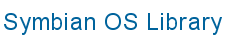
![]() |
![]() |
|
Location:
WSPEncoder.h
Link against: inetprotutil.lib
class CWspHeaderEncoder : public CBase;
This class can be used to encode one header field at a time, with all its values and parameters.
It has no knowledge of encoding the BNF of a particular header field, but the functions provided can be used in combination, producing an 8-bit buffer containing the encoded header.
Intended usage would be to call a series of functions. The first one being StartHeader, The final one being EndHeader, which
would return a buffer containing the complete encoded header field. eg: encoder->StartHeaderL()
; encoder->AddLongIntL()
; encoder->AddTextStringL()
; HBufC8* output = encoder->EndHeaderL()
;
CBase
- Base class for all classes to be instantiated on the heap
CWspHeaderEncoder
- This class can be used to encode one header field at a time, with all its values and parameters
Defined in CWspHeaderEncoder
:
AddDataL()
, AddDateL()
, AddIntegerL()
, AddLongIntL()
, AddShortIntL()
, AddShortLengthL()
, AddTextStringL()
, AddTextStringL()
, AddTokenL()
, AddTokenTextL()
, AddUintVarL()
, EndHeaderL()
, EndValueLengthL()
, NewL()
, NewLC()
, StartHeaderL()
, StartHeaderL()
, StartHeaderL()
, StartValueLengthL()
, ~CWspHeaderEncoder()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
static IMPORT_C CWspHeaderEncoder *NewL();
Static factory constructor.
|
|
static IMPORT_C CWspHeaderEncoder *NewLC();
Static factory constructor.
|
|
IMPORT_C void StartHeaderL(TUint8 aToken);
Starts a new encoded header.
|
|
IMPORT_C void StartHeaderL(const TDesC8 &aString);
Starts a new encoded header.
|
|
IMPORT_C void StartHeaderL(const RStringF aString);
Starts a new encoded header.
|
|
IMPORT_C HBufC8 *EndHeaderL();
The function StartHeaderL should have been called.
Completes and returns encoded field 8 bit buffer. This method will panic if an EndValueLengthL()
is not called after a StartValueLength().
Encoder is reset ready to be used again.
|
|
IMPORT_C void AddIntegerL(const TUint aInt);
StartHeaderL needs to have been called.
Encodes input Integer value and adds it to the encoded field. Choice of encoded form dependent on the size of the input.Either ShortInt or LongInt method chosen.
|
|
IMPORT_C void AddShortIntL(const TUint8 aValue);
StartHeaderL needs to have been called.
Encodes input and adds it to the encoded field. Encodes parameter value using WSP ShortInt method.
|
|
IMPORT_C void AddShortLengthL(const TUint8 aValue);
StartHeaderL needs to have been called.
Encodes input and adds it to the encoded field. For short length the value must be between octet 0 - 31.
|
|
IMPORT_C void AddLongIntL(const TUint32 aValue);
StartHeaderL needs to have been called.
Encodes input and adds it to the encoded field. Encodes parameter value using WSP LongInt method.
|
|
IMPORT_C void AddUintVarL(const TUint aInt);
StartHeaderL needs to have been called.
Encodes input and adds it to the encoded field. Encodes parameter value using WSP UIntVar method.
|
|
IMPORT_C void AddTextStringL(const RString &aText);
StartHeaderL needs to have been called.
Encodes input and adds it to the encoded field. Encodes parameter value using WSP TextString method.
|
|
IMPORT_C void AddTextStringL(const TDesC8 &aText);
StartHeaderL needs to have been called.
Encodes input and adds it to the encoded field. Encodes parameter value using WSP TextString method.
|
|
IMPORT_C void AddDateL(const TDateTime aDate);
StartHeaderL needs to have been called.
Encodes input and adds it to the encoded field.Encodes parameter value using WSP Date method.
|
|
IMPORT_C void AddTokenL(const TUint8 aToken);
StartHeaderL and StartValueLengthL should have been called.
Encodes input and adds it to the encoded field. Adds value as-is to the encoded field.
EndValueLengthL needs to be called subsequently.
|
IMPORT_C void AddTokenTextL(const TDesC8 &aTokenText);
Encodes input and adds it to the encoded field. Encodes parameter value using WSP TokenText method.
|
|
IMPORT_C void AddDataL(const TDesC8 &aData);
Encodes input and adds it to the encoded field. Adds value as-is to the encoded field.
|
|
IMPORT_C void StartValueLengthL();
StartHeaderL should have been called.
From calling this function, the length in bytes of all encodings added subsequently will be calculated and stored as part of the encoded string, as specified in WSP spec.Can be nested. i.e.
encoder->StartHeaderL();
encoder->StartValueLengthL();
encoder->StartValueLengthL();
encoder->AddLongIntL();
encoder->EndValueLengthL();
encoder->AddTextStringL();
encoder->EndValueLengthL();
HBufC8* output = encoder->EndHeaderL();
EndValueLengthL needs to be called subsequently.
|
IMPORT_C void EndValueLengthL();
StartHeaderL and StartValueLengthL should have been called.
Needs to be called at the point in the construction of a header when ValueLength can be calculated.
ValueLength has been calculated and added, together with the encoded header, to the internal representation of the header buffer.
|