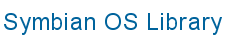
![]() |
![]() |
|
Location:
SpriteAnimation.h
Link against: animation.lib
class CSpriteAnimation : public CAnimation, public MAnimationDataProviderObserver;
Implementation of CAnimation
for sprite based animations.
A sprite animation displays the image using a sprite, which is a server side graphic object. This is likely to be more efficient than a basic animation, and requires slightly less work in the client application. However, it also provides less control over the actual rendering of the image.
A sprite animation must be associated with an RWindow
. However, no redraw events will be generated for it by the animation, and the client application does not need to take any
action once the animation has started. Holding the animation when the sprite is not visible is handled automatically on the
server side.
MAnimationDataProviderObserver
- Interface from a data provider to an animation
CBase
- Base class for all classes to be instantiated on the heap
CAnimation
- Pure virtual base class for animations
CSpriteAnimation
- Implementation of
Defined in CSpriteAnimation
:
CSpriteAnimation_Reserved2()
, DataProvider()
, DataProviderEventL()
, Freeze()
, Hold()
, NewL()
, NewL()
, NewL()
, NewL()
, Pause()
, Resume()
, SetHostL()
, SetPosition()
, Size()
, Start()
, Stop()
, Unfreeze()
, Unhold()
, ~CSpriteAnimation()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from MAnimationDataProviderObserver
:
MAnimationDataProviderObserver_Reserved1()
,
MAnimationDataProviderObserver_Reserved2()
static IMPORT_C CSpriteAnimation *NewL(CAnimationDataProvider *aDataProvider, const TPoint &aPoint, RWsSession &aWsSession,
RWindow &aWindow, MAnimationObserver *aObserver=0);
Two stage constructor.
This creates and returns a new sprite animation.
|
|
static IMPORT_C CSpriteAnimation *NewL(CAnimationDataProvider *aDataProvider, const TPoint &aPoint, RWsSession &aWsSession,
RWindow &aWindow, const TDesC8 &aDataType, MAnimationObserver *aObserver=0);
Two stage constructor.
This is identical to the other NewL except that it allows an alternative data type to be specified. Unless you are trying to use a custom animator class the other form of constructor should be used.
|
|
static IMPORT_C CSpriteAnimation *NewL(CAnimationDataProvider *aDataProvider, const TPoint &aPoint, MAnimationObserver *aObserver=0,
const CCoeControl *aHost=0);
Two stage constructor.
This creates and returns a new sprite animation.
|
|
static IMPORT_C CSpriteAnimation *NewL(CAnimationDataProvider *aDataProvider, const TPoint &aPoint, const TDesC8 &aDataType,
MAnimationObserver *aObserver=0, const CCoeControl *aHost=0);
Two stage constructor.
This is identical to the other NewL except that it allows an alternative data type to be specified. Unless you are trying to use a custom animator class the other form of constructor should be used.
|
|
inline CAnimationDataProvider *DataProvider();
Provides an CSpriteAnimation DataProvider interface to the client application.
|
virtual void Start(const TAnimationConfig &aConfig);
Implements CAnimation::Start
.
|
virtual void SetPosition(const TPoint &aPoint);
Implements CAnimation::SetPosition
.
|
virtual IMPORT_C void SetHostL(const CCoeControl *aHost);
|
private: virtual void DataProviderEventL(TInt aEvent, TAny *aData, TInt aDataSize);
Receives an event from a data provider.
|