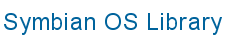
![]() |
![]() |
|
Location:
SHGAPI.H
Link against: sheng.lib
class CSheetEngine : public CBase;
Spreadsheet application engine.
CBase
- Base class for all classes to be instantiated on the heap
CSheetEngine
- Spreadsheet application engine
Defined in CSheetEngine
:
CSheetEngine()
, Compress()
, ConstructL()
, ConstructL()
, ConstructL()
, ConstructL()
, CreateCellFromTextL()
, CurrentWorkSheet()
, DisableUndoAndRedo()
, EForceText
, ERedoDataAvailable
, EUndoDataAvailable
, EndEditOperationL()
, ExistsCircularDependency()
, FormulaViewer()
, GetAnyCircularDependency()
, HasChanged()
, IsRedoAvailable()
, IsUndoAvailable()
, LocaleHasChanged()
, MarkUnchanged()
, NameSet()
, NewEmptyL()
, NewL()
, NewL()
, NewL()
, NewL()
, NewLC()
, RecalculateL()
, RecalculationInProgress()
, RecalculationNeeded()
, RecalculationToBeDoneAutomatically()
, RecalculationToBeDoneInBackground()
, RedoOperationL()
, Reset()
, RestoreL()
, SetCallBackAPI()
, SetCurrentWorkSheet()
, SetRecalculationToBeDoneAutomatically()
, SetRecalculationToBeDoneInBackgroundL()
, SetTemplateAdditionalDataL()
, ShgCellAsText()
, ShgCellAsText()
, StartEditOperation()
, StoreL()
, SyntaxErrorPos()
, TCleanupStackableRollBack
, TemplateAdditionalData()
, UndoOperationL()
, WorkBook()
, anonymous
, anonymous
, ~CSheetEngine()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
static IMPORT_C CSheetEngine *NewL();
Allocates and constructs a new spreadsheet engine object.
|
static IMPORT_C CSheetEngine *NewLC();
Allocates and constructs a new spreadsheet engine object, leaving the object on the cleanup stack.
|
static IMPORT_C CSheetEngine *NewL(CShgCellAdditionalData *aAdditionalData);
Allocates and constructs a new spreadsheet engine object, specifying additional cell formatting data.
|
|
static IMPORT_C CSheetEngine *NewL(CShgCellAdditionalData *aAdditionalData, const TDesC &aResourceFileName);
Allocates and constructs a new spreadsheet engine object, specifying additional cell formatting data and a resource file.
The engine's resource file specifies text for formulae and errors.
|
|
static IMPORT_C CSheetEngine *NewL(const TDesC &aResourceFileName);
Allocates and constructs a new spreadsheet engine object, specifying a resource file.
The engine's resource file specifies text for formulae and errors.
|
|
protected: IMPORT_C void ConstructL(CShgCellAdditionalData *aAdditionalData);
Second-phase constructor, specifying a resource file.
|
protected: IMPORT_C void ConstructL(CShgCellAdditionalData *aAdditionalData, const TDesC &aResourceFileName);
Second-phase constructor, specifying additional cell formatting data and a resource file.
|
protected: IMPORT_C void ConstructL(const TDesC &aResourceFileName);
Second-phase constructor, specifying a resource file.
|
static IMPORT_C CSheetEngine *NewEmptyL(const CSheetEngine *aSheetEngine);
Allocates and constructs a new spreadsheet engine object, specifying an existing spreadsheet engine as a template.
|
|
IMPORT_C void SetCallBackAPI(MShgCallBackAPI *aCallBackAPI);
Sets a callback object, so that the engine can inform the client of events.
|
IMPORT_C void LocaleHasChanged();
Informs the engine that the system locale has changed.
IMPORT_C TBool HasChanged() const;
Tests if the engine has performed updates that the client (e.g. UI) may need to get.
Once all updates have been processed, use MarkUnchanged()
to reset the changed flag.
|
IMPORT_C TStreamId StoreL(CStreamStore &aStore) const;
Stores the engine.
|
|
IMPORT_C void RestoreL(const CStreamStore &aStore, TStreamId aId);
Restores the engine.
|
IMPORT_C TShgError CreateCellFromTextL(TShgCell &aCell, const TDesC &aText, const CShgWorkSheet *aWorkSheet);
Initialises a cell with content specified in a descriptor.
The function attempts to parse the descriptor content to set the cell type and content.
|
|
IMPORT_C void ShgCellAsText(TDes &aDes, const TShgCell &aShgCell) const;
Gets the cell contents as a descriptor.
|
IMPORT_C void ShgCellAsText(TDes &aDes, const TShgCell &aShgCell, TInt aWorkSheetNo) const;
Gets the cell contents as a descriptor, specifying the cell's work sheet.
|
IMPORT_C void SetRecalculationToBeDoneAutomatically(TBool aRecalculationToBeDoneAutomatically);
Sets whether the engine should automatically recalculate the spreadsheet when required.
|
IMPORT_C TBool RecalculationToBeDoneAutomatically() const;
Tests if the engine automatically recalculates the spreadsheet when required.
|
IMPORT_C void SetRecalculationToBeDoneInBackgroundL(TBool aRecalculationToBeDoneInBackground);
Sets whether the engine should recalculate the spreadsheet in the background, as a low priority active object, or synchronously.
|
IMPORT_C TBool RecalculationToBeDoneInBackground() const;
Tests if the engine recalculates the spreadsheet in the background, as a low priority active object, or synchronously.
|
IMPORT_C void RecalculateL();
Recalculates the spreadsheet.
This can be a synchronous or asynchronous operation, depending on the value of RecalculationToBeDoneInBackground()
.
IMPORT_C TBool RecalculationNeeded() const;
Tests if the spreadsheet needs recalculating.
|
IMPORT_C TBool RecalculationInProgress() const;
Tests if a background recalculation is in progress.
|
IMPORT_C TBool ExistsCircularDependency() const;
Tests if circular dependency exists between cells.
|
IMPORT_C void GetAnyCircularDependency(TBool &aKnown, TInt &aWorkSheetNo, TCellRef &aCoord) const;
Finds a cell causing a circular dependency.
|
IMPORT_C void DisableUndoAndRedo();
Sets the engine to not provide support for undoing and redoing operations.
IMPORT_C TBool IsUndoAvailable() const;
Tests if the engine supports undo.
|
IMPORT_C TBool IsRedoAvailable() const;
Tests if the engine supports redo.
|
IMPORT_C CShgNameSet *NameSet() const;
Gets the engine's name set.
|
IMPORT_C void SetCurrentWorkSheet(const TDesC &aName);
Sets the engine's current worksheet.
|
IMPORT_C CShgWorkSheet *CurrentWorkSheet() const;
Gets the engine's current worksheet.
|
IMPORT_C CShgWorkBook *WorkBook() const;
Gets the engine's workbook.
|
IMPORT_C TInt SyntaxErrorPos() const;
Gets the position where a formula syntax error has been detected.
|
IMPORT_C CShgFormulaViewer *FormulaViewer() const;
Gets the engine's formula viewer.
|
IMPORT_C void SetTemplateAdditionalDataL(CShgCellAdditionalData *aData);
Sets default additional cell formatting data.
|
IMPORT_C const CShgCellAdditionalData *TemplateAdditionalData() const;
Gets default additional cell formatting data.
|
IMPORT_C TShgError EndEditOperationL(TShgError aShgError);
Marks the end of an edit operation.
|
|
class TCleanupStackableRollBack : public TCleanupItem;
TCleanupItem
- Encapsulates a cleanup operation and an object on which the operation is to be performed
CSheetEngine::TCleanupStackableRollBack
- (No abstract)