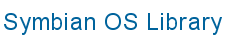
![]() |
![]() |
|
Location:
DAMDBMS.H
Link against: damodl.lib
class CDaModel : public CBase;
Database application engine.
The class:
wraps a store database (RDbStoreDatabase
) object: a database that stores data using a stream network within a store (a CStreamStore
). The class uses the standard database concepts, such as views, rows, indexes, and column sets: see the description of DBMS
for more information.
creates and manages a database application helper object (CDaUserDbDesc
), to simplify application access to the database
creates a SQL parameters object (CDaSqlParams
), which holds SQL queries for sorting, filtering, and searching the database
creates an undo-stack (CDaUndoStack
) to allow operations on the database to be undone.
CBase
- Base class for all classes to be instantiated on the heap
CDaModel
- Database application engine
Defined in CDaModel
:
AlterTable()
, AtRow()
, Attach()
, BuildViewL()
, BuildViewL()
, BuildViewSyncL()
, BuildViewSyncL()
, CloseDatabase()
, CloseView()
, ColSet()
, CreateDatabaseL()
, CreateDatabaseL()
, CreateIndex()
, CurrentRow()
, Database()
, DbRecordCount()
, DbStreamId()
, DefaultIndexName()
, DeleteRowL()
, EAtRow
, ENotAtRow
, ETriedBeforeFirst
, ETriedBeyondLast
, ExternalizeL()
, FirstL()
, GetL()
, GoToBookmarkL()
, HandleFirstFindL()
, HasOpenDatabase()
, HasRDbView()
, HowManySteps()
, InternalizeL()
, IsValid()
, LastL()
, MoveToRowL()
, NewDatabaseL()
, NewL()
, NewL()
, NextL()
, Observer()
, OpenDatabaseL()
, OpenDatabaseL()
, Panic()
, PreviousL()
, PutL()
, RecordStatsWin()
, RowPosState()
, RowPosState()
, RowSetCount()
, SetCurrentRow()
, SetDbColNo()
, SetDbRecordCountL()
, SetRecordStatsWin()
, SetRowSetCountL()
, SetYesNoArray()
, SqlParams()
, TState
, TableDef()
, UndoDeleteRowL()
, UndoStack()
, UpdateAllViewsL()
, UserDbDesc()
, View()
, View()
, ViewHasRows()
, YesNoArray()
, iDebugWin
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
static IMPORT_C CDaModel *NewL();
Allocates and constructs a new database application engine.
|
static IMPORT_C CDaModel *NewL(CFileStore &fileStore);
Allocates and constructs a new database application engine, initialising it from a store holding a store database.
The store database is found in the specified store, and opened.
|
|
inline void Attach(MDaObserver *aObserver);
Sets a database application engine observer.
|
IMPORT_C void NewDatabaseL(CStreamStore &aStore);
Creates and opens a new store database for the object.
The existing user column set is set for the new database.
|
IMPORT_C void ExternalizeL(RWriteStream &aStream) const;
Externalises the engine setttings to a stream.
|
IMPORT_C void InternalizeL(RReadStream &source);
Internalises the engine setttings from a stream.
|
IMPORT_C void OpenDatabaseL(CStreamStore &aStore);
Opens an existing store database.
If a database is already open, it is closed.
|
IMPORT_C void OpenDatabaseL(RDbStoreDatabase &aDatabase, CStreamStore &aStore);
Opens and returns an existing store database.
If a database is already open, it is closed.
|
IMPORT_C void CloseView();
Closes the open database view and destroys the associated column set.
IMPORT_C void NextL();
Moves to the next row in the view.
If the position is already on the last row, it remains there.
IMPORT_C void PreviousL();
Moves to the previous row in the view.
If the position is already on the first row, it remains there.
IMPORT_C void MoveToRowL(TInt aRow);
Moves to a specified row in the view.
If the requested row is greater than the last row, the position is set to the last row. If the requested row in less than the first row, the position is set to the first row.
|
inline TBool IsValid() const;
Tests if a valid position in a valid view is set.
|
inline const TPtrC &DefaultIndexName() const;
Gets the default index name.
|
inline TBool HasRDbView() const;
Tests if a valid view is set.
|
inline TBool ViewHasRows() const;
Tests if the view has any rows (i.e. is not empty).
|
inline TBool HasOpenDatabase() const;
Tests if the object has an open database.
|
inline TInt DbRecordCount() const;
Gets the stored record count variable (settable with SetDbRecordCountL()
).
|
inline TInt RowSetCount() const;
Gets the stored row set count variable (settable with SetRowSetCountL()
).
|
inline CDbColSet *ColSet() const;
Gets the column definition set for the view.
|
inline CDaUserDbDesc *UserDbDesc() const;
Gets the object's database application helper object.
|
inline TInt CurrentRow() const;
Gets the stored current row variable (settable with SetCurrentRow()
).
|
inline RDbDatabase &Database();
Gets the current database.
|
inline TStreamId DbStreamId() const;
Gets the ID of the stream in the store holding the database.
|
inline TState RowPosState() const;
Gets the result of the last row traversal operation.
|
inline CDaSqlParams *SqlParams() const;
Gets the SQL parameters object.
|
inline CDbColSet *TableDef() const;
Gets the column definition set for the database table.
|
inline MDaObserver *RecordStatsWin();
Gets the database observer set through SetRecordStatsWin()
.
|
inline const RDbView &View() const;
Gets the database view (const overload).
|
inline MDaObserver *Observer() const;
Gets the database observer set through Attach()
.
|
inline const CDaUndoStack &UndoStack() const;
Gets the object's undo stack.
|
inline const CDesCArray *YesNoArray() const;
Gets the string array set by SetYesNoArray()
.
The array is not used by the engine.
|
inline void SetCurrentRow(TInt aCurrentRow);
Sets the stored current row variable.
|
inline void RowPosState(TState aState);
Sets the result of the last row traversal operation.
|
inline void SetDbRecordCountL();
Sets the database record count variable to the number of rows in the current view.
inline void SetRowSetCountL();
Sets the database row set count variable to the number of rows in the current view.
inline void SetRecordStatsWin(MDaObserver *anObserver);
Sets the statistics window database engine observer.
The observer is called for every Put() operation.
|
inline void SetYesNoArray(const CDesCArray &aYesNoArray);
Sets the yes/no string array.
This is not used by the engine.
|
IMPORT_C TInt AlterTable(CDaUserColSet *aNewUserColSet);
Changes the structure of a specified table in the database to the specified column set.
! for now
|
|
IMPORT_C void CreateDatabaseL(CDaUserColSet *aUserColSet);
Creates a new database with a specified column set.
An already open database is closed.
|
IMPORT_C void CreateDatabaseL(CStreamStore *aStore, CDaUserColSet *aUserColSet);
Creates and opens a new database with a specified column set.
|
IMPORT_C TInt CreateIndex(const TDesC &anIndexName, CDbKey *aCDbKey);
Creates a new index with the specified key.
|
|
IMPORT_C TInt HowManySteps(RDbIncremental &anAlterTable, CDaUserColSet *aNewUserColSet);
Initiates an incremental table alteration operation on a database.
|
|
IMPORT_C void BuildViewL(const TDesC &aFindString);
Builds a database view asynchronously from the specified search string.
|
IMPORT_C void BuildViewL();
Builds a database view asynchronously from the last requested find string.
IMPORT_C void BuildViewSyncL();
Builds a database view synchronously from the last requested find string.
IMPORT_C void BuildViewSyncL(const TDesC &aFindString);
Builds a database view synchronously from the specified search string.
|
IMPORT_C void HandleFirstFindL();
Called by the SQL statement evaluator on finding the first result.
IMPORT_C void DeleteRowL();
Deletes the current row.
The delete operation is put on the undo stack.
It then moves to the next (or last) row in the view.
!! -1 if now rows in view
inline void GetL();
Gets the current row data for access using the view's column extractor functions.
IMPORT_C void UpdateAllViewsL(MDaObserver::TNotification=MDaObserver::EDefaultNotification);
Sends a specified event to the database engine observer.
!late
|
IMPORT_C void GoToBookmarkL(const TDbBookmark &aBookmark);
! Only one view is active at any one time
Moves to the specified database bookmark.
|
static IMPORT_C void Panic(TInt aReason);
Produces a "DaModel" category panic.
|
TState
Row traversal states.
|
MDaObserver * iDebugWin;