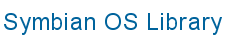
![]() |
![]() |
|
Location:
S32FILE.H
Link against: estor.lib
class RFileBuf : public TStreamBuf;
A stream buffer hosted by a file.
Instances of this class are used by file based persistent stores, i.e. CFileStore
type objects. An RFileBuf object is associated with a file and the file is said to be attached to the stream buffer.
An RFileBuf object is also used by RFileReadStream
and RFileWriteStream
objects to provide buffered file I/O.
The stream buffer has intermediate buffering capabilities.
When used as the basis for a file store, it hosts multiple streams through the TStreamExchange
and RShareBuf
classes
MStreamBuf
- A stream buffer that provides a generic I/O interface for streamed data
TStreamBuf
- Adds buffering capabilities to a stream buffer
RFileBuf
- A stream buffer hosted by a file
Defined in RFileBuf
:
Attach()
, Close()
, Create()
, Detach()
, DoReadL()
, DoReadL()
, DoRelease()
, DoSeekL()
, DoSynchL()
, DoWriteL()
, DoWriteL()
, File()
, Open()
, OverflowL()
, RFileBuf()
, RFileBuf()
, Reattach()
, Replace()
, Reset()
, Reset()
, SetBuf()
, SetBuf()
, SetBuf()
, SetSizeL()
, Temp()
, UnderflowL()
Inherited from MStreamBuf
:
ERead
,
EWrite
,
PushL()
,
Read()
,
ReadL()
,
Release()
,
SeekL()
,
SizeL()
,
Synch()
,
SynchL()
,
TMark
,
TRead
,
TWrite
,
TellL()
,
Write()
,
WriteL()
Inherited from TStreamBuf
:
Avail()
,
End()
,
Ptr()
,
SetEnd()
,
SetPtr()
,
TArea
IMPORT_C RFileBuf();
Constructs the object with a default intermediate buffer size.
The size of the intermediate buffer is the value of the constant KDefaultFileBufSize.
IMPORT_C RFileBuf(TInt aSize);
Constructs the object with the specified intermediate buffer size.
If the intermediate buffer size is zero, then the class provides an MStreamBuf
interface to unbuffered file I/O.
|
IMPORT_C void Reset();
Frees the intermediate buffer.
If there is any read data in the intermediate buffer, then the function reverts the read position within the stream.
The intermediate buffer must not contain any outstanding write data, otherwise the function raises a STORE-File 6 panic.
inline void Reset(TInt aSize);
Frees the intermediate buffer and changes the size of any future intermediate buffer to the specified value.
The intermediate buffer must not contain any outstanding write data, otherwise the function raises a STORE-File 6 panic.
|
IMPORT_C TInt Open(RFs &aFs, const TDesC &aName, TUint aFileMode);
Opens the specified file and attaches it to this stream buffer.
If the file cannot be opened, then it is not attached to this stream buffer.
|
|
IMPORT_C TInt Create(RFs &aFs, const TDesC &aName, TUint aFileMode);
Creates a file with the specified name and attaches it to this stream buffer.
The file must not already exist.
If the file cannot be created and opened, then it is not attached to this stream buffer.
|
|
IMPORT_C TInt Replace(RFs &aFs, const TDesC &aName, TUint aFileMode);
Replaces the file with the specified name and attaches it to this stream buffer.
If there is an existing file with the same name, then this function overwrites it. If the file does not already exist, it is created.
If the file cannot be replaced, then it is not attached to this stream buffer.
|
|
IMPORT_C TInt Temp(RFs &aFs, const TDesC &aPath, TFileName &aName, TUint aFileMode);
Creates and opens a temporary file with a unique name and attaches it to this stream buffer.
|
|
IMPORT_C void Attach(RFile &aFile, TInt aPos=0);
Attaches the specified file to this stream buffer.
The function also re-sets the intermediate buffer's read and write marks to the beginning of the intermediate buffer and sets the read and write stream positions to the specified offset within the file.
|
IMPORT_C void Close();
Writes any outstanding data from the intermediate buffer before freeing the intermediate buffer and closing the attached file.
inline void Detach();
Detaches the file from this stream buffer.
The intermediate buffer's read and write marks are not changed, and the stream positions are not changed. This means that the contents of the file should not change while it is detached.
inline void Reattach(RFile &aFile);
Re-attaches the specified file to this stream buffer.
The intermediate buffer's read and write marks are not changed, and the stream positions are not changed.
The file should be the one that was detached using the Detach()
function.
|
IMPORT_C void SetSizeL(TInt aSize);
Changes the size of the file attached to this buffer to the specified value.
Writes any outstanding data from the intermediate buffer to the stream hosted by the file. Any data in the intermediate buffer that would lie beyond the end of the truncated file, is not written.
|
inline RFile &File() const;
Gets a reference to the file attached to this stream buffer.
|
protected: virtual IMPORT_C TInt UnderflowL(TInt aMaxLength);
Re-fills the intermediate buffer and resets the start and end points of the read area.
The implementation of this function depends on the way the stream itself is implemented. For example, the in-memory streams have simple implementations.
|
|
protected: virtual IMPORT_C void OverflowL();
Empties the intermediate buffer and resets the start and end points of the write area.
The implementation of this function depends on the way the stream itself is implemented. For example, the in-memory streams have simple implementations.
protected: virtual IMPORT_C void DoRelease();
Frees resources before abandoning the stream buffer.
It is called by Release()
.
This implementation is empty, but classes derived from MStreamBuf
can provide their own implementation, if necessary.
protected: virtual IMPORT_C void DoSynchL();
Synchronises the stream buffer with the stream, leaving if any error occurs.
In effect, this ensures that buffered data is delivered to the stream.
It is called by SynchL()
.
This implementation is empty, but classes derived from MStreamBuf
can provide their own implementation, if necessary.
protected: virtual IMPORT_C TInt DoReadL(TAny *aPtr, TInt aMaxLength);
Reads data from the intermediate buffer into the specified memory location.
The function calls the virtual function UnderfLowL() to give concrete implementations the chance to refill the intermediate buffer, and satisfy the caller's requirements.
This implementation overrides the one supplied by the base class MStreamBuf
, and is called by, MStreamBuf::ReadL(TAny*,TInt)
.
|
|
protected: virtual IMPORT_C TInt DoReadL(TDes8 &aDes, TInt aMaxLength, TRequestStatus &aStatus);
Reads data from the stream buffer into the specified descriptor.
This function is called by ReadL(TDes8&,TInt,TRequestStatus&)
.
This implementation deals with the request synchronously, and completes the request with KErrNone. Other implementations may choose to deal with this in a true asynchronous manner.
In addition, the read operation itself uses the DoReadL(TAny*,TInt)
variant.
|
|
protected: virtual IMPORT_C void DoWriteL(const TAny *aPtr, TInt aLength);
Writes data from the specified memory location into the intermediate buffer.
The function calls the virtual function OverfLowL() to give concrete implementations the chance to forward the intermediate buffer content to its destination.
This implementation overrides the one supplied by the base class MStreamBuf
, and is called by MStreamBuf::WriteL(const TAny*,TInt)
.
|
protected: virtual IMPORT_C TInt DoWriteL(const TDesC8 &aDes, TInt aMaxLength, TRequestStatus &aStatus);
Writes data from the specified descriptor into this stream buffer.
This function is called by WriteL(const TDesC8&,TInt,TRequestStatus&)
.
This implementation deals with the request synchronously, and completes the request with KErrNone. Other implementations may choose to deal with this in a true asynchronous manner.
In addition, the write operation itself uses the DoWriteL(TAny*,TInt) variant.
|
|
protected: virtual IMPORT_C TStreamPos DoSeekL(TMark aMark, TStreamLocation aLocation, TInt anOffset);
|
|
protected: inline void SetBuf(TRead, TUint8 *aPtr, TUint8 *anEnd);
Sets the start and end points of the read area within the intermediate buffer.
A start point is always within an area; an end point is always the first byte beyond the end of an area.
|
protected: inline void SetBuf(TWrite, TUint8 *aPtr, TUint8 *anEnd);
Sets the start and end points of the write area within the intermediate buffer.
A start point is always within an area; an end point is always the first byte beyond the end of an area.
|
protected: inline void SetBuf(TArea anArea, TUint8 *aPtr, TUint8 *anEnd);
Sets the start and end points of the read and/or the write area within the intermediate buffer.
A start point is always within an area; an end point is always the first byte beyond the end of an area.
|