iPcCamera Struct Reference
The representation of a camera. More...
#include <propclass/camera.h>
Inheritance diagram for iPcCamera:
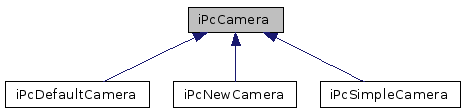
Public Member Functions | |
virtual void | DisableDistanceClipping ()=0 |
Disable distance clipping. | |
virtual void | Draw ()=0 |
Render. | |
virtual void | EnableAdaptiveDistanceClipping (float min_fps, float max_fps, float min_dist)=0 |
Enable adaptive distance clipping. | |
virtual void | EnableFixedDistanceClipping (float dist)=0 |
Enable fixed distance clipping. | |
virtual float | GetAdaptiveMaxFPS () const =0 |
Get the maximum fps used for adaptive distance clipping. | |
virtual float | GetAdaptiveMinDistance () const =0 |
Get the minimum distance used for adaptive distance clipping. | |
virtual float | GetAdaptiveMinFPS () const =0 |
Get the minimum fps used for adaptive distance clipping. | |
virtual iCamera * | GetCamera () const =0 |
Get the camera. | |
virtual bool | GetClearScreen () const =0 |
Get the clear screen flag. | |
virtual bool | GetClearZBuffer () const =0 |
Get the clear zbuffer flag. | |
virtual int | GetDrawFlags ()=0 |
Get camera drawing flags. | |
virtual float | GetFixedDistance () const =0 |
Get the fixed distance (returns < 0 if adaptive is used). | |
virtual iView * | GetView () const =0 |
Get the view. | |
virtual void | SetAutoDraw (bool auto_draw)=0 |
Set the auto-draw option. | |
virtual void | SetClearScreen (bool flag)=0 |
Set flag indicating if the camera should clear screen every frame. | |
virtual void | SetClearZBuffer (bool flag)=0 |
Set flag indicating if the camera should clear z-buffer every frame. | |
virtual void | SetRectangle (int x, int y, int w, int h)=0 |
Set the view rectangle to use on screen. | |
virtual bool | SetRegion (iPcRegion *region, bool point=true, const char *name=0)=0 |
Set Camera to a Region. | |
virtual bool | SetZoneManager (const char *entityname, bool point, const char *regionname, const char *name=0)=0 |
Set Camera to a zone manager. | |
virtual bool | SetZoneManager (iPcZoneManager *zonemgr, bool point, const char *regionname, const char *name=0)=0 |
Set Camera to a zone manager. | |
virtual void | UpdateCamera ()=0 |
Update camera position. | |
virtual bool | UseDistanceClipping () const =0 |
Returns true if we use distance clipping (either fixed or adaptive). | |
virtual bool | UseFixedDistanceClipping () const =0 |
Returns true if we use fixed distance clipping (as opposed to adaptive). |
Detailed Description
The representation of a camera.
Definition at line 36 of file camera.h.
Member Function Documentation
virtual void iPcCamera::DisableDistanceClipping | ( | ) | [pure virtual] |
Disable distance clipping.
virtual void iPcCamera::Draw | ( | ) | [pure virtual] |
Render.
This will clear the screen then draw on top of it.
Implemented in iPcNewCamera, and iPcSimpleCamera.
virtual void iPcCamera::EnableAdaptiveDistanceClipping | ( | float | min_fps, | |
float | max_fps, | |||
float | min_dist | |||
) | [pure virtual] |
Enable adaptive distance clipping.
In this mode the clipping plane will vary depending on the desired minimum and maximum fps.
- Parameters:
-
min_fps is the minimum fps that we would like to have. If we go beyond that then we will move the clipping plane closer. max_fps is the maximum fps that is ok. If we go beyond that we will move the clipping plane further away. min_dist is the minimum clipping plane distance. We will never move the clipping closer to the camera then this distance.
virtual void iPcCamera::EnableFixedDistanceClipping | ( | float | dist | ) | [pure virtual] |
Enable fixed distance clipping.
In this mode there is a fixed plane at the specified distance. All geometry that is fully behind that plane will not be rendered. This can speed up rendering.
- Parameters:
-
dist is the distance from the camera to the "far" clipping plane.
virtual float iPcCamera::GetAdaptiveMaxFPS | ( | ) | const [pure virtual] |
Get the maximum fps used for adaptive distance clipping.
virtual float iPcCamera::GetAdaptiveMinDistance | ( | ) | const [pure virtual] |
Get the minimum distance used for adaptive distance clipping.
virtual float iPcCamera::GetAdaptiveMinFPS | ( | ) | const [pure virtual] |
Get the minimum fps used for adaptive distance clipping.
virtual iCamera* iPcCamera::GetCamera | ( | ) | const [pure virtual] |
Get the camera.
virtual bool iPcCamera::GetClearScreen | ( | ) | const [pure virtual] |
Get the clear screen flag.
virtual bool iPcCamera::GetClearZBuffer | ( | ) | const [pure virtual] |
Get the clear zbuffer flag.
virtual int iPcCamera::GetDrawFlags | ( | ) | [pure virtual] |
Get camera drawing flags.
virtual float iPcCamera::GetFixedDistance | ( | ) | const [pure virtual] |
Get the fixed distance (returns < 0 if adaptive is used).
virtual iView* iPcCamera::GetView | ( | ) | const [pure virtual] |
Get the view.
virtual void iPcCamera::SetAutoDraw | ( | bool | auto_draw | ) | [pure virtual] |
Set the auto-draw option.
If this option is enabled, the camera will automatically render.
- Parameters:
-
auto_draw will cause the camera to automatically render every frame if set to true, or do nothing if set to false.
virtual void iPcCamera::SetClearScreen | ( | bool | flag | ) | [pure virtual] |
Set flag indicating if the camera should clear screen every frame.
Off by default unless the loaded map specifies this.
- Parameters:
-
flag if set to true, the camera will clear the screen every frame, otherwise it will not clear the screen.
virtual void iPcCamera::SetClearZBuffer | ( | bool | flag | ) | [pure virtual] |
Set flag indicating if the camera should clear z-buffer every frame.
Off by default unless the loaded map specifies this.
- Parameters:
-
flag if set to true, the camera will clear the z-buffer every frame, otherwise it will not clear the z-buffer.
virtual void iPcCamera::SetRectangle | ( | int | x, | |
int | y, | |||
int | w, | |||
int | h | |||
) | [pure virtual] |
Set the view rectangle to use on screen.
- Parameters:
-
x is the distance from the left side of the screen to the left side of the view. y is the distance from the top of the screen to the top of the view. w is the width of the view. h is the height of the view.
virtual bool iPcCamera::SetRegion | ( | iPcRegion * | region, | |
bool | point = true , |
|||
const char * | name = 0 | |||
) | [pure virtual] |
Set Camera to a Region.
- Parameters:
-
region is a pointer to the region to add the camera to. point if true, set the camera to a given start position. name is the name of the start position to move the camera to.
virtual bool iPcCamera::SetZoneManager | ( | const char * | entityname, | |
bool | point, | |||
const char * | regionname, | |||
const char * | name = 0 | |||
) | [pure virtual] |
Set Camera to a zone manager.
- Parameters:
-
entityname is a name of the entity with the zone manager. point if true, set the camera to a given start position. regionname is the name of the region to add the camera to. name is the name of the start position to move the camera to.
virtual bool iPcCamera::SetZoneManager | ( | iPcZoneManager * | zonemgr, | |
bool | point, | |||
const char * | regionname, | |||
const char * | name = 0 | |||
) | [pure virtual] |
Set Camera to a zone manager.
- Parameters:
-
zonemgr is a pointer to the zone manager to add the camera to. point if true, set the camera to a given start position. regionname is the name of the region to add the camera to. name is the name of the start position to move the camera to.
virtual void iPcCamera::UpdateCamera | ( | ) | [pure virtual] |
Update camera position.
This method will update camera position without rendering (you will have to call iView::Draw manual later).
virtual bool iPcCamera::UseDistanceClipping | ( | ) | const [pure virtual] |
Returns true if we use distance clipping (either fixed or adaptive).
virtual bool iPcCamera::UseFixedDistanceClipping | ( | ) | const [pure virtual] |
Returns true if we use fixed distance clipping (as opposed to adaptive).
The documentation for this struct was generated from the following file:
- propclass/camera.h
Generated for CEL: Crystal Entity Layer by doxygen 1.4.7