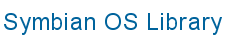
![]() |
![]() |
|
Location:
BITSTD.H
Link against: bitgdi.lib
class CFbsBitGc : public CBitmapContext;
Concrete implementation of a bitmapped graphics context.
The class provides new functionality, and implementations of the pure virtual functions defined in CGraphicsContext
and CBitmapContext
.
CBase
- Base class for all classes to be instantiated on the heap
CGraphicsContext
- Abstract base class for all graphics contexts
CBitmapContext
- An abstract, device-independent, interface to bitmapped graphics contexts
CFbsBitGc
- Concrete implementation of a bitmapped graphics context
Defined in CFbsBitGc
:
APIExtension()
, Activate()
, ActivateNoJustAutoUpdate()
, AlphaBlendBitmaps()
, AlphaBlendBitmaps()
, AlphaBlendBitmaps()
, BitBlt()
, BitBlt()
, BitBlt()
, BitBlt()
, BitBltMasked()
, CancelClipping()
, CancelClippingRect()
, CancelClippingRegion()
, Clear()
, Clear()
, CopyRect()
, CopySettings()
, Device()
, DiscardBrushPattern()
, DiscardFont()
, DrawArc()
, DrawBitmap()
, DrawBitmap()
, DrawBitmap()
, DrawBitmapMasked()
, DrawBitmapMasked()
, DrawEllipse()
, DrawLine()
, DrawLineBy()
, DrawLineTo()
, DrawPie()
, DrawPolyLine()
, DrawPolyLine()
, DrawPolyLineNoEndPoint()
, DrawPolyLineNoEndPoint()
, DrawPolygon()
, DrawPolygon()
, DrawRect()
, DrawRoundRect()
, DrawText()
, DrawText()
, DrawText()
, DrawText()
, DrawText()
, DrawTextVertical()
, DrawTextVertical()
, DrawTextVertical()
, DrawTextVertical()
, DrawTextVertical()
, EGraphicsOrientationNormal
, EGraphicsOrientationRotated180
, EGraphicsOrientationRotated270
, EGraphicsOrientationRotated90
, FadeArea()
, IsBrushPatternUsed()
, IsFontUsed()
, Load16()
, MapColors()
, MoveBy()
, MoveTo()
, NewL()
, OrientationsAvailable()
, Plot()
, RectDrawnTo()
, Reserved_CBitmapContext_1()
, Reserved_CBitmapContext_2()
, Reserved_CBitmapContext_3()
, Reserved_CFbsBitGc_1()
, Reserved_CFbsBitGc_2()
, Reserved_CFbsBitGc_3()
, Reserved_CFbsBitGc_4()
, Reserved_CFbsBitGc_5()
, Reserved_CGraphicsContext_2()
, Reset()
, Resized()
, SetBrushColor()
, SetBrushOrigin()
, SetBrushStyle()
, SetCharJustification()
, SetClippingRect()
, SetClippingRegion()
, SetClippingRegion()
, SetDitherOrigin()
, SetDrawMode()
, SetFadeMode()
, SetFaded()
, SetFadingParameters()
, SetFadingParameters()
, SetOrientation()
, SetOrigin()
, SetPenColor()
, SetPenSize()
, SetPenStyle()
, SetShadowMode()
, SetStrikethroughStyle()
, SetUnderlineStyle()
, SetUserDisplayMode()
, SetWordJustification()
, ShadowArea()
, TGraphicsOrientation
, UpdateJustification()
, UpdateJustificationVertical()
, UseBrushPattern()
, UseBrushPattern()
, UseFont()
, UseFont()
, UseFontNoDuplicate()
, ~CFbsBitGc()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CGraphicsContext
:
DrawTextExtended()
,
EAlternate
,
EAnd
,
ECenter
,
EDashedPen
,
EDiamondCrossHatchBrush
,
EDotDashPen
,
EDotDotDashPen
,
EDottedPen
,
EDrawModeAND
,
EDrawModeANDNOT
,
EDrawModeNOTAND
,
EDrawModeNOTANDNOT
,
EDrawModeNOTOR
,
EDrawModeNOTORNOT
,
EDrawModeNOTPEN
,
EDrawModeNOTSCREEN
,
EDrawModeNOTXOR
,
EDrawModeOR
,
EDrawModeORNOT
,
EDrawModePEN
,
EDrawModeWriteAlpha
,
EDrawModeXOR
,
EForwardDiagonalHatchBrush
,
EHorizontalHatchBrush
,
EInvertPen
,
EInvertScreen
,
ELeft
,
ELogicalOp
,
ENullBrush
,
ENullPen
,
EOr
,
EPatternedBrush
,
EPenmode
,
ERearwardDiagonalHatchBrush
,
ERight
,
ESolidBrush
,
ESolidPen
,
ESquareCrossHatchBrush
,
EVerticalHatchBrush
,
EWinding
,
EWriteAlpha
,
EXor
,
GetUnderlineMetrics()
,
JustificationInPixels()
,
Reserved()
,
TBrushStyle
,
TDrawMode
,
TDrawModeComponents
,
TDrawTextExtendedParam
,
TDrawTextParam
,
TFillRule
,
TPenStyle
,
TTextAlign
static IMPORT_C CFbsBitGc *NewL();
Factory function for creating a CFbsBitGc object The object is then ready for a call to Activate()
.
|
IMPORT_C void Activate(CFbsDevice *aDevice);
Sets the object to draw to a particular device
|
IMPORT_C void ActivateNoJustAutoUpdate(CFbsDevice *aDevice);
Sets the object to draw to a particular device but doesn't 'use up' justification settings when drawing text. This is similar
to Activate()
.
|
IMPORT_C void BitBlt(const TPoint &aPoint, const CFbsBitGc &aGc);
Draws from another CFbsBitGc.
|
IMPORT_C void BitBlt(const TPoint &aPoint, const CFbsBitGc &aGc, const TRect &aSourceRect);
Draws a particular rectangle from another CFbsBitGc.
|
virtual IMPORT_C void BitBlt(const TPoint &aPoint, const CFbsBitmap *aBitmap);
Draws the whole of a CFbsBitmap
.
|
virtual IMPORT_C void BitBlt(const TPoint &aPoint, const CFbsBitmap *aBitmap, const TRect &aSourceRect);
Draws a particular rectangle from a CFbsBitmap
.
|
virtual IMPORT_C void BitBltMasked(const TPoint &aPoint, const CFbsBitmap *aBitmap, const TRect &aSourceRect, const CFbsBitmap
*aMaskBitmap, TBool aInvertMask);
Performs a masked bitmap block transfer.
The function provides a concrete implementation of the pure virtual function CBitmapContext::BitBltMasked()
. The function behaviour is the same as documented in that class.
There are several points to note about this implementation of BitBltMasked()
:
1.For best performance the aMaskBitmap and source aBitmap should have the same display mode as the destination device/bitmap.
2.For performance reasons this implementation does not validate the contents of the aMaskBitmap. The caller must ensure the
mask pixels are either black or white otherwise undefined blitting causing unpredictable discoloration will result. This is
especially true for index (where pixel is palette entry) display modes (e.g. EColor16). It is up to the caller to decide if
they wish to utilise CFbsBitmap::IsMonochrome()
.
3.Alpha blending is used when the display mode of the aMaskBitmap is EGray256.
|
virtual IMPORT_C void CancelClippingRect();
Cancels any clipping rectangle. Clipping reverts to the full device area, the default. The function provides a concrete implementation
of the pure virtual function CGraphicsContext::CancelClippingRect()
. The function behaviour is the same as documented in that class.
virtual IMPORT_C void Clear();
Clears the whole bitmap or a rectangular area of a bitmap.
The cleared area is filled with the current brush colour.
The function provides a concrete implementation of the pure virtual function CBitmapContext::Clear()
. The function behaviour is the same as documented in that class.
virtual IMPORT_C void Clear(const TRect &aRect);
Clears a rectangular area.
The cleared area is filled with the current brush colour.The function provides a concrete implementation of the pure virtual
function CBitmapContext::Clear(const TRect& aRect)
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void CopyRect(const TPoint &aOffset, const TRect &aRect);
Copies a rectangle.
The function provides a concrete implementation of the pure virtual function CBitmapContext::CopyRect()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void CopySettings(const CFbsBitGc &aGc);
Copies all settings from the specified bitmap graphics context.
|
virtual IMPORT_C CGraphicsDevice *Device() const;
Gets a pointer to the graphics device for the graphics context. The graphics device is the device currently being drawn to.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::Device()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void DiscardBrushPattern();
Discards a non-built-in brush pattern. The function provides a concrete implementation of the pure virtual function CGraphicsContext::DiscardBrushPattern()
. The function behaviour is the same as documented in that class.
virtual IMPORT_C void DiscardFont();
Discards a selected device font. The function provides a concrete implementation of the pure virtual function CGraphicsContext::DiscardFont()
. The function behaviour is the same as documented in that class.
virtual IMPORT_C void DrawArc(const TRect &aRect, const TPoint &aStart, const TPoint &aEnd);
Draws an arc.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawArc()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void DrawPie(const TRect &aRect, const TPoint &aStart, const TPoint &aEnd);
Draws and fills a pie slice.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawPie()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void DrawBitmap(const TPoint &aTopLeft, const CFbsBitmap *aSource);
Draws a bitmap.
The function has 3 overloads. The first draws the bitmap given the top left hand corner, doing a compress/stretch based on
its internally stored size in twips. The second does a compress/stretch to fit a given rectangle. The third takes a rectangular
section of the source bitmap and does a compress/stretch to fit a given destination rectangle.The functions provide a concrete
implementation of the pure virtual function CGraphicsContext::DrawBitmap()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void DrawBitmap(const TRect &aDestRect, const CFbsBitmap *aSource);
Draws a bitmap to fit a given rectangle.
The bitmap is compressed or stretched based on its internally stored size in pixels.
Notes:
This member function uses the bitmap's size in pixels and does a stretch/compress blit using a linear DDA.
As this function scales the bitmap, it is unavoidably slow. Therefore, where possible, use CBitmapContext::BitBlt()
instead. If the bitmap has to be scaled, consider creating another bitmap along with an CFbsBitmapDevice
etc., doing DrawBitmap()
once and using BitBlt()
subsequently.
Note that all bitmaps are clipped to the device boundaries.
|
virtual IMPORT_C void DrawBitmap(const TRect &aDestRect, const CFbsBitmap *aSource, const TRect &aSourceRect);
Draws a specified rectangle of a source bitmap to fit into a given destination rectangle.
Notes:
This member function uses rectangle sizes in pixels and does a stretch/compress blit using a linear DDA.
As this function scales the bitmap, it is unavoidably slow. Therefore, where possible, use CBitmapContext::BitBlt()
instead. If the bitmap has to be scaled, consider creating another bitmap along with an CFbsBitmapDevice
etc., doing DrawBitmap()
once and using BitBlt()
subsequently.
Note that all bitmaps are clipped to the device boundaries.
|
virtual IMPORT_C void DrawBitmapMasked(const TRect &aDestRect, const CFbsBitmap *aBitmap, const TRect &aSourceRect, const
CFbsBitmap *aMaskBitmap, TBool aInvertMask);
aBitmap != NULL
aBitmap->Handle() != 0
aMaskBitmap != NULL
aMaskBitmap->Handle() != 0
!aSourceRect.IsEmpty()
The method draws a specified rectangle from a bitmap and its mask into another rectangle and does a compress/stretch to fit a given destination rectangle.
Note: When using this function with a 256 Mask bitmap, it blends. Otherwise (e.g. with a 4bpp mask), this function masks rather
than blends. If a user wants to blend the source into the destination they should use CFbsBitGc::AlphaBlendBitmaps()
instead.
|
virtual IMPORT_C void DrawBitmapMasked(const TRect &aDestRect, const CWsBitmap *aBitmap, const TRect &aSourceRect, const CWsBitmap
*aMaskBitmap, TBool aInvertMask);
aBitmap != NULL
aBitmap->Handle() != 0
aMaskBitmap != NULL
aMaskBitmap->Handle() != 0
!aSourceRect.IsEmpty()
The method draws a specified rectangle from a bitmap and its mask into another rectangle and does a compress/stretch to fit a given destination rectangle.
This is an overload, which takes CWsBitmap* as argument, which in turn calls the other overload.
Note: A pointer to CWsBitmap must have the same pointer value as a pointer to the associated CFbsBitmap
, otherwise code in BitGdi component will be Broken.
Note: When using this function with a 256 Mask bitmap, it blends. Otherwise (e.g. with a 4bpp mask), this function masks rather
than blends. If a user wants to blend the source into the destination they should use CFbsBitGc::AlphaBlendBitmaps()
instead.
|
virtual IMPORT_C void DrawRoundRect(const TRect &aRect, const TSize &aEllipse);
Draws and fills a rectangle with rounded corners.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawRoundRect()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void DrawPolyLine(const CArrayFix< TPoint > *aPointList);
Draws a polyline from a set of points specified in a list.
The functions provides a concrete implementation of the pure virtual functions CGraphicsContext::DrawPolyLine()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void DrawPolyLineNoEndPoint(const CArrayFix< TPoint > *aPointList);
Draws a polyline from a set of points specified in an array, but does not draw the final point of the last line.
|
virtual IMPORT_C void DrawPolyLine(const TPoint *aPointList, TInt aNumPoints);
Draws a polyline from a set of points specified in a list.
The functions provides a concrete implementation of the pure virtual functions CGraphicsContext::DrawPolyLine()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void DrawPolyLineNoEndPoint(const TPoint *aPointList, TInt aNumPoints);
Draws a polyline from a set of points specified in a list, but does not draw the final point of the last line.
|
IMPORT_C TInt DrawPolygon(const CArrayFix< TPoint > *aPointList, CGraphicsContext::TFillRule aFillRule=CGraphicsContext::EAlternate);
Draws and fills a polygon defined using a list of points.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawPolygon()
. The function behaviour is the same as documented in that class.
|
|
IMPORT_C TInt DrawPolygon(const TPoint *aPointList, TInt aNumPoints, CGraphicsContext::TFillRule aFillRule=CGraphicsContext::EAlternate);
Draws and fills a polygon defined using a list of points.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawPolygon()
. The function behaviour is the same as documented in that class.
|
|
virtual IMPORT_C void DrawEllipse(const TRect &aRect);
Draws and fills an ellipse.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawEllipse()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void DrawLine(const TPoint &aStart, const TPoint &aEnd);
Draws a straight line between two points.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawLine()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void DrawLineTo(const TPoint &aPoint);
Draws a straight line from the current drawing point to a specified point.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawLineTo()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void DrawLineBy(const TPoint &aVector);
Draws a straight line relative to the current drawing point, using a vector.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawLineBy()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void DrawRect(const TRect &aRect);
Draws and fills a rectangle.
The function provides a concrete implementation of the pure virtual function CGraphicsContext::DrawRect()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void DrawText(const TDesC &aText);
Draws text at the last print position.
|
virtual IMPORT_C void DrawText(const TDesC &aText, const TPoint &aPosition);
Draws text at the specified position and updates the print position.
|
IMPORT_C void DrawText(const TDesC &aText, const TRect &aBox);
Draws text clipped to the specified rectangle.
|
virtual IMPORT_C void DrawText(const TDesC &aText, const TRect &aBox, TInt aBaselineOffset, TTextAlign aHrz=ELeft, TInt aMargin=0);
Draws text clipped to the specified rectangle using a baseline offset, horizontal alignment and a margin.
|
IMPORT_C void DrawText(const TDesC &aText, const TRect &aBox, TInt aBaselineOffset, TInt aTextWidth, TTextAlign aHrz=ELeft,
TInt aMargin=0);
Draws text clipped to the specified rectangle.
|
IMPORT_C void DrawTextVertical(const TDesC &aText, TBool aUp);
Draws text at the last print position and then rotates it into a vertical position.
|
virtual IMPORT_C void DrawTextVertical(const TDesC &aText, const TPoint &aPosition, TBool aUp);
Draws text vertically from the specified position.
|
IMPORT_C void DrawTextVertical(const TDesC &aText, const TRect &aBox, TBool aUp);
Draws text clipped to the specified rectangle and then rotates it into a vertical position.
|
virtual IMPORT_C void DrawTextVertical(const TDesC &aText, const TRect &aBox, TInt aBaselineOffset, TBool aUp, TTextAlign
aVert=ELeft, TInt aMargin=0);
Draws text vertically, clipped to a specified rectangle, using a baseline offset, alignment and margin.
|
IMPORT_C void DrawTextVertical(const TDesC &aText, const TRect &aBox, TInt aBaselineOffset, TInt aTextWidth, TBool aUp, TTextAlign
aVert=ELeft, TInt aMargin=0);
Draws text vertically, clipped to a specified rectangle, using a baseline offset, alignment and margin.
|
virtual IMPORT_C void MapColors(const TRect &aRect, const TRgb *aColors, TInt aNumPairs=2, TBool aMapForwards=ETrue);
Maps pixels in the specified rectangle. The function tries to match the colour of a pixel with one of the RGB values in an array of RGB pairs. If there is a match, the colour is changed to the value specified in the other RGB in the RGB pair.
|
virtual IMPORT_C void MoveTo(const TPoint &aPoint);
Sets the internal drawing position relative to the co-ordinate origin. A subsequent call to DrawLineTo()
or DrawLineBy()
uses the new drawing point as the start point for the line drawn.The function provides a concrete implementation of the pure
virtual function CGraphicsContext::MoveTo()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void MoveBy(const TPoint &aVector);
Sets the drawing point relative to the current co-ordinates. The function provides a concrete implementation of the pure virtual
function CGraphicsContext::MoveBy()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void OrientationsAvailable(TBool aOrientation[4]);
Gets the orientations supported.
|
virtual IMPORT_C void Plot(const TPoint &aPoint);
Draws a single point.
The point is drawn with the current pen settings using the current drawing mode.The function provides a concrete implementation
of the pure virtual function CGraphicsContext::Plot()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void RectDrawnTo(TRect &aRect);
Sets the bounding rectangle of all drawing done since this function was last called.
|
virtual IMPORT_C void Reset();
Resets the graphics context to its default settings. The function provides a concrete implementation of the pure virtual function
CGraphicsContext::Reset()
. The function behaviour is the same as documented in that class.
IMPORT_C void Resized();
Needs to be called if the device is resized. This only applies to devices of type CFbsBitmapDevice
.
virtual IMPORT_C void SetBrushColor(const TRgb &aColor);
Sets the brush colour. The function provides a concrete implementation of the pure virtual function CGraphicsContext::SetBrushColor()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetBrushOrigin(const TPoint &aOrigin);
Sets the brush pattern origin. The function provides a concrete implementation of the pure virtual function CGraphicsContext::SetBrushOrigin()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetBrushStyle(TBrushStyle aBrushStyle);
Sets the brush style. The function provides a concrete implementation of the pure virtual function CGraphicsContext::SetBrushStyle()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void SetClippingRegion(const TRegion *aRegion);
Sets a clipping region by storing a pointer to the TRegion
parameter.
|
virtual IMPORT_C void SetClippingRect(const TRect &aRect);
Sets the clipping rectangle the area of visible drawing depends on the clipping region. The default clipping rectangle is
the full device area.The function provides a concrete implementation of the pure virtual function CGraphicsContext::SetClippingRect()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void SetDitherOrigin(const TPoint &aPoint);
Sets the dither origin. This is only useful for modes that do dithering. If the display is scrolled an odd number of pixels then the (2x2) dither pattern will not match up for new drawing unless this is called.
|
IMPORT_C void SetDrawMode(TDrawMode);
Sets the drawing mode. This affects the colour that is actually drawn, because it defines the way that the current screen
colour logically combines with the current pen colour and brush colour. The function provides a concrete implementation of
the pure virtual function CGraphicsContext::SetDrawMode()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetOrigin(const TPoint &aPoint=TPoint(0, 0));
Sets the position of the co-ordinate origin. All subsequent drawing operations are then done relative to this origin.The function
provides a concrete implementation of the pure virtual function CGraphicsContext::SetOrigin()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetPenColor(const TRgb &aColor);
Sets the pen colour. The function provides a concrete implementation of the pure virtual function CGraphicsContext::SetPenColor()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetPenStyle(TPenStyle);
Sets the line drawing style for the pen. The function provides a concrete implementation of the pure virtual function CGraphicsContext::SetPenStyle()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetPenSize(const TSize &aSize);
Sets the line drawing size for the pen. The function provides a concrete implementation of the pure virtual function CGraphicsContext::SetPenSize()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetCharJustification(TInt aExcessWidth, TInt aNumGaps);
Sets the character justification. The function provides a concrete implementation of the pure virtual function CGraphicsContext::SetCharJustification()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetWordJustification(TInt aExcessWidth, TInt aNumChars);
Sets the word justification. The function provides a concrete implementation of the pure virtual function CGraphicsContext::SetWordJustification()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetUnderlineStyle(TFontUnderline aUnderlineStyle);
Sets the underline style for all subsequently drawn text. The function provides a concrete implementation of the pure virtual
function CGraphicsContext::SetUnderlineStyle()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void SetUserDisplayMode(TDisplayMode aDisplayMode);
Simulates another graphics mode. Some devices running in some modes can simulate other modes (EGray16 will do EGray4 and EGray2, EGray4 will do EGray2).
|
virtual IMPORT_C void SetStrikethroughStyle(TFontStrikethrough aStrikethroughStyle);
Sets the strikethrough style for all subsequently drawn text. The function provides a concrete implementation of the pure
virtual function CGraphicsContext::SetStrikethroughStyle()
. The function behaviour is the same as documented in that class.
|
IMPORT_C void SetShadowMode(TBool aShadowMode=EFalse);
Sets the shadow mode on or off.
|
virtual IMPORT_C void SetFaded(TBool aFaded);
Sets whether the graphics context is faded. The function provides a concrete implementation of the pure virtual function CBitmapContext::SetFaded()
. The function behaviour is the same as documented in that class.
|
virtual IMPORT_C void SetFadingParameters(TUint8 aBlackMap, TUint8 aWhiteMap);
Set fading parameters. The function provides a concrete implementation of the pure virtual function CBitmapContext::SetFadingParameters()
. The function behaviour is the same as documented in that class.
|
IMPORT_C TBool SetOrientation(TGraphicsOrientation aOrientation);
Sets the orientation.
|
|
IMPORT_C void ShadowArea(const TRegion *aRegion);
Sets the shadow area.
|
IMPORT_C void FadeArea(const TRegion *aRegion);
Sets the fade area.
|
IMPORT_C void UpdateJustification(const TDesC &aText);
Updates the justification settings. This function assumes that ActivateNoJustAutoUpdate()
has been used.
|
IMPORT_C void UpdateJustificationVertical(const TDesC &aText, TBool aUp);
Updates the justification for vertical text.
|
virtual IMPORT_C void UseBrushPattern(const CFbsBitmap *aBitmap);
Sets the specified bitmap to be used as the brush pattern.
|
IMPORT_C TInt UseBrushPattern(TInt aFbsBitmapHandle);
Sets the specified bitmap to be used as the brush pattern.
|
|
virtual IMPORT_C void UseFont(const CFont *aFont);
Selects the device font, identified by handle number, to be used for text drawing. Notes: When the font is no longer required,
use DiscardFont()
to free up the memory used. If UseFont()
is used again without using DiscardFont()
then the previous font is discarded automatically. If no font has been selected, and an attempt is made to draw text with
DrawText()
, then a panic occurs.
|
IMPORT_C TInt UseFont(TInt aFontHandle);
Selects the device font, identified by handle, to be used for text drawing. Notes:When the font is no longer required, use
DiscardFont()
to free up the memory used. If UseFont()
is used again without using DiscardFont()
then the previous font is discarded automatically.If no font has been selected, and an attempt is made to draw text with
DrawText()
, then a panic occurs.
|
|
IMPORT_C void UseFontNoDuplicate(const CFbsBitGcFont *aFont);
Selects a device font for text drawing but does not take a copy. The original must not be destroyed until UseFont()
, UseFontNoDuplicate()
, DiscardFont()
or the destructor is called.
|
IMPORT_C TBool IsBrushPatternUsed() const;
Tests whether a brush pattern is being used.
|
IMPORT_C TBool IsFontUsed() const;
Tests whether a font is used.
|
static inline TInt16 Load16(const TUint8 *aPtr);
|
|
IMPORT_C TInt AlphaBlendBitmaps(const TPoint &aDestPt, const CFbsBitmap *aSrcBmp1, const CFbsBitmap *aSrcBmp2, const TRect
&aSrcRect1, const TPoint &aSrcPt2, const CFbsBitmap *aAlphaBmp, const TPoint &aAlphaPt);
The method performs an alpha blending of the source data - aSrcBmp1 and aSrcBmp2, using the data from aAlphaBmp as an alpha blending factor. The formula used for that, is: (C1 * A + C2 * (255 - A)) / 255, where:
C1 - a pixel from aSrcBmp1;
C2 - a pixel from aSrcBmp2;
A - a pixel from aAlphaBmp; The content of source and alpha bitmap is preserved. The calculated alpha blended pixels are written to the destination - the screen or a bitmap.
|
|
virtual IMPORT_C TInt AlphaBlendBitmaps(const TPoint &aDestPt, const CFbsBitmap *aSrcBmp, const TRect &aSrcRect, const CFbsBitmap
*aAlphaBmp, const TPoint &aAlphaPt);
The method performs an alpha blending of the source data - aSrcBmp - with the existing image, using the data from aAlphaBmp as an alpha blending factor. The formula used for that, is: (C * A + D * (255 - A)) / 255, where:
C - a pixel from aSrcBmp;
D - a pixel from the destination;
A - a pixel from aAlphaBmp; The content of source and alpha bitmap is preserved. The calculated alpha blended pixels are written to the destination - the screen or a bitmap.
|
|
virtual IMPORT_C TInt SetClippingRegion(const TRegion &aRegion);
Sets a clipping region by storing a copy of the TRegion
parameter.
|
|
virtual IMPORT_C TInt AlphaBlendBitmaps(const TPoint &aDestPt, const CWsBitmap *aSrcBmp, const TRect &aSrcRect, const CWsBitmap
*aAlphaBmp, const TPoint &aAlphaPt);
The method performs an alpha blending of the source data, aSrcBmp, with the CBitmapContext
, using the data from aAlphaBmp as an alpha blending factor. For information on how this function works, see the other overload.
|
|
protected: virtual IMPORT_C TInt APIExtension(TUid aUid, TAny *&aOutput, TAny *aInput);
This function should not be used by externals but must retain the same ordinal number to maintain BC, thus is exported.
|
|
private: virtual IMPORT_C void Reserved_CGraphicsContext_2();
A reserved virtual function for future use.
TGraphicsOrientation
Defines possible rotation values.
|