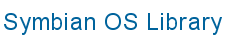
![]() |
![]() |
|
Location:
mmfswcodecwrapper.h
class CMMFSwCodec : public CBase;
Class for a software codec used by the CMMFSwCodecWrapper
class to make the CMMFSwCodec a CMMFHwDevice
plugin. The CMMFSwCodec processes source data in a certain fourCC coding type and converts it to a destination buffer of
another fourCC coding type.
A CMMFSwCodec object would usually not be instantiated directly but instead would be instantiated via the CMMFSwCodecWrapper
class's Codec() method.
The processing of the data is handled by the codecs ProcessL()
member. The intention is that the source buffer for conversion is converted to the appropriate coding type in the destination
buffer. The size of the buffers passed in are determined by SourceBufferSize()
and SinkBufferSize()
methods. The buffer sizes should be chosen such that the ProcessL()
method can be guaranteed to have enough destination buffer to completely process one source buffer.
The ProcessL should return a TCodecProcessResult
returning the number of source bytes processed and the number of destination bytes processed along with a process result
code defined thus:
EProcessComplete: the codec processed all the source data into the sink buffer
EProcessIncomplete: the codec filled sink buffer before all the source buffer was processed
EDstNotFilled: the codec processed the source buffer but the sink buffer was not filled
EEndOfData: the codec detected the end data - all source data in processed but sink may not be full
EProcessError: the codec process error condition
Unlike the 7.0s CMMFCodec::ProcessL
method, the CMMFSwCodec::ProcessL
method should not return EProcessIncomplete as this case is not handled by the CMMFSwCodecWrapper
.
CBase
- Base class for all classes to be instantiated on the heap
CMMFSwCodec
- Class for a software codec used by the
Defined in CMMFSwCodec
:
ProcessL()
, SinkBufferSize()
, SourceBufferSize()
, TCodecProcessResult
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
virtual TCodecProcessResult ProcessL(const CMMFBuffer &aSource, CMMFBuffer &aDest)=0;
Processes the data in the specified source buffer and writes the processed data to the specified destination buffer.
This function is synchronous, when the function returns the data has been processed. This is a virtual function that each derived class must implement.
|
|
virtual TUint SourceBufferSize()=0;
Gets the max size of the source buffer passed into the CMMFSwCodec::ProcessL
function.
Note that this means that this is the Max size of each buffer passed to the codec. The actual size of the data could be less than the max size. This is a virtual function that each derived class must implement.
|
virtual TUint SinkBufferSize()=0;
Gets the max size of the sink (destination) buffer passed into the CMMFSwCodec::ProcessL
method.
Note that this means that this is the Max size of each buffer passed to the codec. The actual size of the data written to this buffer could be less than the max size. This is a virtual function that each derived class must implement.
|
class TCodecProcessResult;
Indicates the result of processing data from the source buffer to a destination buffer and provides functions to compare the
result code. The CMMFSwCodec
buffer sizes should be set to return EProcessComplete The other return codes are to keep the ProcessL method compatible with
the 7.0s CMMFCodec
API.
Defined in CMMFSwCodec::TCodecProcessResult
:
EDstNotFilled
, EEndOfData
, EProcessComplete
, EProcessError
, EProcessIncomplete
, TCodecProcessResult()
, TCodecProcessResultStatus
, iCodecProcessStatus
, iDstBytesAdded
, iSrcBytesProcessed
, operator!=()
, operator==()
TCodecProcessResult()
inline TCodecProcessResult();
Default constructor.
operator==()
inline TBool operator==(const TCodecProcessResultStatus aStatus) const;
Overloaded operator to test equality.
|
|
operator!=()
inline TBool operator!=(const TCodecProcessResultStatus aStatus) const;
Overloaded operator to test inequality.
|
|
TCodecProcessResultStatus
TCodecProcessResultStatus
Flag to track the codec's processing status.
|
iCodecProcessStatus
TCodecProcessResultStatus iCodecProcessStatus;
The codec's processing status
iSrcBytesProcessed
TUint iSrcBytesProcessed;
The number of source bytes processed
iDstBytesAdded
TUint iDstBytesAdded;
The number of bytes added to the destination buffer