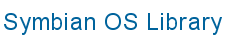
![]() |
![]() |
|
Location:
VCAL.H
Link against: vcal.lib
class CParserVCal : public CVersitParser;
A vCalendar parser.
Adds support for parsing vToDos and vEvents, and associated alarms (see CParserPropertyValueAlarm
) to the functionality of CVersitParser
.
Adds a constructor and overrides CVersitParser::InternalizeL()
for streams, ExternalizeL()
for streams, RecognizeToken()
, RecognizeEntityName()
and MakeEntityL()
.
The vCalendar data is read from or written to a stream or file, using the InternalizeL()
and ExternalizeL()
functions. Most users of this class will only need to use these functions.
Note: if you are sequentially creating and destroying multiple parsers, a major performance improvement may be achieved by
using thread local storage to store an instance of CVersitUnicodeUtils
which persists and can be used by all of the parsers.
See CVersitTlsData
for more information.
CBase
- Base class for all classes to be instantiated on the heap
CVersitParser
- A generic
CParserVCal
- A vCalendar parser
Defined in CParserVCal
:
ExternalizeL()
, InternalizeL()
, MakeEntityL()
, NewL()
, RecognizeEntityName()
, RecognizeToken()
, Reserved1()
, Reserved2()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
Inherited from CVersitParser
:
AddEntityL()
,
AddPropertyL()
,
AdjustAllPropertyDateTimesToMachineLocalL()
,
AnalysesEncodingCharset()
,
AppendBeginL()
,
AppendEndL()
,
ArrayOfEntities()
,
ArrayOfProperties()
,
BufPtr()
,
ClearSupportsVersion()
,
ConstructL()
,
ConvertAllPropertyDateTimesToMachineLocalL()
,
ConvertFromUnicodeToISOL()
,
ConvertToUnicodeFromISOL()
,
DecodeDateTimeL()
,
DecodePropertyValueL()
,
DecodeTimePeriodL()
,
DecodeTimeZoneL()
,
DefaultCharSet()
,
DefaultCharSetId()
,
DefaultEncoding()
,
DoAddPropertyL()
,
ECarriageReturn
,
ECharSetIdentified
,
EHTab
,
EImportSyncML
,
ELineFeed
,
ENoVersionProperty
,
ESpace
,
ESupportsVersion
,
EUseAutoDetection
,
EUseDefaultCharSetForAllProperties
,
EntityL()
,
EntityName()
,
FindFirstField()
,
FindRemainingField()
,
GetNumberL()
,
GetPropertyParamsLC()
,
IsValidLabel()
,
IsValidParameterValue()
,
LineCharSet()
,
LineCharSetId()
,
LineEncoding()
,
LineEncodingId()
,
LoadBinaryValuesFromFilesL()
,
MakePropertyL()
,
MakePropertyValueCDesCArrayL()
,
MakePropertyValueDaylightL()
,
MakePropertyValueL()
,
MakePropertyValueMultiDateTimeL()
,
Observer()
,
ParseBeginL()
,
ParseEndL()
,
ParseEntityL()
,
ParseParamL()
,
ParsePropertiesL()
,
ParsePropertyL()
,
PlugIn()
,
PropertyL()
,
ReadLineAndDecodeParamsLC()
,
ReadMultiLineValueL()
,
ResetAndDestroyArrayOfDateTimes()
,
ResetAndDestroyArrayOfEntities()
,
ResetAndDestroyArrayOfParams()
,
ResetAndDestroyArrayOfProperties()
,
RestoreLineCodingDetailsToDefault()
,
SaveBinaryValuesToFilesL()
,
SetAutoDetect()
,
SetCharacterConverter()
,
SetDefaultCharSet()
,
SetDefaultCharSetId()
,
SetDefaultEncoding()
,
SetEntityNameL()
,
SetFlags()
,
SetLineCharacterSet()
,
SetLineCharacterSetId()
,
SetLineCoding()
,
SetLineEncoding()
,
SetObserver()
,
SetPlugIn()
,
SetSupportsVersion()
,
SupportsVersion()
,
TCharCodes
,
TParserCodingDetails
,
TVersitParserFlags
,
UnicodeUtils()
,
Val()
,
iArrayOfEntities
,
iArrayOfProperties
,
iAutoDetectCharSets
,
iCurrentProperty
,
iCurrentPropertyCodingDetails
,
iDecodedValue
,
iDefaultCodingDetails
,
iDefaultVersion
,
iEntityName
,
iFlags
,
iLargeDataBuf
,
iLineReader
,
iObserver
,
iOwnedLineReader
,
iPlugIn
,
iStaticUtils
,
iWriteStream
static IMPORT_C CParserVCal *NewL();
Allocates and constructs a vCalendar parser.
|
virtual IMPORT_C void InternalizeL(RReadStream &aStream);
Internalises a vCalendar entity from a read stream.
The presence of this function means that the standard templated operator>>()
(defined in s32strm.h
) is available to internalise objects of this class.
|
virtual IMPORT_C void ExternalizeL(RWriteStream &aStream);
Externalises a vCalendar entity (and all sub-entities) to a write stream.
Sets the entity name to KVersitVarTokenVCALENDAR if it hasn't already been set.
Adds a version property to the start of the current entity's array of properties if the entity supports this. (If there isn't an array of properties then one is made).
The presence of this function means that the standard templated operator<<()
(defined in s32strm.h
) is available to externalise objects of this class.
|
protected: virtual IMPORT_C CVersitParser *MakeEntityL(TInt aEntityUid, HBufC *aEntityName);
|
|
virtual IMPORT_C TUid RecognizeToken(const TDesC8 &aToken) const;
Returns a UID that identifies a specified token's type.
For example, if aToken contains the property name DAYLIGHT the function returns KVersitPropertyDaylightUid. If the token is
not recognized as vCalendar-specific, the function calls CVersitParser::RecognizeToken()
, which recognizes generic Versit
tokens.
|
|
virtual IMPORT_C TInt RecognizeEntityName() const;
Tests the current value to see if it a vEvent or vTodo entity.
|
private: virtual IMPORT_C void Reserved2();