csFontCache Class Reference
[Common Plugin Classes]
A cache for font glyphs.
More...
#include <csplugincommon/canvas/fontcache.h>
Inheritance diagram for csFontCache:
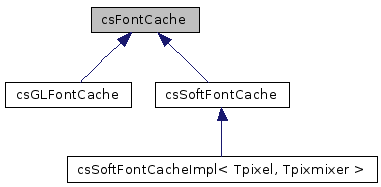
Public Member Functions | |
KnownFont * | CacheFont (iFont *font) |
Set up stuff to cache glyphs of this font. | |
GlyphCacheData * | CacheGlyph (KnownFont *font, utf32_char glyph, uint flags) |
Store glyph-specific information. | |
GlyphCacheData * | GetCacheData (KnownFont *font, utf32_char glyph, uint flags) |
Request cached data for a glyph of a known font. | |
KnownFont * | GetCachedFont (iFont *font) |
Request whether a font is known already. | |
GlyphCacheData * | GetLeastUsed () |
Get cached data for the least used glyph. | |
void | PurgeEmptyPlanes () |
Delete empty PlaneGlyphs from known fonts. | |
void | SetClipRect (int x1, int y1, int x2, int y2) |
void | UncacheFont (iFont *font) |
Uncache this font. | |
void | UncacheGlyph (GlyphCacheData *cacheData) |
Uncache cached glyph data. | |
virtual void | WriteString (iFont *font, int x, int y, int fg, int bg, const void *text, bool isWide, uint flags) |
Draw a string. | |
Public Attributes | |
int | ClipX1 |
the current clipping rect | |
int | ClipX2 |
int | ClipY1 |
int | ClipY2 |
Protected Types | |
typedef csArray< PlaneGlyphs *, PlaneGlyphElementHandler > | PlaneGlyphsArray |
Array of a number of glyphs. | |
Protected Member Functions | |
void | AddCacheData (KnownFont *font, utf32_char glyph, GlyphCacheData *cacheData) |
Add a glyph to the cache. | |
GlyphCacheData * | CacheGlyphUnsafe (KnownFont *font, utf32_char glyph, uint flags) |
Store glyph-specific information, but omit some safety checks. | |
LRUEntry * | FindLRUEntry (GlyphCacheData *cacheData) |
Find an LRU entry for a specific cache data. | |
LRUEntry * | FindLRUEntry (KnownFont *font, utf32_char glyph) |
Find an LRU entry for a specific font/glyph pair. | |
virtual GlyphCacheData * | InternalCacheGlyph (KnownFont *font, utf32_char glyph, uint flags) |
Cache canvas-dependent information for a specific font/glyph pair. | |
GlyphCacheData * | InternalGetCacheData (KnownFont *font, utf32_char glyph) |
Request cached data for a glyph of a known font. | |
virtual void | InternalUncacheGlyph (GlyphCacheData *cacheData) |
Uncache canvas-dependent information. | |
void | RemoveCacheData (GlyphCacheData *cacheData) |
Remove a glyph from the cache. @@ Does not update PlaneGlyphs! | |
void | RemoveLRUEntry (LRUEntry *entry) |
Remove a glyph from the cache. | |
void | SetupCacheData (GlyphCacheData *cacheData, KnownFont *font, utf32_char glyph, uint flags) |
Fill the basic cache data. | |
Static Protected Member Functions | |
static csArrayCmp< KnownFont *, iFont * > | KnownFontArrayKeyFunctor (iFont *f) |
Protected Attributes | |
FontDeleteNotify * | deleteCallback |
LRUEntry * | head |
First entry in LRU list. | |
csArray< KnownFont * > | knownFonts |
Array of known fonts. | |
csBlockAllocator< LRUEntry > | LRUAlloc |
Allocator for LRU list entries. | |
csSet< csPtrKey< KnownFont > > | purgeableFonts |
LRUEntry * | tail |
Last entry in LRU list. | |
Classes | |
struct | FontDeleteNotify |
Font deletion callback. More... | |
struct | GlyphCacheData |
Some basic data associated with a glyph. More... | |
struct | KnownFont |
A font known to the cache. More... | |
struct | LRUEntry |
An entry in the LRU list. More... | |
class | PlaneGlyphElementHandler |
struct | PlaneGlyphs |
Array of a number of glyphs. More... |
Detailed Description
A cache for font glyphs.It is intended as a baseclass, which a canvas extends to store glyphs in a canvas-dependent way. It provides facilities to quickly locate data associated with a specific glyph of a specific font, as well as means to manage glyphs if only a limited space to store them is present.
Definition at line 53 of file fontcache.h.
Member Typedef Documentation
typedef csArray<PlaneGlyphs*, PlaneGlyphElementHandler> csFontCache::PlaneGlyphsArray [protected] |
Array of a number of glyphs.
This is the "first" dimension of the glyphs array, and consists of a variable number of "planes". If a plane doesn't contain a glyph, it doesn't take up memory.
Definition at line 139 of file fontcache.h.
Member Function Documentation
void csFontCache::AddCacheData | ( | KnownFont * | font, | |
utf32_char | glyph, | |||
GlyphCacheData * | cacheData | |||
) | [protected] |
Add a glyph to the cache.
Set up stuff to cache glyphs of this font.
Referenced by csG2DDrawText< Tpixel, Tpixmixer1, Tpixmixer2, Tpixmixer3 >::DrawText().
GlyphCacheData* csFontCache::CacheGlyph | ( | KnownFont * | font, | |
utf32_char | glyph, | |||
uint | flags | |||
) |
Store glyph-specific information.
Referenced by csG2DDrawText< Tpixel, Tpixmixer1, Tpixmixer2, Tpixmixer3 >::DrawText().
GlyphCacheData* csFontCache::CacheGlyphUnsafe | ( | KnownFont * | font, | |
utf32_char | glyph, | |||
uint | flags | |||
) | [protected] |
Store glyph-specific information, but omit some safety checks.
LRUEntry* csFontCache::FindLRUEntry | ( | GlyphCacheData * | cacheData | ) | [protected] |
Find an LRU entry for a specific cache data.
LRUEntry* csFontCache::FindLRUEntry | ( | KnownFont * | font, | |
utf32_char | glyph | |||
) | [protected] |
Find an LRU entry for a specific font/glyph pair.
GlyphCacheData* csFontCache::GetCacheData | ( | KnownFont * | font, | |
utf32_char | glyph, | |||
uint | flags | |||
) |
Request cached data for a glyph of a known font.
Request whether a font is known already.
Referenced by csG2DDrawText< Tpixel, Tpixmixer1, Tpixmixer2, Tpixmixer3 >::DrawText().
GlyphCacheData* csFontCache::GetLeastUsed | ( | ) |
Get cached data for the least used glyph.
virtual GlyphCacheData* csFontCache::InternalCacheGlyph | ( | KnownFont * | font, | |
utf32_char | glyph, | |||
uint | flags | |||
) | [protected, virtual] |
Cache canvas-dependent information for a specific font/glyph pair.
GlyphCacheData* csFontCache::InternalGetCacheData | ( | KnownFont * | font, | |
utf32_char | glyph | |||
) | [protected] |
Request cached data for a glyph of a known font.
virtual void csFontCache::InternalUncacheGlyph | ( | GlyphCacheData * | cacheData | ) | [protected, virtual] |
Uncache canvas-dependent information.
void csFontCache::PurgeEmptyPlanes | ( | ) |
Delete empty PlaneGlyphs from known fonts.
Referenced by csG2DDrawText< Tpixel, Tpixmixer1, Tpixmixer2, Tpixmixer3 >::DrawText().
void csFontCache::RemoveCacheData | ( | GlyphCacheData * | cacheData | ) | [protected] |
Remove a glyph from the cache. @@ Does not update PlaneGlyphs!
void csFontCache::RemoveLRUEntry | ( | LRUEntry * | entry | ) | [protected] |
Remove a glyph from the cache.
void csFontCache::SetupCacheData | ( | GlyphCacheData * | cacheData, | |
KnownFont * | font, | |||
utf32_char | glyph, | |||
uint | flags | |||
) | [protected] |
Fill the basic cache data.
void csFontCache::UncacheFont | ( | iFont * | font | ) |
Uncache this font.
void csFontCache::UncacheGlyph | ( | GlyphCacheData * | cacheData | ) |
Uncache cached glyph data.
Member Data Documentation
the current clipping rect
Definition at line 152 of file fontcache.h.
Referenced by csG2DDrawText< Tpixel, Tpixmixer1, Tpixmixer2, Tpixmixer3 >::DrawText().
LRUEntry* csFontCache::head [protected] |
csArray<KnownFont*> csFontCache::knownFonts [protected] |
csBlockAllocator<LRUEntry> csFontCache::LRUAlloc [protected] |
LRUEntry* csFontCache::tail [protected] |
The documentation for this class was generated from the following file:
- csplugincommon/canvas/fontcache.h
Generated for Crystal Space by doxygen 1.4.7