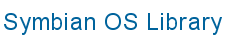
![]() |
![]() |
|
Location:
cprotocolhandler.h
Link against: http.lib
class CProtocolHandler : public CActive, public MHTTPFilter;
An abstract protocol handler. Protocol handlers are required to act as the bridge between abstract representations of sessions, transactions and headers (the client side of the HTTP architecture) and specific comms transports (the network side of the architecture).
Each instance of a concrete subclass of CProtocolHandler is associated with a specific client session, and hence with a particular choice of proxy type, and by implication, transport type. It is designed to appear like a filter in order to be placed at the end of a session's filter queue. This allows it to receive transaction-related events in the same way that any other filter (or indeed, the client) does. An active object, it may implement a queuing system for submitted transactions, according to the chosen internal service model.
In order to divide the abstract functionality associated with handling the HTTP protocol handler from the specifics needed for a particular choice of transport, this class defines a number of pure virtual methods which allow it to defer transport-specific choices or mechamisms. These are mainly concerned with the service model (ie. allocation of transactions to objects that can handle them), the codec model (ie. on-demand encoding/decoding of HTTP header data) and general housekeeping (eg. instantiation and cleanup of objects at particular points in a transaction lifecycle).
MHTTPFilterBase
- A HTTP Filter
MHTTPFilter
- A HTTP filter
CBase
- Base class for all classes to be instantiated on the heap
CActive
- The core class of the active object abstraction
CProtocolHandler
- An abstract protocol handler
Defined in CProtocolHandler
:
CProtocolHandler()
, CancelTransactionHook()
, ClosedTransactionHook()
, Codec()
, CompleteSelf()
, ConstructL()
, CreateCodecL()
, CreateProtTransactionL()
, DoCancel()
, FindTransaction()
, GetInterfaceL()
, MHFLoad()
, MHFRunError()
, MHFRunL()
, MHFSessionRunError()
, MHFSessionRunL()
, MHFUnload()
, NotifyNewRequestBodyPart()
, NumActiveTransactions()
, Reserved2()
, RunError()
, RunL()
, ServiceL()
, SessionServerCert()
, SessionServerCert()
, TransactionCompletedL()
, TransactionFailed()
, TransactionServerCert()
, TransactionServerCert()
, iCodec
, iDummyLoggerNotUsed
, iSecurityPolicy
, iSession
, ~CProtocolHandler()
Inherited from CActive
:
Cancel()
,
Deque()
,
EPriorityHigh
,
EPriorityIdle
,
EPriorityLow
,
EPriorityStandard
,
EPriorityUserInput
,
Extension_()
,
IsActive()
,
IsAdded()
,
Priority()
,
SetActive()
,
SetPriority()
,
TPriority
,
iStatus
Inherited from CBase
:
Delete()
,
operator new()
Inherited from MHTTPFilter
:
ECache
,
EClient
,
EClientFilters
,
ECookies
,
EProtocolHandler
,
EStatusCodeHandler
,
ETidyUp
,
EUAProf
,
TPositions
protected: IMPORT_C CProtocolHandler(RHTTPSession aSession);
Constructs a protocol handler associated with the supplied HTTP client session.
|
protected: IMPORT_C void ConstructL(RHTTPSession aSession);
Second phase construction in which any necessary allocation is done Implementations of this interface may leave with KErrNoMemory
|
IMPORT_C CHeaderCodec *Codec() const;
Obtain the protocol handler's header codec.
|
IMPORT_C const CCertificate *SessionServerCert();
Get the Server Certificate for the current session.
|
IMPORT_C const CCertificate *TransactionServerCert(RHTTPTransaction aTransaction);
Get the Server Certificate for the specified transaction.
|
|
virtual TInt SessionServerCert(TCertInfo &aServerCert)=0;
Intended Usage: Get the Server Certificate for the current session.
|
|
virtual TInt TransactionServerCert(TCertInfo &aServerCert, RHTTPTransaction aTransaction)=0;
Intended Usage: Get the Server Certificate for the specified transaction.
|
|
virtual IMPORT_C void MHFRunL(RHTTPTransaction aTransaction, const THTTPEvent &aEvent);
Intended Usage: Called when the filter's registration conditions are satisfied for events that occur on a transaction. Any Leaves must be handled by the appropriate MHFRunError. Note that this function is not allowed to leave if called with certain events.
|
|
virtual IMPORT_C void MHFSessionRunL(const THTTPSessionEvent &aEvent);
Intended Usage: Called when the filters registration conditions are satisfied for events that occur on the session. Any leaves must be handled by the appropriate MHFRunError.
|
|
virtual IMPORT_C TInt MHFRunError(TInt aError, RHTTPTransaction aTransaction, const THTTPEvent &aEvent);
Intended Usage: Called when RunL leaves from a transaction event. This works in the same way as CActve::RunError; return KErrNone if you have handled the error. If you don't completely handle the error, a panic will occur.
|
|
virtual IMPORT_C TInt MHFSessionRunError(TInt aError, const THTTPSessionEvent &aEvent);
Intended Usage: Called when MHFRunL leaves from a session event. This works in the same way as CActve::RunError. If you don't completely handle the error, a panic will occur.
|
|
virtual IMPORT_C void MHFUnload(RHTTPSession aSession, THTTPFilterHandle aHandle);
Intended Usage: Called when the filter is being removed from a session's filter queue.
|
virtual IMPORT_C void MHFLoad(RHTTPSession aSession, THTTPFilterHandle aHandle);
Intended Usage: Called when the filter is being added to the session's filter queue.
|
protected: IMPORT_C void TransactionCompletedL(RHTTPTransaction aTrans, THTTPEvent aEventStatus);
Callback method for concrete protocol handler sub-classes to inform the base protocol handler that a transaction has completed. The concrete protocol handler must call this method in order to supply a completion event that will be sent to the client. In addition, the method allows the base protocol handler to do some queue management.
|
|
protected: IMPORT_C TInt NumActiveTransactions() const;
Obtain the number of currently active transactions
|
protected: IMPORT_C void TransactionFailed(RHTTPTransaction aTrans);
Callback method for concrete protocol handler sub-classes to inform the base protocol handler that a transaction has failed utterly. (i.e. the sub-class used aTrans.Fail().) The base protocol handler sets the transaction state to be cancelled.
|
protected: IMPORT_C void CompleteSelf();
Completes this active object - allows the protocol handler to reevaluate the queue of pending transactions and service new ones if possible.
protected: IMPORT_C TInt FindTransaction(RHTTPTransaction aTransaction, const CProtTransaction *&aProtTransaction) const;
Searches the array of CProtTransaction
objects to if the aTransaction object is wrapped by one of them. If one is found aProtTransaction is set to it
|
|
private: virtual void CreateCodecL()=0;
Intended usage: Creates the specific type of codec required for a specific type of protocol handler.
This must be implemented by a concrete protocol handler sub-class.
private: virtual CProtTransaction *CreateProtTransactionL(RHTTPTransaction aTransaction)=0;
Intended Usage: Creates a representation of a client transaction to be used in the protocol handler. Since the protocol handler
deals with the low- level data for a transaction as sent over a particular transport, an appropriate CProtTransaction-derived
class is used that owns a CRxData
and a CTxData
to handle the low-level data.
This must be implemented by a concrete protocol handler sub-class.
|
|
|
private: virtual TBool ServiceL(CProtTransaction &aTrans)=0;
Intended Usage: Attempt to service the transaction. This implies that the concrete protocol handler will allocate some transport resources to the transaction - which could fail if the protocol handler has hit an internal limit of resources or bandwidth. Implementations of this interface may leave with any of KErrHttpInvalidUri, KErrGeneral, KErrNoMemory
This must be implemented by a concrete protocol handler sub-class.
|
|
private: virtual void ClosedTransactionHook(CProtTransaction *aTrans)=0;
Intended Usage: Called when the RHTTPTransaction
object corresponding to aTrans has been closed by the client. This allows the concrete protocol handler to do any cleanup
required for this particular transaction.
Ownership of the CProtTransaction
object is transferred back to the concrete protocol handler, which then has deletion responsibility for it. By the time this
function has been called, the base protocol handler will have dequeued the transaction.
The client's RHTTPTransaction
will be closed when this function returns, so it is not possible to send events to the client during the function's execution.
This must be implemented by a concrete protocol handler sub-class.
|
private: virtual void CancelTransactionHook(CProtTransaction &aTransaction)=0;
Intended Usage: Called when the RHTTPTransaction
object corresponding to aTrans has been cancelled by the client or an intermediate filter. This allows the concrete protocol
handler to do any cleanup and to perform the necessary actions for cancellation on its transport layer.
This must be implemented by a concrete protocol handler sub-class.
|
private: virtual void NotifyNewRequestBodyPart(CProtTransaction &aTransaction)=0;
Intended Usage: Called to notify the concrete protocol handler that new request body data is available for transmission.
This must be implemented by a concrete protocol handler sub-class.
|
protected: virtual IMPORT_C void RunL();
Intended Usage: Do some processing when a previous asynchronous request made by this object has completed.
protected: virtual IMPORT_C TInt RunError(TInt aError);
Intended Usage: Do any cleanup required should RunL leave
|
|
protected: virtual IMPORT_C void DoCancel();
Intended Usage: Cancel outstanding asynchronous requests that this object has made
protected: inline virtual void GetInterfaceL(TUid aInterfaceId, MProtHandlerInterface *&aInterfacePtr);
Intended Usage: This is a mechanism for allowing future change to CProtocolHandler API without breaking BC.
|
inline virtual void Reserved2();
Intended Usage: Reserve a slot in the v-table to preserve future BC
protected: RHTTPSession iSession;
The session to which this protocol handler is dedicated
protected: CHeaderCodec * iCodec;
The codec used for this protocol handler (to be specialised in subclasses)
protected: CSecurityPolicy * iSecurityPolicy;
An interface providing the security policy. This may be NULL if there is no security policy plugin