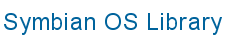
![]() |
![]() |
|
Location:
S32STOR.H
Link against: estor.lib
class CEmbeddedStore : public CPersistentStore;
Encapsulates an embedded store.
The embedded store may contain an arbitrarily complex network of streams, but is viewed as simply another stream by the embedding store. This means that the embedded store can dealt with as a single stream for purposes of copying or deleting.
Once streams within the embedded store have been committed and closed, they cannot subsequently be changed, i.e. streams cannot be replaced, deleted, extended or changed in any way.
CBase
- Base class for all classes to be instantiated on the heap
CStreamStore
- Provides the core abstract framework for stores allowing streams to be created and manipulated
CPersistentStore
- Persistent store abstract base class
CEmbeddedStore
- Encapsulates an embedded store
Defined in CEmbeddedStore
:
CEmbeddedStore()
, ConstructL()
, Detach()
, DoCommitL()
, DoCreateL()
, DoReadL()
, DoSetRootL()
, FromL()
, FromLC()
, Host()
, MarshalL()
, NewL()
, NewLC()
, Position()
, Reattach()
, Start()
, ~CEmbeddedStore()
Inherited from CBase
:
Extension_()
,
operator new()
Inherited from CPersistentStore
:
Root()
,
SetRootL()
,
iRoot
Inherited from CStreamStore
:
Commit()
,
CommitL()
,
CompactL()
,
Delete()
,
DeleteL()
,
DoCompactL()
,
DoDeleteL()
,
DoExtendL()
,
DoReclaimL()
,
DoReplaceL()
,
DoRevertL()
,
DoWriteL()
,
ExtendL()
,
ReclaimL()
,
Revert()
,
RevertL()
static IMPORT_C CEmbeddedStore *NewL(RWriteStream &aHost);
Creates an embedded store within the specified host stream.
Note that ownership of the stream passes to the store and the referenced RWriteStream
is cleared.
|
|
static IMPORT_C CEmbeddedStore *NewLC(RWriteStream &aHost);
Creates an embedded store within the specified host stream, putting a pointer to the store onto the cleanup stack.
Putting a pointer to the embedded store object onto the cleanup stack allows the object and allocated resources to be cleaned up if a subsequent leave occurs.
Note that ownership of the stream passes to the store and the referenced RWriteStream
is cleared.
|
|
IMPORT_C ~CEmbeddedStore();
Frees resources owned by the object, prior to its destruction. In particular, the destructor closes the associated file.
static IMPORT_C CEmbeddedStore *FromL(RReadStream &aHost);
Opens the store hosted by the specified stream.
Note that ownership of the stream passes to the store; the referenced RReadStream
is cleared.
|
|
static IMPORT_C CEmbeddedStore *FromLC(RReadStream &aHost);
Open the store hosted by the specified stream, putting a pointer to the store onto the cleanup stack.
Putting a pointer to the embedded store object onto the cleanup stack allows the object and allocated resources to be cleaned up if a subsequent leave occurs.
Note that ownership of the stream passes to the store and the referenced RReadStream
is cleared.
|
|
static inline TStreamPos Position(TStreamId anId);
Returns the position of the specified stream, within the host stream.
|
|
IMPORT_C void Detach();
Gives up ownership of the host stream buffer. The caller takes on the responsibility for discarding the buffer.
inline void Reattach(MStreamBuf *aHost);
Takes ownership of the specified stream buffer. On return from this function, the embedded store assumes this to be the host stream buffer.
|
inline MStreamBuf *Host() const;
Returns a pointer to the stream buffer which is acting as host to this embedded store. The embedded store retains ownership of the host stream buffer.
|
inline TStreamPos Start() const;
Returns the start position of this embedded store within the host stream.
|
protected: virtual IMPORT_C MStreamBuf *DoReadL(TStreamId anId) const;
Opens the requested stream for reading. The function should return a stream buffer positioned at the beginning of this stream.
This function is called by the OpenL() and OpenLC() member functions of RStoreReadStream
.
|
|
protected: virtual IMPORT_C MStreamBuf *DoCreateL(TStreamId &anId);
Creates a new stream in the store. The function gets the allocated stream id in the anId parameter. A stream buffer for the
stream should be returned, ready to write into the new stream. This provides the implementation for the RStoreWriteStream::CreateL()
functions.
|
|
private: virtual IMPORT_C void DoSetRootL(TStreamId anId);
Implements the setting of theroot stream.
This function is called by SetRootL()
|
private: virtual IMPORT_C void DoCommitL();
Commits any changes to the store. For a store that provides atomic updates, this writes all of the pending updates to the to the permanent storage medium. After committing the store contains all or none of the updates since the last commit/revert.
This function provides the implementation for the public CommitL()
function.