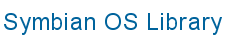
![]() |
![]() |
|
Location:
FNTSTORE.H
Link against: fntstr.lib
class CBitmapFont : public CFont;
Bitmap font class. An object of this class may either access and use a bitmap font (a CFontBitmap
) or an open font (a COpenFont
). Stored in a CFontStore
.
CBase
- Base class for all classes to be instantiated on the heap
CFont
- Abstract font interface
CBitmapFont
- Bitmap font class
Defined in CBitmapFont
:
BitmapEncoding()
, CharacterMetrics()
, CharacterNeedsToBeRasterized()
, DoAscentInPixels()
, DoBaselineOffsetInPixels()
, DoCharWidthInPixels()
, DoFontSpecInTwips()
, DoHeightInPixels()
, DoMaxCharWidthInPixels()
, DoMaxNormalCharWidthInPixels()
, DoTextCount()
, DoTextCount()
, DoTextWidthInPixels()
, DoTypeUid()
, FontWithCharacter()
, GetCharacterData()
, GetFaceAttrib()
, GetFontMetrics()
, GlyphBitmapType()
, HasCharacterL()
, IsOpenFont()
, OpenFont()
, Rasterize()
, iAlgStyle
, operator delete()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CFont
:
AscentInPixels()
,
BaselineOffsetInPixels()
,
CharWidthInPixels()
,
CharactersJoin()
,
DescentInPixels()
,
DoDescentInPixels()
,
DoGetCharacterData()
,
EAllCharacterData
,
ECharacterWidthOnly
,
EHorizontal
,
ENoCharacterData
,
EVertical
,
ExtendedFunction()
,
FontCapitalAscent()
,
FontLineGap()
,
FontMaxAscent()
,
FontMaxDescent()
,
FontMaxHeight()
,
FontSpecInTwips()
,
FontStandardDescent()
,
GetCharacterPosition2()
,
HeightInPixels()
,
MaxCharWidthInPixels()
,
MaxNormalCharWidthInPixels()
,
MeasureText()
,
TCharacterDataAvailability
,
TMeasureTextInput
,
TMeasureTextOutput
,
TPositionParam
,
TTextDirection
,
TextCount()
,
TextWidthInPixels()
,
TypeUid()
,
WidthZeroInPixels()
IMPORT_C TCharacterMetrics CharacterMetrics(TInt aCode, const TUint8 *&aBytes) const;
Gets a pointer to a bitmap and the metrics for a specified character, but only if a CFontBitmap
is being used by the bitmap font object.
This function does not work when a COpenFont
is being used, but GetCharacterData()
can be used instead.
If the specified character does not exist in the font then the bitmap pointer and character metrics are gotten for a replacement character, KReplacementCharacter.
|
|
IMPORT_C TBool GetCharacterData(TInt aSessionHandle, TInt aCode, TOpenFontCharMetrics &aMetrics, const TUint8 *&aBitmap) const;
Gets a pointer to a bitmap and the metrics for a specified character.
Note that this function calls CharacterMetrics()
if a CFontBitmap
is being used by the bitmap font object, and maps the TCharacterMetrics
values returned by that function to aMetrics.
If the function fails to get the bitmap and metric values (because the character is in an open font and has not yet been rasterized) returns EFalse.
|
|
IMPORT_C TBool Rasterize(TInt aSessionHandle, TInt aCode, TOpenFontGlyphData *aGlyphData) const;
Attempts to rasterize a character (aCode) into a data area (aGlyphData) in shared memory.
This works only for open fonts: where the bitmap font object is using a COpenFont
.
Bitmap fonts are deemed to be fully rasterized after they have been loaded from file.
Returns ETrue if the character was successfully rasterized or was already in the cache. This function can be called only by the server; rasterization uses memory and other resources (e.g., file handles) owned by the server.
|
|
IMPORT_C void GetFontMetrics(TOpenFontMetrics &aMetrics) const;
Gets the open font metrics.
These metrics distinguish between maximum character height and depth and typographic ascent and descent, so that the clipping rectangle for text can be distinguished from the distance to neighbouring baselines.
|
IMPORT_C TBool GetFaceAttrib(TOpenFontFaceAttrib &aAttrib) const;
Gets the open font typeface attributes if possible.
At present no attempt is made to sythesize these attributes for bitmap fonts (CFontBitmaps).
|
|
IMPORT_C TInt BitmapEncoding() const;
Gets encoding if a bitmap font (a CFontBitmap
) is used.
|
IMPORT_C TBool HasCharacterL(TInt aCode) const;
Gets whether the open or bitmap font has the specified character.
|
|
IMPORT_C TBool CharacterNeedsToBeRasterized(TInt aSessionHandle, TInt aCode) const;
Gets whether the specified character needs to be rasterised.
False is returned if it is a bitmap font (a CFontBitmap
) being used by the bitmap font object (so no rasterization is required) or if is an open font (a COpenFont
) and the character has been rasterized.
|
|
inline TBool IsOpenFont() const;
Returns whether the bitmap font object is an open font (a COpenFont
) rather than a bitmap font (a CFontBitmap
).
|
inline COpenFont *OpenFont() const;
Returns a pointer to the open font being used by the bitmap font object.
|
inline TGlyphBitmapType GlyphBitmapType() const;
Gets the anti-aliasing setting for the font, see TGlyphBitmapType for the range of values.
This is only applicable to open fonts (COpenFonts) not bitmap fonts (CBitmapFonts).
Note that this setting is stored in the device-independent font specification which is set when the bitmap font object is constructed.
|
IMPORT_C CBitmapFont *FontWithCharacter(TInt aCode, CLinkedFontInformation *&aInfo, TBool aSearchAllFonts, TBool aGetCanonicalDefault)
const;
|
|
private: virtual IMPORT_C TUid DoTypeUid() const;
Returns the font type identifier:KCBitmapFontUidVal.
|
private: virtual IMPORT_C TInt DoHeightInPixels() const;
Returns the font height in pixels. For an open font this will be the design height (notional rather than exact).
|
private: virtual IMPORT_C TInt DoAscentInPixels() const;
Returns the font ascent in pixels.
The ascent is usually the height of a Latin capital letter above the baseline. For an open font, the returned value is likely to be inaccurate. Don't rely on it to get exact metrics at the pixel level.
|
private: virtual IMPORT_C TInt DoCharWidthInPixels(TChar aChar) const;
Returns the width, in pixels, of the given character.
If all characters have the same width (i.e. the font is mono - an attribute stored in iAlgStyle) then this is the maximum
normal width, returned using MaxNormalCharWidthInPixels()
.
|
|
private: virtual IMPORT_C TInt DoTextWidthInPixels(const TDesC &aText) const;
Returns the width, in pixels, of the given text.
|
|
private: virtual IMPORT_C TInt DoBaselineOffsetInPixels() const;
Returns the baseline offset in pixels, as stored in iAlgStyle.
|
private: virtual IMPORT_C TInt DoTextCount(const TDesC &aText, TInt aWidthInPixels) const;
Returns the number of whole characters of aText, starting from the beginning, that fit into the given pixel width.
|
|
private: virtual IMPORT_C TInt DoTextCount(const TDesC &aText, TInt aWidthInPixels, TInt &aExcessWidthInPixels) const;
Returns the number of whole characters of aText, starting from the beginning, that fit into the given pixel width, and gets the excess width.
|
|
private: virtual IMPORT_C TInt DoMaxCharWidthInPixels() const;
Returns the font's maximum character width in pixels.
|
private: virtual IMPORT_C TInt DoMaxNormalCharWidthInPixels() const;
Returns the font's normal maximum character width in pixels.
Note that when a CFontBitmap
is used this value is the same as the maximum character width in pixels returned by MaxCharWidthInPixels()
, but when a COpenFont
is used the value may be different.
|
private: virtual IMPORT_C TFontSpec DoFontSpecInTwips() const;
Returns the device-independent font specification for the font.
Note that this is set when the bitmap font object is constructed.
|
TAlgStyle iAlgStyle;
The algorithmic font style.