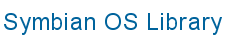
![]() |
![]() |
|
Location:
GRDSTD.H
Link against: grid.lib
class CGridImg : public CBase;
Draws the contents of the grid.
CBase
- Base class for all classes to be instantiated on the heap
CGridImg
- Draws the contents of the grid
Defined in CGridImg
:
AddColToSelectedL()
, AddRangeToSelectedL()
, AddRegionToSelectedL()
, AddRowToSelectedL()
, AnchorPos()
, CheckSideLabelWidth()
, CheckSideLabelWidthAndScrollL()
, ClearTitleLineRegionL()
, CursorPos()
, DrawCellL()
, DrawL()
, DrawL()
, DrawRangeL()
, DrawSelectedL()
, DrawTitleLines()
, EIsAbsoluteMove
, EIsAtBoundary
, EIsColumnSelected
, EIsRowSelected
, EIsWithControl
, EIsWithDrag
, EIsWithSelect
, EScaleOneToOne
, ESelectAppend
, ESelectOverwrite
, FinishLabelDragL()
, FinishLabelResizeL()
, GridLabelImg()
, GridRect()
, MainPoint()
, MainRect()
, MaxSideLabelWidthInPixels()
, MoveCursorL()
, NewCursorPos()
, NewL()
, NewL()
, NotifyGridRangeResize()
, PrintGridLinesAndCellsInRangeL()
, ResetReferencePoints()
, ResetSelectedL()
, ScrollL()
, Selected()
, SetAnchorPosL()
, SetCursorMoveCallBack()
, SetCursorPosL()
, SetCursorWithPointerL()
, SetGridLabelImg()
, SetGridLay()
, SetGridRect()
, SetPrintGridRect()
, SetWindow()
, SideLabelWidthInPixels()
, StartLabelDrag()
, StartLabelResize()
, TSelectType
, TitlePoint()
, TopLabelHeightInPixels()
, UpdateLabelDrag()
, UpdateLabelResize()
, anonymous
, anonymous
, ~CGridImg()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
virtual IMPORT_C ~CGridImg();
Destructor.
Frees resources before destruction of the object.
static IMPORT_C CGridImg *NewL(CGraphicsDevice *aGraphicsDevice, CGridCellImg *aGridCellImg, CGridLay *aGridLay);
Creates a new grid image object.
|
|
static IMPORT_C CGridImg *NewL(CGridCellImg *aGridCellImg, CGridLay *aGridLay);
Creates a new grid image object suitable for printing.
|
|
inline void SetGridLay(CGridLay *aGridLay);
Sets the specified grid layout object.
|
inline void SetWindow(RWindow *aWin);
Sets the specified window.
|
IMPORT_C void SetGridLabelImg(CGridLabelImg *aGridLabelImg);
Sets an object that draws a cell label.
|
inline void SetCursorMoveCallBack(MGridCursorMoveCallBack *aCursorMoveCallBack);
Sets the cursor moved call-back object.
The call-back object encapsulates the implementation of a call-back function that is called whenever there is a change to the cursor position.
|
inline const CGridCellRegion *Selected() const;
Gets the currently selected region.
|
inline const CGridLabelImg *GridLabelImg() const;
Gets the the object that is used to draw a cell label.
|
IMPORT_C void ScrollL(const TPoint &aOffset);
Scrolls the grid by the specified number of pixels.
Draws the minimum necessary, i.e. grid labels, grid lines, grid cells, selected regions and the cursor.
|
IMPORT_C void MoveCursorL(TMoveDirectionAndAmount aCursorMove, TUint aSelectState);
Moves the cursor to the specified position.
The screen is scrolled as necessary.
|
IMPORT_C void SetCursorWithPointerL(const TPoint &aPoint, TUint aFlagList);
Moves the cursor to an absolute position.
|
IMPORT_C void AddRangeToSelectedL(const TRangeRef &aRange, TSelectType aType=ESelectOverwrite);
Adds the specified range to, or replaces, the currently selected region.
The range can be added to the existing selected region or it can replace the existing selected region.
|
IMPORT_C void AddRegionToSelectedL(const CArrayFix< TRangeRef > *aCellRegion, TSelectType aType=ESelectOverwrite);
Adds the specified region to, or replaces, the currently selected region.
The region can be added to the existing selected region, or it can replace the existing selected region.
|
IMPORT_C void AddRowToSelectedL(TInt aRow, TSelectType aType=ESelectOverwrite);
Adds a row to, or replaces, the currently selected region.
The row can be added to the existing selected region, or it can replace the existing selected region.
|
IMPORT_C void AddColToSelectedL(TInt aCol, TSelectType aType=ESelectOverwrite);
Adds a column to, or replaces, the currently selected region.
The column can be added to the existing selected region, or it can replace the existing selected region.
|
IMPORT_C void DrawL(CGraphicsContext *aGc) const;
Draws the entire grid and its contents.
|
IMPORT_C void DrawL(CGraphicsContext *aGc, const TRect &aRect) const;
Draws the grid and its contents that are visible within the specified rectangle.
|
IMPORT_C void DrawCellL(const TCellRef &aCell) const;
Draws the content of the specified cell.
|
IMPORT_C void DrawRangeL(const TRangeRef &aRange) const;
Draws the rectangle corresponding to the specified range.
|
IMPORT_C void ClearTitleLineRegionL(const TPoint &aCrossPoint) const;
Clears the region where the the title lines are.
|
IMPORT_C void PrintGridLinesAndCellsInRangeL(CGraphicsContext *aPrinterGc, const TRangeRef &aRange, TInt aScaleFactor) const;
Prints the grid lines and cells in the specified range.
|
inline TCellRef CursorPos() const;
Gets the current position of the cursor.
|
IMPORT_C void SetCursorPosL(const TCellRef &aCursorPos);
Sets the new position of the cursor.
This is the proposed position that the cursor would occupy after a keypress.
|
inline TCellRef NewCursorPos() const;
Gets the new position of the cursor.
|
inline TCellRef AnchorPos() const;
Gets the cursor's anchor position.
This is the cell reference of the position that the cursor must return to after a series of selected movements have been made.
|
IMPORT_C void SetAnchorPosL(const TCellRef &aAnchorPos);
Sets the cursor's anchor position.
Note that the cursor's anchor position is unchanged if the specified position lies outside the currently selected region.
|
inline TRect GridRect() const;
Gets the rectangle containing the grid.
|
IMPORT_C void SetGridRect(const TRect &aNewRect);
Sets the rectangle that is to contain the grid.
|
IMPORT_C void SetPrintGridRect(const TRect &aPrintRect);
Sets the rectangle that is to contain the grid for the purpose of printing.
|
inline TPoint TitlePoint() const;
Gets the position of the title.
This is the point at the top left of the grid excluding the labels.
|
inline TPoint MainPoint() const;
Gets the position at which the visible range starts.
|
inline TRect MainRect() const;
Gets the rectangle that corresponds to the visible range.
|
IMPORT_C void ResetReferencePoints();
Resets the reference points.
The reference points are the mainpoint and the titlepoint, i.e the point at which the visible range starts, and the point at the top left of the grid not including the labels.
IMPORT_C void CheckSideLabelWidthAndScrollL();
Checks the side label width and, if changed, scrolls the screen.
IMPORT_C TInt CheckSideLabelWidth();
Checks whether there has been a change in the width of the side label.
|
IMPORT_C TInt SideLabelWidthInPixels() const;
Gets the width of the side label.
|
IMPORT_C TInt MaxSideLabelWidthInPixels() const;
Gets the maximum width of the side label.
|
IMPORT_C TInt TopLabelHeightInPixels() const;
Gets the height of the top labels.
|
IMPORT_C void FinishLabelDragL();
Deals with the end of a drag operation.
Typically, the function is called whan handling a pointer event in a control.
The function removes the dotted drag line indicating a drag operation, and, if necessary, updates the column width/row height, and then scrolls and redraws the necessary regions.
IMPORT_C TBool StartLabelDrag(const TPoint &aPoint);
Deals with the start of a drag operation.
Typically, the function is called whan handling a pointer event in a control.
The function checks that the pointer event is within 10 pixels of the boundary between label cells, and draws a dotted line along the row or column boundary together with pairs of arrows on either side of that line, and prepares for the continuation or end of a drag operation on the boundary separating a row or a column (to expand or contract that row or column).
The dotted line is a visual indication that a drag operation is in progress.
|
|
IMPORT_C TBool UpdateLabelDrag(const TPoint &aPoint);
Deletes the dotted drag line and and redraws it at the new pointer position.
Typically, the function is called whan handling a pointer event in a control.
|
|
IMPORT_C TBool StartLabelResize(TBool aIsColumnLabel, TInt aIndex);
Begins the resizing of a column or a row.
|
|
IMPORT_C TBool UpdateLabelResize(TInt aDelta);
Changes the size of the row or column currently being resized.
|
|
IMPORT_C void FinishLabelResizeL(TBool aResize);
Completes the resizing of a row or column.
|
n/a
|
n/a
|
TSelectType
|