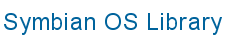
![]() |
![]() |
|
Location:
MdaAudioTonePlayer.h
Link against: mediaclientaudio.lib
class CMdaAudioToneUtility : public CBase, public MMMFClientUtility;
Generates tones on an audio capable EPOC device.
The class offers an interface for generating tones on all audio capable EPOC devices.
To use the tone utility:
1. Create an instance by calling NewL()
.
2. Call the appropriate PrepareToPlay variant for the required tone type and wait for the callback indicating success.
3. Call Play and either wait for the callback to indicate completion, or call CancelPlay to end playback early.
4. Delete the instance.
It is possible to call Play before calling any PrepareToPlay variant. This will result in a default fixed sequence tone being played.
MMMFClientUtility
- No description.
CBase
- Base class for all classes to be instantiated on the heap
CMdaAudioToneUtility
- Generates tones on an audio capable EPOC device
Defined in CMdaAudioToneUtility
:
CancelPlay()
, CancelPrepare()
, CustomInterface()
, FixedSequenceCount()
, FixedSequenceName()
, GetBalanceL()
, MaxVolume()
, NewL()
, NewL()
, Play()
, PrepareToPlayDTMFString()
, PrepareToPlayDesSequence()
, PrepareToPlayDualTone()
, PrepareToPlayFileSequence()
, PrepareToPlayFileSequence()
, PrepareToPlayFixedSequence()
, PrepareToPlayTone()
, SetBalanceL()
, SetDTMFLengths()
, SetPriority()
, SetRepeats()
, SetVolume()
, SetVolumeRamp()
, State()
, Volume()
, iProperties
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Capability: | MultimediaDD | A process requesting or using this method that has MultimediaDD capability will always have precedence over a process that does not have MultimediaDD. |
static IMPORT_C CMdaAudioToneUtility *NewL(MMdaAudioToneObserver &aObserver, CMdaServer *aServer=0);
Creates a new instance of the tone player utility. The default volume is set to MaxVolume()
/ 2.
|
|
Capability: | MultimediaDD | A process requesting or using this method that has MultimediaDD capability will always have precedence over a process that does not have MultimediaDD. |
static IMPORT_C CMdaAudioToneUtility *NewL(MMdaAudioToneObserver &aObserver, CMdaServer *aServer, TInt aPriority, TMdaPriorityPreference
aPref=EMdaPriorityPreferenceTimeAndQuality);
Creates a new instance of the tone player utility. The default volume is set to MaxVolume()
/ 2.
|
|
virtual TMdaAudioToneUtilityState State();
Returns the current state of the audio tone utility.
|
virtual TInt MaxVolume();
Returns the maximum volume supported by the device. This is the maximum value which can be passed to CMdaAudioToneUtility::SetVolume()
.
|
virtual TInt Volume();
Returns an integer representing the current volume of the audio device.
|
virtual void SetVolume(TInt aVolume);
Changes the volume of the audio device.
The volume can be changed before or during play and is effective immediately.
|
Capability: | MultimediaDD | A process requesting or using this method that has MultimediaDD capability will always have precedence over a process that does not have MultimediaDD. |
virtual void SetPriority(TInt aPriority, TMdaPriorityPreference aPref);
Changes the clients priority.
|
virtual void SetDTMFLengths(TTimeIntervalMicroSeconds32 aToneLength, TTimeIntervalMicroSeconds32 aToneOffLength, TTimeIntervalMicroSeconds32
aPauseLength);
Changes the duration of DTMF tones, the gaps between DTMF tones and the pauses.
|
virtual void SetRepeats(TInt aRepeatNumberOfTimes, const TTimeIntervalMicroSeconds &aTrailingSilence);
Sets the number of times the tone sequence is to be repeated during the play operation.
A period of silence can follow each playing of the tone sequence. The tone sequence can be repeated indefinitely.
|
virtual void SetVolumeRamp(const TTimeIntervalMicroSeconds &aRampDuration);
Defines the period over which the volume level is to rise smoothly from nothing to the normal volume level.
|
virtual TInt FixedSequenceCount();
Returns the number of available pre-defined tone sequences.
|
virtual const TDesC &FixedSequenceName(TInt aSequenceNumber);
Returns the name assigned to a specific pre-defined tone sequence.
|
|
virtual void PrepareToPlayTone(TInt aFrequency, const TTimeIntervalMicroSeconds &aDuration);
Configures the audio tone player utility to play a single tone.
This function is asynchronous. On completion, the observer callback function MMdaAudioToneObserver::MatoPrepareComplete()
is called, indicating the success or failure of the configuration operation.The configuration operation can be cancelled
by calling CMdaAudioToneUtility::CancelPrepare()
. The configuration operation cannot be started if a play operation is in progress.
|
virtual void PrepareToPlayDTMFString(const TDesC &aDTMF);
Configures the audio tone utility player to play a DTMF (Dual-Tone Multi-Frequency) string.
This function is asynchronous. On completion, the observer callback function MMdaAudioToneObserver::MatoPrepareComplete()
is called, indicating the success or failure of the configuration operation. The configuration operation can be cancelled
by calling CMdaAudioToneUtility::CancelPrepare()
. The configuration operation cannot be started if a play operation is in progress.
|
virtual void PrepareToPlayDesSequence(const TDesC8 &aSequence);
Configures the audio tone player utility to play a tone sequence contained in a descriptor.
This function is asynchronous. On completion, the observer callback function MMdaAudioToneObserver::MatoPrepareComplete()
is called, indicating the success or failure of the configuration operation. The configuration operation can be cancelled
by calling CMdaAudioToneUtility::CancelPrepare()
. The configuration operation cannot be started if a play operation is in progress.
|
virtual void PrepareToPlayFileSequence(const TDesC &aFileName);
Configures the audio tone player utility to play a tone sequence contained in a file.
This function is asynchronous. On completion, the observer callback function MMdaAudioToneObserver::MatoPrepareComplete()
is called, indicating the success or failure of the configuration operation. The configuration operation can be cancelled
by calling CMdaAudioToneUtility::CancelPrepare()
. The configuration operation cannot be started if a play operation is in progress.
|
virtual void PrepareToPlayFixedSequence(TInt aSequenceNumber);
Configures the audio tone player utility to play the specified pre-defined tone sequence.
This function is asynchronous. On completion, the observer callback function MMdaAudioToneObserver::MatoPrepareComplete()
is called, indicating the success or failure of the configuration operation. The configuration operation can be cancelled
by calling CMdaAudioToneUtility::CancelPrepare()
. The configuration operation cannot be started if a play operation is in progress.
|
virtual void CancelPrepare();
Cancels the configuration operation.
The observer callback function MMdaAudioToneObserver::MatoPrepareComplete()
is not called.
virtual void Play();
Plays the tone.
The tone played depends on the current configuration.This function is asynchronous. On completion, the observer callback function
MMdaAudioToneObserver::MatoPlayComplete()
is called, indicating the success or failure of the play operation.The play operation can be cancelled by calling CMdaAudioToneUtility::CancelPlay()
.
virtual void CancelPlay();
Cancels the tone playing operation.
The observer callback function MMdaAudioToneObserver::MatoPlayComplete()
is not called.
IMPORT_C void SetBalanceL(TInt aBalance=KMMFBalanceCenter);
Sets the stereo balance for playback.
|
IMPORT_C TInt GetBalanceL();
Returns The current playback balance.This function may not return the same value as passed to SetBalanceL depending on the internal implementation in the underlying components.
|
IMPORT_C void PrepareToPlayDualTone(TInt aFrequencyOne, TInt aFrequencyTwo, const TTimeIntervalMicroSeconds &aDuration);
Configures the audio tone player utility to play a dual tone. The generated tone consists of two sine waves of different frequencies summed together.
This function is asynchronous. On completion, the observer callback function MMdaAudioToneObserver::MatoPrepareComplete()
is called, indicating the success or failure of the configuration operation. The configuration operation can be cancelled
by calling CMdaAudioToneUtility::CancelPrepare()
. The configuration operation cannot be started if a play operation is in progress.
|
IMPORT_C void PrepareToPlayFileSequence(RFile &aFile);
Configures the audio tone player utility to play a tone sequence contained in a file.
This function is asynchronous. On completion, the observer callback function MMdaAudioToneObserver::MatoPrepareComplete()
is called, indicating the success or failure of the configuration operation. The configuration operation can be cancelled
by calling CMdaAudioToneUtility::CancelPrepare()
. The configuration operation cannot be started if a play operation is in progress.
|
IMPORT_C TAny *CustomInterface(TUid aInterfaceId);
Retrieves a custom interface to the underlying device.
|
|
protected: CMMFMdaAudioToneUtility * iProperties;
This member is internal and not intended for use.