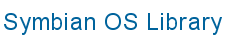
![]() |
![]() |
|
Location:
parserfeature.h
namespace Xml
Defined in Xml
:
CMatchData
, CParser
, EAttributeType_CDATA
, EAttributeType_ENTITIES
, EAttributeType_ENTITY
, EAttributeType_ID
, EAttributeType_IDREF
, EAttributeType_IDREFS
, EAttributeType_NMTOKEN
, EAttributeType_NMTOKENS
, EAttributeType_NONE
, EAttributeType_NOTATION
, EConvertTagsToLowerCase
, EErrorOnUnrecognisedTags
, ELastFeature
, ERawContent
, EReplaceIntEntityFromExtSubsetByRef
, EReportNamespaceMapping
, EReportNamespacePrefixes
, EReportNamespaces
, EReportUnrecognisedTags
, ESendFullContentInOneChunk
, EXmlVersion_1_1
, KErrXmlBadCharacterConversion
, KErrXmlBadIndex
, KErrXmlDocumentCorrupt
, KErrXmlFirst
, KErrXmlGeneratorPluginNotFound
, KErrXmlLast
, KErrXmlMissingStringDictionary
, KErrXmlMoreThanOneParserMatched
, KErrXmlParserPluginNotFound
, KErrXmlPluginNotFound
, KErrXmlStringDictionaryPluginNotFound
, KErrXmlStringPoolTableNotFound
, KErrXmlUnavailableCharacterSet
, KErrXmlUnsupportedAttribute
, KErrXmlUnsupportedAttributeValue
, KErrXmlUnsupportedCharacterSet
, KErrXmlUnsupportedDocumentVersion
, KErrXmlUnsupportedElement
, KErrXmlUnsupportedExtInterface
, KXmlSymbianPluginVariant
, MContentHandler
, MWbxmlExtensionHandler
, ParseL()
, ParseL()
, ParseL()
, RAttribute
, RAttributeArray
, RDocumentParameters
, RStringDictionaryCollection
, RTagInfo
, TAttributeType
, TParserFeature
IMPORT_C void ParseL(CParser &aParser, const TDesC8 &aDocument);
|
IMPORT_C void ParseL(CParser &aParser, RFs &aFs, const TDesC &aFilename);
|
IMPORT_C void ParseL(CParser &aParser, RFile &aFile);
|
class RDocumentParameters;
The RDocumentParameters
class contains information about the document to be passed to the client.
Defined in Xml::RDocumentParameters
:
CharacterSetName()
CharacterSetName()
IMPORT_C const RString &CharacterSetName() const;
The objects member have been pre-set accordingly.
This method returns a handle to the character encoding name, which is the Internet-standard name of a character set, which is identified in Symbian OS by its UID.
|
class RTagInfo;
The RTagInfo
class holds information that describes an element or attribute.
Defined in Xml::RTagInfo
:
LocalName()
, Prefix()
, Uri()
Uri()
IMPORT_C const RString &Uri() const;
This method obtains the uri member of this object.
|
LocalName()
IMPORT_C const RString &LocalName() const;
This method obtains the LocalName member of this object.
|
Prefix()
IMPORT_C const RString &Prefix() const;
This method obtains the Prefix member of this object.
|
class RStringDictionaryCollection;
The RStringDictionaryCollection
class holds a collection of Dictionaries requested by the user.
Defined in Xml::RStringDictionaryCollection
:
Close()
, CurrentDictionaryL()
, OpenDictionaryL()
, OpenL()
, RStringDictionaryCollection()
, StringPool()
RStringDictionaryCollection()
IMPORT_C RStringDictionaryCollection();
Default constructor
This object is properly constructed.
OpenL()
IMPORT_C void OpenL();
This method opens this resource incrementing the reference count. It must be the first method called after construction.
The object is ready to be used.
Close()
IMPORT_C void Close();
This method cleans up the object before destruction. It releases all resources in accordance to the R Class pattern.
This object may be allowed to go out of scope.
OpenDictionaryL()
IMPORT_C void OpenDictionaryL(const TDesC8 &aDictionaryDescription);
Connect has been called.
This method loads the Dictionary.
The Dictionary has been loaded.
|
StringPool()
IMPORT_C RStringPool &StringPool();
This method obtains a handle to the RStringPool
|
CurrentDictionaryL()
IMPORT_C MStringDictionary &CurrentDictionaryL() const;
This method obtains the current string dictionary in use. Also, serves as a way to test if any dictionaries have been loaded as none needs be.
|
|
class MWbxmlExtensionHandler;
The Wbxml api extension class. Inherited by classes wishing to cater for this interface, e.g. WbxmlParser.
Defined in Xml::MWbxmlExtensionHandler
:
EExtInterfaceUid
, OnExtensionL()
, TExtInterfaceUid
OnExtensionL()
virtual void OnExtensionL(const RString &aData, TInt aToken, TInt aErrorCode)=0;
This method is a receive notification of an extension instruction. The type of extension is specified in aToken and is based on the global tokens specified in WBXML documents.
|
TExtInterfaceUid
TExtInterfaceUid
The uid identifying this extension interface.
|
class MContentHandler;
This class defines the interface required by a client of the xml framework. It allows a client to be placed in a chain with
other clients, i.e. a Parser, a Validator, or a User
, and therefore allows the flow of information between these links. It provides callbacks analogous to that of the SAX 2.0
interface.
Defined in Xml::MContentHandler
:
GetExtendedInterface()
, OnContentL()
, OnEndDocumentL()
, OnEndElementL()
, OnEndPrefixMappingL()
, OnError()
, OnIgnorableWhiteSpaceL()
, OnProcessingInstructionL()
, OnSkippedEntityL()
, OnStartDocumentL()
, OnStartElementL()
, OnStartPrefixMappingL()
OnStartDocumentL()
virtual void OnStartDocumentL(const RDocumentParameters &aDocParam, TInt aErrorCode)=0;
This method is a callback to indicate the start of the document.
|
OnEndDocumentL()
virtual void OnEndDocumentL(TInt aErrorCode)=0;
This method is a callback to indicate the end of the document.
|
OnStartElementL()
virtual void OnStartElementL(const RTagInfo &aElement, const RAttributeArray &aAttributes, TInt aErrorCode)=0;
This method is a callback to indicate an element has been parsed.
|
OnEndElementL()
virtual void OnEndElementL(const RTagInfo &aElement, TInt aErrorCode)=0;
This method is a callback to indicate the end of the element has been reached.
|
OnContentL()
virtual void OnContentL(const TDesC8 &aBytes, TInt aErrorCode)=0;
This method is a callback that sends the content of the element. Not all the content may be returned in one go. The data may be sent in chunks. When an OnEndElementL is received this means there is no more content to be sent.
|
OnStartPrefixMappingL()
virtual void OnStartPrefixMappingL(const RString &aPrefix, const RString &aUri, TInt aErrorCode)=0;
This method is a notification of the beginning of the scope of a prefix-URI Namespace mapping. This method is always called before the corresponding OnStartElementL method.
|
OnEndPrefixMappingL()
virtual void OnEndPrefixMappingL(const RString &aPrefix, TInt aErrorCode)=0;
This method is a notification of the end of the scope of a prefix-URI mapping. This method is called after the corresponding DoEndElementL method.
|
OnIgnorableWhiteSpaceL()
virtual void OnIgnorableWhiteSpaceL(const TDesC8 &aBytes, TInt aErrorCode)=0;
This method is a notification of ignorable whitespace in element content.
|
OnSkippedEntityL()
virtual void OnSkippedEntityL(const RString &aName, TInt aErrorCode)=0;
This method is a notification of a skipped entity. If the parser encounters an external entity it does not need to expand it - it can return the entity as aName for the client to deal with.
|
OnProcessingInstructionL()
virtual void OnProcessingInstructionL(const TDesC8 &aTarget, const TDesC8 &aData, TInt aErrorCode)=0;
This method is a receive notification of a processing instruction.
|
OnError()
virtual void OnError(TInt aErrorCode)=0;
This method indicates an error has occurred.
|
GetExtendedInterface()
virtual TAny *GetExtendedInterface(const TInt32 aUid)=0;
This method obtains the interface matching the specified uid.
|
|
class CParser : public CBase;
If you need to parse xml this is the class you need to use.
CBase
- Base class for all classes to be instantiated on the heap
Xml::CParser
- If you need to parse xml this is the class you need to use
Defined in Xml::CParser
:
AddPreloadedDictionaryL()
, DisableFeature()
, EnableFeature()
, IsFeatureEnabled()
, NewL()
, NewL()
, NewLC()
, NewLC()
, ParseBeginL()
, ParseBeginL()
, ParseBeginL()
, ParseEndL()
, ParseL()
, SetProcessorChainL()
, StringDictionaryCollection()
, StringPool()
, ~CParser()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
NewL()
static IMPORT_C CParser *NewL(const TDesC8 &aParserMimeType, MContentHandler &aCallback);
This method creates a parser that is ready to parse documents of the specified mime type.
If there are multiple parser plugins in the system which can parse the mime type, the XML framework will choose a parser.
The criteria used to choose a parser, from many matching parsers, is as follows:
A Symbian-supplied parser (with variant field set to "Symbian") will be selected by default. If there are multiple Symbian-supplied parsers, the one with the lowest Uid will be selected. Otherwise, the non-Symbian parser with the lowest Uid will be selected.
|
|
|
NewLC()
static IMPORT_C CParser *NewLC(const TDesC8 &aParserMimeType, MContentHandler &aCallback);
This method is similar to NewL, but leaves the created parser on the cleanup stack.
|
|
|
NewL()
static IMPORT_C CParser *NewL(const CMatchData &aCriteria, MContentHandler &aCallback);
This method creates the particular parser specified in CMatchData
parameter.
The parser plugin resolution process is based on mime type and variant field. Both are provided in CMatchData
parameter. Mime Type is a mandatory string for the resolution process and it is matched against the data field of plugin
resource files. Variant string is optional. If it exists, it is matched against the first entry of the opaque data field of
plugin resource files.
If the query is narrowed down to many parsers, the XML framework might either leave with an error (KErrXmlMoreThanOneParserMatched), or choose a parser. The behaviour is specified by LeaveOnMany flag. The default value of the flag is FALSE ('choose a parser' behaviour).
The criteria used to choose a parser, from many matching parsers, is as follows:
If the optional Variant field is specified, the XML framework will choose the parser with the lowest Uid from the list.
If the optional Variant field is not specified, a Symbian-supplied parser (with variant field set to "Symbian") will be selected by default. If there are multiple Symbian-supplied parsers, the one with the lowest Uid will be selected. Otherwise, the non-Symbian parser with the lowest Uid will be selected.
Case sensitivity of the string matching process is applied according to the relevant flag in CMatchData
. The default value is TRUE (Case Sensitivity enabled).
Only ROM-based parsers are returned if the relevant flag is set in CMatchData
. The default value is FALSE (all parsers are considered).
|
|
|
NewLC()
static IMPORT_C CParser *NewLC(const CMatchData &aCriteria, MContentHandler &aCallback);
This method creates the particular parser specified in CMatchData
parameter. It performs the same way as NewL with the exception that it leaves the object on the cleanup stack.
|
|
|
~CParser()
virtual ~CParser();
This method is the destructor for the object.
ParseBeginL()
IMPORT_C void ParseBeginL();
This method tells the parser that we're going to start parsing a document using the default mime type specified on construction.
The processor chain and features will be cleared if the parser currently set is not the default, all old features are removed as these generally have no meaning between parsers.
|
ParseBeginL()
IMPORT_C void ParseBeginL(const TDesC8 &aDocumentMimeType);
This method tells the parser that we're going to start parsing a document using the parser associated with this mime type.
|
ParseBeginL()
IMPORT_C void ParseBeginL(const CMatchData &aCriteria);
This method tells the parser that we're going to start parsing a document using the parser associated with given CMatchData
criteria.
|
|
ParseL()
IMPORT_C void ParseL(const TDesC8 &aFragment);
This method tells the parser to parse a fragment of a document. Could be the whole document. ParseEndL should be called once the whole document has been parsed.
The parser currently set will be used.
|
|
ParseEndL()
IMPORT_C void ParseEndL();
This method tells the parser that we've finished parsing the current document and should be called after calling CParser::ParseL
for the final time, as this will initiate error callbacks via MContentHandler
, and clean up memory where appropriate, should an error have occured during the parsing process. Such an error could occur
when trying to parse a truncated document.
|
SetProcessorChainL()
IMPORT_C void SetProcessorChainL(const RContentProcessorUids &aPlugins);
This method changes the client and plugin chain.
|
|
EnableFeature()
IMPORT_C TInt EnableFeature(TInt aParserFeature);
This method enables a specific feature of the parser.
|
|
DisableFeature()
IMPORT_C TInt DisableFeature(TInt aParserFeature);
This method disables a specific feature of the parser.
|
|
IsFeatureEnabled()
IMPORT_C TBool IsFeatureEnabled(TInt aParserFeature) const;
This method tell whether a specific feature of the parser is enabled.
|
|
AddPreloadedDictionaryL()
IMPORT_C void AddPreloadedDictionaryL(const TDesC8 &aPublicId);
This method preloads a string dictionary prior to parsing.
|
|
StringPool()
IMPORT_C RStringPool &StringPool();
This method obtains a handle to the current string pool.
|
StringDictionaryCollection()
IMPORT_C RStringDictionaryCollection &StringDictionaryCollection();
This method obtains a handle to the current StringDictionaryCollection.
|
class CMatchData : public CBase;
CMatchData
class provides detailed parser information for XML framework. Client application should use this class to precisely specify
the plugin, which should be used as a parsing engine in XML framework.
CBase
- Base class for all classes to be instantiated on the heap
Xml::CMatchData
-
Defined in Xml::CMatchData
:
MimeType()
, NewL()
, NewLC()
, SetCaseSensitivity()
, SetLeaveOnMany()
, SetMimeTypeL()
, SetRomOnly()
, SetVariantL()
, Variant()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
NewL()
static IMPORT_C CMatchData *NewL();
Creates CMatchData
object with its default values.
|
|
NewLC()
static IMPORT_C CMatchData *NewLC();
Creates CMatchData
object with its default values. Leaves an obeject pointer on a cleanup stack.
|
|
SetMimeTypeL()
IMPORT_C void SetMimeTypeL(const TDesC8 &aData);
Sets up a mime type attribute to a string given as an aData descriptor.
|
|
MimeType()
IMPORT_C const TPtrC8 MimeType() const;
Returns a pointer to the mime type string.
|
SetVariantL()
IMPORT_C void SetVariantL(const TDesC8 &aVariant);
Sets up a variant attribute to a string given as an aVariant descriptor. If this is not set OR is set to a string of length 0 the Variant ID is not used during parser resolution and hence the variant IDs of plug-in parsers are ignored.
|
|
Variant()
IMPORT_C const TPtrC8 Variant() const;
Returns a pointer to the variant string.
|
SetLeaveOnMany()
IMPORT_C void SetLeaveOnMany(TBool aSetting);
Sets the LeaveOnManyFlag flag. If set, it notifies customized resolver it should leave when the query is resolved to more than one parser. By default this flag is not set, so the framework chooses a parser in this case.
|
SetRomOnly()
IMPORT_C void SetRomOnly(TBool aSetting);
Sets the Rom Only flag. If set, it notifies customized resolver the request is for ROM-based parsers only. By default this flag is not set, so the framework searches for rom and non-rom based parsers.
|
SetCaseSensitivity()
IMPORT_C void SetCaseSensitivity(TBool aSetting);
Sets the Case Sensitivity flag. Customized resolver uses this setting to turn on or off case sensitivity for strings matching.
|
class RAttribute;
The RAttribute
class holds an attribute's description belonging to an element.
Defined in Xml::RAttribute
:
Attribute()
, Type()
, Value()
Attribute()
IMPORT_C const RTagInfo &Attribute() const;
The objects members have been pre-set accordingly.
This method returns a handle to the attribute's name details.
|
Value()
IMPORT_C const RString &Value() const;
The objects members have been pre-set accordingly.
This method returns a handle to the attribute value. If the attribute value is a list of tokens (IDREFS, ENTITIES or NMTOKENS),
the tokens will be concatenated into a single RString
with each token separated by a single space.
|
Type()
IMPORT_C TAttributeType Type() const;
The objects members have been pre-set accordingly.
This method returns the attribute's type.
|
TAttributeType
Specifies the type of this attribute object. These values are defined in the xml specification. For other specifications they may also be used or 'none'.
|
TParserFeature
Features defined for XML Framework parser plug-ins.
Every parser has a set of optional features that it may implement. This enumeration is the set of all features known to the framework and its plug-ins. Each feature is assigned a flag bit from the 32 possible.
|
typedef RArray<RAttribute> Xml::RAttributeArray;
Defines a list of attributes for an element.
const TLitC8< sizeof("Symbian") KXmlSymbianPluginVariant;
The plugin variant string of the default Symbian-provided XML parser plugins.
const TInt KErrXmlMoreThanOneParserMatched;
Indicates the parser query is matched to more than one parser. This error can be returned only if the request is to leave in such a case - LeaveOnMany flag is set.
const TInt KErrXmlLast;