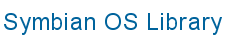
![]() |
![]() |
|
Location:
mmfdatapath.h
Link against: mmfserverbaseclasses.lib
class CMMFDataPath : public CActive, public MDataSink, public MDataSource, public MAsyncEventHandler;
Abstract utility class that moves data from a single data source to a single data sink, via a codec if required.
All functions are exported form the DLL and are virtual to allow plugins to define their own data paths.
MAsyncEventHandler
- Mixin class to define an object capable of handling an event within the controller framework
MDataSource
- Abstract class representing a data source
MDataSink
- Abstract class representing a data sink
CBase
- Base class for all classes to be instantiated on the heap
CActive
- The core class of the active object abstraction
CMMFDataPath
- Abstract utility class that moves data from a single data source to a single data sink, via a codec if required
Defined in CMMFDataPath
:
AddDataSinkL()
, AddDataSourceL()
, BufferEmptiedL()
, BufferFilledL()
, CMMFDataPath()
, CanCreateSinkBuffer()
, CanCreateSourceBuffer()
, ClearPlayWindowL()
, ConstructL()
, ConstructSinkL()
, ConstructSourceL()
, CreateSinkBufferL()
, CreateSinkBufferL()
, CreateSourceBufferL()
, CreateSourceBufferL()
, DoCancel()
, DoSendEventToClient()
, EConverting
, EEndOfData
, EInitializeSink
, EInitializeSource
, ENeedSinkBuffer
, ENeedSinkData
, ENeedSourceBuffer
, ENeedSourceData
, ENeedToMatchSourceToSink
, ENoBuffers
, EPlaying
, EPrimed
, ERecording
, ESendDataToSink
, EStopped
, EWaitSink
, EWaitSource
, EmptyBufferL()
, EndOfData()
, FillBufferL()
, NewL()
, NewL()
, NewL()
, NewL()
, Pause()
, PlayL()
, Position()
, PrimeL()
, ResetL()
, RunError()
, RunL()
, SendEventToClient()
, SetBlockLength()
, SetPlayWindowL()
, SetPositionL()
, SinkDataTypeCode()
, SourceDataTypeCode()
, State()
, Stop()
, TDataPathState
, TNeedBuffer
, TTransferState
, iAllDataSentToSink
, iBuffersToUse
, iCachedSourceDuration
, iCodec
, iCodecProcessResult
, iCompleteCallback
, iCurrentSinkFrameNumber
, iCurrentSourceFrameNumber
, iDataPathCompletedErrorCode
, iDataPathCreated
, iDataSink
, iDataSource
, iEventHandler
, iMediaId
, iNoMoreSourceData
, iObtainingAsyncSinkBuffer
, iObtainingAsyncSourceBuffer
, iPauseCalled
, iPlayWindowEndPosition
, iPlayWindowStartPosition
, iReferenceAudioSamplesPlayed
, iReferenceAudioSamplesRecorded
, iSinkBuffer
, iSinkBufferWithSink
, iSinkCanReceive
, iSinkFourCC
, iSnkBufRef
, iSourceBuffer
, iSourceBufferWithSource
, iSourceFourCC
, iSrcBufRef
, iStartPosition
, iState
, iTransferState
, iUseSuppliedCodecUid
, ~CMMFDataPath()
Inherited from CActive
:
Cancel()
,
Deque()
,
EPriorityHigh
,
EPriorityIdle
,
EPriorityLow
,
EPriorityStandard
,
EPriorityUserInput
,
Extension_()
,
IsActive()
,
IsAdded()
,
Priority()
,
SetActive()
,
SetPriority()
,
TPriority
,
iStatus
Inherited from CBase
:
Delete()
,
operator new()
Inherited from MDataSink
:
DataSinkType()
,
NegotiateL()
,
NewSinkL()
,
SetSinkDataTypeCode()
,
SetSinkPrioritySettings()
,
SinkCustomCommand()
,
SinkPauseL()
,
SinkPlayL()
,
SinkPrimeL()
,
SinkStopL()
,
SinkThreadLogoff()
,
SinkThreadLogon()
Inherited from MDataSource
:
DataSourceType()
,
NegotiateSourceL()
,
NewSourceL()
,
SetSourceDataTypeCode()
,
SetSourcePrioritySettings()
,
SourceCustomCommand()
,
SourcePauseL()
,
SourcePlayL()
,
SourcePrimeL()
,
SourceSampleConvert()
,
SourceStopL()
,
SourceThreadLogoff()
,
SourceThreadLogon()
static IMPORT_C CMMFDataPath *NewL(MAsyncEventHandler &aEventHandler);
Allocates and constructs a data path.
Use this function if the codec UID is not already known by CMMFController
and there is no data path ambiguity - ie only one data path is possible.
Will create codec via fourCC.
|
|
static IMPORT_C CMMFDataPath *NewL(TMediaId aMediaId, MAsyncEventHandler &aEventHandler);
Allocates and constructs a data path according to the specified media ID.
Use this function if the codec UID is not already known by CMMFController
and there is ambiguity with the data path ie. there is more than one possible data path.
|
|
static IMPORT_C CMMFDataPath *NewL(TUid aCodecUid, MAsyncEventHandler &aEventHandler);
Allocates and constructs a data path according to the specified codec UID.
Use this function if the codec UID is already known by CMMFController
and there is no data path ambiguity ie. only one data path is possible will create codec explicitly using the supplied codec
Uid
|
|
static IMPORT_C CMMFDataPath *NewL(TUid aCodecUid, TMediaId aMediaId, MAsyncEventHandler &aEventHandler);
Allocates and constructs a data path according to the specified codec UID.
Use this function if the codec UID is already known by CMMFController
and there is ambiguity ie. more than one possible data path. TMediaId
used to select the path.
|
|
protected: inline CMMFDataPath(TMediaId aMediaId, MAsyncEventHandler &aEventHandler);
|
protected: IMPORT_C void ConstructL(TUid aCodecUid=TUid::Null());
Takes UID of codec on construction, and if not an NULL codec sets the datapath up for codec instantiation.
|
virtual IMPORT_C void ResetL();
Deletes buffers if this datapath's sources and sinks own the buffers returned by PrimeL()
. Typically if buffers are created asychronously, the datapath doesn't own the buffer so leaves cleanup handling to the owner
sources/sinks.
Called when source and sink needs to be de-referenced. Sets iDataPathCreated, iSinkCanReceive, iSnkBufRef and iSrcBufRef to EFalse; sets iState to EStopped.
virtual IMPORT_C void EmptyBufferL(CMMFBuffer *aBuffer, MDataSource *aSupplier, TMediaId aMediaId);
Clears the specified buffer.
Pure virtual dummy implementation, not needed by datapath comes from MDataSink
- CMMFData path is a sink to its MDataSource
.
This is only required for an active push MDataSource
requesting a buffer empty.
|
virtual IMPORT_C void BufferFilledL(CMMFBuffer *aBuffer);
Indicates the data source has filled the specified buffer.
Called by the CMMFDataPath's MDataSource
when it has filled the buffer.
|
virtual IMPORT_C TBool CanCreateSinkBuffer();
Tests whether the data path can create a sink buffer.
The default implementation returns false.
|
virtual IMPORT_C CMMFBuffer *CreateSinkBufferL(TMediaId aMediaId);
Creates a sink buffer according to the specifed media ID.
Intended for synchronous usage (buffers supplied by datapath for an MDataSink
). This method is essentially a dummy implementation of an MDataSink
pure virtual.
The default implementation returns NULL.
|
|
virtual IMPORT_C CMMFBuffer *CreateSinkBufferL(TMediaId aMediaId, TBool &aReference);
Creates a sink buffer according to the specifed media ID and reference.
Intended for asynchronous usage (buffers supplied by Devsound device). This method is essentially a dummy implementation of
an MDataSink
pure virtual.
The default implementation returns NULL.
|
|
virtual IMPORT_C TFourCC SinkDataTypeCode(TMediaId aMediaId);
Gets the sink's data type for the specified media ID.
|
|
virtual IMPORT_C void FillBufferL(CMMFBuffer *aBuffer, MDataSink *aConsumer, TMediaId aMediaId);
Fills the specified buffer.
Pure virtual dummy implementation, not needed by datapath comes from MDataSink
- CMMFData path is a source to its MDataSink
Only required for an active pull MDataSink
requesting a buffer fill. The default implementation is empty.
|
virtual IMPORT_C void BufferEmptiedL(CMMFBuffer *aBuffer);
Indicates the data sink has emptied the buffer.
Called by the CMMFDataPath's MDataSink
when it has emptied the buffer
|
virtual IMPORT_C TBool CanCreateSourceBuffer();
Tests whether the data path can create a source buffer.
Would expect datapath to always return NULL, so this is a default implementation of a pure virtual from MDataSink
.
The default implementation returns EFalse.
|
virtual IMPORT_C CMMFBuffer *CreateSourceBufferL(TMediaId aMediaId);
Creates a source buffer.
Intended for synchronous usage (buffers supplied by datapath for a MDataSource
) This method is essentially a dummy implementation of an MDataSource
pure virtual.
The default implementation leaves with KErrNotSupported and returns NULL.
|
|
virtual IMPORT_C CMMFBuffer *CreateSourceBufferL(TMediaId aMediaId, TBool &aReference);
Creates a source buffer according to the specifed media ID and reference.
Intended for asynchronous usage (buffers supplied by datapath for a MDataSource
) This method is essentially a dummy implementation of an MDataSource
pure virtual.
The default implementation leaves with KErrNotSupported and returns NULL.
|
|
virtual IMPORT_C TFourCC SourceDataTypeCode(TMediaId aMediaId);
Gets the source data type for the specified media ID.
|
|
virtual IMPORT_C void AddDataSourceL(MDataSource *aSource);
Adds a data source to the datapath and, if the sink already exists, tries to establish a connection between the source and sink.
|
virtual IMPORT_C void AddDataSinkL(MDataSink *aSink);
Adds a data sink to the datapath and, if the source already exists, tries to establish a connection between the source and sink.
|
virtual IMPORT_C void PrimeL();
Allocates buffers in preparation to play.
Must be called before calling PlayL()
.
iSnkBufRef and iSrcBufRef contain ETrue if these buffers are created and owned by a MDataSource
or MDataSink
For clean-up purposes, datapath only cleans up buffers allocated directly by PrimeL()
.
virtual IMPORT_C void PlayL();
Starts an active scheduler 'play' loop.
Can only play from the primed state.
virtual IMPORT_C void Pause();
Pauses playing.
Sends KMMFErrorCategoryDataPathGeneralError to the client if an error occurs.
virtual IMPORT_C void Stop();
Stops playing.
Resets datapath position - currently does not clean up buffers. Sends KMMFErrorCategoryDataPathGeneralError to the client if an error occurs.
virtual IMPORT_C TTimeIntervalMicroSeconds Position() const;
Gets the data path position.
|
virtual IMPORT_C void SetPositionL(const TTimeIntervalMicroSeconds &aPosition);
Sets the data path position.
|
virtual IMPORT_C void SetPlayWindowL(const TTimeIntervalMicroSeconds &aStart, const TTimeIntervalMicroSeconds &aEnd);
Sets the play window absolutely (i.e. the parameters are relative to the start of the entire clip).
|
virtual IMPORT_C void ClearPlayWindowL();
Sets the play window to the full length of clip.
virtual IMPORT_C void RunL();
Runs the clip depending on the current data path and transfer state.
For example, fills the sink buffer if TDataPathState is EPlaying and TTransferState is ENeedSinkData.
virtual IMPORT_C void DoCancel();
Cancels the clip.
The default implementation is empty.
virtual IMPORT_C TInt RunError(TInt aError);
Handles errors coming from attached sources and passes them to the clients.
|
|
IMPORT_C TInt DoSendEventToClient(TUid aEventType, TInt aErrorCode);
Passes error handling and general messages up to clients.
|
|
IMPORT_C TInt SetBlockLength(TUint aBlockLength);
|
|
protected: virtual IMPORT_C void ConstructSourceL(const TDesC8 &aInitData);
Constructs a source.
The default implementation leaves with KErrNotSupported.
|
protected: virtual IMPORT_C void ConstructSinkL(const TDesC8 &aInitData);
Constructs a sink.
Overridable constuction specific to this datasource.
The default implementation leaves with KErrNotSupported.
|
protected: virtual IMPORT_C void EndOfData();
Forces and end of data state on the datapath
private: virtual IMPORT_C TInt SendEventToClient(const TMMFEvent &aEvent);
Passes error handling and general messages to clients.
|
|
TDataPathState
Indicates the state of the data path.
Mimics typical MultiMedia behaviour of stopped, primed and playing
|
protected: TTransferState
Indicates the transfer state.
Buffers maybe be filled, emptied, or have "one" shot initialisatings performed upon them.
TTransferState is used within the datapath RunL which drives databuffer exchange.
|
protected: TNeedBuffer
This indicates what buffers are required in order to operate. If a real Codec is in use, buffers are required from both source and sink, else only one is required and source is preferred.
|
protected: MDataSource * iDataSource;
The source of data to which the CMMFDataPath is a MDataSink
for.
protected: MDataSink * iDataSink;
The sink of data for which the CMMFDataPath is a MDataSource
for
protected: TBool iSinkCanReceive;
Set to true when the sink is able to accept data. EFalse otherwise.
protected: TFourCC iSinkFourCC;
The sink's data type. Same as the codec input data type.
protected: TFourCC iSourceFourCC;
The source's data type. Same as the codec output data type.
protected: TMediaId iMediaId;
Identifies which media type and stream within MDataSource
.
protected: TBool iDataPathCreated;
Set to ETrue when the data path has a source and a sink and can send data from the source to the sink.
protected: TBool iUseSuppliedCodecUid;
Set to true if data path has to use a supplied codec in its construction.
protected: CMMFBuffer * iSinkBuffer;
This is set to point to whichever sink buffer is in use.
protected: CMMFBuffer * iSourceBuffer;
This is the pointer to whichever source buffer is in use
protected: TUint iCurrentSourceFrameNumber;
The source's position in terms of frames or some other time fixed parameter.
protected: TUint iCurrentSinkFrameNumber;
The sink's position in terms of frames or some other time fixed parameter.
protected: TBool iNoMoreSourceData;
Indicates that all data has been obtained from the source (ETrue if there is no more source data).
protected: TUint iDataPathCompletedErrorCode;
Datapath completed because of an error; usually KErrNone.
protected: TTimeIntervalMicroSeconds iPlayWindowStartPosition;
Start position of the play window.
protected: TTimeIntervalMicroSeconds iPlayWindowEndPosition;
End position of the play window.
protected: TTimeIntervalMicroSeconds iStartPosition;
The position audio will start playing from. When stopping, this is set to iPlayWindowStartPosition. When pausing, this is set to the current position.
protected: TTimeIntervalMicroSeconds iCachedSourceDuration;
This value can be used to obtain the duration of the source when playing or converting. This is an optimisation as this value will not change if we are playing or converting.
protected: TBool iSrcBufRef;
ETrue if the source buffer is reference to object owned by someone else.
protected: TBool iSnkBufRef;
ETrue if sink buffer is reference to object owned by someone else
protected: TBool iObtainingAsyncSourceBuffer;
Indicates asynchrous buffers from AudioInput.
protected: TBool iObtainingAsyncSinkBuffer;
Indicates asynchrous buffers from AudioOutput.
protected: TBool iSourceBufferWithSource;
Flag to indicate that a buffer is with the source.
This is necessary as it is imperrative that when a buffer is with the source, it must not be referenced in any way. The reason for this is that it is not mandated that sources maintain buffer references. For example, it is valid for DevSound to return recorded data in a different buffer to the one supplied to it.
protected: TBool iSinkBufferWithSink;
Flag to indicate that a buffer is with the sink.
This are necessary as it is imperrative that when a buffer is with the sink, it must not be referenced in any way. The reason for this is that it is not mandated that sinks maintain buffer references. For example, it is valid for DevSound to request more audio data in a different buffer to the one supplied to it.
protected: TInt iReferenceAudioSamplesPlayed;
Holds the number of samples played on audio output at a point where we want to reference play duration from.
protected: TInt iReferenceAudioSamplesRecorded;
Holds the number of samples recorded from audio input at a point where we want to reference record duration from.
protected: CCompleteCallback * iCompleteCallback;
Pointer to internal callback completion class
protected: TInt iBuffersToUse;
Holds the outcome of the call to DetermineBuffersToUseL