iPcNewCamera Struct Reference
This is a camera property class. More...
#include <propclass/newcamera.h>
Inheritance diagram for iPcNewCamera:
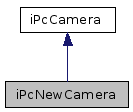
Public Types | |
enum | CEL_CAMERA_MODE |
Public Member Functions | |
virtual size_t | AttachCameraMode (CEL_CAMERA_MODE mode)=0 |
Attaches a built-in camera mode to this camera. | |
virtual size_t | AttachCameraMode (iCelCameraMode *mode)=0 |
Attaches a camera mode to this camera. | |
virtual bool | DetectCollisions () const =0 |
Returns whether the camera will use collision detection to avoid moving through walls. | |
virtual void | Draw ()=0 |
Render. | |
virtual const csVector3 & | GetBaseDir () const =0 |
Gets the base direction of the camera. | |
virtual const csVector3 & | GetBasePos () const =0 |
Gets the base position of the camera in world coordinates. | |
virtual const csReversibleTransform & | GetBaseTrans () const =0 |
Gets the base transform of the camera. | |
virtual const csVector3 & | GetBaseUp () const =0 |
Gets the base up vector of the camera. | |
virtual float | GetCollisionSpringCoefficient () const =0 |
Returns the spring coefficient that is used when a collision is detection. | |
virtual iCelCameraMode * | GetCurrentCameraMode ()=0 |
Gets the current camera mode. | |
virtual size_t | GetCurrentCameraModeIndex () const =0 |
Gets the index of the current camera mode. | |
virtual const csVector3 & | GetPos () const =0 |
Gets the current position of the camera. | |
virtual const csVector3 & | GetTarget () const =0 |
Gets the current target of the camera. | |
virtual float | GetTransitionCutoffPosDistance () const =0 |
Grabs the camera transition cutoff distance from position to position between the camera and the camera mode. | |
virtual float | GetTransitionCutoffTargetDistance () const =0 |
Grabs the camera transition cutoff distance from target to target between the camera and the camera mode. | |
virtual float | GetTransitionSpringCoefficient () const =0 |
This gets the springyness of the transition to a new camera mode when a new camera mode is selected. | |
virtual const csVector3 & | GetUp () const =0 |
Gets the current up vector of the camera. | |
virtual bool | InCameraTransition () const =0 |
Determines whether the camera is currently in a transition from one camera mode to another. | |
virtual void | NextCameraMode ()=0 |
Sets the current camera mode to the next available mode. | |
virtual void | PrevCameraMode ()=0 |
Sets the current camera mode to the previous available mode. | |
virtual void | SetCollisionDetection (bool detectCollisions)=0 |
Sets whether the camera will use collision detection to avoid moving through walls. | |
virtual void | SetCollisionSpringCoefficient (float springCoef)=0 |
Sets the spring coefficient that will be used when a collision is detected. | |
virtual bool | SetCurrentCameraMode (size_t modeIndex)=0 |
Sets the current camera mode. | |
virtual void | SetPositionOffset (const csVector3 &offset)=0 |
Sets the offset from the center of the mesh's iMovable to the position of the camera. | |
virtual void | SetTransitionCutoffDistance (float cutOffPosDist, float cutOffTargetDist)=0 |
If the distance between the current camera position and the new camera mode is within this cutoff distance, then the camera will cease to be in a transition and be in the new camera mode. | |
virtual void | SetTransitionSpringCoefficient (float springCoef)=0 |
This controls the springyness of the transition to a new camera mode when a new camera mode is selected. |
Detailed Description
This is a camera property class.
Definition at line 135 of file newcamera.h.
Member Function Documentation
virtual size_t iPcNewCamera::AttachCameraMode | ( | CEL_CAMERA_MODE | mode | ) | [pure virtual] |
Attaches a built-in camera mode to this camera.
- Parameters:
-
mode The id of the built-in camera mode to attach.
- Returns:
- A unique id for the attached camera mode.
virtual size_t iPcNewCamera::AttachCameraMode | ( | iCelCameraMode * | mode | ) | [pure virtual] |
Attaches a camera mode to this camera.
- Parameters:
-
mode The camera mode to attach.
- Returns:
- A unique id for the attached camera mode.
virtual bool iPcNewCamera::DetectCollisions | ( | ) | const [pure virtual] |
Returns whether the camera will use collision detection to avoid moving through walls.
- Returns:
- True if collision detection is enabled.
virtual void iPcNewCamera::Draw | ( | ) | [pure virtual] |
virtual const csVector3& iPcNewCamera::GetBaseDir | ( | ) | const [pure virtual] |
Gets the base direction of the camera.
- Returns:
- The base direction of the camera.
virtual const csVector3& iPcNewCamera::GetBasePos | ( | ) | const [pure virtual] |
Gets the base position of the camera in world coordinates.
- Returns:
- The base position of the camera in world coordinates.
virtual const csReversibleTransform& iPcNewCamera::GetBaseTrans | ( | ) | const [pure virtual] |
Gets the base transform of the camera.
- Returns:
- The base transform of the camera.
virtual const csVector3& iPcNewCamera::GetBaseUp | ( | ) | const [pure virtual] |
Gets the base up vector of the camera.
- Returns:
- The base up vector of the camera.
virtual float iPcNewCamera::GetCollisionSpringCoefficient | ( | ) | const [pure virtual] |
Returns the spring coefficient that is used when a collision is detection.
- Returns:
- The collision detection spring coefficient.
virtual iCelCameraMode* iPcNewCamera::GetCurrentCameraMode | ( | ) | [pure virtual] |
Gets the current camera mode.
- Returns:
- The current camera mode.
virtual size_t iPcNewCamera::GetCurrentCameraModeIndex | ( | ) | const [pure virtual] |
Gets the index of the current camera mode.
- Returns:
- The index of the current camera mode.
virtual const csVector3& iPcNewCamera::GetPos | ( | ) | const [pure virtual] |
Gets the current position of the camera.
- Returns:
- The current position of the camera.
virtual const csVector3& iPcNewCamera::GetTarget | ( | ) | const [pure virtual] |
Gets the current target of the camera.
- Returns:
- The current target of the camera.
virtual float iPcNewCamera::GetTransitionCutoffPosDistance | ( | ) | const [pure virtual] |
Grabs the camera transition cutoff distance from position to position between the camera and the camera mode.
- Returns:
- The camera transition cutoff distance from target to target.
virtual float iPcNewCamera::GetTransitionCutoffTargetDistance | ( | ) | const [pure virtual] |
Grabs the camera transition cutoff distance from target to target between the camera and the camera mode.
- Returns:
- The camera transition cutoff distance from position to position.
virtual float iPcNewCamera::GetTransitionSpringCoefficient | ( | ) | const [pure virtual] |
This gets the springyness of the transition to a new camera mode when a new camera mode is selected.
- Returns:
- The spring coefficient of the camera transitions.
virtual const csVector3& iPcNewCamera::GetUp | ( | ) | const [pure virtual] |
Gets the current up vector of the camera.
- Returns:
- The current up vector of the camera.
virtual bool iPcNewCamera::InCameraTransition | ( | ) | const [pure virtual] |
Determines whether the camera is currently in a transition from one camera mode to another.
- Returns:
- True if the camera is currently in a transition.
virtual void iPcNewCamera::NextCameraMode | ( | ) | [pure virtual] |
Sets the current camera mode to the next available mode.
virtual void iPcNewCamera::PrevCameraMode | ( | ) | [pure virtual] |
Sets the current camera mode to the previous available mode.
virtual void iPcNewCamera::SetCollisionDetection | ( | bool | detectCollisions | ) | [pure virtual] |
Sets whether the camera will use collision detection to avoid moving through walls.
- Parameters:
-
detectCollisions True if the camera should detect collisions.
virtual void iPcNewCamera::SetCollisionSpringCoefficient | ( | float | springCoef | ) | [pure virtual] |
Sets the spring coefficient that will be used when a collision is detected.
- Parameters:
-
springCoef The new spring coefficient.
virtual bool iPcNewCamera::SetCurrentCameraMode | ( | size_t | modeIndex | ) | [pure virtual] |
Sets the current camera mode.
- Parameters:
-
modeIndex The index of the current camera mode.
- Returns:
- True on successful camera mode change.
virtual void iPcNewCamera::SetPositionOffset | ( | const csVector3 & | offset | ) | [pure virtual] |
Sets the offset from the center of the mesh's iMovable to the position of the camera.
- Parameters:
-
offset the offset from the center of the mesh to the camera position.
virtual void iPcNewCamera::SetTransitionCutoffDistance | ( | float | cutOffPosDist, | |
float | cutOffTargetDist | |||
) | [pure virtual] |
If the distance between the current camera position and the new camera mode is within this cutoff distance, then the camera will cease to be in a transition and be in the new camera mode.
- Parameters:
-
cutOffPosDist The camera transition mode cutoff distance from position to position. cutOffTargetDist The camera transition mode cutoff distance from target to target.
virtual void iPcNewCamera::SetTransitionSpringCoefficient | ( | float | springCoef | ) | [pure virtual] |
This controls the springyness of the transition to a new camera mode when a new camera mode is selected.
- Parameters:
-
springCoef The new spring coefficient of camera transitions.
The documentation for this struct was generated from the following file:
- propclass/newcamera.h
Generated for CEL: Crystal Entity Layer by doxygen 1.4.7