Debugger
The Unity Debugger lets you inspect your code at runtime. For example, it can help you determine when a function is called and with which values. Furthermore, it allows you to look at the value of scripts variables at a given time while running your game. You can locate bugs or logic problems in your scripts by executing them step by step.
Unity uses the MonoDevelop IDE to debug the scripts in your game. You can debug all the languages supported by the engine (JavaScript, C# and Boo).
Note that the debugger has to load all your code and all symbols, so bear in mind that this can have a small impact on the performance of your game during execution. Typically this overhead is not large enough to affect the game framerate.
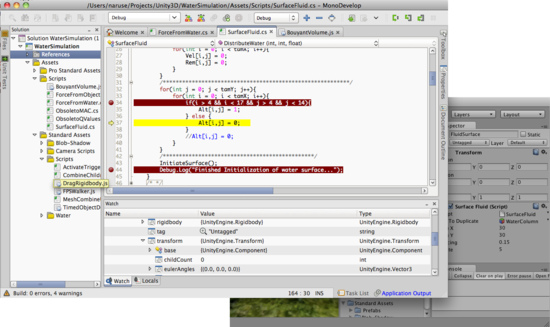
MonoDevelop window debugging a script in unity.
Debugging in Unity.
On Windows, users must choose to install MonoDevelop as part of the Unity installation (selected by default).
- For debugging entire Unity editor sessions, ensure that the correct Unity executable is selected in the Unity debugger preferences:
- Open the MonoDevelop preferences, browse to the Unity/Debugger section, and then browse to where your Unity app or executable is located.
- If you haven't used MonoDevelop with your project before, synchronize your MonoDevelop project. This will open your project inside MonoDevelop.
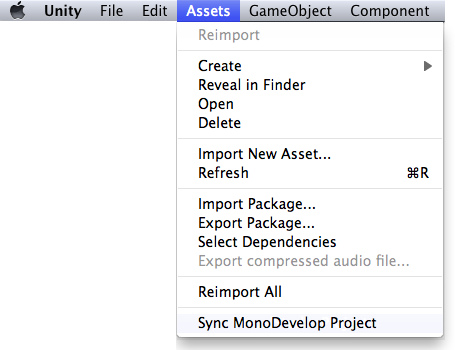
- Set the necessary breakpoints on your scripts by clicking the lines that you want to analyze.
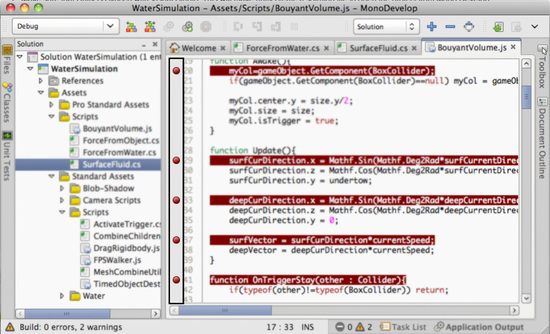
Debugging an entire Unity editing session
- Close Unity
- In MonoDevelop, click the Debug button in the toolbar, or choose Debug from the Run menu. This will launch Unity.
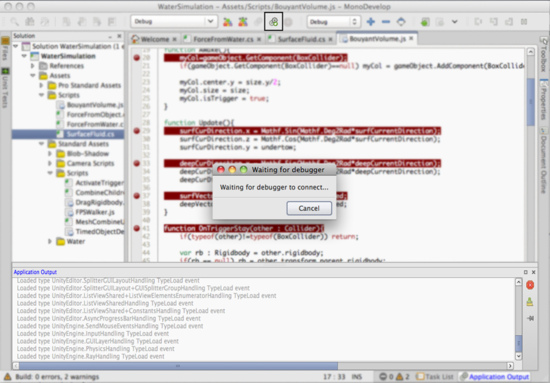
- When you enter play mode, your script code will execute in the debugger.
- When a breakpoint occurs, script execution will stop, and you will be able to use MonoDevelop to step over, into, and out of your script methods, inspect your variables, examine the call stack, etc.
- Note: When you're done debugging a toplevel method (e.g.
Update()
), or you just want to jump to the next breakpoint, you will experience better debugger performance by using the Continue command instead of stepping out or over the end of your function.
- Note: When you're done debugging a toplevel method (e.g.
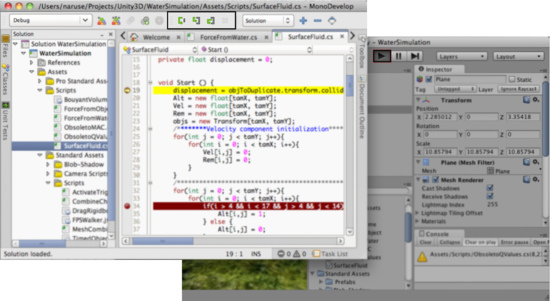
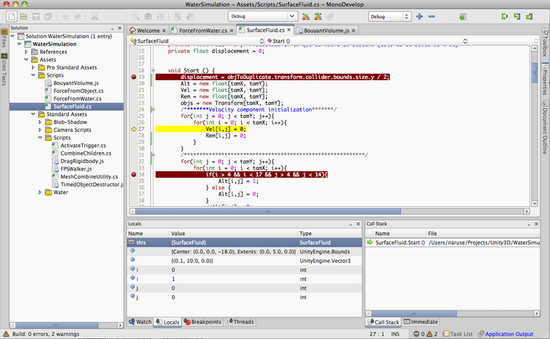
- To end your debugging session, quit Unity as normal.
Attaching the script debugger to an existing session
Starting with Unity 3.2, it is possible to attach the script debugger to a running Unity editor or to a player built with the script debugging option enabled.
- Launch Unity or your player.
- Ensure you have "Allow attached debugging" checked in the Preferences window.
- Open your project in MonoDevelop.
- In MonoDevelop, click the Attach button in the toolbar, or choose Attach from the Run menu.
- From the dialog that appears, choose the item you wish to debug.
- Notes:
- Currently supported attaching targets: Unity editors, desktop standalone players, Android players
- If your player is set not to run in the background (the default), you may need to focus your player for a few seconds in order for it to appear in the list.
- Android players need to have networking enabled when script debugging is enabled. All players need to be on the same network subnet as the computer running MonoDevelop.
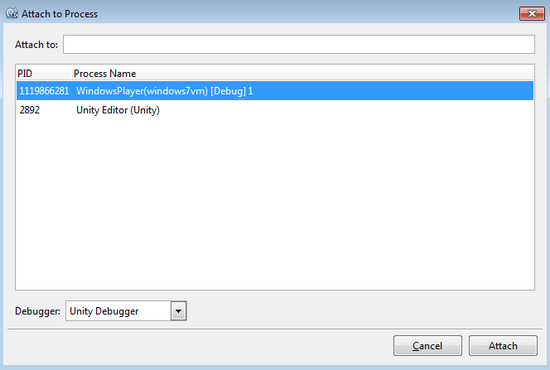
- Switch back to the editor or player you're debugging.
- Debug as normal.
- When you're done debugging, click the Detach button in the toolbar, or choose Detach from the Run menu.
- Note: If you choose Stop instead, the process you're debugging will be terminated.
Hints.
- If you add a watch to the this object, you can inspect the internal values (position, scale, rotation...) of the GameObject to which the script is attached.