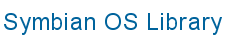
![]() |
![]() |
|
Location:
CDTDModel.h
Link against: dtdmdl.lib
class CDTDModel : public CBNFParser;
Creates a tree that represents a specified DTD.
To use it:
1. call the base class function ProcessDataL()
with the filename of the DTD, and call CommitL()
2. call GeneratedTree()
to get the tree
MDataProviderObserver
- MDataProviderObserver provides a virtual interface for any class to observe any MDataProvider, and provide data receiving
services
CBase
- Base class for all classes to be instantiated on the heap
CBNFParser
- Base class for parsers that use a BNF tree to parse an input stream
CDTDModel
- Creates a tree that represents a specified DTD
Defined in CDTDModel
:
BuildWml11DTDL()
, CDTDElementAttribute
, CDTDModel()
, COwnerRuleMarkedStack
, EConditionalMark
, EGroupMark
, ERuleStartMark
, EndConditional()
, GeneratedTree()
, NewL()
, ResetL()
, StartConditional()
, TRuleMarks
, TreeL()
, iCharRefCheck
, iGEContent
, iInPEDecl
, iNewAttfieldName
, iNewElementAttribute
, iNewRule
, iNewRuleStack
, iNewTree
, ~CDTDModel()
Inherited from CBNFParser
:
AddComponentAttributeL()
,
AddRuleCallbackL()
,
AndL()
,
AttributeLUT()
,
CRuleStack
,
CommitL()
,
CurrentRule()
,
DeleteMark()
,
ExactL()
,
ExecutePostRuleCallbackL()
,
ExecutePreRuleCallbackL()
,
MDataProviderObserverReserved1()
,
MDataProviderObserverReserved2()
,
Mark()
,
MarkCallback()
,
MarkedL()
,
MarkedWithInitialTextL()
,
NMoreL()
,
NewBNFL()
,
NewComponentL()
,
NewRuleL()
,
OptionalL()
,
OptionalMatched()
,
OrL()
,
ParseL()
,
PerformRuleL()
,
ProcessDataL()
,
RangeL()
,
ReferenceL()
,
RuleMatched()
,
SelectL()
,
SetBaseUriL()
,
SetDataExpected()
,
SetDocumentTypeL()
,
SetState()
,
SetStatus()
,
State()
,
StringL()
,
TRuleCallback
,
Valid()
,
WithoutL()
,
iCurrentRule
,
iLUT
,
iMatched
,
iMoreCount
,
iMoreMaximum
,
iMoreMinimum
,
iOptionalMatched
,
iParsing
,
iPostRuleCallback
,
iPreRuleCallback
,
iRangeEnd
,
iRangeStart
,
iReferenceString
,
iRuleStack
,
iString
,
iStringComplete
,
iSubRule
,
iSubRuleMatched
,
iTree
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
static IMPORT_C CDTDModel *NewL(CAttributeLookupTable &aLUT);
Allocates and constructs a new BNF parser.
|
|
protected: IMPORT_C CDTDModel(CAttributeLookupTable &aLUT);
|
virtual IMPORT_C void ResetL();
Reset the parser to a state where it can accept and parse new input.
If no BNF tree yet exists the virtual method TreeL()
is called to obtain the BNF tree for this parser. Any existing state of parsing and input data is destroyed.
IMPORT_C CBNFNode *GeneratedTree();
Gets the root node of the tree generated to process the DTD.
It transfers ownership of the tree to the caller.
|
IMPORT_C void BuildWml11DTDL(CBNFNode &aPackageRootNode);
Builds a parser tree for the WML1.1 DTD.
|
protected: virtual IMPORT_C CBNFNode *TreeL();
Creates a BNF rule tree to parse the input stream.
This overrides CBNFParser::TreeL()
.
|
protected: virtual IMPORT_C void StartConditional(TParserNodeTypes aRuleType);
|
protected: virtual IMPORT_C void EndConditional(TParserNodeTypes aRuleType, TBool aSuccess);
|
class CDTDElementAttribute : public CBase;
Represents an attribute of a DTD element.
CBase
- Base class for all classes to be instantiated on the heap
CDTDModel::CDTDElementAttribute
- Represents an attribute of a DTD element
Defined in CDTDModel::CDTDElementAttribute
:
EDefault
, EFixed
, EImplied
, EReference
, ERequired
, KValueType
, iType
, iValue
, iValueType
, ~CDTDElementAttribute()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
~CDTDElementAttribute()
inline virtual ~CDTDElementAttribute();
Destructor.
KValueType
KValueType
DTD element attribute value types.
|
iType
CBNFNode * iType;
Node that specifies the attribute type.
iValue
CBNFNode * iValue;
Node that specifies the attribute value.
iValueType
KValueType iValueType;
Attribute value type.
protected: COwnerRuleMarkedStack iNewRuleStack;