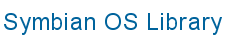
![]() |
![]() |
|
Location:
BITDEV.H
Link against: bitgdi.lib
class CFbsBitmapDevice : public CFbsDevice;
A graphics device to which a bitmap managed by the font and bitmap server can be drawn.
The class specialises the bitmap graphics device interface CBitmapDevice
for drawing to in-memory bitmaps.
MGraphicsDeviceMap
- Interface class for mapping between twips and device-specific units (pixels)
CBase
- Base class for all classes to be instantiated on the heap
CGraphicsDevice
- Specifies the interface for concrete device classes
CBitmapDevice
- Defines an abstract interface for the capabilities and attributes of a bitmapped graphics device
CFbsDevice
- Abstract base class for graphics devices to which bitmaps and fonts can be drawn
CFbsBitmapDevice
- A graphics device to which a bitmap managed by the font and bitmap server can be drawn
Defined in CFbsBitmapDevice
:
DrawingBegin()
, DrawingEnd()
, GetPalette()
, GetPixel()
, GetScanLine()
, HorizontalPixelsToTwips()
, HorizontalTwipsToPixels()
, NewL()
, NewL()
, PaletteAttributes()
, Resize()
, SetBits()
, SetPalette()
, SizeInTwips()
, SwapWidthAndHeight()
, VerticalPixelsToTwips()
, VerticalTwipsToPixels()
, ~CFbsBitmapDevice()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CBitmapDevice
:
CreateBitmapContext()
Inherited from CFbsDevice
:
AddFile()
,
CancelSprite()
,
CreateContext()
,
DisplayMode()
,
DisplayMode16M()
,
FontHeightInPixels()
,
FontHeightInTwips()
,
GetDrawRect()
,
GetFontById()
,
GetNearestFontInPixels()
,
GetNearestFontInTwips()
,
GetNearestFontToDesignHeightInPixels()
,
GetNearestFontToDesignHeightInTwips()
,
GetNearestFontToMaxHeightInPixels()
,
GetNearestFontToMaxHeightInTwips()
,
GraphicsAccelerator()
,
HideSprite()
,
NumTypefaces()
,
Orientation()
,
RectCompare()
,
ReleaseFont()
,
RemoveFile()
,
SetCustomPalette()
,
SetScalingFactor()
,
ShowSprite()
,
SizeInPixels()
,
TypefaceSupport()
,
iBitBltMaskedBuffer
,
iDrawDevice
,
iFbs
,
iGraphicsAccelerator
,
iOrientation
,
iScreenDevice
,
iSpare
,
iTypefaceStore
Inherited from MGraphicsDeviceMap
:
PixelsToTwips()
,
TwipsToPixels()
static IMPORT_C CFbsBitmapDevice *NewL(CFbsBitmap *aFbsBitmap);
Allocates and constructs the device with the bitmap. Also creates a 2D graphics accelerator which is owned and used by the device.
|
|
|
|
static IMPORT_C CFbsBitmapDevice *NewL(CFbsBitmap *aFbsBitmap, const TDesC &aLibname);
Constructs the object from the specified Font and Bitmap server managed bitmap.
|
|
virtual IMPORT_C ~CFbsBitmapDevice();
Frees all resources owned by the object prior to its destruction.
IMPORT_C TInt Resize(const TSize &aSize);
Resizes the device.
|
|
virtual IMPORT_C void GetScanLine(TDes8 &aBuf, const TPoint &aStartPixel, TInt aLength, TDisplayMode iDispMode) const;
Copies a scanline into a buffer.
The function provides a concrete implementation of the pure virtual function CBitmapDevice::GetScanLine()
.
|
virtual IMPORT_C void GetPixel(TRgb &aColor, const TPoint &aPixel) const;
Gets the RGB colour of an individual pixel on a bitmapped graphics device.
The function provides a concrete implementation of the pure virtual function CBitmapDevice::GetPixel()
.
|
virtual IMPORT_C TInt HorizontalPixelsToTwips(TInt aPixels) const;
Converts a horizontal dimension of a device in pixels to a horizontal dimension in twips.
The function provides a concrete implementation of the pure virtual function MGraphicsDeviceMap::HorizontalPixelsToTwips()
.
|
|
virtual IMPORT_C TInt VerticalPixelsToTwips(TInt aPixels) const;
Converts a vertical dimension of a device in pixels to a vertical dimension in twips.
The function provides a concrete implementation of the pure virtual function MGraphicsDeviceMap::VerticalPixelsToTwips()
.
|
|
virtual IMPORT_C TInt HorizontalTwipsToPixels(TInt aTwips) const;
Converts a horizontal dimension of a device in twips to a horizontal dimension in pixels.
The function provides a concrete implementation of the pure virtual function MGraphicsDeviceMap::HorizontalTwipsToPixels()
.
|
|
virtual IMPORT_C TInt VerticalTwipsToPixels(TInt aTwips) const;
Converts a vertical dimension of a device in twips to a vertical dimension in pixels.
The function provides a concrete implementation of the pure virtual function MGraphicsDeviceMap::VerticalTwipsToPixels()
.
|
|
virtual IMPORT_C TSize SizeInTwips() const;
Gets the size of the device, in twips.
|
virtual IMPORT_C void PaletteAttributes(TBool &aModifiable, TInt &aNumEntries) const;
Gets the palette attributes of the device.
The function provides a concrete implementation of the pure virtual function CGraphicsDevice::PaletteAttributes()
.
|
virtual IMPORT_C void SetPalette(CPalette *aPalette);
Sets the device's palette to the specified palette.
The function provides a concrete implementation of the pure virtual function CGraphicsDevice::SetPalette()
.
|
virtual IMPORT_C TInt GetPalette(CPalette *&aPalette) const;
Gets the device's current palette.
The function provides a concrete implementation of the pure virtual function CGraphicsDevice::GetPalette()
.
|
|
virtual IMPORT_C void DrawingBegin(TBool aAlways=EFalse);
This method is called when you are about to start direct drawing to the bitmap memory. Calls to DrawingBegin()
must be paired with a subsequent call to DrawingEnd()
. Also, code must not leave between a DrawingBegin()
- DrawingEnd()
pair.
|
virtual IMPORT_C void DrawingEnd(TBool aAlways=EFalse);
This method is called when you have finished direct drawing to the bitmap memory. Calls to DrawingEnd()
must correspond to a prior call to DrawingBegin()
.
|
IMPORT_C TInt SwapWidthAndHeight();
The method swaps bitmap device's width and height. For example: if the size is (40, 20), the swapped size will be (20, 40). The device's content is not preserved. The method leaves CFbsBitmapDevice object in a consistent state - scaling settings will be set with their default values (the scaling is switched off), the device's dither origin will be set to (0,0), scaling origin to (0,0).
Note: If the device was scaled or its dither origin was set with a non-default value, it has to be rescaled again, respectivelly the dither origin has to be set again.
Note: All graphics contexts, already created by the device, should be re-activated calling CFbsBitGc::Activate()
.
Note: Do not call SwapWidthAndHeight()
between DrawingBegin()
and DrawingEnd()
calls!
|
private: virtual void SetBits();
Sets the bit level.