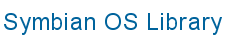
![]() |
![]() |
|
Location:
MmfFile.h
Link against: mmfstdsourceandsinkplugin.lib
class CMMFFile : public CMMFClip;
MultiMedia framework class. Represents a physical file.
This may be source file from which data is read or destination to which data is written.
Intended to be used by controller plugin developers for source and sink plugins.
MDataSink
- Abstract class representing a data sink
MDataSource
- Abstract class representing a data source
CBase
- Base class for all classes to be instantiated on the heap
CMMFClip
- Abstract class to represent a source or sink that contains a multimedia clip (i.e
CMMFFile
- MultiMedia framework class
Defined in CMMFFile
:
BufferEmptiedL()
, BufferFilledL()
, BytesFree()
, CanCreateSinkBuffer()
, CanCreateSourceBuffer()
, ConstructSinkL()
, ConstructSourceL()
, CreateSinkBufferL()
, CreateSourceBufferL()
, Data()
, Delete()
, ESinkMode
, ESourceMode
, EmptyBufferL()
, EvaluateIntent()
, ExecuteIntent()
, Extension()
, FileDrive()
, FileL()
, FileName()
, FilePath()
, FillBufferL()
, FullName()
, IsProtectedL()
, ReadBufferL()
, ReadBufferL()
, ReadBufferL()
, SetAgentProperty()
, SetSize()
, SinkDataTypeCode()
, SinkPrimeL()
, SinkStopL()
, SinkStopped()
, SinkThreadLogoff()
, SinkThreadLogon()
, Size()
, SourceDataTypeCode()
, SourcePauseL()
, SourcePrimeL()
, SourceStopL()
, SourceThreadLogoff()
, SourceThreadLogon()
, TMMFileMode
, UniqueId()
, WriteBufferL()
, WriteBufferL()
, WriteBufferL()
, ~CMMFFile()
Inherited from CBase
:
Extension_()
,
operator new()
Inherited from MDataSink
:
DataSinkType()
,
NegotiateL()
,
NewSinkL()
,
SetSinkDataTypeCode()
,
SetSinkPrioritySettings()
,
SinkCustomCommand()
,
SinkPauseL()
,
SinkPlayL()
Inherited from MDataSource
:
DataSourceType()
,
NegotiateSourceL()
,
NewSourceL()
,
SetSourceDataTypeCode()
,
SetSourcePrioritySettings()
,
SourceCustomCommand()
,
SourcePlayL()
,
SourceSampleConvert()
virtual TFourCC SourceDataTypeCode(TMediaId aMediaId);
Returns the data type as a fourCC code of CMMFFile as a data source.
|
|
virtual void FillBufferL(CMMFBuffer *aBuffer, MDataSink *aConsumer, TMediaId aMediaId);
Loads aBuffer from iFile.
The file must already be open for reading. File read is asynchronous. CReadRequest
is created to respond to completion.
|
virtual void BufferEmptiedL(CMMFBuffer *aBuffer);
CMMFFile as a source is always passive so this function is not supported.
|
virtual TBool CanCreateSourceBuffer();
Tests whether a source buffer can be created.
|
virtual CMMFBuffer *CreateSourceBufferL(TMediaId aMediaId, TBool &aReference);
Creates a source buffer.
|
|
virtual TInt SourceThreadLogon(MAsyncEventHandler &aEventHandler);
Source thread logon.
Shares fsSession between threads
|
|
virtual void SourcePrimeL();
Primes the source.
When used as a source, the file prime opens the file as read only.
virtual void SourceStopL();
Stops the file source. When stopping close the file. If the source is a file handle, the position is reset, but the file handle remains open.
virtual TFourCC SinkDataTypeCode(TMediaId aMediaId);
Returns the data type as a fourCC code of CMMFFile as a data sink.
|
|
virtual void EmptyBufferL(CMMFBuffer *aBuffer, MDataSource *aSupplier, TMediaId aMediaId);
Empties aBuffer into iFile. The file must be already open for writing.
|
virtual void BufferFilledL(CMMFBuffer *aBuffer);
CMMFFile as a sink is always passive so this function is not supported.
|
virtual TBool CanCreateSinkBuffer();
Tests whether a sink buffer can be created.
|
virtual CMMFBuffer *CreateSinkBufferL(TMediaId aMediaId, TBool &aReference);
Creates a sink buffer.
|
|
virtual TInt SinkThreadLogon(MAsyncEventHandler &aEventHandler);
Sink thread logon.
Shares fsSession between threads.
|
|
virtual void SinkPrimeL();
Primes the sink.
When used as a sink, the file prime opens the file for read/write access.
virtual void SinkStopL();
Stops the file sink.
When stopping close the file. When the file sink is a file handle, the position is reset, but the file handle remains open
virtual void ReadBufferL(TInt aLength, CMMFBuffer *aBuffer, TInt aPosition, MDataSink *aConsumer);
Loads aLength number of bytes into aBuffer from specified point in iFile.
|
virtual void WriteBufferL(TInt aLength, CMMFBuffer *aBuffer, TInt aPosition, MDataSource *aSupplier);
Empties aLength bytes from aBuffer into iFile at specified location.
|
|
virtual void ReadBufferL(CMMFBuffer *aBuffer, TInt aPosition, MDataSink *aConsumer);
Loads aBuffer from specified point in iFile.
The file must already be open for reading.
|
virtual void WriteBufferL(CMMFBuffer *aBuffer, TInt aPosition, MDataSource *aSupplier);
Empties aBuffer into iFile at the specified location.
|
|
virtual void ReadBufferL(CMMFBuffer *aBuffer, TInt aPosition);
Loads aBuffer from specified point in iFile. Note that this is a synchronous read.
|
virtual void WriteBufferL(CMMFBuffer *aBuffer, TInt aPosition);
Empties aBuffer into iFile at specified location. Note that this is a synchronous write.
|
virtual TInt64 BytesFree();
Gets the number of free bytes in the device's file system.
|
virtual TInt Size();
Returns the size of the file in bytes.
Note: This is not the maximum length.
|
virtual TInt Delete();
Deletes the file.
Closes the currently open file, then deletes it. If the file source is accessing a file handle, the file is truncated to 0 bytes instead.
|
virtual TInt SetSize(TInt aSize);
Sets the file size.
|
|
virtual const TDesC &FileName() const;
Returns the file name of the current file.
Note: This will give the wrong answer if the file is renamed!
|
virtual const TDesC &Extension() const;
Returns the extension of the current file.
Note: This will give the wrong answer if the file is renamed!
|
virtual const TDesC &FilePath() const;
Returns the path of the current file.
Note: This will give the wrong answer if the file is renamed!
|
virtual const TDesC &FileDrive() const;
Returns the drive on which the current file is located.
Note: This will give the wrong answer if the file is renamed!
|
virtual const TFileName FullName() const;
Returns the full name of the current file.
Note: This will give the wrong answer if the file is renamed!
|
virtual RFile &FileL();
Returns an RFile
handle to the current file.
If there is no current file, one is created. If the file exists then it is opened with read access if it is read only, write access otherwise. If the file does not exist then it is opened with write access.
|
|
virtual TBool SinkStopped();
Returns a boolean indicating if the sink has been stopped.
|
protected: virtual void ConstructSourceL(const TDesC8 &aInitData);
Perform source construction dependant on the source construction initialisation data aInitData.
|
protected: virtual void ConstructSinkL(const TDesC8 &aInitData);
Performs sink construction dependant on the sink construction initialisation data aInitData.
|
virtual TInt ExecuteIntent(ContentAccess::TIntent aIntent);
Evaluates and executes a given intent against the rights associated with the file.
The rights object is updated after calling this function.
|
|
virtual TInt EvaluateIntent(ContentAccess::TIntent aIntent) const;
Evaluates a given intent against the rights associated with the file.
The rights are not updated by this function call.
|
|
virtual TBool IsProtectedL() const;
Returns whether the file is protected.
|
virtual TInt SetAgentProperty(ContentAccess::TAgentProperty aProperty, TInt aValue);
|
|
virtual const TDesC &UniqueId() const;
Returns the uniqueID associated with this content. If no uniqueID has been provided, a null descriptor will be provided
|
virtual TInt Data(ContentAccess::CData *&aData);
Returns access to internal CData property
Returns: KErrNotReady if the file is not open/data object has not been created. KErrNotSupported if not supported (e.g. on data sink)
|
|
protected: TMMFileMode
Indicates for which purpose the object instance is being created
|