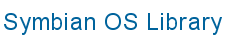
![]() |
![]() |
|
Location:
ImageDisplayPlugin.h
Link against: imagedisplay.lib
class CImageDisplayPlugin : public CBase;
This is the plugin API for the Image Display framework Intended for use by plugin writers only.
CBase
- Base class for all classes to be instantiated on the heap
CImageDisplayPlugin
- This is the plugin API for the Image Display framework Intended for use by plugin writers only
Defined in CImageDisplayPlugin
:
AsyncCallbackImageReady()
, CImageDisplayPlugin()
, CallbackIsRunning()
, CancelCallback()
, DestinationSizeInPixels()
, DisplayMode()
, EImgSrcDescriptor
, EImgSrcFileHandle
, EImgSrcFileName
, EImgSrcNotDefined
, EStatusBusy
, EStatusDisplayThisFrameIndefinetely
, EStatusEraseOutputContents
, EStatusFrameReady
, EStatusNoMoreToDecode
, EStatusPartialFrame
, EStatusPaused
, ExtensionInterface()
, GetBitmap()
, ImageStatus()
, MaintainAspectRatio()
, NumFrames()
, OpenL()
, Options()
, Pause()
, Play()
, RecommendedImageSizes()
, ReservedVirtual1()
, ReservedVirtual2()
, ReservedVirtual3()
, ReservedVirtual4()
, SourceData()
, SourceDataId()
, SourceDataIntent()
, SourceFileHandle()
, SourceFilename()
, SourceImageSubType()
, SourceImageType()
, SourceMimeType()
, SourceRect()
, SourceType()
, StopPlay()
, TImageSourceType
, TPluginStatus
, ValidBitmap()
, iImageSizes
, ~CImageDisplayPlugin()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
IMPORT_C ~CImageDisplayPlugin();
Virtual destructor. Current implementation will call the REComSession::DestroyedImplementation()
to finalize destruction of the ECom plugin
protected: virtual void OpenL()=0;
Initialise the plugin and check the image display settings.
This is called by the ImageDisplay framework when the client app calls CImageDisplay::SetupL()
.
The plugin should check the validity of the source image and all other settings set by the client API. If any of these is unsupported then it should leave with KErrArgument
A plugin implementing CImageDisplayPluginExtension to allow extension of the client API should initialise it here.
This is a virtual function that each individual plugin must implement.
|
protected: virtual void Play()=0;
Initiate the image display operation
This is a virtual function that each individual plugin must implement.
protected: virtual void Pause()=0;
Pause image decoding
This is a virtual function that each individual plugin must implement.
protected: virtual void StopPlay()=0;
Cancel the image display operation May be called by the framework even when there is no outstanding request.
This is a virtual function that each individual plugin must implement.
protected: virtual const CImageDisplay::RImageSizeArray &RecommendedImageSizes() const=0;
Should return an array of recommended image sizes i.e. sizes which would be processed faster This is a virtual function that each individual plugin must implement.
|
protected: IMPORT_C TImageSourceType SourceType() const;
returns an image datasource type
|
protected: IMPORT_C const TDesC &SourceFilename() const;
returns an image source file name
|
|
protected: IMPORT_C RFile &SourceFileHandle() const;
returns an image source file handle
|
|
protected: IMPORT_C const TDesC8 &SourceData() const;
returns an image source descriptor
|
|
protected: IMPORT_C ContentAccess::TIntent SourceDataIntent() const;
returns an intent that is requested by client for opening the image source
|
protected: IMPORT_C const TDesC &SourceDataId() const;
returns requested content data Id that should be used to open content
|
protected: IMPORT_C const TDesC8 &SourceMimeType() const;
returns an image source MIME type or KNullDesC8 if not defined
|
protected: IMPORT_C const TUid SourceImageType() const;
returns a global image type
|
protected: IMPORT_C const TUid SourceImageSubType() const;
returns a global image subtype or KNullUid if not defined
|
protected: IMPORT_C TBool SourceRect(TRect &aRect) const;
returns a requested by an API client source clipping rect
|
|
protected: IMPORT_C const TSize &DestinationSizeInPixels() const;
returns a requested by an API client destination image size
|
protected: IMPORT_C TUint Options() const;
returns a requested by an API client image options e.g. combination of the TImageOptions
|
protected: IMPORT_C TBool MaintainAspectRatio() const;
returns ETrue if an API client requested to preserve source image aspect ratio
|
protected: IMPORT_C TDisplayMode DisplayMode() const;
returns a requested by an API client display mode for an image being decoded
|
protected: virtual IMPORT_C TInt ExtensionInterface(TUid aIFaceUid, TAny *&aIFacePtr);
A plug-in that supports an extension interface has to override this function
|
|
protected: virtual IMPORT_C void GetBitmap(const CFbsBitmap *&aBitmap, const CFbsBitmap *&aMask) const;
A plug-in that supports image mask getting has to override this function
|
protected: virtual IMPORT_C TBool ValidBitmap() const;
A plug-in that supports target bitmap status checkig has to override this function
|
protected: virtual IMPORT_C TUint ImageStatus() const;
A plug-in that supports source image status checking has to override this function
|
protected: virtual IMPORT_C TInt NumFrames(TInt &aNumFrames) const;
A plug-in that supports getting of source image number of frames has to override this function
|
|
protected: IMPORT_C TBool CallbackIsRunning() const;
A plug-in may use this function to determine if a previuos callback function to an API client has been completed
|
protected: IMPORT_C void AsyncCallbackImageReady(const CFbsBitmap *aBitmap, TUint aStatus, const TRect &aUpdatedArea, TInt
aError);
A plug-in should use this function to pass information asinchronously to an API client. This function is asinchronous.
|
protected: IMPORT_C void CancelCallback();
A plug-in should use this function to cancel callback to a client if it is stil pending
TImageSourceType
Data source type definitions for plug-ins
|
TPluginStatus
Defines an ImageDisplay plug-in status flag values
|
protected: CImageDisplay::RImageSizeArray iImageSizes;
An array containing the sizes of the images.