Creating Visual Effects in JavaFX
8 Adding a Perspective
The perspective effect creates a three-dimensional effect of otherwise two-dimensional object.
Figure 8-1 shows the perspective effect.
A perspective transformation can map any square to another square, while preserving the straightness of the lines. Unlike affine transformations, the parallelism of lines in the source is not necessarily preserved in the output.
Note: This effect does not adjust the coordinates of input events or any methods that measure containment on a node. Mouse clicking and the containment methods are undefined if a perspective effect is applied to a node. |
Example 8-1 is a code snippet from the sample application that shows how to create a perspective effect.
Example 8-1 Perspective Effect
static Node perspective() { Group g = new Group(); PerspectiveTransform pt = new PerspectiveTransform(); pt.setUlx(10.0f); pt.setUly(10.0f); pt.setUrx(210.0f); pt.setUry(40.0f); pt.setLrx(210.0f); pt.setLry(60.0f); pt.setLlx(10.0f); pt.setLly(90.0f); g.setEffect(pt); g.setCache(true); Rectangle r = new Rectangle(); r.setX(10.0f); r.setY(10.0f); r.setWidth(280.0f); r.setHeight(80.0f); r.setFill(Color.DARKBLUE); Text t = new Text(); t.setX(20.0f); t.setY(65.0f); t.setText("Perspective"); t.setFill(Color.RED); t.setFont(Font.font("null", FontWeight.BOLD, 36)); g.getChildren().add(r); g.getChildren().add(t); return g; }
Figure 8-2 shows which coordinates affect the resulting image.
Figure 8-2 Coordinates for Perspective Effect
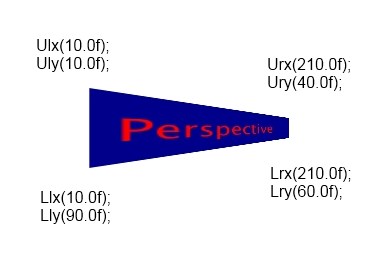
Description of "Figure 8-2 Coordinates for Perspective Effect"