scfImplementation< Class > Class Template Reference
[Shared Class Facility (SCF)]
Baseclass for the SCF implementation templates.
More...
#include <csutil/scf_implementation.h>
Inheritance diagram for scfImplementation< Class >:
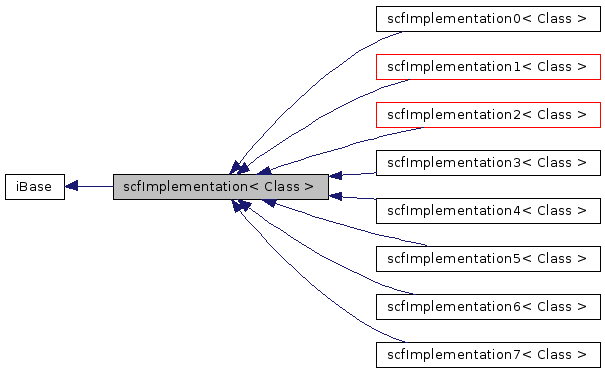
Public Member Functions | |
virtual void | AddRefOwner (void **ref_owner) |
For weak references: add a reference owner. | |
virtual void | DecRef () |
Decrement the reference count. | |
virtual int | GetRefCount () |
Get the ref count (only for debugging). | |
virtual void | IncRef () |
Increment the number of references to this object. | |
scfImplementation & | operator= (const scfImplementation &) |
Assign to another instance. | |
virtual void | RemoveRefOwner (void **ref_owner) |
For weak references: remove a reference owner. | |
scfImplementation (const scfImplementation &) | |
Copy constructor. | |
scfImplementation (Class *object, iBase *parent=0) | |
Constructor. | |
virtual | ~scfImplementation () |
Protected Types | |
typedef csArray< void **, csArrayElementHandler< void ** >, CS::Memory::AllocatorMalloc, csArrayCapacityLinear< csArrayThresholdFixed< 4 > > > | WeakRefOwnerArray |
Protected Member Functions | |
void * | QueryInterface (scfInterfaceID iInterfaceID, scfInterfaceVersion iVersion) |
Query a particular interface implemented by this object. | |
void | scfRemoveRefOwners () |
Protected Attributes | |
Class * | scfObject |
iBase * | scfParent |
int | scfRefCount |
WeakRefOwnerArray * | scfWeakRefOwners |
Detailed Description
template<class Class>
class scfImplementation< Class >
Baseclass for the SCF implementation templates.
Provides common methods such as reference counting and handling of weak references.
Definition at line 126 of file scf_implementation.h.
Constructor & Destructor Documentation
scfImplementation< Class >::scfImplementation | ( | Class * | object, | |
iBase * | parent = 0 | |||
) | [inline] |
Constructor.
Initialize the SCF-implementation in class named Class. Will be called from scfImplementation(Ext)N constructor
Definition at line 133 of file scf_implementation.h.
scfImplementation< Class >::scfImplementation | ( | const scfImplementation< Class > & | ) | [inline] |
Copy constructor.
Use of the default copy constructor is nor desired since the information is insufficient to fully initialize scfImplementation; hence, an explicit copy constructor must be created in the derived class that initializes scfImplementation like in the normal constructor, i.e. "scfImplementation (this)".
Definition at line 149 of file scf_implementation.h.
Member Function Documentation
virtual void scfImplementation< Class >::AddRefOwner | ( | void ** | ref_owner | ) | [inline, virtual] |
For weak references: add a reference owner.
Implements iBase.
Definition at line 201 of file scf_implementation.h.
virtual void scfImplementation< Class >::DecRef | ( | ) | [inline, virtual] |
Decrement the reference count.
Implements iBase.
Reimplemented in csGLScreenShot, csPoolEvent, and scfImplementationPooled< scfImplementationExt0< csParasiticDataBufferPooled, csParasiticDataBufferBase > >.
Definition at line 174 of file scf_implementation.h.
virtual int scfImplementation< Class >::GetRefCount | ( | ) | [inline, virtual] |
Get the ref count (only for debugging).
Implements iBase.
Definition at line 196 of file scf_implementation.h.
virtual void scfImplementation< Class >::IncRef | ( | ) | [inline, virtual] |
Increment the number of references to this object.
Implements iBase.
Reimplemented in csGLScreenShot.
Definition at line 188 of file scf_implementation.h.
scfImplementation& scfImplementation< Class >::operator= | ( | const scfImplementation< Class > & | ) | [inline] |
Assign to another instance.
When assigning an SCF object to another, anything contained in this class should not be copied, since it's all instance- specific. Hence the assignment operator does nothing.
Definition at line 169 of file scf_implementation.h.
void* scfImplementation< Class >::QueryInterface | ( | scfInterfaceID | iInterfaceID, | |
scfInterfaceVersion | iVersion | |||
) | [inline, protected, virtual] |
Query a particular interface implemented by this object.
You are _not_ allowed to cast this to anything but a pointer to this interface (not even iBase). Use scfQueryInterface<interface> instead of using this method directly.
Implements iBase.
Reimplemented in scfImplementation0< Class >, scfImplementation1< Class, I1 >, scfImplementation2< Class, I1, I2 >, scfImplementation3< Class, I1, I2, I3 >, scfImplementation4< Class, I1, I2, I3, I4 >, scfImplementation5< Class, I1, I2, I3, I4, I5 >, scfImplementation6< Class, I1, I2, I3, I4, I5, I6 >, scfImplementation7< Class, I1, I2, I3, I4, I5, I6, I7 >, scfImplementation1< FramePrinter, iEventHandler >, scfImplementation1< csObject, iObject >, scfImplementation1< csRenderBuffer, iRenderBuffer >, scfImplementation1< csThreadJobQueue, iJobQueue >, scfImplementation1< scfStringArray, iStringArray >, scfImplementation1< csShaderExpressionAccessor, iShaderVariableAccessor >, scfImplementation1< csMemFile, iFile >, scfImplementation1< csImageBase, iImage >, scfImplementation1< csCommonImageFile::LoaderJob, iJob >, scfImplementation1< csTiledCoverageBuffer, iDebugHelper >, scfImplementation1< csClipper, iClipper2D >, scfImplementation1< csDataBuffer, iDataBuffer >, scfImplementation1< csNullCacheManager, iCacheManager >, scfImplementation1< csDocumentNodeCommon, iDocumentNode >, scfImplementation1< CS::DocumentHelper::Implementation::FilterDocumentNodeIterator< T >, iDocumentNodeIterator >, scfImplementation1< csConfigFile, iConfigFile >, scfImplementation1< csTextProgressMeter, iProgressMeter >, scfImplementation1< csEventAttributeIterator, iEventAttributeIterator >, scfImplementation1< csTinyDocumentSystem, iDocumentSystem >, scfImplementation1< csNormalizationCubeAccessor, iShaderVariableAccessor >, scfImplementation1< csObjectModel, iObjectModel >, scfImplementation1< scfArrayWrapConst< iGradientShades, csArray< csGradientShade > >, iGradientShades >, scfImplementation1< csEmptyDocumentNodeIterator, iDocumentNodeIterator >, scfImplementation1< scfString, iString >, scfImplementation1< scfArrayWrap< IF, Backend >, IF >, scfImplementation1< csVerbosityManager, iVerbosityManager >, scfImplementation1< csPolygonMeshBox, iPolygonMesh >, scfImplementation1< csPhysicalFile, iFile >, scfImplementation1< csKeyComposer, iKeyComposer >, scfImplementation1< CS::SndSys::SndSysBasicStream, iSndSysStream >, scfImplementation1< csCommonImageFileLoader, iImageFileLoader >, scfImplementation1< csTextureManager, iTextureManager >, scfImplementation1< scfArray< IF, Backend >, IF >, scfImplementation1< csVfsCacheManager, iCacheManager >, scfImplementation1< csEmptyDocumentAttributeIterator, iDocumentAttributeIterator >, scfImplementation1< csView, iView >, scfImplementation1< csEventNameRegistry, iEventNameRegistry >, scfImplementation1< CS::SndSys::SndSysBasicData, iSndSysData >, scfImplementation1< csConfigDocument, iConfigFile >, scfImplementation1< csCommandLineParser, iCommandLineParser >, scfImplementation1< csEventCord, iEventCord >, scfImplementation1< csPluginManager, iPluginManager >, scfImplementation1< csEventQueue, iEventQueue >, scfImplementation1< csEventOutlet, iEventOutlet >, scfImplementation1< csTextureHandle, iTextureHandle >, scfImplementation1< csObjectRegistry, iObjectRegistry >, scfImplementation1< csMeshFactory, iMeshObjectFactory >, scfImplementation1< csPolygonMesh, iPolygonMesh >, scfImplementation1< csFontCache::FontDeleteNotify, iFontDeleteNotify >, scfImplementation1< csKDTree, iDebugHelper >, scfImplementation1< csEventHandlerRegistry, iEventHandlerRegistry >, scfImplementation1< csGeomDebugHelper, iDebugHelper >, scfImplementation1< scfArrayWrapConst< IF, Backend >, IF >, scfImplementation1< csScfStringSet, iStringSet >, scfImplementation1< csParasiticDataBufferBase, iDataBuffer >, scfImplementation1< csBaseEventHandler::EventHandlerImpl, iEventHandler >, scfImplementation1< csShaderVariableContext, scfFakeInterface< iShaderVariableContext > >, scfImplementation1< csDocumentAttributeCommon, iDocumentAttribute >, scfImplementation1< csPath, iPath >, scfImplementation1< csEventTimer, iEventTimer >, scfImplementation1< csWin32RegistryConfig, iConfigFile >, scfImplementation1< csEvent, iEvent >, scfImplementation1< csVirtualClock, iVirtualClock >, scfImplementation1< csGradient, iGradient >, scfImplementation1< FrameBegin3DDraw, iEventHandler >, scfImplementation1< csBaseTextureFactory, iTextureFactory >, scfImplementation2< csKeyboardDriver, iKeyboardDriver, iEventHandler >, scfImplementation2< csJoystickDriver, iJoystickDriver, iEventHandler >, scfImplementation2< csMeshType, iMeshObjectType, iComponent >, scfImplementation2< csEventQueue::PreProcessFrameEventDispatcher, csEventQueue::iTypedFrameEventDispatcher, scfFakeInterface< iEventHandler > >, scfImplementation2< FrameSignpost_Logic3D, iFrameEventSignpost, scfFakeInterface< iEventHandler > >, scfImplementation2< csMouseDriver, iMouseDriver, iEventHandler >, scfImplementation2< csBaseRenderStepType, iRenderStepType, iComponent >, scfImplementation2< csEventQueue::ProcessFrameEventDispatcher, csEventQueue::iTypedFrameEventDispatcher, scfFakeInterface< iEventHandler > >, scfImplementation2< csInputBinder, iInputBinder, iEventHandler >, scfImplementation2< FrameSignpost_ConsoleDebug, iFrameEventSignpost, scfFakeInterface< iEventHandler > >, scfImplementation2< csShaderProgram, iShaderProgram, iShaderDestinationResolver >, scfImplementation2< csConfigManager, iConfigManager, scfFakeInterface< iConfigFile > >, scfImplementation2< FrameSignpost_3D2D, iFrameEventSignpost, scfFakeInterface< iEventHandler > >, scfImplementation2< csEventQueue::PostProcessFrameEventDispatcher, csEventQueue::iTypedFrameEventDispatcher, scfFakeInterface< iEventHandler > >, scfImplementation2< csBaseRenderStepLoader, iLoaderPlugin, iComponent >, scfImplementation2< csEventQueue::FinalProcessFrameEventDispatcher, csEventQueue::iTypedFrameEventDispatcher, scfFakeInterface< iEventHandler > >, scfImplementation2< FrameSignpost_DebugFrame, iFrameEventSignpost, scfFakeInterface< iEventHandler > >, scfImplementation2< FrameSignpost_2DConsole, iFrameEventSignpost, scfFakeInterface< iEventHandler > >, scfImplementation7< csGraphics2D, iGraphics2D, iComponent, iNativeWindow, iNativeWindowManager, iPluginConfig, iDebugHelper, iEventHandler >, scfImplementationExt0< csParasiticDataBufferPooled, csParasiticDataBufferBase >, scfImplementationExt0< csDocumentNodeReadOnly, csDocumentNodeCommon >, scfImplementationExt0< csImageMemory, csImageBase >, scfImplementationExt0< csScreenShot, csImageBase >, scfImplementationExt0< csImageCubeMapMaker, csImageBase >, scfImplementationExt0< csNewtonianParticleSystem, csParticleSystem >, scfImplementationExt0< csParasiticDataBuffer, csParasiticDataBufferBase >, scfImplementationExt0< csImageVolumeMaker, csImageBase >, scfImplementationExt0< csCommonImageFile, csImageMemory >, scfImplementationExt0< csGLScreenShot, csImageBase >, scfImplementationExt1< csMapNode, csObject, iMapNode >, scfImplementationExt1< csColliderWrapper, csObject, scfFakeInterface< csColliderWrapper > >, scfImplementationExt1< csMeshObject, csObjectModel, iMeshObject >, scfImplementationExt1< csKeyValuePair, csObject, iKeyValuePair >, scfImplementationExt2< csParticleSystem, csObjectModel, iMeshObject, iParticleState >, scfImplementationExt2< csSaverFile, csObject, iSaverFile, iSelfDestruct >, scfImplementationExt2< csGraphics2DGLCommon, csGraphics2D, iEventPlug, iOpenGLDriverDatabase >, scfImplementationExt2< csProcTexture, csObject, iTextureWrapper, iProcTexture >, scfImplementationExt2< csAddonReference, csObject, iAddonReference, iSelfDestruct >, and scfImplementationExt2< csLibraryReference, csObject, iLibraryReference, iSelfDestruct >.
Definition at line 245 of file scf_implementation.h.
virtual void scfImplementation< Class >::RemoveRefOwner | ( | void ** | ref_owner | ) | [inline, virtual] |
For weak references: remove a reference owner.
Implements iBase.
Definition at line 208 of file scf_implementation.h.
The documentation for this class was generated from the following file:
- csutil/scf_implementation.h
Generated for Crystal Space by doxygen 1.4.7