csMouseDriver Class Reference
Generic Mouse Driver. More...
#include <csutil/csinput.h>
Inheritance diagram for csMouseDriver:
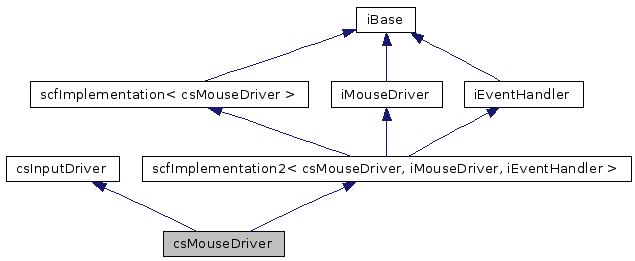
Public Member Functions | |
csMouseDriver (iObjectRegistry *) | |
Initialize mouse interface. | |
virtual void | DoButton (int button, bool down, int x, int y) |
virtual void | DoButton (int button, bool down, const int32 *axes, uint numAxes) |
virtual void | DoButton (uint number, int button, bool down, const int32 *axes, uint numAxes) |
Call this to add a 'mouse button down/up' event to queue. | |
virtual void | DoMotion (int x, int y) |
virtual void | DoMotion (const int32 *axes, uint numAxes) |
virtual void | DoMotion (uint number, const int32 *axes, uint numAxes) |
Call this to add a 'mouse moved' event to queue. | |
virtual void | GainFocus () |
virtual const int32 * | GetLast (uint n) const |
Query last mouse axis array for mouse n (0, 1, 2, ...). | |
virtual int | GetLast (uint n, uint axis) const |
Query last mouse position on axis (0, 1, 2, ...) for mouse n (0, 1, 2, ...). | |
virtual bool | GetLastButton (uint number, int button) const |
Query the last known mouse button state. | |
virtual bool | GetLastButton (int button) const |
Query the last known mouse button state for mouse #0. | |
virtual int | GetLastX (uint n) const |
Query last mouse X position for mouse #n (0, 1, 2, ...). | |
virtual int | GetLastY (uint n) const |
Query last mouse Y position for mouse #n (0, 1, 2, ...). | |
virtual void | LostFocus () |
Application lost focus. | |
virtual void | Reset () |
Call to release all mouse buttons. | |
virtual CS_EVENTHANDLER_NIL_CONSTRAINTS void | SetDoubleClickTime (int iTime, size_t iDist) |
Set double-click mouse parameters. | |
virtual | ~csMouseDriver () |
Destructor. | |
Protected Member Functions | |
iKeyboardDriver * | GetKeyboardDriver () |
Get the generic keyboard driver (for checking modifier states). | |
Protected Attributes | |
uint | Axes [CS_MAX_MOUSE_COUNT] |
bool | Button [CS_MAX_MOUSE_COUNT][CS_MAX_MOUSE_BUTTONS] |
Mouse buttons state. | |
size_t | DoubleClickDist |
Mouse double click max distance. | |
csTicks | DoubleClickTime |
Mouse double click max interval in 1/1000 seconds. | |
int32 | Last [CS_MAX_MOUSE_COUNT][CS_MAX_MOUSE_AXES] |
Last mouse positions. | |
int | LastClick [CS_MAX_MOUSE_COUNT][CS_MAX_MOUSE_AXES] |
Last "mouse down" event position. | |
int | LastClickButton [CS_MAX_MOUSE_COUNT] |
Last "mouse down" event button. | |
csTicks | LastClickTime [CS_MAX_MOUSE_COUNT] |
Last "mouse down" event time. |
Detailed Description
Generic Mouse Driver.Mouse driver should generate events and put them into the event queue. Also it is responsible for generating double-click events. Mouse button numbers are 0-based.
- Todo:
- The csMouseDriver and csJoystickDriver should be unified, since there's no real distinction between them under the hood.
Definition at line 203 of file csinput.h.
Constructor & Destructor Documentation
csMouseDriver::csMouseDriver | ( | iObjectRegistry * | ) |
Initialize mouse interface.
virtual csMouseDriver::~csMouseDriver | ( | ) | [virtual] |
Destructor.
Member Function Documentation
iKeyboardDriver* csMouseDriver::GetKeyboardDriver | ( | ) | [protected] |
Get the generic keyboard driver (for checking modifier states).
Query last mouse position on axis (0, 1, 2, ...) for mouse n (0, 1, 2, ...).
Implements iMouseDriver.
virtual bool csMouseDriver::GetLastButton | ( | uint | number, | |
int | button | |||
) | const [inline, virtual] |
Query the last known mouse button state.
Implements iMouseDriver.
Definition at line 265 of file csinput.h.
References CS_MAX_MOUSE_BUTTONS, and CS_MAX_MOUSE_COUNT.
virtual bool csMouseDriver::GetLastButton | ( | int | button | ) | const [inline, virtual] |
virtual int csMouseDriver::GetLastX | ( | uint | n | ) | const [inline, virtual] |
virtual int csMouseDriver::GetLastY | ( | uint | n | ) | const [inline, virtual] |
virtual void csMouseDriver::LostFocus | ( | ) | [inline, virtual] |
virtual void csMouseDriver::Reset | ( | ) | [virtual] |
virtual CS_EVENTHANDLER_NIL_CONSTRAINTS void csMouseDriver::SetDoubleClickTime | ( | int | iTime, | |
size_t | iDist | |||
) | [virtual] |
Member Data Documentation
bool csMouseDriver::Button[CS_MAX_MOUSE_COUNT][CS_MAX_MOUSE_BUTTONS] [protected] |
size_t csMouseDriver::DoubleClickDist [protected] |
csTicks csMouseDriver::DoubleClickTime [protected] |
int32 csMouseDriver::Last[CS_MAX_MOUSE_COUNT][CS_MAX_MOUSE_AXES] [protected] |
int csMouseDriver::LastClick[CS_MAX_MOUSE_COUNT][CS_MAX_MOUSE_AXES] [protected] |
int csMouseDriver::LastClickButton[CS_MAX_MOUSE_COUNT] [protected] |
csTicks csMouseDriver::LastClickTime[CS_MAX_MOUSE_COUNT] [protected] |
The documentation for this class was generated from the following file:
- csutil/csinput.h
Generated for Crystal Space by doxygen 1.4.7