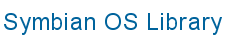
![]() |
![]() |
|
Location:
APACMDLN.H
Link against: apparc.lib
class CApaCommandLine : public CBase;
Information for launching an application.
This is often referred to as a command line and contains:
the name of an application EXE to be launched,
a document name,
a command code that defines the way the application is launched
trailing data; the structure of this depends on the application to be launched.
The information is held in a buffer implemented by a heap descriptor.
CBase
- Base class for all classes to be instantiated on the heap
CApaCommandLine
- Information for launching an application
Defined in CApaCommandLine
:
Command()
, DefaultScreen()
, DocumentName()
, EIpcFirstFreeSlot
, EnvironmentSlotForPublicUse()
, ExecutableName()
, NewL()
, NewLC()
, OpaqueData()
, ParentProcessId()
, ParentWindowGroupID()
, ServerRequired()
, SetCommandL()
, SetDefaultScreenL()
, SetDocumentNameL()
, SetExecutableNameL()
, SetOpaqueDataL()
, SetParentProcessId()
, SetParentWindowGroupID()
, SetProcessEnvironmentL()
, SetServerNotRequiredL()
, SetServerRequiredL()
, SetTailEndL()
, TailEnd()
, anonymous
, ~CApaCommandLine()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
static IMPORT_C CApaCommandLine *NewL();
Creates an empty command line object.
|
static IMPORT_C CApaCommandLine *NewLC();
Creates an empty command line object, and puts a pointer to it onto the cleanup stack.
|
IMPORT_C ~CApaCommandLine();
Destructor.
Frees resources owned by the object prior to deletion.
IMPORT_C void SetProcessEnvironmentL(RProcess &aProcess) const;
Assigns a command line to a process (EKA2 only).
This replaces the EKA1 method which involved retrieving the full command line (using CApaCommandLine::FullCommandLine()) and passing it to the process (or thread on the emulator).
This function is used as follows (the order of the first 2 steps is irrelevant):-
create the process and load the executable (RProcess::Create()
),
create the command line object (CApaCommandLine::NewLC()
), and set it up using the various setter functions, for instance SetDocumentNameL()
,
call SetProcessEnvironmentL()
to assign the command line to the process,
call Resume() on the process.
Note that this sequence of steps bypasses the application architecture, and is not recommended. RApaLsSession::StartApp()
is the recommended way to launch an application with a command line.
|
|
IMPORT_C void SetDocumentNameL(const TDesC &aDocName);
Sets the document name from the specified descriptor.
If the document name has embedded spaces, then it must be enclosed within quotation marks.
|
IMPORT_C TPtrC DocumentName() const;
Gets the document name from the launch information.
|
IMPORT_C void SetExecutableNameL(const TDesC &aAppName);
Sets the executable name from the specified descriptor.
|
IMPORT_C TPtrC ExecutableName() const;
Gets the executable name from the launch information.
|
IMPORT_C void SetOpaqueDataL(const TDesC8 &aOpaqueData);
Sets the opaque-data from the specified 8-bit descriptor.
This is called internally and populated from the data in the application's registration resource file, i.e. from the resource indicated by the opaque_data field of the APP_REGISTRATION_INFO resource (if the opaque_data field was non-zero).
|
IMPORT_C TPtrC8 OpaqueData() const;
Gets the opaque-data from the launch information.
See the description of SetOpaqueDataL. By default, this attribute is an empty descriptor.
|
IMPORT_C void SetTailEndL(const TDesC8 &aTailEnd);
Sets the trailing data.
The UI Framework provides a specific set of pre-defined command line options. Additional user defined or pre-defined command line options, may be passed to an application by setting the TailEnd.
|
IMPORT_C TPtrC8 TailEnd() const;
Gets the trailing data from the launch information.
See the description of SetTailEndL.
|
IMPORT_C void SetCommandL(TApaCommand aCommand);
Sets the command code.
The command code is used to indicate how an application is to be launched.
|
IMPORT_C TApaCommand Command() const;
Gets the command code from the launch information.
See the description of SetCommandL.
|
IMPORT_C void SetParentProcessId(TProcessId aProcessId);
Sets the Parent Process ID for the Child Process launched with this command line.
This establishes a Parent-Child relationship which ensures that the child process is terminated when the parent terminates.
|
IMPORT_C TProcessId ParentProcessId() const;
Gets the Parent Process ID of the Child Process launched with this command line.
See the description of SetParentProcessId.
|
IMPORT_C void SetServerNotRequiredL();
Sets that no server is required.
The value of server differentiator is set to zero, to indicate that no server is required.
See the description of SetServerRequiredL.
IMPORT_C void SetServerRequiredL(TUint aServerDifferentiator);
Sets the required server.
The server differentiator is a number generated by the client that helps to uniquely identify the server. It is used by an application to indicate whether a server should be created and how it should be named.
|
IMPORT_C TUint ServerRequired() const;
Gets the server differentiator.
See the description of SetServerRequiredL.
|
IMPORT_C void SetDefaultScreenL(TInt aDefaultScreenNumber);
Provides support for devices with more than one screen. A number representing the default or startup screen may be passed to an application. Screen numbers and characteristics are defined in the window server initialisation file (wsini.ini).
|
IMPORT_C TInt DefaultScreen() const;
Extracts and returns the default (startup) screen that was specified in the command line.
|
IMPORT_C void SetParentWindowGroupID(TInt aParentWindowGroupID);
Sets the ID of the parent window-group - the application should create its own window-group as a child off this parent.
|
IMPORT_C TInt ParentWindowGroupID() const;
Returns the ID of the parent window-group - the application should create its own window-group as a child of this parent.
|
static IMPORT_C TInt EnvironmentSlotForPublicUse(TInt aIndex);
Returns the index of a process environment-slot for public use (in other words, one that is not used internally by CApaCommandLine).
The number of slots available for public use can be found in the (private) enum value CApaCommandLine::ENumberOfEnvironmentSlotsForPublicUse
, (this value may be increased over time). The returned value can then be passed into any of the Open(TInt,...) functions
on RSessionBase
, RMutex
, RChunk
, RCondVar
, etc, or into User::GetTIntParameter()
, User::GetDesParameter()
, etc, depending on the type of the object in that environment slot.
|
|