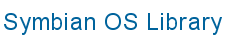
![]() |
![]() |
|
Location:
POP3SET.H
Link against: imcm.lib
class CImPop3Settings : public CImBaseEmailSettings;
Run-time configuration settings for POP3.
Messaging clients should use an instance of this class to specify and retrieve configuration settings that are used by the POP3 service when executing email operations.
Service settings such as the user name and password, whether to authenticate using APOP or plain text, the maximum size of an email to download, and the maximum number of messages to synchronise can be specified using this class. Storing and restoring from the message store is also supported.
To use this class to change a setting: 1) Set the current context to the POP3 service entry using CMsvStore
. 2) Create an instance of CImPop3Settings and put it on the cleanup stack. 3) Retrieve the existing settings by calling CImPop3Settings::RestoreL().
4) Change the inbox synchronisation limit setting by calling CImPop3Settings::SetInboxSynchronisationLimit()
. 5) Save the new settings by calling CImPop3Settings::StoreL(). 6) Pop and destroy the CImPop3Settings instance.
CBase
- Base class for all classes to be instantiated on the heap
CImBaseEmailSettings
- Parent class for
CImPop3Settings
- Run-time configuration settings for POP3
Defined in CImPop3Settings
:
AcknowledgeReceipts()
, Apop()
, AutoSendOnConnect()
, CImPop3Settings()
, CopyL()
, DeleteEmailsWhenDisconnecting()
, DisconnectedUserMode()
, GetMailOptions()
, InboxSynchronisationLimit()
, LoginName()
, MaxEmailSize()
, Password()
, PopulationLimit()
, Reset()
, SetAcknowledgeReceipts()
, SetApop()
, SetAutoSendOnConnect()
, SetDeleteEmailsWhenDisconnecting()
, SetDisconnectedUserMode()
, SetGetMailOptions()
, SetInboxSynchronisationLimit()
, SetLoginNameL()
, SetMaxEmailSize()
, SetPasswordL()
, SetPopulationLimitL()
, operator==()
, ~CImPop3Settings()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CImBaseEmailSettings
:
EBaseEmailSettingsClearFlag
,
EBaseEmailSettingsLastUsedFlag
,
EBaseEmailSettingsSSLWrappedSockets
,
EBaseEmailSettingsSecureSockets
,
Port()
,
SSLWrapper()
,
SecureSockets()
,
ServerAddress()
,
SetPort()
,
SetSSLWrapper()
,
SetSecureSockets()
,
SetServerAddressL()
,
TImBaseEmailSettings
,
iFlags
,
iPortNumber
IMPORT_C CImPop3Settings();
Allocates and creates a new CImPop3Settings object initialised to default values.
IMPORT_C const TPtrC8 LoginName() const;
Retrieves the configured login user name (mailbox) of the POP3 service.
|
IMPORT_C void SetLoginNameL(const TDesC8 &);
Configures the login user name (mailbox) for the POP3 service.
|
IMPORT_C const TPtrC8 Password() const;
Retrieves the configured plain text login password that will be sent to the POP3 server if APOP is not configured.
|
IMPORT_C void SetPasswordL(const TDesC8 &);
Configures the login password for the POP3 service. The plain text password will be sent during authentication with the POP3
server. It can also be used to store the shared secret when authenticating using APOP if SetApop()
is enabled.
|
IMPORT_C TBool AutoSendOnConnect() const;
Checks whether queued emails associated with the same internet access point as the POP3 service will be sent first before
logging onto the POP3 server. The internet access point for each email service (POP3, IMAP4, SMTP) is configured using CImIAPPreferences
.
|
IMPORT_C void SetAutoSendOnConnect(TBool aFlag);
Configures the POP3 service to send queued emails associated with the same internet access point to be sent first before logging onto the POP3 server.
The internet access point for each email service (POP3, IMAP4, SMTP) is configured using CImIAPPreferences
.
|
IMPORT_C TBool Apop() const;
Checks whether the POP3 service is configured to log on using plain text authentication or APOP encrypted authentication.
The default setting is EFalse.
|
IMPORT_C void SetApop(TBool aFlag);
Configures the POP3 service to authenticate using either a plain text password or an APOP encrypted digest.
If configured to use APOP authentication, the configured password set by the call to SetPasswordL()
is used to store the shared secret between the server and the POP3 service. The timestamp sent in the servers' hello banner
and the password are joined and encrypted into a digest before being sent using the MD5 algorithm.
The default setting is EFalse.
|
IMPORT_C TBool DisconnectedUserMode() const;
Checks if disconnected user mode is configured.
If disconnected user mode is enabled by calling SetDisconnectedUserMode()
, then the POP3 client MTM (CPop3ClientMtm
) will accept message operations, such as deleting messages from a server, while the user is offline. The operations are stored
and executed when the user connects again.
|
IMPORT_C void SetDisconnectedUserMode(TBool aFlag);
Enables the POP3 client MTM (CPop3ClientMtm
) to accept message operations while disconnected.
If disconnected user mode is enabled the POP3 client MTM will accept message operations such as deleting messages from a server, while the user is offline. The operations are stored and executed when the user connects again.
|
IMPORT_C TBool DeleteEmailsWhenDisconnecting() const;
Checks whether the caller has enabled this setting by calling SetDeleteEmailsWhenDisconnecting()
.
This flag is not used by the Messaging subsystem.
|
IMPORT_C void SetDeleteEmailsWhenDisconnecting(TBool aFlag);
Sets or resets a flag.
This flag is not used by the Messaging subsystem.
|
IMPORT_C TBool AcknowledgeReceipts() const;
Checks whether the caller has enabled this setting by calling SetAcknowledgeReceipts()
.
This flag is a convenience setting for Messaging client applications that may wish to store whether or not to send a receipt
email message to the sender when the email has been received. The Messaging subsystem does not use this setting, and has to
be explicitly commanded by the Messaging client application to send a receipt notification. Messaging applications can use
CImHeader
to check if a message has a return receipt address. Receipt notification messages can be created using CImEmailOperation
, and sent using the SMTP client MTM.
|
IMPORT_C void SetAcknowledgeReceipts(TBool aFlag);
Sets or resets a flag.
This flag is a convenience setting for Messaging client applications that may wish to store whether or not to send a receipt
email message to the sender when the email has been received. The Messaging subsystem does not use this setting, and has to
be explicitly commanded by the Messaging client application to send a receipt notification. Messaging applications can use
CImHeader
to check if a message has a return receipt address. Receipt notification messages can be created using CImEmailOperation
, and sent using the SMTP client MTM.
|
IMPORT_C TInt MaxEmailSize() const;
Returns the maximum message size in bytes to be downloaded. This value is set by a calling SetMaxEmailSize()
.
The Messaging subsystem does not use this setting. The default setting is KMaxInt.
Messaging applications can use CImPOP3GetMail
or CPop3ClientMtm
to specify a maximum email size to retrieve by passing a TImPop3GetMailInfo
parameter in method calls.
|
IMPORT_C void SetMaxEmailSize(const TInt aMaxEmailSize);
Sets the maximum message size in bytes to be downloaded.
The Messaging subsystem does not use this setting. The default setting is KMaxInt.
Messaging applications can use CImPOP3GetMail
or CPop3ClientMtm
to specify a maximum email size to retrieve by passing a TImPop3GetMailInfo
parameter in method calls.
|
IMPORT_C TPop3GetMailOptions GetMailOptions() const;
Retrieves an enumeration that specifies whether only the messages headers or the entire message will be downloaded when synchronising
with the POP3 server. This is specified by calling SetGetMailOptions()
.
|
IMPORT_C void SetGetMailOptions(TPop3GetMailOptions aGetMailOptions);
Specifies whether to download headers only, or entire messages when synchronising with the POP3 server.
|
IMPORT_C CImPop3Settings &CopyL(const CImPop3Settings &aCImPop3Settings);
Copies the configuration settings from another POP3 settings object into this object.
|
|
IMPORT_C TBool operator==(const CImPop3Settings &aCImPop3Settings) const;
Equality operator.
|
|
IMPORT_C TInt32 InboxSynchronisationLimit() const;
Returns whether or not the POP3 service will synchronise with all emails on the server after connecting, or the maximum number
of new messages that will be synchronised if there are new messages on the server. This setting is configured by calling SetInboxSynchronisationLimit()
.
The default setting is to synchronise all messages.
|
IMPORT_C void SetInboxSynchronisationLimit(const TInt32 aInboxSyncLimit);
Specifies whether or not the POP3 service will synchronise with all emails on the server after connecting, or the maximum number of new messages that will be synchronised if there are new messages on the server.
The default setting is to synchronise all messages.
|
IMPORT_C TInt32 PopulationLimit() const;
Retrieves the currently stored TOP population limit from settings. If this is set, it does not mean that this limit is applied but rather is just a convenient place to store the current limit
|
IMPORT_C void SetPopulationLimitL(const TInt32 aPopulationLimit);
Sets the currently stored TOP population limit to settings. If this is set, it does not mean that this limit is applied but rather is just a convenient place to store the current limit, the limit must be passed in the parameters of the command
|