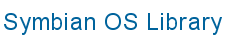
![]() |
![]() |
|
Location:
MmfAudioOutput.h
Link against: mmfaudiooutput.lib
class CMMFAudioOutput : public CBase, public MMMFAudioOutput, public MDevSoundObserver;
The interface into DevSound.
Abstract data sink class providing an interface into hardware sound output.
Uses CMMFDevSound
to access such output.
MDevSoundObserver
- An interface to a set of DevSound callback functions
MDataSink
- Abstract class representing a data sink
MMMFAudioOutput
- Interface class to allow dynamic linkage to
CBase
- Base class for all classes to be instantiated on the heap
CMMFAudioOutput
- The interface into DevSound
Defined in CMMFAudioOutput
:
BufferFilledL()
, BufferToBeEmptied()
, BufferToBeFilled()
, BytesPlayed()
, CanCreateSinkBuffer()
, ConstructSinkL()
, ConvertError()
, CreateSinkBufferL()
, DataType()
, DeviceMessage()
, EmptyBufferL()
, HWEmptyBufferL()
, InitializeComplete()
, NegotiateL()
, PlayError()
, RecordError()
, SendEventToClient()
, SetDataTypeL()
, SetSinkDataTypeCode()
, SetSinkPrioritySettings()
, SinkDataTypeCode()
, SinkPauseL()
, SinkPlayL()
, SinkPrimeL()
, SinkStopL()
, SinkThreadLogoff()
, SinkThreadLogon()
, SoundDevice()
, ToneFinished()
, ~CMMFAudioOutput()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from MDataSink
:
DataSinkType()
,
NewSinkL()
,
SinkCustomCommand()
Inherited from MMMFAudioOutput
:
NewAudioOutputL()
virtual TFourCC SinkDataTypeCode(TMediaId aMediaId);
Gets the sink's data type code.
Used by datapath MDataSource
/ MDataSink
for codec matching.
|
|
virtual void EmptyBufferL(CMMFBuffer *aBuffer, MDataSource *aSupplier, TMediaId aMediaId);
Sends audio to MMFDevsound.
|
virtual void BufferFilledL(CMMFBuffer *aBuffer);
Called by MDataSource
to pass back a full buffer to the sink.
Should never be called by a sink, as sinks empty buffers, not fill them.
|
virtual TBool CanCreateSinkBuffer();
Tests whether a sink buffer can be created.
The default implementation returns true.
|
virtual CMMFBuffer *CreateSinkBufferL(TMediaId aMediaId, TBool &aReference);
Creates a sink buffer.
Intended for asynchronous usage (buffers supplied by Devsound device)
|
|
virtual TInt SinkThreadLogon(MAsyncEventHandler &aEventHandler);
Logs on the sink's thread.
Thread specific initialization procedure for this device. Runs automatically on thread construction.
|
|
virtual void SinkThreadLogoff();
Logs off the sink thread.
Thread specific destruction procedure for this device. Runs automatically on thread destruction.
virtual void SinkPrimeL();
Prime's the sink.
This is a virtual function that each derived class must implement, but may be left blank for default behaviour.
Called by CMMFDataPath::PrimeL()
.
virtual void SinkPauseL();
Pauses the sink.
This is a virtual function that each derived class must implement, but may be left blank for default behaviour.
virtual void SinkPlayL();
Starts playing the sink.
This is a virtual function that each derived class must implement, but may be left blank for default behaviour.
virtual void SinkStopL();
Stops the sink.
This is a virtual function that each derived class must implement, but may be left blank for default behaviour.
virtual void SetSinkPrioritySettings(const TMMFPrioritySettings &aPrioritySettings);
Sets the sink's priority settings.
|
virtual void NegotiateL(MDataSource &aSource);
Negotiates with the source to set, for example, the sample rate and number of channels.
Called if the sink's setup depends on source.
|
virtual TInt BytesPlayed();
Returns the number of bytes played.
|
virtual void HWEmptyBufferL(CMMFBuffer *aBuffer, MDataSource *aSupplier);
Gets audio from hardware device abstracted MMFDevsound (not used).
|
virtual CMMFDevSound &SoundDevice();
Returns the sound device.
Accessor function exposing public CMMFDevsound methods.
|
virtual TInt SetSinkDataTypeCode(TFourCC aSinkFourCC, TMediaId aMediaId);
Sets the sink's data type code.
|
|
virtual void SetDataTypeL(TFourCC aAudioType);
This method should not be used - it is provided to maintain SC with v7.0s.
|
virtual TFourCC DataType() const;
This method should not be used - it is provided to maintain SC with v7.0s.
|
protected: virtual void ConstructSinkL(const TDesC8 &aInitData);
Overridable constuction specific to this datasource.
The default implementation does nothing.
|
private: virtual void InitializeComplete(TInt aError);
InitializeComplete MMFDevSoundObserver called when devsound initialisation completed.
|
private: virtual void ToneFinished(TInt aError);
ToneFinished MMFDevSoundObserver called when a tone has finished or interrupted
Should never get called.
|
private: virtual void BufferToBeFilled(CMMFBuffer *aBuffer);
BufferToBeFilled MMFDevSoundObserver. Called when buffer used up.
|
private: virtual void PlayError(TInt aError);
PlayError MMFDevSoundObserver.
Called when stopped due to error or EOF.
|
private: virtual void BufferToBeEmptied(CMMFBuffer *aBuffer);
BufferToBeEmptied MMFDevSoundObserver - should never get called.
|
private: virtual void RecordError(TInt aError);
RecordError MMFDevSoundObserver called when recording has halted.
Should never get called.
|
private: virtual void ConvertError(TInt aError);
ConvertError MMFDevSoundObserver.
Should never get called.
|
private: virtual void DeviceMessage(TUid aMessageType, const TDesC8 &aMsg);
DeviceMessage MMFDevSoundObserver
|
private: virtual void SendEventToClient(const TMMFEvent &);
Handles policy request completion event.
A derived class must provide an implementation to handle the policy request completion event.
CMMFDevSound
object calls this function when an attempt to acquire sound device is rejected by audio policy server.
|