iThingFactoryState Struct Reference
[Mesh plugins]
This is the state interface to access the internals of a thing mesh factory.
More...
#include <imesh/thing.h>
Inheritance diagram for iThingFactoryState:
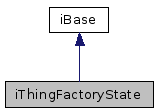
Public Member Functions | |
virtual int | AddEmptyPolygon ()=0 |
Add an empty polygon. | |
virtual int | AddInsideBox (const csVector3 &bmin, const csVector3 &bmax)=0 |
Add a box that can be seen from the inside. | |
virtual int | AddOutsideBox (const csVector3 &bmin, const csVector3 &bmax)=0 |
Add a box that can be seen from the outside. | |
virtual int | AddPolygon (int num,...)=0 |
Add a general polygon using vertex indices. | |
virtual int | AddPolygon (csVector3 *vertices, int num)=0 |
Add a general polygon. | |
virtual void | AddPolygonVertex (const csPolygonRange &range, int vt)=0 |
Add a vertex index to all polygons in the given range. | |
virtual void | AddPolygonVertex (const csPolygonRange &range, const csVector3 &vt)=0 |
Add a vertex to all polygons in the given range. | |
virtual int | AddQuad (const csVector3 &v1, const csVector3 &v2, const csVector3 &v3, const csVector3 &v4)=0 |
Add a quad. | |
virtual int | AddTriangle (const csVector3 &v1, const csVector3 &v2, const csVector3 &v3)=0 |
Add a triangle. | |
virtual void | CompressVertices ()=0 |
Compress the vertex table so that all nearly identical vertices are compressed. | |
virtual csPtr< iPolygonHandle > | CreatePolygonHandle (int polygon_idx)=0 |
Create a polygon handle that can be used to refer to some polygon. | |
virtual int | CreateVertex (const csVector3 &vt)=0 |
Create a vertex given his object-space coords and return his index. | |
virtual void | DeleteVertex (int idx)=0 |
Delete a vertex. | |
virtual void | DeleteVertices (int from, int to)=0 |
Delete a range of vertices (inclusive). | |
virtual int | FindPolygonByName (const char *name)=0 |
Find a polygon index with a name. | |
virtual float | GetCosinusFactor () const =0 |
Get cosinus factor. | |
virtual csVector3 * | GetNormals ()=0 |
Gets the normals. | |
virtual int | GetPolygonCount ()=0 |
Query number of polygons in this thing. | |
virtual csFlags & | GetPolygonFlags (int polygon_idx)=0 |
Get the flags of the specified polygon. | |
virtual iMaterialWrapper * | GetPolygonMaterial (int polygon_idx)=0 |
Get the material for the specified polygon. | |
virtual const char * | GetPolygonName (int polygon_idx)=0 |
Get the name of the specified polygon. | |
virtual const csPlane3 & | GetPolygonObjectPlane (int polygon_idx)=0 |
Get object space plane of the specified polygon. | |
virtual void | GetPolygonTextureMapping (int polygon_idx, csMatrix3 &m, csVector3 &v)=0 |
Get the texture space information for the specified polygon. | |
virtual const csVector3 & | GetPolygonVertex (int polygon_idx, int vertex_idx)=0 |
Get a vertex from a polygon. | |
virtual int | GetPolygonVertexCount (int polygon_idx)=0 |
Get number of vertices for polygon. | |
virtual int * | GetPolygonVertexIndices (int polygon_idx)=0 |
Get table with vertex indices from polygon. | |
virtual bool | GetSmoothingFlag ()=0 |
Gets the smoothing flag. | |
virtual const csVector3 & | GetVertex (int idx) const =0 |
Get the given vertex coordinates in object space. | |
virtual int | GetVertexCount () const =0 |
Query number of vertices in set. | |
virtual const csVector3 * | GetVertices () const =0 |
Get the vertex coordinates in object space. | |
virtual bool | IsPolygonTextureMappingEnabled (int polygon_idx) const =0 |
Check if texture mapping is enabled for the specified polygon. | |
virtual bool | IsPolygonTransparent (int polygon_idx)=0 |
Return true if this polygon or the texture it uses is transparent. | |
virtual bool | PointOnPolygon (int polygon_idx, const csVector3 &v)=0 |
Return true if an object space point is on (or very nearly on) the given polygon. | |
virtual void | RemovePolygon (int idx)=0 |
Delete a polygon given an index. | |
virtual void | RemovePolygons ()=0 |
Delete all polygons. | |
virtual void | ResetPolygonFlags (const csPolygonRange &range, uint32 flags)=0 |
Reset the given flags to all polygons in the range. | |
virtual void | SetCosinusFactor (float cosfact)=0 |
Set cosinus factor. | |
virtual void | SetPolygonFlags (const csPolygonRange &range, uint32 mask, uint32 flags)=0 |
Set the given flags to all polygons in the range. | |
virtual void | SetPolygonFlags (const csPolygonRange &range, uint32 flags)=0 |
Set the given flags to all polygons in the range. | |
virtual void | SetPolygonMaterial (const csPolygonRange &range, iMaterialWrapper *material)=0 |
Set the material of all polygons in the given range. | |
virtual void | SetPolygonName (const csPolygonRange &range, const char *name)=0 |
Set the name of all polygons in the given range. | |
virtual bool | SetPolygonTextureMapping (const csPolygonRange &range, float len)=0 |
Set texture mapping of all polygons in the given range to use the texture mapping as specified by the two first vertices on the polygon. | |
virtual bool | SetPolygonTextureMapping (const csPolygonRange &range, const csVector3 &v_orig, const csVector3 &v1, float len1, const csVector3 &v2, float len2)=0 |
Set texture mapping of all polygons in the given range to use the texture mapping as specified by two vertices on the polygon. | |
virtual bool | SetPolygonTextureMapping (const csPolygonRange &range, const csVector3 &v_orig, const csVector3 &v, float len)=0 |
Set texture mapping of all polygons in the given range to use the texture mapping as specified by two vertices on the polygon. | |
virtual bool | SetPolygonTextureMapping (const csPolygonRange &range, const csVector3 &p1, const csVector2 &uv1, const csVector3 &p2, const csVector2 &uv2, const csVector3 &p3, const csVector2 &uv3)=0 |
Set texture mapping of all polygons in the given range to use the given uv coordinates for the specified three vertices of every polygon. | |
virtual bool | SetPolygonTextureMapping (const csPolygonRange &range, const csVector2 &uv1, const csVector2 &uv2, const csVector2 &uv3)=0 |
Set texture mapping of all polygons in the given range to use the given uv coordinates for the first three vertices of every polygon. | |
virtual bool | SetPolygonTextureMapping (const csPolygonRange &range, const csMatrix3 &m, const csVector3 &v)=0 |
Set texture mapping of all polygons in the given range to use the transform. | |
virtual void | SetPolygonTextureMappingEnabled (const csPolygonRange &range, bool enabled)=0 |
Enable or disable texture mapping for the range of polygons. | |
virtual void | SetPolygonVertexIndices (const csPolygonRange &range, int num, int *indices)=0 |
Set the given polygon index table for all polygons in the given range. | |
virtual void | SetSmoothingFlag (bool smoothing)=0 |
Sets the smoothing flag. | |
virtual void | SetVertex (int idx, const csVector3 &vt)=0 |
Set the object space vertices for a given vertex. | |
Lightmap query | |
virtual bool | GetLightmapLayout (int polygon_idx, size_t &slm, csRect &slmSubRect, float *slmCoord)=0 |
Query the lightmap layout of a polygon. |
Detailed Description
This is the state interface to access the internals of a thing mesh factory.Main creators of instances implementing this interface:
- Thing mesh object plugin (crystalspace.mesh.object.thing)
- iMeshObjectType::NewFactory()
Main ways to get pointers to this interface:
- scfQueryInterface() on iMeshFactoryWrapper::GetMeshObjectFactory()
- scfQueryInterface() on iMeshObject::GetFactory()
Main users of this interface:
- Thing Factory Loader plugin (crystalspace.mesh.loader.factory.thing)
Definition at line 203 of file thing.h.
Member Function Documentation
virtual int iThingFactoryState::AddEmptyPolygon | ( | ) | [pure virtual] |
Add an empty polygon.
- Returns:
- the index of the created polygon.
virtual int iThingFactoryState::AddInsideBox | ( | const csVector3 & | bmin, | |
const csVector3 & | bmax | |||
) | [pure virtual] |
Add a box that can be seen from the inside.
This will add six polygons.
By default the texture mapping is set so that the texture is aligned on the u-axis with the 'v1'-'v2' vector and the scale is set so that the texture tiles once for every unit (i.e. if you have the vertices v1 and v2 are 5 units separated from each other then the texture will repeat exactly five times between v1 and v2).
- Returns:
- the index of the first created polygon.
virtual int iThingFactoryState::AddOutsideBox | ( | const csVector3 & | bmin, | |
const csVector3 & | bmax | |||
) | [pure virtual] |
Add a box that can be seen from the outside.
This will add six polygons.
By default the texture mapping is set so that the texture is aligned on the u-axis with the 'v1'-'v2' vector and the scale is set so that the texture tiles once for every unit (i.e. if you have the vertices v1 and v2 are 5 units separated from each other then the texture will repeat exactly five times between v1 and v2).
- Returns:
- the index of the first created polygon.
virtual int iThingFactoryState::AddPolygon | ( | int | num, | |
... | ||||
) | [pure virtual] |
Add a general polygon using vertex indices.
By default the texture mapping is set so that the texture is aligned on the u-axis with the 'v1'-'v2' vector and the scale is set so that the texture tiles once for every unit (i.e. if you have the vertices v1 and v2 are 5 units separated from each other then the texture will repeat exactly five times between v1 and v2).
- Returns:
- the index of the created polygon.
virtual int iThingFactoryState::AddPolygon | ( | csVector3 * | vertices, | |
int | num | |||
) | [pure virtual] |
Add a general polygon.
By default the texture mapping is set so that the texture is aligned on the u-axis with the 'v1'-'v2' vector and the scale is set so that the texture tiles once for every unit (i.e. if you have the vertices v1 and v2 are 5 units separated from each other then the texture will repeat exactly five times between v1 and v2).
- Returns:
- the index of the created polygon.
virtual void iThingFactoryState::AddPolygonVertex | ( | const csPolygonRange & | range, | |
int | vt | |||
) | [pure virtual] |
Add a vertex index to all polygons in the given range.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. vt the vertex index to be added to the polygons.
virtual void iThingFactoryState::AddPolygonVertex | ( | const csPolygonRange & | range, | |
const csVector3 & | vt | |||
) | [pure virtual] |
Add a vertex to all polygons in the given range.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. vt the vertex index to be added to the polygons.
virtual int iThingFactoryState::AddQuad | ( | const csVector3 & | v1, | |
const csVector3 & | v2, | |||
const csVector3 & | v3, | |||
const csVector3 & | v4 | |||
) | [pure virtual] |
Add a quad.
Note that quads are the most optimal kind of polygon for a thing so you should try to use these as much as possible.
By default the texture mapping is set so that the texture is aligned on the u-axis with the 'v1'-'v2' vector and the scale is set so that the texture tiles once for every unit (i.e. if you have the vertices v1 and v2 are 5 units separated from each other then the texture will repeat exactly five times between v1 and v2).
- Returns:
- the index of the created polygon.
virtual int iThingFactoryState::AddTriangle | ( | const csVector3 & | v1, | |
const csVector3 & | v2, | |||
const csVector3 & | v3 | |||
) | [pure virtual] |
Add a triangle.
By default the texture mapping is set so that the texture is aligned on the u-axis with the 'v1'-'v2' vector and the scale is set so that the texture tiles once for every unit (i.e. if you have the vertices v1 and v2 are 5 units separated from each other then the texture will repeat exactly five times between v1 and v2).
- Returns:
- the index of the created polygon.
virtual void iThingFactoryState::CompressVertices | ( | ) | [pure virtual] |
Compress the vertex table so that all nearly identical vertices are compressed.
The polygons in the set are automatically adapted. This function can be called at any time in the creation of the object and it can be called multiple time but it normally only makes sense to call this function after you have finished adding all polygons and all vertices.
virtual csPtr<iPolygonHandle> iThingFactoryState::CreatePolygonHandle | ( | int | polygon_idx | ) | [pure virtual] |
Create a polygon handle that can be used to refer to some polygon.
This can be useful in situations where an SCF handle is required to be able to reference a polygon. The thing will not keep a reference to this handle so you are fully responsible for it after calling this function. The polygon handle created here will not have a mesh object or thing state set since it is created from the factory.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon.
virtual int iThingFactoryState::CreateVertex | ( | const csVector3 & | vt | ) | [pure virtual] |
Create a vertex given his object-space coords and return his index.
virtual void iThingFactoryState::DeleteVertex | ( | int | idx | ) | [pure virtual] |
Delete a vertex.
Warning this will invalidate all polygons that use vertices after this vertex because their vertex indices will no longer be ok.
virtual void iThingFactoryState::DeleteVertices | ( | int | from, | |
int | to | |||
) | [pure virtual] |
Delete a range of vertices (inclusive).
Warning this will invalidate all polygons that use vertices after these vertices because their vertex indices will no longer be ok. This function does bounds-checking so an easy way to delete all vertices is DeleteVertices(0,1000000000).
virtual int iThingFactoryState::FindPolygonByName | ( | const char * | name | ) | [pure virtual] |
Find a polygon index with a name.
virtual float iThingFactoryState::GetCosinusFactor | ( | ) | const [pure virtual] |
Get cosinus factor.
virtual bool iThingFactoryState::GetLightmapLayout | ( | int | polygon_idx, | |
size_t & | slm, | |||
csRect & | slmSubRect, | |||
float * | slmCoord | |||
) | [pure virtual] |
Query the lightmap layout of a polygon.
- Parameters:
-
polygon_idx Index of the polygon to query. slm Returns on which superlightmap the lightmap is. slmSubRect Returns the rectangle of the lightmap on the superlightmap. slmCoord The coordinates of the lightmap on the superlightmap.
- Returns:
- 'True' if the polygon has a lightmap, 'false' otherwise.
virtual csVector3* iThingFactoryState::GetNormals | ( | ) | [pure virtual] |
Gets the normals.
virtual int iThingFactoryState::GetPolygonCount | ( | ) | [pure virtual] |
Query number of polygons in this thing.
virtual csFlags& iThingFactoryState::GetPolygonFlags | ( | int | polygon_idx | ) | [pure virtual] |
Get the flags of the specified polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon.
virtual iMaterialWrapper* iThingFactoryState::GetPolygonMaterial | ( | int | polygon_idx | ) | [pure virtual] |
Get the material for the specified polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon.
virtual const char* iThingFactoryState::GetPolygonName | ( | int | polygon_idx | ) | [pure virtual] |
Get the name of the specified polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon.
virtual const csPlane3& iThingFactoryState::GetPolygonObjectPlane | ( | int | polygon_idx | ) | [pure virtual] |
Get object space plane of the specified polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon.
virtual void iThingFactoryState::GetPolygonTextureMapping | ( | int | polygon_idx, | |
csMatrix3 & | m, | |||
csVector3 & | v | |||
) | [pure virtual] |
Get the texture space information for the specified polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon. m the matrix which will receive the mapping. v the vector which will receive the mapping.
virtual const csVector3& iThingFactoryState::GetPolygonVertex | ( | int | polygon_idx, | |
int | vertex_idx | |||
) | [pure virtual] |
Get a vertex from a polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon. vertex_idx the desired vertex.
virtual int iThingFactoryState::GetPolygonVertexCount | ( | int | polygon_idx | ) | [pure virtual] |
Get number of vertices for polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon.
virtual int* iThingFactoryState::GetPolygonVertexIndices | ( | int | polygon_idx | ) | [pure virtual] |
Get table with vertex indices from polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon.
virtual bool iThingFactoryState::GetSmoothingFlag | ( | ) | [pure virtual] |
Gets the smoothing flag.
virtual const csVector3& iThingFactoryState::GetVertex | ( | int | idx | ) | const [pure virtual] |
Get the given vertex coordinates in object space.
virtual int iThingFactoryState::GetVertexCount | ( | ) | const [pure virtual] |
Query number of vertices in set.
virtual const csVector3* iThingFactoryState::GetVertices | ( | ) | const [pure virtual] |
Get the vertex coordinates in object space.
virtual bool iThingFactoryState::IsPolygonTextureMappingEnabled | ( | int | polygon_idx | ) | const [pure virtual] |
Check if texture mapping is enabled for the specified polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon.
virtual bool iThingFactoryState::IsPolygonTransparent | ( | int | polygon_idx | ) | [pure virtual] |
Return true if this polygon or the texture it uses is transparent.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon.
virtual bool iThingFactoryState::PointOnPolygon | ( | int | polygon_idx, | |
const csVector3 & | v | |||
) | [pure virtual] |
Return true if an object space point is on (or very nearly on) the given polygon.
- Parameters:
-
polygon_idx is a polygon index or CS_POLYINDEX_LAST for last created polygon. v the point in object space to test against the polygon.
virtual void iThingFactoryState::RemovePolygon | ( | int | idx | ) | [pure virtual] |
Delete a polygon given an index.
virtual void iThingFactoryState::RemovePolygons | ( | ) | [pure virtual] |
Delete all polygons.
virtual void iThingFactoryState::ResetPolygonFlags | ( | const csPolygonRange & | range, | |
uint32 | flags | |||
) | [pure virtual] |
Reset the given flags to all polygons in the range.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. flags a mask of the polygon flags, such as CS_POLY_LIGHTING, CS_POLY_COLLDET, etc.
virtual void iThingFactoryState::SetCosinusFactor | ( | float | cosfact | ) | [pure virtual] |
Set cosinus factor.
This cosinus factor controls how lighting affects the polygons relative to the angle. If no value is set here then the default is used.
virtual void iThingFactoryState::SetPolygonFlags | ( | const csPolygonRange & | range, | |
uint32 | mask, | |||
uint32 | flags | |||
) | [pure virtual] |
Set the given flags to all polygons in the range.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. mask a mask of the polygon flags, such as CS_POLY_LIGHTING, CS_POLY_COLLDET, etc. Only the indicated bits from `flags' will be assigned. flags The set of bits to assign to the corresponding flags indicated by `mask'.
virtual void iThingFactoryState::SetPolygonFlags | ( | const csPolygonRange & | range, | |
uint32 | flags | |||
) | [pure virtual] |
Set the given flags to all polygons in the range.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. flags a mask of the polygon flags, such as CS_POLY_LIGHTING, CS_POLY_COLLDET, etc.
virtual void iThingFactoryState::SetPolygonMaterial | ( | const csPolygonRange & | range, | |
iMaterialWrapper * | material | |||
) | [pure virtual] |
Set the material of all polygons in the given range.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. material the material to be assigned to the indicated polygons.
virtual void iThingFactoryState::SetPolygonName | ( | const csPolygonRange & | range, | |
const char * | name | |||
) | [pure virtual] |
Set the name of all polygons in the given range.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. name the name to be assigned to the indicated polygons.
virtual bool iThingFactoryState::SetPolygonTextureMapping | ( | const csPolygonRange & | range, | |
float | len | |||
) | [pure virtual] |
Set texture mapping of all polygons in the given range to use the texture mapping as specified by the two first vertices on the polygon.
The first vertex is seen as the origin and the second as the u-axis of the texture space coordinate system. The v-axis is calculated on the plane of the polygons and orthogonal to the given u-axis. The length of the u-axis and the v-axis is given as the 'len' parameter.
For example, if 'len1' is equal to 2 this means that texture will be tiled exactly two times between the two first vertices.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. len length of the u-axis and the v-axis is given as the 'len1' parameter.
- Returns:
- false in case the texture mapping was invalid. In that case a default texture mapping will be set on the polygon.
virtual bool iThingFactoryState::SetPolygonTextureMapping | ( | const csPolygonRange & | range, | |
const csVector3 & | v_orig, | |||
const csVector3 & | v1, | |||
float | len1, | |||
const csVector3 & | v2, | |||
float | len2 | |||
) | [pure virtual] |
Set texture mapping of all polygons in the given range to use the texture mapping as specified by two vertices on the polygon.
The first vertex is seen as the origin, the second as the u-axis of the texture space coordinate system, and the third as the v-axis. The length of the u-axis and the v-axis is given with the 'len1' and 'len2' parameters.
For example, if 'len1' is equal to 2 this means that texture will be tiled exactly two times between vertex 'v_orig' and 'v1'.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. v_orig origin. v1 u-axis in texture space coordinate system. len1 length of u-axis. v2 v-axis in texture space coordinate system. len2 length of v-axis.
- Returns:
- false in case the texture mapping was invalid. In that case a default texture mapping will be set on the polygon.
virtual bool iThingFactoryState::SetPolygonTextureMapping | ( | const csPolygonRange & | range, | |
const csVector3 & | v_orig, | |||
const csVector3 & | v, | |||
float | len | |||
) | [pure virtual] |
Set texture mapping of all polygons in the given range to use the texture mapping as specified by two vertices on the polygon.
The first vertex is seen as the origin and the second as the u-axis of the texture space coordinate system. The v-axis is calculated on the plane of the polygons and orthogonal to the given u-axis. The length of the u-axis and the v-axis is given as the 'len' parameter.
For example, if 'len' is equal to 2 this means that texture will be tiled exactly two times between vertex 'v_orig' and 'v'.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. v_orig origin. v u-axis in texture space coordinate system. len length of u-axis.
- Returns:
- false in case the texture mapping was invalid. In that case a default texture mapping will be set on the polygon.
virtual bool iThingFactoryState::SetPolygonTextureMapping | ( | const csPolygonRange & | range, | |
const csVector3 & | p1, | |||
const csVector2 & | uv1, | |||
const csVector3 & | p2, | |||
const csVector2 & | uv2, | |||
const csVector3 & | p3, | |||
const csVector2 & | uv3 | |||
) | [pure virtual] |
Set texture mapping of all polygons in the given range to use the given uv coordinates for the specified three vertices of every polygon.
Note that this function is only useful for polygon ranges that are on the same plane.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. p1 vertex 1. uv1 uv coordinates for vertex 1. p2 vertex 2. uv2 uv coordinates for vertex 2. p3 vertex 3. uv3 uv coordinates for vertex 3.
- Returns:
- false in case the texture mapping was invalid. In that case a default texture mapping will be set on the polygon.
virtual bool iThingFactoryState::SetPolygonTextureMapping | ( | const csPolygonRange & | range, | |
const csVector2 & | uv1, | |||
const csVector2 & | uv2, | |||
const csVector2 & | uv3 | |||
) | [pure virtual] |
Set texture mapping of all polygons in the given range to use the given uv coordinates for the first three vertices of every polygon.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. uv1 uv coordinates for vertex 1. uv2 uv coordinates for vertex 2. uv3 uv coordinates for vertex 3.
- Returns:
- false in case the texture mapping was invalid. In that case a default texture mapping will be set on the polygon.
virtual bool iThingFactoryState::SetPolygonTextureMapping | ( | const csPolygonRange & | range, | |
const csMatrix3 & | m, | |||
const csVector3 & | v | |||
) | [pure virtual] |
Set texture mapping of all polygons in the given range to use the transform.
This function is usually not used by application code as it is complicated to specify texture mapping like this. It is recommended to use one of the other texture mapping routines.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon m the matrix which defines the mapping. v the vector which defines the mapping. range.
- Returns:
- false in case the texture mapping was invalid. In that case a default texture mapping will be set on the polygon.
virtual void iThingFactoryState::SetPolygonTextureMappingEnabled | ( | const csPolygonRange & | range, | |
bool | enabled | |||
) | [pure virtual] |
Enable or disable texture mapping for the range of polygons.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon range. enabled boolean flag indicating if mapping should be enabled or disabled.
virtual void iThingFactoryState::SetPolygonVertexIndices | ( | const csPolygonRange & | range, | |
int | num, | |||
int * | indices | |||
) | [pure virtual] |
Set the given polygon index table for all polygons in the given range.
It is more optimal to call this routine as opposed to calling AddPolygonVertex() all the time.
- Parameters:
-
range is one of the CS_POLYRANGE defines to specify a polygon num number of entires in `indices'. indices polygon index table to be assigned to range. range.
virtual void iThingFactoryState::SetSmoothingFlag | ( | bool | smoothing | ) | [pure virtual] |
Sets the smoothing flag.
virtual void iThingFactoryState::SetVertex | ( | int | idx, | |
const csVector3 & | vt | |||
) | [pure virtual] |
Set the object space vertices for a given vertex.
The documentation for this struct was generated from the following file:
- imesh/thing.h
Generated for Crystal Space by doxygen 1.4.7