IsCameraPresent()
, IsCameraPresent()
, IsExternalFlashPresent()
, IsFlashReady()
, IsoRate()
, ManualFlashPowerLevel()
, MeteringMode()
, NewL()
, OpticalZoom()
, PictureOrientation()
, PixelAspectRatio()
, RedEyeReduceOn()
, SetAperture()
, SetApertureExposureLockOn()
, SetAutoFocusArea()
, SetAutoFocusLockOn()
, SetAutoFocusType()
, SetAutomaticSizeSelectionChangeOn()
, SetBracketMerge()
, SetBracketMode()
, SetBracketParameter()
, SetBracketStep()
, SetBurstImages()
, SetContinuousAutoFocusTimeout()
, SetDigitalZoom()
, SetDriveMode()
, SetExposureCompensation()
, SetExposureCompensationStep()
, SetExposureLockOn()
, SetExposureMode()
, SetFlashCompensation()
, SetFlashCompensationStep()
, SetFlashMode()
, SetFocusDistance()
, SetFocusMode()
, SetFocusRange()
, SetIsoRate()
, SetManualFlashPowerLevel()
, SetMeteringMode()
, SetOpticalZoom()
, SetPictureOrientation()
, SetPixelAspectRatio()
, SetRedEyeReduceOn()
, SetShootClickOn()
, SetShutterSpeed()
, SetStabilizationComplexity()
, SetStabilizationEffect()
, SetStabilizationMode()
, SetTimeLapse()
, SetTimerInterval()
, SetWBColorTemperature()
, SetWBRgbValue()
, SetWhiteBalanceMode()
, SetYuvRange()
, ShootClickOn()
, ShutterSpeed()
, StabilizationComplexity()
, StabilizationEffect()
, StabilizationMode()
, SupportedAutoFocusAreas()
, SupportedAutoFocusTypes()
, SupportedBracketModes()
, SupportedBracketParameters()
, SupportedBracketSteps()
, SupportedDriveModes()
, SupportedExposureModes()
, SupportedFlashModes()
, SupportedFocusModes()
, SupportedFocusRanges()
, SupportedMeteringModes()
, SupportedPixelAspectRatios()
, SupportedStabilizationComplexityValues()
, SupportedStabilizationEffects()
, SupportedStabilizationModes()
, SupportedWBUnits()
, SupportedWhiteBalanceModes()
, SupportedYuvRanges()
, TAutoFocusArea
, TAutoFocusType
, TBracketMode
, TBracketParameter
, TBracketStep
, TCameraType
, TDriveMode
, TFocusMode
, TFocusRange
, TMeteringMode
, TPictureOrientation
, TPixelAspectRatio
, TStabilizationAlgorithmComplexity
, TStabilizationEffect
, TStabilizationMode
, TWBUnits
, TYuvRange
, TimerInterval()
, WBColorTemperature()
, WhiteBalanceMode()
, YuvRange()
, ~CCameraAdvancedSettings()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
NewL()
static IMPORT_C CCameraAdvancedSettings *NewL(CCamera &aCamera);
Factory function for creating the CCameraAdvancedSettings
object.
Note: Clients using MCameraObserver
are not recommended to use this extension class since they cannot handle events.
|
|
|
~CCameraAdvancedSettings()
IMPORT_C ~CCameraAdvancedSettings();
Destructor
CameraType()
IMPORT_C TCameraType CameraType() const;
Gets the type of this camera.
|
CameraType()
IMPORT_C TCameraType CameraType(TInt aCameraIndex);
Get the type of a specific camera denoted by its index. A pluggable camera may not necessarily be physically present. The type denotes whether the slot allocated to that index is for pluggable or onboard camera.
|
|
IsCameraPresent()
IMPORT_C TBool IsCameraPresent() const;
Checks whether the current camera is present.
|
IsCameraPresent()
IMPORT_C TBool IsCameraPresent(TInt aCameraIndex);
Checks whether the camera, denoted by its index, is currently present. The index uniquely identifies the camera on the device.
|
|
CameraIndex()
IMPORT_C TInt CameraIndex() const;
Gets current camera index. The index uniquely identifies the camera on the device.
|
SupportedStabilizationModes()
IMPORT_C TInt SupportedStabilizationModes() const;
Gets all of the supported stabilization modes on the camera. The result is a bitfield of the valid TStabilizationMode flags.
|
StabilizationMode()
IMPORT_C TStabilizationMode StabilizationMode() const;
Gets current stabilization mode on the camera. The result is a valid TStabilizationMode value.
|
SetStabilizationMode()
IMPORT_C void SetStabilizationMode(TStabilizationMode aStabilizationMode);
Sets a specific stabilization mode on the camera.
Stabilization mode change fires a KUidECamEventCameraSettingStabilizationMode event to all MCameraObserver2
clients of this specific camera.
|
SupportedFocusModes()
IMPORT_C TInt SupportedFocusModes() const;
Gets all of the supported focus modes on the camera. The result is a bitfield of the valid TFocusMode flags.
|
FocusMode()
IMPORT_C TFocusMode FocusMode() const;
Gets current focus mode on the camera. The result is a valid TFocusMode value.
|
SetFocusMode()
IMPORT_C void SetFocusMode(TFocusMode aFocusMode);
Sets a specific focus mode on the camera. Focus mode change fires a KUidECamEventCameraSettingFocusMode event to all MCameraObserver2
clients of the camera.
|
SupportedFocusRanges()
IMPORT_C TInt SupportedFocusRanges() const;
Gets all supported focus ranges on the camera.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added enum values. So, any extra enum value passed
from the implementation will be filtered at this point. To receive extra added enum values, application should rather use
CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised enum values
|
FocusRange()
IMPORT_C TFocusRange FocusRange() const;
Gets current focus range on the camera.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added enum values. So, any extra enum value received
from the implementation will be dropped and EFocusRangeAuto would be passed instead. To receive extra added enum values, application
should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised enum values
|
SetFocusRange()
IMPORT_C void SetFocusRange(TFocusRange aFocusRange);
Sets a specific focus range on the camera. The focus range change fires both, KUidECamEventCameraSettingFocusRange and KUidECamEventCameraSettingFocusRange2
event to all MCameraObserver2
clients of the camera.
|
SupportedAutoFocusTypes()
IMPORT_C TInt SupportedAutoFocusTypes() const;
Gets all supported auto focus types on the camera.
|
AutoFocusType()
IMPORT_C TAutoFocusType AutoFocusType() const;
Gets current auto focus type on the camera.
|
SetAutoFocusType()
IMPORT_C void SetAutoFocusType(TAutoFocusType aAutoFocusType);
Sets a specific auto focus type on the camera. The focus type change fires both, KUidECamEventCameraSettingAutoFocusType and
KUidECamEventCameraSettingAutoFocusType2 event to all MCameraObserver2
clients of the camera.
|
SupportedAutoFocusAreas()
IMPORT_C TInt SupportedAutoFocusAreas() const;
Gets all supported auto focus areas on the camera.
|
AutoFocusArea()
IMPORT_C TAutoFocusArea AutoFocusArea() const;
Gets current chosen auto focus area on the camera.
|
SetAutoFocusArea()
IMPORT_C void SetAutoFocusArea(TAutoFocusArea aAutoFocusArea);
Sets a specific auto focus area on the camera. Focus area change fires a KUidECamEventCameraSettingAutoFocusArea event to
all MCameraObserver2
clients of the camera.
|
FocusDistance()
IMPORT_C TInt FocusDistance() const;
Get focus distance in millimetres.
|
SetFocusDistance()
IMPORT_C void SetFocusDistance(TInt aDistance);
Set focus distance in millimetres. Focus distance change fires a KUidECamEventCameraSettingFocusDistance event to all MCameraObserver2
clients of the camera.
|
GetMinFocalLength()
IMPORT_C TInt GetMinFocalLength() const;
Get minimum focus distance in millimetres
Note: Current Focal length is calculated as focalLength = opticalZoom * minFocalLength;
|
GetSupportedIsoRatesL()
IMPORT_C void GetSupportedIsoRatesL(RArray< TInt > &aSupportedIsoRates) const;
Gets the set of camera supported ISO rates.
Note: When camera device is incapable of revealing the ISO rates supported, it has to be assumed that camera will work only on the permanently set value. If this value is not known, empty array may be returned; corresponding getter/setters for this feature should not be used in such a case.
|
IsoRate()
IMPORT_C TInt IsoRate() const;
Gets current ISO rate.
Note: The returned value may be checked with the list of supported ISO rates. If value returned does not belong to this list, then it may be treated as an error case.
|
SetIsoRate()
IMPORT_C void SetIsoRate(TInt aRate);
Set current ISO rate for the camera. Triggers KUidECamEventCameraSettingIsoRate to all MCameraObserver2
clients of the camera.
|
GetAperturesL()
IMPORT_C void GetAperturesL(RArray< TInt > &aFStops, TValueInfo &aInfo) const;
Gets the current discrete aperture steps (F-stops) supported by the camera.
Note: When camera device is incapable of revealing the aperture openings supported, it has to be assumed that camera will work only on the parmanently set value. If this value is not known, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
|
Aperture()
IMPORT_C TInt Aperture() const;
Get current aperture value.
|
SetAperture()
IMPORT_C void SetAperture(TInt aFStop);
Set a new aperture value. All MCameraObserver2
clients of the camera receive a KUidECamEventCameraSettingAperture event notification when aperture value is changed.
Note: The aperture parameter value is an integer, multiplied by KECamFineResolutionFactor. For example to set an aperture of F2.8, call SetAperture(280).
|
GetShutterSpeedsL()
IMPORT_C void GetShutterSpeedsL(RArray< TInt > &aShutterSpeeds, TValueInfo &aInfo) const;
Gets the set of supported shutter speeds.
Note: When camera device is incapable of revealing the shutter speeds supported, it has to be assumed that camera will work only on the parmanently set value. If this value is not known, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
|
ShutterSpeed()
IMPORT_C TInt ShutterSpeed() const;
Gets the current shutter speed
|
SetShutterSpeed()
IMPORT_C void SetShutterSpeed(TInt aShutterSpeed);
Sets the current shutter speed. When set, all MCameraObserver2
clients of the camera receive a KUidECamEventCameraSettingShutterSpeed event
|
SupportedMeteringModes()
IMPORT_C TInt SupportedMeteringModes() const;
Get all supported metering modes on this camera represented as bitfield of type TMeteringMode.
|
MeteringMode()
IMPORT_C TMeteringMode MeteringMode() const;
Get current metering mode.
|
SetMeteringMode()
IMPORT_C void SetMeteringMode(TMeteringMode aMeteringMode);
Set the current metering mode. When set, all MCameraObserver2
clients are notified with a KUidECamEventCameraSettingMeteringMode event.
|
SupportedDriveModes()
IMPORT_C TInt SupportedDriveModes() const;
Get all supported drive modes as bitfields of TDriveMode type.
|
DriveMode()
IMPORT_C TDriveMode DriveMode() const;
Gets currently active drive mode.
|
SetDriveMode()
IMPORT_C void SetDriveMode(TDriveMode aDriveMode);
Set the current metering mode. When set all MCameraObserver2
clients are notified with KUidECamEventCameraSettingDriveMode.
|
SupportedBracketModes()
IMPORT_C TInt SupportedBracketModes() const;
Get all supported bracket modes as bitfields.
|
BracketMode()
IMPORT_C TBracketMode BracketMode() const;
Get current bracket mode.
|
SetBracketMode()
IMPORT_C void SetBracketMode(TBracketMode aBracketMode);
Set new bracket mode. All MCameraObserver2
clients are notified with KUidECamEventCameraSettingBracketMode.
|
SupportedBracketParameters()
IMPORT_C TInt SupportedBracketParameters() const;
Get all supported bracket parameters as bitfields.
|
BracketParameter()
IMPORT_C TBracketParameter BracketParameter() const;
Get current bracket parameter.
|
SetBracketParameter()
IMPORT_C void SetBracketParameter(TBracketParameter aBracketParameter);
Set new bracket parameter When set all clients are notified with KUidECamEventCameraSettingBracketParameter.
|
SupportedBracketSteps()
IMPORT_C TInt SupportedBracketSteps() const;
Get all supported bracket steps as bitfields.
|
BracketStep()
IMPORT_C TBracketStep BracketStep() const;
Get current bracket step.
|
SetBracketStep()
IMPORT_C void SetBracketStep(TBracketStep aBracketStep);
Set new bracket step. All MCameraObserver2
clients are notified with KUidECamEventCameraSettingBracketStep.
|
GetBracketMerge()
IMPORT_C void GetBracketMerge(TInt &aStartIndex, TInt &aFrames) const;
Gets the settings for which frames to merge. Valid only in EDriveModeBracketMerge mode.
Note: there must be at least two images to merge. All are assumed to form a sequence and are identified using the first frame index and number of frames e.g. to merge two frames - one on and one +1, when in EBracketMode3Image, one sets the start index to 1 and frames to two.
Note: It is very much TBracketMode setting dependent operation.
|
SetBracketMerge()
IMPORT_C void SetBracketMerge(TInt aStartIndex, TInt aFrames);
Sets the settings for which frames to merge. Valid only in EDriveModeBracketMerge mode.
Note: there must be at least two images to merge. All are assumed to form a sequence and are identified using the first frame
index and number of frames e.g. to merge two frames - one on and one +1, when in EBracketMode3Image, one sets the start index
to 1 and frames to 2. MCameraObserver2
clients are notified with a KUidECamEventBracketMerge event.
Note: It is very TBracketMode setting dependent.
|
SupportedFlashModes()
IMPORT_C TInt SupportedFlashModes() const;
Get camera all supported flash modes CCamera::TFlash
Note: Some of the flash modes are available only for clients using either CCamera::New2L()
or CCamera::NewDuplicate2L()
|
FlashMode()
IMPORT_C CCamera::TFlash FlashMode() const;
Gets the currently set flash mode.
Note: Clients not using the CCamera::New2L()
or CCamera::NewDuplicate2L()
would be given CCamera::EFlashAuto
if current flash mode exceeds CCamera::EFlashManual
|
SetFlashMode()
IMPORT_C void SetFlashMode(CCamera::TFlash aMode);
Sets the flash mode.
Triggers a KUidECamEventCameraSettingFlashMode event to all camera MCameraObserver2
clients.
|
RedEyeReduceOn()
IMPORT_C TBool RedEyeReduceOn() const;
Gets whether the flash red eye reduction is switched on.
|
SetRedEyeReduceOn()
IMPORT_C void SetRedEyeReduceOn(TBool aState);
Sets the flash red eye reduction on or off.
Triggers a KUidECamEventCameraSettingFlashRedEyeReduceOn event to all camera MCameraObserver2
clients.
|
GetFlashCompensationStepsL()
IMPORT_C void GetFlashCompensationStepsL(RArray< TInt > &aFlashCompensationSteps, TValueInfo &aInfo) const;
Get flash compensation steps as integers multiplied by KECamFineResolutionFactor. For example 0.5 EV is 50.
Note: When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
Note: When camera device is incapable of revealing the flash compensation steps supported, it has to be assumed that camera will work only on the parmanently set value. If this value is not known, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
|
FlashCompensationStep()
IMPORT_C TInt FlashCompensationStep() const;
Get current flash power compensation step.
|
SetFlashCompensationStep()
IMPORT_C void SetFlashCompensationStep(TInt aFlashCompensationStep);
Set current flash compensation step as an integer multiplied by KECamFineResolutionFactor. For example to set a compensation of -0.3 EV, one should use a parameter with value -30. All clients receive a KUidECamEventCameraSettingFlashCompensationStep event, when the value has changed.
|
GetFlashCompensationRangeInSteps()
IMPORT_C void GetFlashCompensationRangeInSteps(TInt &aNegativeCompensation, TInt &aPositiveCompensation) const;
Get current flash power compensation range measured in a already selected compensation step magnitude.
Note: This range may change if a different compensation step is selected. For example if flash compensation range is in the range -1EV 1.5EV and the selected flash compensation step is selected to be 0.3 EV, the result of this call will be aNegativeCompensation = -3 and aPositiveCompensation = 5. as there can be only three full steps for negative compensation (1/0.3) and five for flash power boost (1.5/0.3). In this way developers, having pre-selected a step value from the supported set, would need to provide just the multiplier (in steps) and the direction (the sign). Steps are always assumed integers.
|
FlashCompensation()
IMPORT_C TInt FlashCompensation() const;
Get the current flash compensation value as integer steps. Positive values boost flash power, negative reduce flash power. The change is not cumulative i.e. the change is stateless. Each call assumes no previous compensation has been performed i.e. that there is a zero compensation.
Note: if returned value is 2 (compensation steps) and the current flash compensation step is 0.3 EV, then the actual compensation effect will be 0.6 EV.
|
SetFlashCompensation()
IMPORT_C void SetFlashCompensation(TInt aFlashCompensationInSteps);
Set the current flash compensation value as integer steps. Positive values increase power, negative reduce power. The change is not acumulative e.g. the change is stateless. Each call assumes no previous compensation has been performed i.e. that there is a zero compensation. Triggers a KUidECamEventCameraSettingFlashCompensation event.
|
IsExternalFlashPresent()
IMPORT_C TBool IsExternalFlashPresent() const;
Check whether there is an external flash source.
|
GetManualFlashPowerLevelsL()
IMPORT_C void GetManualFlashPowerLevelsL(RArray< TInt > &aManualFlashPowerLevels, TValueInfo &aInfo) const;
Gets the current discrete manual flash power levels supported by the camera in range 0-100 as a percentage of maximum power level.
Note: When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case. When camera device is incapable of revealing the manual flash power levels supported, it has to be assumed that camera will work only on the permanently set value. If this value is not known, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
|
ManualFlashPowerLevel()
IMPORT_C TInt ManualFlashPowerLevel() const;
Gets the current manual flash power level on the camera.
|
SetManualFlashPowerLevel()
IMPORT_C void SetManualFlashPowerLevel(TInt aManualFlashPowerLevel);
Sets the current manual flash power level on the camera. Triggers a KUidECamEventCameraSettingManualFlashPower event to all
MCameraObserver2
clients.
|
SupportedExposureModes()
IMPORT_C TInt SupportedExposureModes() const;
Get Supported exposure modes - bitfields of CCamera::TExposure
|
ExposureMode()
IMPORT_C CCamera::TExposure ExposureMode() const;
Gets the currently set exposure setting value.
|
SetExposureMode()
IMPORT_C void SetExposureMode(CCamera::TExposure aExposureMode);
Sets the exposure mode of the camera. Triggers a KUidECamEventCameraSettingExposure event to all MCameraObserver2
clients.
|
GetExposureCompensationStepsL()
IMPORT_C void GetExposureCompensationStepsL(RArray< TInt > &aExposureCompensationSteps, TValueInfo &aInfo) const;
Get exposure compensation steps as integers multiplied by KECamFineResolutionFactor. For example 0.3 EV is 30.
Note: When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case. When camera device is incapable of revealing the exposure compensation steps supported, it has to be assumed that camera will work only on the permanently set value. If this value is not known, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
|
ExposureCompensationStep()
IMPORT_C TInt ExposureCompensationStep() const;
Get current exposure compensation step.
|
SetExposureCompensationStep()
IMPORT_C void SetExposureCompensationStep(TInt aExposureCompensationStep);
Set current exposure compensation step as an integer multiplied by KECamFineResolutionFactor. All MCameraObserver2
clients receive a KUidECamEventCameraSettingExposureCompensationStep event, when the value has changed.
|
GetExposureCompensationRangeInSteps()
IMPORT_C void GetExposureCompensationRangeInSteps(TInt &aNegativeCompensation, TInt &aPositiveCompensation) const;
Get current exposure compensation range in steps. It depends on the previously selected exposure compensation step.
|
ExposureCompensation()
IMPORT_C TInt ExposureCompensation() const;
Get the current exposure compensation steps. Positive values increase exposure times, negative reduce exposure times. The change is not cumulative i.e. the change is stateless. Each call assumes no previous compensation has been performed i.e. that there is a zero compensation.
Note: if returned value is 2 (compensation steps) and the current exposure compensation step is 0.3 EV, then the actual compensation effect will be 0.6 EV.
|
SetExposureCompensation()
IMPORT_C void SetExposureCompensation(TInt aExposureCompensationInSteps);
Set the current exposure compensation value as integer steps. Triggers a KUidECamEventCameraSettingExposureCompensation event
to all MCameraObserver2
clients.
|
SupportedWhiteBalanceModes()
IMPORT_C TInt SupportedWhiteBalanceModes() const;
Gets camera supported set of white balance adjustments.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added enum values. So, any extra enum value(unrecognised)
passed from the implementation will be filtered at this point. To receive extra added enum values, application should rather
use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised enum values
|
WhiteBalanceMode()
IMPORT_C CCamera::TWhiteBalance WhiteBalanceMode() const;
Gets the current white balance value.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added enum values. So, any extra enum value(unrecognised)
received from the implementation will be dropped and EWBAuto would be passed instead. To receive extra added enum values,
application should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised enum values
|
SetWhiteBalanceMode()
IMPORT_C void SetWhiteBalanceMode(CCamera::TWhiteBalance aWhiteBalanceMode);
Sets the white balance adjustment of the camera.
No effect if this is not supported, see TCameraInfo::iWhiteBalanceModesSupported
. Triggers KUidECamEventCameraSettingWhiteBalance event to all MCameraObserver2
clients.
|
ApertureExposureLockOn()
IMPORT_C TBool ApertureExposureLockOn() const;
Gets the current state for aperture and exposure lock.
|
SetApertureExposureLockOn()
IMPORT_C void SetApertureExposureLockOn(TBool aAELock);
Sets the current state for aperture and exposure lock. Triggers a KUidECamEventAELock event to all MCameraObserver2
clients.
|
ShootClickOn()
IMPORT_C TBool ShootClickOn() const;
Gets the current state for button clicking sound effect.
|
SetShootClickOn()
IMPORT_C void SetShootClickOn(TBool aShootClickOn);
Sets the button clicking sound on /off. Triggers a KUidECamEventSoundClick event to all MCameraObserver2
clients.
|
GetTimerIntervalsL()
IMPORT_C void GetTimerIntervalsL(RArray< TInt > &aTimerIntervals, TValueInfo &aInfo) const;
Get camera supported timer values. Active only when drive mode EDriveModeTimed. Time
is in microseconds. As time interval is expected to be relatively short, integer value is considered sufficient.
Note: When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
TimerInterval()
IMPORT_C TInt TimerInterval() const;
Get current timer value. Active only when drive mode is EDriveModeTimed. Timer resolution is in microseconds.
|
SetTimerInterval()
IMPORT_C void SetTimerInterval(TInt aTimerInterval);
Set current timer value. Active only when drive mode EDriveModeTimed. This is the time interval (delay) in microseconds between
user pressing the button and image is taken. The setting of the value triggers a KUidECamEventCameraSettingTimerInterval event
to all MCameraObserver2
clients.
|
GetTimeLapsePeriodRange()
IMPORT_C void GetTimeLapsePeriodRange(TTime &aTimeLapseMin, TTime &aTimeLapseMax) const;
Get camera supported time lapse period range. Active only when drive mode EDriveModeTimeLapse. The time lapse is denoted as the uniform time period between consecutive frames.
|
GetTimeLapse()
IMPORT_C void GetTimeLapse(TTime &aStart, TTime &aEnd, TTime &aInterval) const;
Get current time lapse value. Active only when drive mode EDriveModeTimeLapse. The time lapse is denoted as the uniform time period between consecutive frames and operation is configurable by its start, end and a fixed interval.
|
SetTimeLapse()
IMPORT_C void SetTimeLapse(const TTime &aStart, const TTime &aEnd, const TTime &aInterval);
Set current time lapse value. Active only when drive mode EDriveModeTimeLapse. The time lapse is denoted as the uniform time
period between consecutive frames. Setting triggers a KUidECamEventCameraSettingTimeLapse event to all MCameraObserver2
camera clients.
|
PictureOrientation()
IMPORT_C TPictureOrientation PictureOrientation() const;
Get current picture orientation
|
SetPictureOrientation()
IMPORT_C void SetPictureOrientation(TPictureOrientation aOrientation);
Set a new picture orientation This triggers a KUidECamEventPictureOrientation event to all MCameraObserver2
clients.
|
SupportedPixelAspectRatios()
IMPORT_C TInt SupportedPixelAspectRatios() const;
Get supported pixel aspect ratio.
Note: Some of the pixel aspect ratios are available only for clients using either CCamera::New2L()
or CCamera::NewDuplicate2L()
Mask out new features for old clients
|
PixelAspectRatio()
IMPORT_C TPixelAspectRatio PixelAspectRatio() const;
Get current pixel aspect ratio.
Note: Some of the pixel aspect ratios are available only for clients using either CCamera::New2L()
or CCamera::NewDuplicate2L()
Mask out new features for old clients
|
SetPixelAspectRatio()
IMPORT_C void SetPixelAspectRatio(TPixelAspectRatio aPixelAspectRatio);
Set a new pixel aspect ratio. This triggers a KUidECamEventPixelAspectRatio event to all MCameraObserver2
clients.
|
SupportedYuvRanges()
IMPORT_C TInt SupportedYuvRanges() const;
Get supported YUV ranges.
|
YuvRange()
IMPORT_C TYuvRange YuvRange() const;
Get current YUV range.
|
SetYuvRange()
IMPORT_C void SetYuvRange(TYuvRange aYuvRange);
Set a new YUV range. This triggers a KUidECamEventYuvRange event to all MCameraObserver2
clients.
|
BurstImages()
IMPORT_C TInt BurstImages() const;
Get the number of images captured normally under EDriveModeBurst condition.
Note: : due to memory or image size limitations the actual number may be less.
|
SetBurstImages()
IMPORT_C void SetBurstImages(TInt aImages);
Set the number of images captured normally under EDriveModeBurst condition. Triggers a KUidECamEventBurstImages event to all
MCameraObserver2
clients.
Note: : due to memory or image size limitations the actual number may be less.
|
GetOpticalZoomStepsL()
IMPORT_C void GetOpticalZoomStepsL(RArray< TInt > &aOpticalZoomSteps, TValueInfo &aInfo) const;
Gets the optical zoom levels.
Note: Such approach allows for support for both linear and non-linear zoom steps. When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
|
OpticalZoom()
IMPORT_C TInt OpticalZoom() const;
Gets the currently set zoom value.
|
SetOpticalZoom()
IMPORT_C void SetOpticalZoom(TInt aOpticalZoom);
Sets the zoom using a specific value. Triggers a KUidECamEventCameraSettingOpticalZoom event to all MCameraObserver2
clients.
|
GetDigitalZoomStepsL()
IMPORT_C void GetDigitalZoomStepsL(RArray< TInt > &aDigitalZoomSteps, TValueInfo &aInfo) const;
Gets the digital zoom levels.
Note: Such approach allows for support for both linear and non-linear zoom steps. When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
|
DigitalZoom()
IMPORT_C TInt DigitalZoom() const;
Gets the currently set digital zoom value.
|
SetDigitalZoom()
IMPORT_C void SetDigitalZoom(TInt aDigitalZoom);
Sets the digital zoom value. Triggers a KUidECamEventCameraSettingDigitalZoom event to all MCameraObserver2
clients.
|
ExposureLockOn()
IMPORT_C TBool ExposureLockOn() const;
Checks whether exposure value is locked or not.
|
SetExposureLockOn()
IMPORT_C void SetExposureLockOn(TBool aState);
Sets exposure lock state. Triggers a KUidECamEventCameraSettingExposureLock event to all MCameraObserver2
clients.
|
AutoFocusLockOn()
IMPORT_C TBool AutoFocusLockOn() const;
Checks whether AutoFocus value is locked or not.
|
SetAutoFocusLockOn()
IMPORT_C void SetAutoFocusLockOn(TBool aState);
Sets autofocus lock state. Triggers a KUidECamEventCameraSettingAutoFocusLock event to all MCameraObserver2
clients.
|
GetSupportedSettingsL()
IMPORT_C void GetSupportedSettingsL(RArray< TUid > &aSettings) const;
Gets an array of all the advanced settings parameters supported by the camera. These are identified by UIDs and relate to the set or subset of it of all defined settings UIDs.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added uid values (new settings added). So, any extra
uid value passed from the implementation will be filtered at this point. To receive extra added uid values, application should
rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised uid values
KUidECamEventCameraSettingAutoFocusType2UidValue is the baseline. Any settings with greater uid value means that it has been added in later versions
|
|
GetActiveSettingsL()
IMPORT_C void GetActiveSettingsL(RArray< TUid > &aActiveSettings) const;
Gets an array of all the advanced settings parameters currently active on the camera. These are identified by UIDs and relate to the set or subset of it of all supported settings UIDs.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added uid values (new settings added). So, any extra
uid value passed from the implementation will be filtered at this point. To receive extra added uid values, application should
rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised uid values
KUidECamEventCameraSettingAutoFocusType2UidValue is the baseline. Any settings with greater uid value means that it has been added in later versions
|
|
GetDisabledSettingsL()
IMPORT_C void GetDisabledSettingsL(RArray< TUid > &aDisabledSettings) const;
Gets an array of all the advanced settings parameters currently disabled on the camera. These are identified by UIDs and relate to the set or subset of it of all supported settings UIDs.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added uid values (new settings added). So, any extra
uid value passed from the implementation will be filtered at this point. To receive extra added uid values, application should
rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised uid values
KUidECamEventCameraSettingAutoFocusType2UidValue is the baseline. Any settings with greater uid value means that it has been added in later versions
|
|
AutomaticSizeSelectionChangeOn()
IMPORT_C TBool AutomaticSizeSelectionChangeOn() const;
Retrieves the state for automatic size selection option. Default value is EFalse.
|
SetAutomaticSizeSelectionChangeOn()
IMPORT_C void SetAutomaticSizeSelectionChangeOn(TBool aSetOn);
Allow camera to proactively change image size due external factors. Default value is EFalse. Triggers a KUidECamEventCameraSettingAutomaticSizeSelection event notification.
|
GetSupportedContinuousAutoFocusTimeoutsL()
IMPORT_C void GetSupportedContinuousAutoFocusTimeoutsL(RArray< TInt > &aTimeouts, TValueInfo &aInfo) const;
Retrieves the timeout values camera supported by the camera when in continuous auto focus mode. Timeouts are in microseconds.
Note: When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
|
ContinuousAutoFocusTimeout()
IMPORT_C TInt ContinuousAutoFocusTimeout() const;
Gets the current value for continuous autofocus timeout. Timeouts are in microseconds.
|
SetContinuousAutoFocusTimeout()
IMPORT_C void SetContinuousAutoFocusTimeout(TInt aTimeout);
Sets the current value for continuous autofocus timeout. Timeouts are in microseconds. All MCameraObserver2
clients of the camera receive a KUidECamEventCameraSettingsContinuousAutoFocusTimeout event notification when timeout value
is changed.
|
SupportedStabilizationEffects()
IMPORT_C TInt SupportedStabilizationEffects() const;
Gets the current stabilization effect on the camera.
|
StabilizationEffect()
IMPORT_C TStabilizationEffect StabilizationEffect() const;
Gets all supported stabilization effects on the camera.
|
SetStabilizationEffect()
IMPORT_C void SetStabilizationEffect(TStabilizationEffect aEffect);
Sets a specific stabilization effect on the camera. When a value is set, MCameraObserver2
clients for that camera will receive a KUidECamEventCameraSettingsStabilizationEffect event notification.
|
SupportedStabilizationComplexityValues()
IMPORT_C TInt SupportedStabilizationComplexityValues() const;
Gets all supported stabilization algorithm values on the camera.
|
StabilizationComplexity()
IMPORT_C TStabilizationAlgorithmComplexity StabilizationComplexity() const;
Gets current active stabilization algorithm selection on the camera.
|
SetStabilizationComplexity()
IMPORT_C void SetStabilizationComplexity(TStabilizationAlgorithmComplexity aComplexity);
Sets a specific stabilization algorithm on the camera. When a value is set, MCameraObserver2
clients for that camera will receive a KUidECamEventSettingsStabilizationAlgorithmComplexity event notification.
|
SupportedWBUnits()
IMPORT_C TWBUnits SupportedWBUnits() const;
Gets the units in which the white balance is measured on the camera.
Note: The methods used to get or set these differ depending on the supported unit type. It is expected that a camera will support only a single type or none.
|
GetWBRgbValue()
IMPORT_C void GetWBRgbValue(TRgb &aValue) const;
Get white balance value represented as a RGB triplet.
|
SetWBRgbValue()
IMPORT_C void SetWBRgbValue(const TRgb &aValue);
Set white balance value using a RGB triplet. Change in value causes an event notification KUidECamEventCameraSettingsWBValue
to be sent to all MCameraObserver2
clients of this camera.
|
GetWBSupportedColorTemperaturesL()
IMPORT_C void GetWBSupportedColorTemperaturesL(RArray< TInt > &aWBColorTemperatures, TValueInfo &aInfo) const;
Get the white balance values, as temperature measured in Kelvin, supported on the camera.
Note: When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
WBColorTemperature()
IMPORT_C TInt WBColorTemperature() const;
Get the white balance as a temperature in Kelvin
|
SetWBColorTemperature()
IMPORT_C void SetWBColorTemperature(TInt aWBColorTemperature);
Set the white balance as a temperature in Kelvin
|
IsFlashReady()
IMPORT_C TInt IsFlashReady(TBool &aReady) const;
Checks whether the flash is ready.
|
|
GetCurrentFocusModeStepsL()
IMPORT_C void GetCurrentFocusModeStepsL(RArray< TInt > &aFocusModeSteps, TValueInfo &aInfo) const;
Get the number of focus steps for current focus mode.
Note: When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
|
|
TCameraType
TCameraType
Specifies camera type.
|
TStabilizationMode
TStabilizationMode
Specifies stabilization mode of the camera.
|
TStabilizationEffect
TStabilizationEffect
Supported magnitudes of stabilization effect when in manual mode.
|
TStabilizationAlgorithmComplexity
TStabilizationAlgorithmComplexity
Supported stabilization algorithms, graded on complexity.
|
TFocusMode
TFocusMode
Supported focus modes.
|
TFocusRange
TFocusRange
Supported focus ranges.
|
TAutoFocusType
TAutoFocusType
Specifies the supported autofocus types.
|
TAutoFocusArea
TAutoFocusArea
Specifies the autofocus area.
|
TMeteringMode
TMeteringMode
Specifies the Metering mode for the camera. EMeteringModeAuto is the default value.
|
TDriveMode
TDriveMode
Specifies the drive mode for the camera. This determines how and in what sucession are images shot. EDriveModeSingleShot is the default.
|
TBracketMode
TBracketMode
Specifies Bracket mode.
|
TBracketParameter
TBracketParameter
Supported parameters used for bracketing.
Note: Bracket mode parameter value changes by a selected uniform step between successive image shots.
|
TBracketStep
TBracketStep
Specifies the magnitude of bracketing step. The actual value and availability is parameter dependent.
|
TPictureOrientation
TPictureOrientation
Specifies the orientation of the picture.
|
TPixelAspectRatio
TPixelAspectRatio
Specifies the pixel aspect ratio
Note: It is specified as a fraction of (x) horizontal pixel size divided by vertical (y) pixel size. The pixel aspect ratio for square pixels is 1/1.
|
TYuvRange
TYuvRange
Specifies YUV colour space dynamic range.
Note: video compressors often use narrower than the default range. The nominal Y range is [16:235] and the U and V ranges [16:240].
|
TWBUnits
TWBUnits
Specifies the units supported by the camera for manual white balance setting.
|
class CCameraPresets : public CBase;
This API is used to simplify user - camera interaction by allowing simultaneous setting of various advanced camera hardware settings using a single parameter.
Preset is identified by a single UID and relates to a known predefined outcome. For example the 'Night' Preset will be used to set the camera into a night mode so that the user can take night photos.
The particular set of settings associated with the specific preset and their specific values and ranges are camera hardware specific and outside the scope of this API.
Note: This class is not intended for sub-classing and used to standardise existing varieties of implementations.
CBase
- Base class for all classes to be instantiated on the heap
CCamera::CCameraPresets
- This API is used to simplify user - camera interaction by allowing simultaneous setting of various advanced camera hardware
settings using a single parameter
Defined in CCamera::CCameraPresets
:
GetAffectedSettingsL()
, GetAssociatedSettingsL()
, GetSupportedPresetsL()
, NewL()
, Preset()
, SetPreset()
, ~CCameraPresets()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
NewL()
static IMPORT_C CCameraPresets *NewL(CCamera &aCamera);
Factory function for creating the CCameraPresets
object.
Note: Clients using MCameraObserver
are not recommended to use this extension class since they cannot handle events.
|
|
|
~CCameraPresets()
IMPORT_C ~CCameraPresets();
Destructor
GetSupportedPresetsL()
IMPORT_C void GetSupportedPresetsL(RArray< TUid > &aPresets) const;
Gets the presets supported by the camera. These are identified by UIDs and relate to a known predefined outcome. The settings associated with a particular preset and their specific values and ranges are specific to each type of camera hardware and are therefore not defined by the API.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added uid values (new presets added). So, any extra
uid value(unrecognised) passed from the implementation will be filtered at this point. To receive extra added uid values,
application should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised uid values.
Note: Any preset uid, if added in future, has to have a uid value greater than KUidECamPresetFactoryDefaultUidValue otherwise unfamilar preset uids could not be checked.
|
|
SetPreset()
IMPORT_C void SetPreset(TUid aPreset);
Sets a specific preset supported by the device. All clients, implementing the MCameraObserver2
interface will receive a notification with the UID of the specific preset, signalling a new preset has been selected.
|
Preset()
IMPORT_C TUid Preset() const;
Gets the current preset set on the camera.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added uid values (new presets added). So, any extra
uid(unrecognised) value received from the implementation will be mapped to KUidECamPresetFactoryDefault at this point. To
receive extra added uid values, application should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised uid values
|
GetAffectedSettingsL()
IMPORT_C void GetAffectedSettingsL(RArray< TUid > &aSettings) const;
Get all settings affected by the current preset. This is to say that all settings which are related to the preset in question will be included in the list, even though the value might not have changed.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added uid values (new settings added). So, any extra
uid value passed from the implementation will be filtered at this point. To receive extra added uid values, application should
rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised uid values
|
|
GetAssociatedSettingsL()
IMPORT_C void GetAssociatedSettingsL(TUid aPreset, RArray< TUid > &aSettings) const;
Get all settings associated with a specific preset, identified by a UID. This function does not require a preset to have been
set prior the call as in GetAffectedSettingsL()
function. The returned array will contain the UIDs of all settings which are associated and potentially affected by that
particular preset.
Note: if CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added uid values (new settings added). So, any extra
uid value passed from the implementation will be filtered at this point. To receive extra added uid values, application should
rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised uid values
|
|
class CCameraImageProcessing : public CBase;
This class is used to perform image processing operations on the camera. These include brightness, contrast, gamma, hue, sharpness and saturation adjustments. The client is also able to perform simple image transformations like cropping, rotation, mirroring, scaling, noise reduction and glare reduction. . When an operation selection is complete, all clients are notified with the respective event UID.
As often cameras may support only a subset of discrete values of the allowed range, the API allows the client to retrieve those and use them explicitly.
Note: This class is not intended for sub-classing and used to standardise existing varieties of implementations.
Note: it is assumed that setting a new value for a transformations(transform, adjust, effect) effectively activates the transformations. Whilst for effects and adjustments there is always a value, transforms may have a dependency on other parameters and crop - requires setting of source rectangle. scale - will use setting of source rectangle, and the magnification factor is determined by the source rectangle and the output size. This is always magnification. if a value is set, it is assumed to be a scaling factor multiplied by KECamFineResolutionFactor and set to integer. mirror - values of TMirror type. rotation - the angle in degrees. noise reduction - TNoiseReduction. glare removal - TGlareReduction.
Example
// Lets assume that an application would need to check whether gamma correction is
// supported on a particular platform. After obtaining a valid pointer to the interface,
// it would call GetSupportedTransformationsL() to obtain the list of the supported
// transformations and check whether KUidECamEventImageProcessingAdjustGamma
// is in the list. If it is then call SetTranformationValue(KUidECamEventImageProcessingAdjustGamma, 200);
// to set the new value. A notification will be generated to the client to indicate success.
RArray<TUid> suppTransforms; // array of supported transformations
CleanupClosePushL(suppTransforms);
imageProcess->GetSupportedTransformationsL(suppTransfUids);
...
// if the gamma is supported
TInt gammaCorrection = 200; // gamma correction of 2.0
imageProcess->SetTranformationValue(KUidECamEventImageProcessingAdjustGamma, gammaCorrection);
...
// pop stack to close the RArray
CBase
- Base class for all classes to be instantiated on the heap
CCamera::CCameraImageProcessing
- This class is used to perform image processing operations on the camera
Defined in CCamera::CCameraImageProcessing
:
EEffectEmboss
, EEffectLowSharpening
, EEffectMonochrome
, EEffectNegative
, EEffectNeutral
, EEffectNone
, EEffectRedEyeReduction
, EEffectSepia
, EEffectSolarize
, EEffectVivid
, EGammaAuto
, EGlareReductionBasic
, EGlareReductionNone
, EHueAuto
, EMirrorBoth
, EMirrorHorizontal
, EMirrorNone
, EMirrorVertical
, ENoiseReductionBasic
, ENoiseReductionNone
, ESaturationAuto
, ESharpnessAuto
, GetActiveTransformSequenceL()
, GetActiveTransformationsL()
, GetSourceRect()
, GetSupportedTransformationsL()
, GetTransformationSupportedValuesL()
, NewL()
, SetActiveTransformSequenceL()
, SetSourceRect()
, SetTransformationValue()
, TEffect
, TGamma
, TGlareReduction
, THue
, TMirror
, TNoiseReduction
, TSaturation
, TSharpness
, TransformationValue()
, ~CCameraImageProcessing()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
NewL()
static IMPORT_C CCameraImageProcessing *NewL(CCamera &aCamera);
Factory function for creating the CCameraImageProcessing
object.
Note: Clients using MCameraObserver
are not recommended to use this extension class since they cannot handle events.
|
|
|
~CCameraImageProcessing()
IMPORT_C ~CCameraImageProcessing();
Destructor
GetSupportedTransformationsL()
IMPORT_C void GetSupportedTransformationsL(RArray< TUid > &aTransformations) const;
Get all transformations supported on the camera.
|
|
GetActiveTransformationsL()
IMPORT_C void GetActiveTransformationsL(RArray< TUid > &aTransformations) const;
Get currently active transformations on the camera.
|
|
GetTransformationSupportedValuesL()
IMPORT_C void GetTransformationSupportedValuesL(TUid aTransformation, RArray< TInt > &aValues, TValueInfo &aInfo) const;
Get all values supported by an active transformation.
Note: Depending on the value of aInfo parameter, same array of values may describe different set of values. When camera device doesn't support this, empty array may be returned and TValueInfo may be ENotActive; corresponding getter/setters for this feature should not be used in such a case.
Note: If CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added enum values for Effects. So, any extra enum
value(unrecognised) passed from the implementation will be filtered at this point. To receive extra added enum values, application
should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised enum values
it is assumed that implementation will use EBitField to pack all supported effects
|
|
TransformationValue()
IMPORT_C TInt TransformationValue(TUid aTransformation) const;
Get the current value of a transformation
Note: If CCamera::New2L()
or CCamera::NewDuplicate2L()
is not used to create CCamera
object, it is assumed that application is not prepared to receive extra added enum values for Effects. So, any extra enum
value (unrecognised) received from the implementation will be dropped and EEffectNone would be passed instead. To receive
extra added enum values, application should rather use CCamera::New2L()
or CCamera::NewDuplicate2L()
to create camera object. In this case, application is assumed to be prepared to receive unrecognised enum values
|
|
SetTransformationValue()
IMPORT_C void SetTransformationValue(TUid aTransformation, TInt aValue);
Set new value for a transformation. A notification event with the transformation UID is sent to all clients. UIDs are in the form KUidECamEventImageProcessingXXXX.
|
GetActiveTransformSequenceL()
IMPORT_C void GetActiveTransformSequenceL(RArray< TUid > &aTransformSequence) const;
Get the sequence of all active transforms, ordered in order of execution.
|
|
SetActiveTransformSequenceL()
IMPORT_C void SetActiveTransformSequenceL(RArray< TUid > &aTransformSequence);
Set the order of all active transform in terms of execution. The transforms with smaller index are executed earlier.
|
|
SetSourceRect()
IMPORT_C void SetSourceRect(const TRect &aRect);
Set the source rectangle for KUidECamEventImageProcessingTransformScale or KUidECamEventImageProcessingTransformCrop. The coordinates should fall within the current image rectangle. The result is always a logical AND operation between the two rectangles.
|
GetSourceRect()
IMPORT_C void GetSourceRect(TRect &aRect) const;
Get the source rectangle for KUidECamEventImageProcessingTransformScale or KUidECamEventImageProcessingTransformCrop. The coordinates should fall within the current image rectangle. The result is always a logical AND operation between the two rectangles.
|
TGamma
TGamma
Gamma settings
|
TSharpness
TSharpness
Sharpness Settings
|
TSaturation
TSaturation
Saturation Settings
|
THue
THue
Hue Settings
|
TEffect
TEffect
Settings for the supported effects
|
TMirror
TMirror
Mirror settings
|
TNoiseReduction
TNoiseReduction
Noise filter settings
|
TGlareReduction
TGlareReduction
Glare reduction settings
|
TFormat
Possible still image and video frame formats
Formats are read from left to right, starting at the top of the image. YUV format is as defined by ITU-R BT.601-4.
|
TContrast
Specifies whether contrast is set automatically.
|
TBrightness
Specifies whether brightness is set automatically.
|
TFlash
Specifies the type of flash.
|
TExposure
Specifies the type of exposure. - EExposureAuto is the default value.
|
TWhiteBalance
Specifies how the white balance is set.
|