iPcDefaultCamera Struct Reference
This is a property class that wraps a standard first-person and third-person camera and related functionality. More...
#include <propclass/defcam.h>
Inheritance diagram for iPcDefaultCamera:
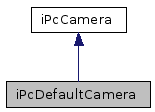
Public Types | |
enum | CameraMode |
Camera modes. More... | |
Public Member Functions | |
virtual void | CenterCamera ()=0 |
Center camera in any mode except first person mode. | |
virtual float | GetDistance (int mode=-1)=0 |
Get the distance between the character's eyes and the camera. | |
virtual float | GetDistanceVelocity () const =0 |
Gets the distance (zoom) velocity of the camera. | |
virtual CameraMode | GetMode () const =0 |
Get camera mode. | |
virtual const char * | GetModeName () const =0 |
Get camera mode name. | |
virtual CameraMode | GetNextMode () const =0 |
Get next possible camera mode. | |
virtual float | GetPitch () const =0 |
Get current pitch. | |
virtual float | GetPitchVelocity () const =0 |
Get current pitch velocity. | |
virtual float | GetYaw (int mode=-1) const =0 |
Returns the yaw (left/right) of the camera. | |
virtual float | GetYawVelocity () const =0 |
Gets the yaw (up/down) velocity of the camera. | |
virtual void | MovePitch (float deltaPitch, int mode=-1)=0 |
Moves the pitch (up/down) of the camera. | |
virtual void | MoveYaw (float deltaYaw, int mode=-1)=0 |
Moves the yaw (left/right) of the camera. | |
virtual bool | PointCamera (const char *start)=0 |
Point the camera to some given location. | |
virtual void | SetDistance (float distance, int mode=-1)=0 |
Set the distance between the character's eyes and the camera. | |
virtual void | SetDistanceVelocity (float distanceVel)=0 |
Sets the distance (zoom) velocity of the camera. | |
virtual void | SetFirstPersonOffset (const csVector3 &offset)=0 |
Set offset for first person camera (offset for camera relative to player model). | |
virtual void | SetFollowEntity (iCelEntity *entity)=0 |
Set the destination entity manually. | |
virtual void | SetMinMaxCameraDistance (float minDistance, float maxDistance)=0 |
Set the minimum and maximum distance between camera and player. | |
virtual bool | SetMode (CameraMode m, bool use_cd=true)=0 |
Set camera mode. | |
virtual bool | SetModeName (const char *m, bool use_cd=true)=0 |
Set camera mode by name. | |
virtual void | SetPitch (float pitch)=0 |
Control pitch. | |
virtual void | SetPitchVelocity (float pitchVel)=0 |
Control velocity of pitch. | |
virtual void | SetSpringParameters (float springCoef, float intertialDampeningCoef, float springLength)=0 |
Set spring parameters for the current camera mode. | |
virtual void | SetSwingCoef (float swingCoef)=0 |
Set the swing coefficient for the camera. | |
virtual void | SetThirdPersonOffset (const csVector3 &offset)=0 |
Set offset for third person camera (offset for camera relative to player model). | |
virtual void | SetTurnSpeed (float turnSpeed)=0 |
Set the turn speed for the camera. | |
virtual void | SetYaw (float yaw, int mode=-1)=0 |
Sets the yaw (left/right) of the camera. | |
virtual void | SetYawVelocity (float yawVel)=0 |
Sets the yaw (up/down) velocity of the camera. |
Detailed Description
This is a property class that wraps a standard first-person and third-person camera and related functionality.It depends on either iPcMesh or iPcLinearMovement.
This property class supports the following actions (add prefix 'cel.action.' to get the ID of the action and add prefix 'cel.parameter.' to get the ID of the parameter):
- SetCamera: parameters 'modename' (string), 'spring' (vector3), 'turnspeed' (float), 'swingcoef' (float), 'fpoffset' (vector3), 'tpoffset' (vector3), 'pitch' (float), 'pitchvelocity' (float), 'yaw' (float), 'yawvelocity' (float) and 'distance' (vector3=min,def,max).
- SetZoneManager: parameters 'entity' (string), 'region' (string) and 'start' (string).
- CenterCamera: no parameters.
- PointCamera: parameters 'start'.
This property class supports the following properties (add prefix 'cel.property.' to get the ID of the property:
- pitchvelocity (float, read/write): pitch velocity.
- yawvelocity (float, read/write): yaw velocity.
- distancevelocity (float, read/write): distance (zoom) velocity.
- pitch (float, read/write): pitch.
- yaw (float, read/write): yaw.
- distance (float, read/write): distance (zoom).
Definition at line 55 of file defcam.h.
Member Enumeration Documentation
Member Function Documentation
virtual void iPcDefaultCamera::CenterCamera | ( | ) | [pure virtual] |
Center camera in any mode except first person mode.
This will basically force the camera behind the actor.
virtual float iPcDefaultCamera::GetDistance | ( | int | mode = -1 |
) | [pure virtual] |
Get the distance between the character's eyes and the camera.
The camera algorithms are responsible for calculating the actual position of the camera.
virtual float iPcDefaultCamera::GetDistanceVelocity | ( | ) | const [pure virtual] |
Gets the distance (zoom) velocity of the camera.
- Returns:
- the distance (zoom) velocity of the camera
virtual CameraMode iPcDefaultCamera::GetMode | ( | ) | const [pure virtual] |
Get camera mode.
virtual const char* iPcDefaultCamera::GetModeName | ( | ) | const [pure virtual] |
Get camera mode name.
virtual CameraMode iPcDefaultCamera::GetNextMode | ( | ) | const [pure virtual] |
Get next possible camera mode.
This is useful for looping over all camera modes with some key in a game.
virtual float iPcDefaultCamera::GetPitch | ( | ) | const [pure virtual] |
Get current pitch.
virtual float iPcDefaultCamera::GetPitchVelocity | ( | ) | const [pure virtual] |
Get current pitch velocity.
virtual float iPcDefaultCamera::GetYaw | ( | int | mode = -1 |
) | const [pure virtual] |
Returns the yaw (left/right) of the camera.
- Parameters:
-
mode Optional - the camera mode to get it from (leave blank for current)
- Returns:
- the yaw (left/right) of the camera
virtual float iPcDefaultCamera::GetYawVelocity | ( | ) | const [pure virtual] |
Gets the yaw (up/down) velocity of the camera.
- Returns:
- the yaw (up/down) of the camera
virtual void iPcDefaultCamera::MovePitch | ( | float | deltaPitch, | |
int | mode = -1 | |||
) | [pure virtual] |
Moves the pitch (up/down) of the camera.
- Parameters:
-
deltaPitch the amount to move from the current pitch mode Optional - the camera mode to apply it to (leave blank for current)
virtual void iPcDefaultCamera::MoveYaw | ( | float | deltaYaw, | |
int | mode = -1 | |||
) | [pure virtual] |
Moves the yaw (left/right) of the camera.
- Parameters:
-
deltaYaw the amount to move from the current yaw mode Optional - the camera mode to apply it to (leave blank for current)
virtual bool iPcDefaultCamera::PointCamera | ( | const char * | start | ) | [pure virtual] |
Point the camera to some given location.
Returns false if the location could not be found.
virtual void iPcDefaultCamera::SetDistance | ( | float | distance, | |
int | mode = -1 | |||
) | [pure virtual] |
Set the distance between the character's eyes and the camera.
The camera algorithms are responsible for calculating the actual position of the camera.
virtual void iPcDefaultCamera::SetDistanceVelocity | ( | float | distanceVel | ) | [pure virtual] |
Sets the distance (zoom) velocity of the camera.
- Parameters:
-
distanceVel the velocity of the distance
virtual void iPcDefaultCamera::SetFirstPersonOffset | ( | const csVector3 & | offset | ) | [pure virtual] |
Set offset for first person camera (offset for camera relative to player model).
virtual void iPcDefaultCamera::SetFollowEntity | ( | iCelEntity * | entity | ) | [pure virtual] |
Set the destination entity manually.
If this is not set then the camera will attempt to fetch mesh, linmove, mechobj from current entity. If 'entity' is 0 it will go back to use the ones from the current entity.
virtual void iPcDefaultCamera::SetMinMaxCameraDistance | ( | float | minDistance, | |
float | maxDistance | |||
) | [pure virtual] |
Set the minimum and maximum distance between camera and player.
Only used by m64_thirdperson, lara_thirdperson, and freelook.
virtual bool iPcDefaultCamera::SetMode | ( | CameraMode | m, | |
bool | use_cd = true | |||
) | [pure virtual] |
Set camera mode.
virtual bool iPcDefaultCamera::SetModeName | ( | const char * | m, | |
bool | use_cd = true | |||
) | [pure virtual] |
Set camera mode by name.
virtual void iPcDefaultCamera::SetPitch | ( | float | pitch | ) | [pure virtual] |
Control pitch.
virtual void iPcDefaultCamera::SetPitchVelocity | ( | float | pitchVel | ) | [pure virtual] |
Control velocity of pitch.
This can be useful for looking up or down.
virtual void iPcDefaultCamera::SetSpringParameters | ( | float | springCoef, | |
float | intertialDampeningCoef, | |||
float | springLength | |||
) | [pure virtual] |
Set spring parameters for the current camera mode.
virtual void iPcDefaultCamera::SetSwingCoef | ( | float | swingCoef | ) | [pure virtual] |
Set the swing coefficient for the camera.
Only used by lara_thirdperson.
virtual void iPcDefaultCamera::SetThirdPersonOffset | ( | const csVector3 & | offset | ) | [pure virtual] |
Set offset for third person camera (offset for camera relative to player model).
virtual void iPcDefaultCamera::SetTurnSpeed | ( | float | turnSpeed | ) | [pure virtual] |
Set the turn speed for the camera.
Only used by lara_thirdperson and m64_thirdperson.
virtual void iPcDefaultCamera::SetYaw | ( | float | yaw, | |
int | mode = -1 | |||
) | [pure virtual] |
Sets the yaw (left/right) of the camera.
- Parameters:
-
yaw the new yaw of the camera mode Optional - the camera mode to apply it to (leave blank for current)
virtual void iPcDefaultCamera::SetYawVelocity | ( | float | yawVel | ) | [pure virtual] |
Sets the yaw (up/down) velocity of the camera.
- Parameters:
-
yawVel the velocity of the yaw
The documentation for this struct was generated from the following file:
- propclass/defcam.h
Generated for CEL: Crystal Entity Layer by doxygen 1.4.7