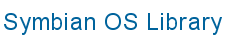
![]() |
![]() |
|
Location:
S32SHARE.H
Link against: estor.lib
class RShareBuf : public MStreamBuf;
A shared stream buffer.
This class is part of the framework used by CFileStore
that allows multiple stream buffers to concurrently access the single hosting file buffer, an RFileBuf
object.
Each shared stream buffer maintains its own read and write positions.
Read and write operations to RShareBuf are directed through separate TStreamMark
objects, one for read and one for write. The TStreamMark
objects re-direct read/write operations through a TStreamExchange
object to the RFileBuf
object, which handles the actual file read and write operations.
This stream buffer can also be accessed through RShareReadStream
and RShareWriteStream
objects.
MStreamBuf
- A stream buffer that provides a generic I/O interface for streamed data
RShareBuf
- A shared stream buffer
Defined in RShareBuf
:
DoReadL()
, DoReadL()
, DoReadL()
, DoRelease()
, DoSeekL()
, DoWriteL()
, DoWriteL()
, DoWriteL()
, Open()
, Open()
, RShareBuf()
Inherited from MStreamBuf
:
Close()
,
DoSynchL()
,
ERead
,
EWrite
,
PushL()
,
Read()
,
ReadL()
,
Release()
,
SeekL()
,
SizeL()
,
Synch()
,
SynchL()
,
TMark
,
TRead
,
TWrite
,
TellL()
,
Write()
,
WriteL()
IMPORT_C RShareBuf();
Constructs an empty shared stream buffer object.
Call one of the Open()
functions to prepare the stream.
IMPORT_C void Open(TStreamExchange &aHost, TStreamPos aPos, TInt aMode=ERead|EWrite);
Prepares the shared stream buffer for streaming.
The function sets the read mark and/or the write mark to the specified position within the host stream.
|
inline void Open(TStreamExchange &aHost, TInt aMode=ERead|EWrite);
Prepares the shared stream buffer for streaming.
The function sets the read mark and/or the write mark to the beginning of the host stream.
|
protected: virtual IMPORT_C void DoRelease();
Frees resources before abandoning the stream buffer.
It is called by Release()
.
This implementation is empty, but classes derived from MStreamBuf
can provide their own implementation, if necessary.
protected: virtual IMPORT_C TInt DoReadL(TAny *aPtr, TInt aMaxLength);
|
|
protected: virtual IMPORT_C TInt DoReadL(TDes8 &aDes, TInt aMaxLength, TRequestStatus &aStatus);
Reads data from the stream buffer into the specified descriptor.
This function is called by ReadL(TDes8&,TInt,TRequestStatus&)
.
This implementation deals with the request synchronously, and completes the request with KErrNone. Other implementations may choose to deal with this in a true asynchronous manner.
In addition, the read operation itself uses the DoReadL(TAny*,TInt)
variant.
|
|
protected: virtual IMPORT_C TStreamTransfer DoReadL(MStreamInput &anInput, TStreamTransfer aTransfer);
Reads data from the stream into the specified data sink.
It is called by ReadL(MStreamInput&,TStreamTransfer)
.
This implementation calls the sink's ReadFromL() function, which performs the read (transfer) operation.
This implementation of DoReadL()
is called for streams that do not have buffering capabilities, and that are derived directly from this class.
|
|
protected: virtual IMPORT_C void DoWriteL(const TAny *aPtr, TInt aLength);
|
protected: virtual IMPORT_C TInt DoWriteL(const TDesC8 &aDes, TInt aMaxLength, TRequestStatus &aStatus);
Writes data from the specified descriptor into this stream buffer.
This function is called by WriteL(const TDesC8&,TInt,TRequestStatus&)
.
This implementation deals with the request synchronously, and completes the request with KErrNone. Other implementations may choose to deal with this in a true asynchronous manner.
In addition, the write operation itself uses the DoWriteL(TAny*,TInt) variant.
|
|
protected: virtual IMPORT_C TStreamTransfer DoWriteL(MStreamOutput &anOutput, TStreamTransfer aTransfer);
|
|
protected: virtual IMPORT_C TStreamPos DoSeekL(TMark aMark, TStreamLocation aLocation, TInt anOffset);
|
|