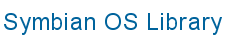
![]() |
![]() |
|
Location:
GDI.H
Link against: gdi.lib
class CPrinterDevice : public CGraphicsDevice;
Printer graphics device interface.
This abstract class represents a physical graphics device that is used for printing.
This class is used to:
set and get the page specification
map between the co-ordinates of the printed page (in twips) and the co-ordinates of the image device (in pixels)
get and set the printer model entry
create and delete a printer control.
A printer driver is defined in terms of a printer device and a printer control. A printer device can own either a single or no printer control. The control determines the progress and termination of the print job and is responsible for producing output.
MGraphicsDeviceMap
- Interface class for mapping between twips and device-specific units (pixels)
CBase
- Base class for all classes to be instantiated on the heap
CGraphicsDevice
- Specifies the interface for concrete device classes
CPrinterDevice
- Printer graphics device interface
Defined in CPrinterDevice
:
CPrinterDevice()
, CreateControlL()
, CurrentPageSpecInTwips()
, DeleteControl()
, ExternalizePropertiesL()
, InternalizePropertiesL()
, Model()
, PrintablePageInPixels()
, RestorePropertiesL()
, SelectPageSpecInTwips()
, SetModel()
, StorePropertiesL()
, iControl
, iCurrentPageSpecInTwips
, ~CPrinterDevice()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CGraphicsDevice
:
CreateContext()
,
DisplayMode()
,
FontHeightInTwips()
,
GetPalette()
,
NumTypefaces()
,
PaletteAttributes()
,
SetPalette()
,
SizeInPixels()
,
SizeInTwips()
,
TypefaceSupport()
Inherited from MGraphicsDeviceMap
:
GetNearestFontInTwips()
,
GetNearestFontToDesignHeightInTwips()
,
GetNearestFontToMaxHeightInTwips()
,
HorizontalPixelsToTwips()
,
HorizontalTwipsToPixels()
,
PixelsToTwips()
,
ReleaseFont()
,
TwipsToPixels()
,
VerticalPixelsToTwips()
,
VerticalTwipsToPixels()
IMPORT_C ~CPrinterDevice();
Destructor. It frees all resources owned by the object, prior to its destruction.
inline TPageSpec CurrentPageSpecInTwips() const;
Gets the current page specification in twips.
|
virtual IMPORT_C void SelectPageSpecInTwips(const TPageSpec &aPageSpec);
Sets the page specification in twips.
|
virtual IMPORT_C TRect PrintablePageInPixels() const;
Gets the dimensions of the area to which the printer device can print.
These dimensions are normally less than those returned by TPageSpec::OrientedPageSize()
because a margin exists between the boundary of the printable page and the absolute extent of the page.
|
virtual TPrinterModelEntry Model() const=0;
Gets the printer model entry.
|
virtual TInt SetModel(const TPrinterModelHeader &aModel, CStreamStore &aStore)=0;
Sets the printer model header and the store.
|
|
virtual void CreateControlL(CPrinterPort *aPrinterPort)=0;
Creates a printer control.
The printer control is an instance of a CPrinterControl
derived class; it is assigned to this printer device's iControl member.
Note that this function is called by CPrintSetup::StartPrintL().
|
virtual IMPORT_C void DeleteControl();
Deletes the printer control owned by this object.
The function sets the iControl member to NULL.
inline virtual void InternalizePropertiesL(RReadStream &);
Externalizes printer properties to the store.
The default implementation is empty.
|
inline virtual void ExternalizePropertiesL(RWriteStream &) const;
Externalizes printer properties to the store.
The default implementation is empty.
|
CPrinterControl * iControl;
The printer control.
This may be NULL. If implemented, it provides control over the print operation.
protected: TPageSpec iCurrentPageSpecInTwips;
Current page specification in twips.