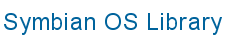
![]() |
![]() |
|
Location:
Mmfclip.h
class CMMFClip : public CBase, public MDataSource, public MDataSink;
Abstract class to represent a source or sink that contains a multimedia clip (i.e. not a stream or hardware device).
Typical examples are a file or an area of memory (descriptor).
MDataSink
- Abstract class representing a data sink
MDataSource
- Abstract class representing a data source
CBase
- Base class for all classes to be instantiated on the heap
CMMFClip
- Abstract class to represent a source or sink that contains a multimedia clip (i.e
Defined in CMMFClip
:
BytesFree()
, CMMFClip()
, Delete()
, ReadBufferL()
, ReadBufferL()
, ReadBufferL()
, SetSize()
, Size()
, WriteBufferL()
, WriteBufferL()
, WriteBufferL()
Inherited from CBase
:
Extension_()
,
operator new()
Inherited from MDataSink
:
BufferFilledL()
,
CanCreateSinkBuffer()
,
ConstructSinkL()
,
CreateSinkBufferL()
,
DataSinkType()
,
EmptyBufferL()
,
NegotiateL()
,
NewSinkL()
,
SetSinkDataTypeCode()
,
SetSinkPrioritySettings()
,
SinkCustomCommand()
,
SinkDataTypeCode()
,
SinkPauseL()
,
SinkPlayL()
,
SinkPrimeL()
,
SinkStopL()
,
SinkThreadLogoff()
,
SinkThreadLogon()
Inherited from MDataSource
:
BufferEmptiedL()
,
CanCreateSourceBuffer()
,
ConstructSourceL()
,
CreateSourceBufferL()
,
DataSourceType()
,
FillBufferL()
,
NegotiateSourceL()
,
NewSourceL()
,
SetSourceDataTypeCode()
,
SetSourcePrioritySettings()
,
SourceCustomCommand()
,
SourceDataTypeCode()
,
SourcePauseL()
,
SourcePlayL()
,
SourcePrimeL()
,
SourceSampleConvert()
,
SourceStopL()
,
SourceThreadLogoff()
,
SourceThreadLogon()
protected: inline CMMFClip(TUid aSourceType, TUid aSinkType);
Protected constructor.
|
virtual void ReadBufferL(TInt aLength, CMMFBuffer *aBuffer, TInt aPosition, MDataSink *aConsumer)=0;
Reads aLength number of bytes of data from the offset, aPosition into the buffer, aBuffer. Intended for asynchronous usage.
This is a virtual function that each derived class must implement.
|
virtual void WriteBufferL(TInt aLength, CMMFBuffer *aBuffer, TInt aPosition, MDataSource *aSupplier)=0;
Writes aLength number of bytes of data from the offset, aPosition from the buffer, aBuffer. Intended for asynchronous usage.
This is a virtual function that each derived class must implement.
|
virtual void ReadBufferL(CMMFBuffer *aBuffer, TInt aPosition, MDataSink *aConsumer)=0;
Reads the maximum number of bytes of data from the offset, aPosition into the buffer, aBuffer. Intended for asynchronous usage.
This is a virtual function that each derived class must implement.
|
virtual void WriteBufferL(CMMFBuffer *aBuffer, TInt aPosition, MDataSource *aSupplier)=0;
Writes the maximum number of bytes of data from the offset, aPosition from the buffer, aBuffer. Intended for asynchronous usage.
This is a virtual function that each derived class must implement.
|
virtual void ReadBufferL(CMMFBuffer *aBuffer, TInt aPosition)=0;
Reads the maximum number of bytes of data from the offset, aPosition into the buffer, aBuffer. Intended for synchronous usage.
This is a virtual function that each derived class must implement.
|
virtual void WriteBufferL(CMMFBuffer *aBuffer, TInt aPosition)=0;
Writes the maximum number of bytes of data from the offset, aPosition from the buffer, aBuffer. Intended for synchronous usage.
This is a virtual function that each derived class must implement.
|
virtual TInt64 BytesFree()=0;
Returns the amount of space available for the clip.
This is a virtual function that each derived class must implement.
|
virtual TInt Size()=0;
Returns the size of the clip in bytes.
This is a virtual function that each derived class must implement.
|
inline virtual TInt Delete();
Deletes the clip.
This should be overriden in the derived classes, the default version returns KErrNotSupported.
|
inline virtual TInt SetSize(TInt aSize);
Sets the size of the clip. This should be overriden in the derived classes, the default version returns KErrNotSupported.
|
|