csPath Class Reference
[Geometry utilities]
A path in 3D.
More...
#include <csgeom/path.h>
Inheritance diagram for csPath:
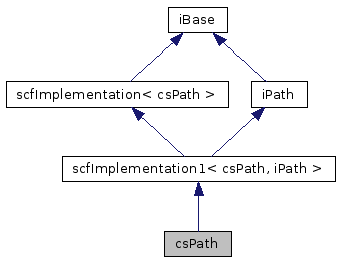
Public Member Functions | |
virtual void | Calculate (float time) |
Calculate internal values for this spline given some time value. | |
virtual void | CalculateAtTime (float time) |
Calculate internal values for this spline given some time value. | |
csPath (int p) | |
Create a path with p points. | |
virtual int | GetCurrentIndex () |
Get current index. | |
float | GetDimensionValue (int dim, int idx) const |
Get the value for some dimension. | |
float const * | GetDimensionValues (int dim) const |
Get the values for some dimension. | |
virtual void | GetForwardVector (int idx, csVector3 &v) |
Get one forward vector. | |
virtual void | GetInterpolatedForward (csVector3 &pos) |
Get the interpolated forward vector. | |
virtual void | GetInterpolatedPosition (csVector3 &pos) |
Get the interpolated position. | |
virtual void | GetInterpolatedUp (csVector3 &pos) |
Get the interpolated up vector. | |
int | GetPointCount () |
Get the number of vector points in this spline. | |
virtual void | GetPositionVector (int idx, csVector3 &v) |
Get one position vector. | |
virtual float | GetTime (int idx) |
Get one time point. | |
float const * | GetTimes () const |
Get the time values. | |
float | GetTimeValue (int idx) const |
Get one time point. | |
float const * | GetTimeValues () const |
Get the time values. | |
virtual void | GetUpVector (int idx, csVector3 &v) |
Get one up vector. | |
void | InsertPoint (int idx) |
Insert a point after some index. | |
virtual int | Length () |
Get the number of vector points in this spline. | |
void | RemovePoint (int idx) |
Remove a point at the index. | |
virtual void | SetForwardVector (int idx, const csVector3 &v) |
Set one forward vector. | |
virtual void | SetForwardVectors (csVector3 *v) |
Set the forward vectors (dimensions 6 to 8). | |
virtual void | SetPositionVector (int idx, const csVector3 &v) |
Set one position vector. | |
virtual void | SetPositionVectors (csVector3 *v) |
Set the position vectors (first three dimensions of the cubic spline). | |
virtual void | SetTime (int idx, float t) |
Set one time point. | |
void | SetTimes (float const *t) |
Set the time values. | |
virtual void | SetTimeValue (int idx, float t) |
Set one time point. | |
void | SetTimeValues (float const *t) |
Set the time values. | |
virtual void | SetUpVector (int idx, const csVector3 &v) |
Set one up vector. | |
virtual void | SetUpVectors (csVector3 *v) |
Set the up vectors (dimensions 3 to 5). | |
virtual | ~csPath () |
Destroy the path. | |
Protected Attributes | |
csCatmullRomSpline | spline |
Detailed Description
A path in 3D.An object or camera can use this object to trace a path in 3D. This is particularly useful in combination with csReversibleTransform::LookAt().
Definition at line 44 of file path.h.
Constructor & Destructor Documentation
csPath::csPath | ( | int | p | ) | [inline] |
virtual csPath::~csPath | ( | ) | [inline, virtual] |
Member Function Documentation
virtual void csPath::Calculate | ( | float | time | ) | [inline, virtual] |
Calculate internal values for this spline given some time value.
- Deprecated:
- Use CalculateAtTime() instead.
virtual void csPath::CalculateAtTime | ( | float | time | ) | [inline, virtual] |
virtual int csPath::GetCurrentIndex | ( | ) | [inline, virtual] |
float csPath::GetDimensionValue | ( | int | dim, | |
int | idx | |||
) | const [inline] |
float const* csPath::GetDimensionValues | ( | int | dim | ) | const [inline] |
virtual void csPath::GetForwardVector | ( | int | idx, | |
csVector3 & | v | |||
) | [inline, virtual] |
Get one forward vector.
Implements iPath.
Definition at line 228 of file path.h.
References csVector3::x, csVector3::y, and csVector3::z.
virtual void csPath::GetInterpolatedForward | ( | csVector3 & | pos | ) | [inline, virtual] |
Get the interpolated forward vector.
Implements iPath.
Definition at line 250 of file path.h.
References csVector3::x, csVector3::y, and csVector3::z.
virtual void csPath::GetInterpolatedPosition | ( | csVector3 & | pos | ) | [inline, virtual] |
Get the interpolated position.
Implements iPath.
Definition at line 236 of file path.h.
References csVector3::x, csVector3::y, and csVector3::z.
virtual void csPath::GetInterpolatedUp | ( | csVector3 & | pos | ) | [inline, virtual] |
Get the interpolated up vector.
Implements iPath.
Definition at line 243 of file path.h.
References csVector3::x, csVector3::y, and csVector3::z.
int csPath::GetPointCount | ( | ) | [inline] |
virtual void csPath::GetPositionVector | ( | int | idx, | |
csVector3 & | v | |||
) | [inline, virtual] |
Get one position vector.
Implements iPath.
Definition at line 214 of file path.h.
References csVector3::x, csVector3::y, and csVector3::z.
virtual float csPath::GetTime | ( | int | idx | ) | [inline, virtual] |
float const* csPath::GetTimes | ( | ) | const [inline] |
float csPath::GetTimeValue | ( | int | idx | ) | const [inline] |
float const* csPath::GetTimeValues | ( | ) | const [inline] |
virtual void csPath::GetUpVector | ( | int | idx, | |
csVector3 & | v | |||
) | [inline, virtual] |
Get one up vector.
Implements iPath.
Definition at line 221 of file path.h.
References csVector3::x, csVector3::y, and csVector3::z.
void csPath::InsertPoint | ( | int | idx | ) | [inline] |
virtual int csPath::Length | ( | ) | [inline, virtual] |
void csPath::RemovePoint | ( | int | idx | ) | [inline] |
virtual void csPath::SetForwardVector | ( | int | idx, | |
const csVector3 & | v | |||
) | [inline, virtual] |
Set one forward vector.
Implements iPath.
Definition at line 207 of file path.h.
References csVector3::x, csVector3::y, and csVector3::z.
virtual void csPath::SetForwardVectors | ( | csVector3 * | v | ) | [inline, virtual] |
virtual void csPath::SetPositionVector | ( | int | idx, | |
const csVector3 & | v | |||
) | [inline, virtual] |
Set one position vector.
Implements iPath.
Definition at line 193 of file path.h.
References csVector3::x, csVector3::y, and csVector3::z.
virtual void csPath::SetPositionVectors | ( | csVector3 * | v | ) | [inline, virtual] |
virtual void csPath::SetTime | ( | int | idx, | |
float | t | |||
) | [inline, virtual] |
void csPath::SetTimes | ( | float const * | t | ) | [inline] |
virtual void csPath::SetTimeValue | ( | int | idx, | |
float | t | |||
) | [inline, virtual] |
void csPath::SetTimeValues | ( | float const * | t | ) | [inline] |
virtual void csPath::SetUpVector | ( | int | idx, | |
const csVector3 & | v | |||
) | [inline, virtual] |
Set one up vector.
Implements iPath.
Definition at line 200 of file path.h.
References csVector3::x, csVector3::y, and csVector3::z.
virtual void csPath::SetUpVectors | ( | csVector3 * | v | ) | [inline, virtual] |
The documentation for this class was generated from the following file:
- csgeom/path.h
Generated for Crystal Space by doxygen 1.4.7