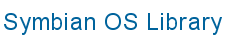
![]() |
![]() |
|
Location:
MIUTHDR.H
Link against: imcm.lib
class CImHeader : public CBase;
Encapsulates an Internet Mail (RFC822) header.
Note that the class contains some obsolete functions that take a narrow descriptor: always use the equivalent wide descriptor function instead.
CBase
- Base class for all classes to be instantiated on the heap
CImHeader
- Encapsulates an Internet Mail (RFC822) header
Defined in CImHeader
:
AddEncodingInfoL()
, BccRecipients()
, BccRecipients()
, BodyEncoding()
, CcRecipients()
, CcRecipients()
, Charset()
, CreateForwardL()
, CreateReceiptL()
, CreateReplyL()
, DataSize()
, EAll
, EOriginator
, ERecipients
, ESender
, EncodingInfo()
, EncodingInfo()
, ExternalizeL()
, From()
, ImMsgId()
, InReplyTo()
, InternalizeL()
, NewLC()
, OverrideCharset()
, ReDecodeL()
, ReceiptAddress()
, RemoteSize()
, ReplyTo()
, ResentBccRecipients()
, ResentBccRecipients()
, ResentCcRecipients()
, ResentCcRecipients()
, ResentFrom()
, ResentMsgId()
, ResentToRecipients()
, ResentToRecipients()
, Reset()
, RestoreL()
, SetBodyEncoding()
, SetCharset()
, SetFromL()
, SetFromL()
, SetImMsgIdL()
, SetInReplyToL()
, SetOverrideCharset()
, SetReceiptAddressL()
, SetReceiptAddressL()
, SetRemoteSize()
, SetReplyToL()
, SetReplyToL()
, SetResentFromL()
, SetResentMsgIdL()
, SetSubjectL()
, SetSubjectL()
, SetVersion()
, StoreL()
, StoreWithoutCommitL()
, Subject()
, TReplyTo
, ToRecipients()
, ToRecipients()
, Version()
, ~CImHeader()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
static IMPORT_C CImHeader *NewLC();
Allocates and creates a new CImHeader object, leaving the object on the cleanup stack.
|
IMPORT_C void InternalizeL(RMsvReadStream &aReadStream);
Internalises the settings from a specified stream.
|
IMPORT_C void ExternalizeL(RMsvWriteStream &aWriteStream) const;
Externalises the settings to a specified stream.
|
IMPORT_C void RestoreL(CMsvStore &aMessageStore);
Restores settings from a specified message store.
|
IMPORT_C void StoreL(CMsvStore &aMessageStore) const;
Stores, but does not commit, settings to a specified message store.
|
IMPORT_C void StoreWithoutCommitL(CMsvStore &aMessageStore) const;
Stores, but does not commit, settings to a specified message store.
|
IMPORT_C const TPtrC Subject() const;
Gets the "Subject" header field.
|
IMPORT_C void SetSubjectL(const TDesC8 &aSubject);
Sets the "Subject" header field.
|
IMPORT_C const TPtrC8 ImMsgId() const;
Gets the "MessageId" header field.
|
IMPORT_C void SetImMsgIdL(const TDesC8 &aImMsgIdL);
Sets the "MessageId" header field.
|
IMPORT_C const TPtrC From() const;
Gets the "From" header field.
This consists of an address and (possibly) an alias.
|
IMPORT_C void SetFromL(const TDesC8 &aFrom);
Sets the "From" header field.
|
IMPORT_C const TPtrC ReplyTo() const;
Gets the "ReplyTo" header field.
|
IMPORT_C void SetReplyToL(const TDesC8 &aReplyTo);
Sets the "ReplyTo" header field.
|
IMPORT_C const TPtrC ReceiptAddress() const;
Gets the "Receipt" header field.
|
IMPORT_C void SetReceiptAddressL(const TDesC8 &aReceiptAddress);
Sets the "Receipt" header field.
|
IMPORT_C TUint Charset() const;
Gets the character set to use when sending the message header.
If set, this overrides the default system character set for sending the header.
Character set and encoding options can also be set on a per header field basis using TImHeaderEncodingInfo
objects. See EncodingInfo()
.
|
IMPORT_C void SetCharset(const TUint aCharset);
Sets the character set to use when sending the message header.
This setting overrides the default system character set for sending the header.
Character set and encoding options can also be set on a per header field basis using TImHeaderEncodingInfo
objects. See AddEncodingInfoL()
.
|
IMPORT_C void SetFromL(const TDesC16 &aFrom);
Sets the "From" header field.
|
IMPORT_C void SetReplyToL(const TDesC16 &aReplyTo);
Sets the "ReplyTo" header field.
|
IMPORT_C void SetReceiptAddressL(const TDesC16 &aReceiptAddress);
Sets the "Receipt" header field.
|
IMPORT_C void SetSubjectL(const TDesC16 &aSubject);
Sets the "Subject" header field.
|
IMPORT_C TInt DataSize();
Gets the combined length of all the field values stored.
|
inline const CDesCArray &ToRecipients() const;
Gets a const list of "To" recipients.
|
inline const CDesCArray &CcRecipients() const;
Gets a const list of "Cc" recipients.
|
inline const CDesCArray &BccRecipients() const;
Gets a const list of "Bcc" recipients.
|
inline CDesCArray &ToRecipients();
Gets a list of "To" recipients.
|
inline CDesCArray &CcRecipients();
Gets a list of "Cc" recipients.
|
inline CDesCArray &BccRecipients();
Gets a list of "Bcc" recipients.
|
inline CArrayFix< TImHeaderEncodingInfo > &EncodingInfo();
Gets information relating to the encoding of header fields in received email.
This includes the charset. This information can be used when forwarding the email, to re-encode the header fields.
|
inline const CArrayFix< TImHeaderEncodingInfo > &EncodingInfo() const;
Gets const information relating to the encoding of header fields in received email.
This includes the charset. This information can be used when forwarding the email, to re-encode the header fields.
|
IMPORT_C void AddEncodingInfoL(TImHeaderEncodingInfo &aInfo);
Adds header field encoding information.
|
IMPORT_C const TPtrC8 InReplyTo() const;
Gets the "In Reply To" header field.
For reply messages, this field stores the ID of the message to which this is a reply. It is set by CImHeader::CreateReplyL()
.
|
IMPORT_C void SetInReplyToL(const TDesC8 &);
Sets the "In Reply To" header field.
For reply messages, this field stores the ID of the message to which this is a reply.
|
IMPORT_C TInt CreateForwardL(CImHeader &, TDesC &);
Populates a new forward header.
The subject line passed in is used to construct the forward subject field value. This is then stored in the new header.
|
|
IMPORT_C TInt CreateReplyL(CImHeader &, TReplyTo, TDesC &);
Populates a new Reply header.
The subject line passed in is used to construct the Reply subject field value. This is then stored in the new header.
|
|
IMPORT_C void CreateReceiptL(CImHeader &, TDesC &);
Populates a Receipt email header.
|
IMPORT_C TMsgOutboxBodyEncoding BodyEncoding() const;
Gets the method of encoding the body of the email message.
The default value (EMsgOutboxMIME) is set so that text parts of the message are sent as MIME multipart/alternative text/html parts, and are encoded using UTF-8.
|
IMPORT_C void SetBodyEncoding(TMsgOutboxBodyEncoding aMessageBodyEncoding);
Sets the method of encoding the body of the email message.
The default value (EMsgOutboxMIME) is set so that text parts of the message are sent as MIME multipart/alternative text/html parts, and are encoded using UTF-8.
|
IMPORT_C void ReDecodeL(RFs &aFS);
Decodes the original message data into the CImHeader fields using the override character set.
8 bit data MUST be decoded using the normal method initially before this method is called.
|
|
IMPORT_C TUint OverrideCharset() const;
Returns the character set to be used when calling ReDecodeL.
|
IMPORT_C void SetOverrideCharset(TUint aCharset);
Sets the character set to be used when calling ReDecodeL.
|
TReplyTo
Flags used to determine where to obtain the ReplyTo address for the header field of that name.
|