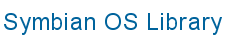
![]() |
![]() |
|
Location:
TXTFRMAT.H
Link against: etext.lib
class CParaFormat : public CBase;
A transient container of paragraph format attributes, including tab stops, bullet points and paragraph borders.
Rich and global text objects store paragraph formatting using paragraph format layers (see class CParaFormatLayer
). The CParaFormat class is used to store the relevant attribute values when setting or sensing a CParaFormatLayer
. It is normally used in combination with a TParaFormatMask
, to specify which attributes are relevant to the function concerned.
On construction, all CParaFormat member data is initialised. The attributes which are not explicitly set are assigned default values.
CBase
- Base class for all classes to be instantiated on the heap
CParaFormat
- A transient container of paragraph format attributes, including tab stops, bullet points and paragraph borders
Defined in CParaFormat
:
AllBordersEqual()
, BordersPresent()
, CParaFormat()
, CopyL()
, CopyL()
, EAbsoluteLeftAlign
, EAbsoluteRightAlign
, EAllAttributes
, EBottomAlign
, ECenterAlign
, ECustomAlign
, EFixedAttributes
, EJustifiedAlign
, ELeftAlign
, ELineSpacingAtLeastInPixels
, ELineSpacingAtLeastInTwips
, ELineSpacingExactlyInPixels
, ELineSpacingExactlyInTwips
, EMaxParaBorder
, EParaBorderBottom
, EParaBorderLeft
, EParaBorderRight
, EParaBorderTop
, ERightAlign
, ETopAlign
, EUnspecifiedAlign
, IsBorderEqual()
, IsEqual()
, IsEqual()
, LocateTab()
, NewL()
, NewL()
, NewLC()
, ParaBorder()
, ParaBorderPtr()
, RemoveAllBorders()
, RemoveAllTabs()
, RemoveTab()
, Reset()
, ResetNonDestructive()
, SetParaBorderL()
, StoreTabL()
, Strip()
, TAlignment
, TLineSpacingControl
, TParaBorderSide
, TParaFormatGetMode
, TabCount()
, TabStop()
, anonymous
, iBorderMarginInTwips
, iBullet
, iDefaultTabWidthInTwips
, iFillColor
, iHorizontalAlignment
, iIndentInTwips
, iKeepTogether
, iKeepWithNext
, iLanguage
, iLeftMarginInTwips
, iLineSpacingControl
, iLineSpacingInTwips
, iRightMarginInTwips
, iSpaceAfterInTwips
, iSpaceBeforeInTwips
, iStartNewPage
, iVerticalAlignment
, iWidowOrphan
, iWrap
, ~CParaFormat()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
static IMPORT_C CParaFormat *NewL();
Allocates and constructs a CParaFormat object. All attributes are initialised with default values.
|
static IMPORT_C CParaFormat *NewLC();
Allocates and constructs a CParaFormat object. All attributes are initialised with default values. Leaves the object on the cleanup stack.
|
static IMPORT_C CParaFormat *NewL(const CParaFormat &aFormat);
Allocates and constructs a new CParaFormat. All attributes are initialised to the values contained in the aFormat argument.
|
|
IMPORT_C CParaFormat();
The default C++ constructor constructs a new CParaFormat initialising all attributes to the default settings.
Note: This function allows a CParaFormat object to be created on the stack. This should only be done if it is known in advance that the object will not be used to store tab stops, bullets or borders.
IMPORT_C ~CParaFormat();
The destructor frees all resources owned by the paragraph format container (tabs, borders and bullets), prior to its destruction.
Note that Strip()
also sets the resource pointers to NULL. This is important in case CParaFormat is on the stack and gets deleted twice: once
by the cleanup stack and once by exceptions being unwound.
IMPORT_C void ResetNonDestructive();
Resets all paragraph format attributes to their default values, but any allocated tab stops, bullet points and paragraph borders are preserved.
IMPORT_C void Reset();
Resets all paragraph format attributes to their default values. All tab stops, paragraph borders and bullet points which have been allocated are deleted and set to NULL.
IMPORT_C void CopyL(const CParaFormat &aFormat, const TParaFormatMask &aMask);
Copies selected attribute values from another paragraph format container. Only the attributes which are set in the mask are copied.
|
IMPORT_C void CopyL(const CParaFormat &aFormat);
Copies all attribute values from another paragraph format container.
|
IMPORT_C void Strip();
Deletes all paragraph borders, bullets and tab stops. No other attributes are affected.
IMPORT_C TBool IsEqual(const CParaFormat &aFormat, const TParaFormatMask &aMask) const;
Compares selected attribute values for equality. Only the attributes specified in the mask are involved in the comparison.
|
|
IMPORT_C TBool IsEqual(const CParaFormat &aFormat) const;
Compares all attribute values for equality.
|
|
IMPORT_C void StoreTabL(const TTabStop &aTabStop);
Adds a tab stop to the list of tab stops, maintaining the ordering of the list, (ascending order of twips position). Multiple tabs with the same twips position are not allowed, so that if aTabStop shares the same twips position as an existing tab stop, regardless of its alignment, the existing tab stop is replaced by aTabStop.
|
IMPORT_C void RemoveTab(TInt aTabTwipsPosition);
Deletes a tab stop identified by its twips position. If the specified tab stop does not exist, the function has no effect.
|
IMPORT_C const TTabStop TabStop(TInt aTabIndex) const;
Gets the tab stop located at the specified index within the tab list (counting from zero). Tab stops are ordered in ascending order of twips position. If the object has no tab list, then a default tab stop is returned.
|
|
inline TInt TabCount() const;
Gets a count of the total number of tab stops in the object's tab list. If the object has no tab list, returns zero.
|
IMPORT_C TInt LocateTab(TInt aTabTwipsPosition) const;
Locates the tab stop specified by its twips position, and returns its offset in the tab list.
|
|
IMPORT_C void SetParaBorderL(TParaBorderSide aSide, const TParaBorder &aBorder);
Sets one side of the object's paragraph border. If a border on the specified side already exists, it is replaced.
Note: Setting a single side of the object's paragraph border incurs the overhead of allocating storage for the three other sides, which are assigned default values.
|
IMPORT_C const TParaBorder ParaBorder(TParaBorderSide aSide) const;
Gets the paragraph border on the side specified. If no paragraph border array has been allocated, returns a default paragraph border.
|
|
inline TBool BordersPresent() const;
Tests whether any paragraph borders have been set.
|
inline TParaBorder *ParaBorderPtr(TParaBorderSide aSide);
Gets a pointer to the paragraph border on the side specified. If no paragraph border array has been allocated, returns NULL.
|
|
IMPORT_C TBool AllBordersEqual(const CParaFormat &aFormat) const;
Tests whether all paragraph borders in the specified paragraph format container are identical to the paragraph borders of this paragraph format container.
|
|
IMPORT_C TBool IsBorderEqual(TParaBorderSide aSide, const CParaFormat &aFormat) const;
Tests whether the paragraph border located on the specified side is the same as the border on the corresponding side in this object. For two borders to be equal, they must both either be set or unset, and if set, they must have the same characteristics.
|
|
n/a
Miscellaneous constants.
|
TParaBorderSide
Paragraph border sides
|
TLineSpacingControl
Line spacing control
|
TAlignment
Paragraph alignment
|
TParaFormatGetMode
Attribute sense mode
|
TLogicalRgb iFillColor;
The background colour of the paragraph. By default the default system background colour. This colour applies to the area bounded by the paragraph border, if one exists.
TInt32 iLanguage;
The language of the paragraph for proofing. By default KParaDefaultLanguage. Used for example when spell checking a document which contains text in more than one language, so that the program recognises the text as being in another language.
TInt32 iLeftMarginInTwips;
The width in twips of the leading margin (left for left-to-right paragraphs, right for right-to-left paragraphs). By default KParaDefaultLeftMargin (zero).
TInt32 iRightMarginInTwips;
The width in twips of the trailing margin (right for left-to-right paragraphs, left for right-to-left paragraphs). By default KParaDefaultRightMargin (zero).
TInt32 iIndentInTwips;
An indent for the first line in the paragraph, relative to the leading margin (left for left-to-right paragraphs, right for right-to-left paragraphs). By default KParaDefaultIndent (zero).
TAlignment iHorizontalAlignment;
Horizontal alignment of paragraph. By default KParaDefaultHorizAlign (left).
TAlignment iVerticalAlignment;
Vertical alignment of paragraph, (intended for use by spreadsheet applications). By default KParaDefaultVertAlign (unspecified).
TInt32 iLineSpacingInTwips;
Inter-line spacing within the paragraph, in twips. By default KParaDefaultLineSpacing (200 twips).
TLineSpacingControl iLineSpacingControl;
Control for the iLineSpacingInTwips value. By default, KParaDefaultLineSpacingControl (ELineSpacingAtLeastInTwips).
TInt32 iSpaceBeforeInTwips;
Space above paragraph. By default KParaDefaultSpaceBefore (zero).
TInt32 iSpaceAfterInTwips;
Space below paragraph. By default KParaDefaultSpaceAfter (zero).
TBool iKeepTogether;
Prevents a page break within paragraph if ETrue. By default KParaDefaultKeepTogether (EFalse).
TBool iKeepWithNext;
Prevents a page break between this paragraph and the following paragraph if ETrue. By default, KParaDefaultKeepWithNext (EFalse).
TBool iStartNewPage;
Inserts a page break immediately before this paragraph if ETrue. By default, KParaDefaultStartNewPage (EFalse).
TBool iWidowOrphan;
Prevents the printing of the last line of a paragraph at the top of the page (referred to as a widow), or the first line of a paragraph at the bottom of the page, (referred to as an orphan). By default, KParaDefaultWidowOrphan (EFalse).
TBool iWrap;
Specifies whether the paragraph should line wrap at the right margin. By default KParaDefaultWrap (ETrue).
TInt32 iBorderMarginInTwips;
Distance in twips between the paragraph border and the enclosed text. By default KParaDefaultBorderMargin (zero).
TBullet * iBullet;
The bullet point associated with the paragraph. A NULL value indicates no bullet point. By default NULL.
TUint32 iDefaultTabWidthInTwips;
Specifies the default tab stop width. By default KParaDefaultTabWidth (360 twips).