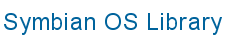
![]() |
![]() |
|
Location:
VERSIT.H
Link against: versit.lib
class CVersitParser : public CBase;
A generic Versit
parser.
Provides generic functions which implement behaviour common to both vCalendar and vCard parsers. For instance:
InternalizeL()
and ExternalizeL()
functions, for writing and reading data from a stream or file.
adding/retrieving properties and sub-entities to/from an existing entity.
encoding and character set conversion capabilities.
Although this is not an abstract class, in practice you would create and use objects of a derived class instead (CParserVCal
or CParserVCard
), as these provide additional functionality needed for parsing vCalendars and vCards.
Note: a flag used in the class constructor indicates whether the entity needs a version property. The version property will be inserted at the start of the array of properties for the entity, and specifies the version of the vCard/vCalendar specification used by the data of this particular vCard/vCalendar. The versions that are currently supported are vCard v2.1 and vCalendar v1.0.
A typical vCard looks like this:
BEGIN VCARD
VERSION 2.1 ...
END VCARD
Note: if you are sequentially creating and destroying multiple parsers, a major performance improvement may be achieved by
using thread local storage to store an instance of CVersitUnicodeUtils
which persists and can be used by all of the parsers.
See CVersitTlsData
for more details.
CBase
- Base class for all classes to be instantiated on the heap
CVersitParser
- A generic
Defined in CVersitParser
:
AddEntityL()
, AddPropertyL()
, AdjustAllPropertyDateTimesToMachineLocalL()
, AnalysesEncodingCharset()
, AppendBeginL()
, AppendEndL()
, ArrayOfEntities()
, ArrayOfProperties()
, BufPtr()
, CVersitParser()
, ClearSupportsVersion()
, ConstructL()
, ConvertAllPropertyDateTimesToMachineLocalL()
, ConvertFromUnicodeToISOL()
, ConvertToUnicodeFromISOL()
, DecodeDateTimeL()
, DecodePropertyValueL()
, DecodePropertyValueL()
, DecodeTimePeriodL()
, DecodeTimeZoneL()
, DefaultCharSet()
, DefaultCharSetId()
, DefaultEncoding()
, DoAddPropertyL()
, ECarriageReturn
, ECharSetIdentified
, EHTab
, EImportSyncML
, ELineFeed
, ENoVersionProperty
, ESpace
, ESupportsVersion
, EUseAutoDetection
, EUseDefaultCharSetForAllProperties
, EntityL()
, EntityName()
, ExternalizeL()
, ExternalizeL()
, FindFirstField()
, FindRemainingField()
, GetNumberL()
, GetPropertyParamsLC()
, InternalizeL()
, InternalizeL()
, InternalizeL()
, IsValidLabel()
, IsValidParameterValue()
, LineCharSet()
, LineCharSetId()
, LineEncoding()
, LineEncodingId()
, LoadBinaryValuesFromFilesL()
, LoadBinaryValuesFromFilesL()
, MakeEntityL()
, MakePropertyL()
, MakePropertyValueCDesCArrayL()
, MakePropertyValueDaylightL()
, MakePropertyValueL()
, MakePropertyValueMultiDateTimeL()
, Observer()
, ParseBeginL()
, ParseEndL()
, ParseEntityL()
, ParseParamL()
, ParsePropertiesL()
, ParsePropertyL()
, PlugIn()
, PropertyL()
, ReadLineAndDecodeParamsLC()
, ReadMultiLineValueL()
, RecognizeEntityName()
, RecognizeToken()
, Reserved1()
, Reserved2()
, ResetAndDestroyArrayOfDateTimes()
, ResetAndDestroyArrayOfEntities()
, ResetAndDestroyArrayOfParams()
, ResetAndDestroyArrayOfProperties()
, RestoreLineCodingDetailsToDefault()
, SaveBinaryValuesToFilesL()
, SaveBinaryValuesToFilesL()
, SetAutoDetect()
, SetCharacterConverter()
, SetDefaultCharSet()
, SetDefaultCharSetId()
, SetDefaultEncoding()
, SetEntityNameL()
, SetFlags()
, SetLineCharacterSet()
, SetLineCharacterSetId()
, SetLineCoding()
, SetLineEncoding()
, SetLineEncoding()
, SetObserver()
, SetPlugIn()
, SetSupportsVersion()
, SupportsVersion()
, TCharCodes
, TParserCodingDetails
, TVersitParserFlags
, UnicodeUtils()
, Val()
, iArrayOfEntities
, iArrayOfProperties
, iAutoDetectCharSets
, iCurrentProperty
, iCurrentPropertyCodingDetails
, iDecodedValue
, iDefaultCodingDetails
, iDefaultVersion
, iEntityName
, iFlags
, iLargeDataBuf
, iLineReader
, iObserver
, iOwnedLineReader
, iPlugIn
, iStaticUtils
, iWriteStream
, ~CVersitParser()
Inherited from CBase
:
Delete()
,
Extension_()
,
operator new()
IMPORT_C CVersitParser(TUint aFlags);
The first phase constructor for a Versit
parser.
Sets the default encoding to Versit::ENoEncoding
and the default character set to Versit::EUSAsciiCharSet
.
Note: this function is called by the CParserVCal
and CParserVCard
constructors. It should only be called directly by a user if creating a new parser
|
IMPORT_C void ConstructL();
The second phase constructor for a Versit
parser.
Stores a pointer to a CVersitTlsData
(thread local storage data class). This is used to allow an instance of CVersitUnicodeUtils
to be shared by all co-existing parsers, which provides a major performance improvement.
Called by the CParserVCal
and CParserVCard
constructors.
Should only be called directly by a user if creating a new parser.
IMPORT_C ~CVersitParser();
Frees all resources owned by the object, prior to its destruction.
IMPORT_C void InternalizeL(RFile &aInputFile, TInt &aBytesThroughFile);
Internalises a versit entity (vCard or vCalendar) and all of its sub-entities and properties from a file.
Stores all date/time in same format as received
|
|
virtual IMPORT_C void InternalizeL(RReadStream &aStream);
Internalises a Versit
entity (vCard or vCalendar) contained in the incoming stream and parses it, breaking it down into its constituent sub-entities
(e.g. to-dos, events, and nested vCards) and properties.
The presence of this function means that the standard templated operator>>()
(defined in s32strm.h
) is available to internalise objects of this class.
This virtual function serves as a base function for derived classes to internalise an entity.
|
virtual IMPORT_C void InternalizeL(HBufC *aEntityName, CLineReader *aLineReader);
Internalises a vCalendar or vCard sub-entity.
(Assumes "BEGIN : <EntityName> CRLF" has been parsed).
This virtual function serves as a base function for derived classes to parse a sub-entity.
|
IMPORT_C void ExternalizeL(RFile &aOutputFile);
Externalises a Versit
entity (vCard or vCalendar) and all of its sub-entities and properties to a file.
Adds a version property to the start of the current entity's array of properties if the entity supports this.
This is a thin layer over the CVersitParser::ExternalizeL(RWriteStream& aStream)
function to enable a versit entity to be externalised into an RFile
.
|
virtual IMPORT_C void ExternalizeL(RWriteStream &aStream);
Externalises a Versit
entity (vCard or vCalendar) and all of its sub-entities and properties to a write stream.
Adds a version property to the start of the current entity's array of properties if the entity supports this. (If there isn't an array of properties then one is made).
The presence of this function means that the standard templated operator<<()
(defined in s32strm.h
) is available to externalise objects of this class.
This virtual function serves as a base function for derived classes to externalise an entity.
|
IMPORT_C void AddEntityL(CVersitParser *aEntity);
Adds a sub-entity (e.g. a to-do, event or a nested vCard) to the current entity.
Sets the default encoding and character set to the default ones of the current Versit
parser, then adds the entity to the array of entities owned by the parser.
Note: This function may be used when building up a Versit
parser object from a client application.
Called by ParseEntityL()
.
|
IMPORT_C void AddPropertyL(CParserProperty *aProperty, TBool aInternalizing=EFalse);
Appends a property to the current entity's array of properties.
This function may be used when building up a Versit
parser object from a client application. It is not called internally.
|
IMPORT_C CArrayPtr< CVersitParser > *EntityL(const TDesC &aEntityName, TBool aTakeOwnership=ETrue);
Gets all sub-entities in the current entity, whose name matches the name specified.
|
|
IMPORT_C CArrayPtr< CVersitParser > *ArrayOfEntities(TBool aTakeOwnership=ETrue);
Gets the current entity's array of sub-entities.
Note: the current entity may be a top level entity, or may itself be a sub-entity.
|
|
IMPORT_C CArrayPtr< CParserProperty > *PropertyL(const TDesC8 &aPropertyName, const TUid &aPropertyUid, TBool aTakeOwnership=ETrue)
const;
Gets all properties in the current entity's array of properties whose name and value match the name and value specified.
|
|
IMPORT_C CArrayPtr< CParserProperty > *ArrayOfProperties(TBool aTakeOwnership=ETrue);
Gets the current entity's array of properties.
|
|
virtual IMPORT_C void ConvertAllPropertyDateTimesToMachineLocalL(const TTimeIntervalSeconds &aIncrement, const CVersitDaylight
*aDaylight);
Converts all date/time property values contained in the current entity's array of properties into machine local values.
This conversion is needed because of differences in universal and local times due to time zones and daylight savings (seasonal time shifts).
First, all of the date/times are converted to universal time, if they are not already:
If daylight saving is in effect then the daylight savings rule (held in the aDaylight parameter) will be used to compensate for differences between universal and local times due to both time zones and the daylight saving. Otherwise, the aIncrement parameter is used to compensate for any difference due to time zones alone.
Then, these universal time values are converted into machine local times by adding the universal time offset for the machine's locale.
|
static IMPORT_C TBool IsValidParameterValue(TInt &aPos, const TDesC &aParamValue);
Tests whether a property parameter name or value is valid.
If the string aParamValue contains any punctuation characters, the string is invalid. Otherwise, it is valid. Punctuation characters are defined as any of the following:-
[] (left or right square bracket)
= (equals sign)
: (colon)
; (semi colon)
. (dot)
, (comma)
|
|
IMPORT_C void SetEntityNameL(const TDesC &aEntityName);
Sets the name for the current entity to one of: VCARD, VCALENDAR, VEVENT or VTODO.
|
|
IMPORT_C TPtrC EntityName() const;
Gets the current entity's name.
If no name has been set, returns an empty descriptor.
|
static IMPORT_C TBool IsValidLabel(const TDesC &aLabel, TInt &aPos);
Tests whether a property name is valid.
The property name is invalid if it contains any of the following characters:-
[] (left or right square bracket)
= (equals sign)
: (colon)
. (dot)
, (comma)
|
|
static IMPORT_C TInt Val(const TDesC &aString, TInt &aNumber);
Converts a string into an integer, for example, it converts "438" to 438.
|
|
IMPORT_C void SetCharacterConverter(Versit::TEncodingAndCharset &encodingAndCharset);
Sets a character converter suitable for converting strings between Unicode and the specified character set.
The function finds a suitable converter for the character set specified in aEncodingAndCharset.iCharSetId, if one is available, and assigns it to aEncodingAndCharset.iConverter.
If there is no converter available for the specified character set then iConverter is set to NULL.
This function is only of use if executing a major change to the externalisation behaviour, particularly if overriding CParserProperty::ExternalizeL()
- the function from which this function is called.
|
IMPORT_C Versit::TVersitEncoding DefaultEncoding() const;
Gets the parser's default encoding.
This value is initialised on construction, to ENoEncoding.
|
IMPORT_C void SetDefaultEncoding(const Versit::TVersitEncoding aEncoding);
Sets the parser's default encoding to aEncoding.
|
|
IMPORT_C Versit::TVersitCharSet DefaultCharSet() const;
Gets the default character set or transformation format. This may be used to represent property values in Versit
objects.
|
IMPORT_C TUint DefaultCharSetId() const;
Gets the default character set or transformation format.
This may be used to represent property values in Versit
objects.
|
IMPORT_C void SetDefaultCharSet(const Versit::TVersitCharSet aCharSet);
Sets the default character set or transformation format.
This may be used to represent property values in Versit
objects.
|
IMPORT_C void SetDefaultCharSetId(TUint aCharSetId);
Sets the default character set or transformation format.
This may be used to represent property values in Versit
objects.
|
IMPORT_C void SetAutoDetect(TBool aOn, const CArrayFix< CCnvCharacterSetConverter::SCharacterSet > *aAutoDetectCharSets=0);
Turns auto detection of character sets on or off.
If a property does not specify a character set, then it is possible to guess its character set. This function turns this behaviour on or off. When the behaviour is on, it also lets the caller specify a restricted set of character sets to be considered.
Auto-detection of character sets is used (if auto detection is on) when converting a property value to Unicode while internalising a stream.
|
inline void SetObserver(MVersitObserver *aObserver);
Sets the Versit
observer.
|
inline MVersitObserver *Observer();
Gets a pointer to the Versit
observer.
|
inline void SetPlugIn(MVersitPlugIn *aPlugIn);
Sets the Versit
plug-in.
If there is one, the Versit
plug-in needs to be set before any properties are added to the parser. This is done for you when internalising (using InternalizeL()
) or adding properties (using AddPropertyL()
).
|
inline MVersitPlugIn *PlugIn();
Gets a pointer to the Versit
plug-in.
|
IMPORT_C TInt LoadBinaryValuesFromFilesL();
Loads all files represented by URI property values and sets the binary data contained in the files to be the property values instead of the URIs.
For every property in the parser, if its value is a URI containing file:// followed by a path and filename, then the file is opened and the binary data it contains is read into a CParserPropertyValueBinary
object. This replaces the URI as the property value. The function also operates on any agents in the vCard that contain URI
property values.
The function creates its own file server session, which is needed to open the files. It leaves if there is a problem opening any of the files.
|
IMPORT_C TInt LoadBinaryValuesFromFilesL(RFs &aFileSession);
Loads all files represented by URI property values and sets the binary data contained in the files to be the property values instead of the URIs.
For every property in the parser, if its value is a URI containing file:// followed by a path and filename, then the file is opened and the binary data it contains is read into a CParserPropertyValueBinary
object. This replaces the URI as the property value. The function also operates on any agents in the vCard that contain URI
property values.
The function uses the file server session supplied, which is needed to open the files. It leaves if there is a problem opening any of the files.
|
|
IMPORT_C TInt SaveBinaryValuesToFilesL(TInt aSizeThreshold, const TDesC &aPath);
Saves all binary property values larger than a specified threshold to files, and sets each property value to be a URI representing the file, rather than the binary data iself.
The files are created in the folder identified by aPath, and are assigned unique filenames that consist of the property name and some random numbers.
Each new URI property value is prefixed with file:// and contains the path and filename of the file created.
If a vCard contains any agent property values and if they contain binary data whose size exceeds the threshold, these property values are replaced with URI property values.
The function sets up its own file server session, which is needed to create the files. It leaves if there is a problem creating any of the files.
|
|
IMPORT_C TInt SaveBinaryValuesToFilesL(TInt aSizeThreshold, const TDesC &aPath, RFs &aFileSession);
Saves all binary property values larger than a specified threshold to files, and sets each property value to be a URI representing the file rather than the binary data iself.
The files are created in the folder identified by aPath, and are assigned unique filenames that consist of the property name and some random numbers.
Each new URI property value is prefixed with file:// and contains the path and filename of the file created.
If a vCard contains any agent property values and if they contain binary data whose size exceeds the threshold, these property values are replaced with URI property values.
The function uses the file server session supplied, which is needed to create the files. It leaves if there is a problem creating any of the files.
|
|
static IMPORT_C TInt ConvertFromUnicodeToISOL(TDes8 &aIso, const TDesC16 &aUnicode, CCnvCharacterSetConverter *aConverter);
Converts text in the Unicode character set (UCS-2) into a non-unicode (International Standards Organisation) character set.
Which ISO character set the string is converted to is determined by the value of the character set identifier in the aConverter parameter.
|
|
IMPORT_C TVersitDateTime *DecodeDateTimeL(TDes &aToken) const;
|
|
protected: IMPORT_C TInt ConvertToUnicodeFromISOL(TDes16 &aUnicode, const TDesC8 &aIso, TUint aCharacterSet);
Converts text in a non-Unicode character set into Unicode (UCS-2).
|
|
protected: IMPORT_C CArrayPtr< CParserParam > *ReadLineAndDecodeParamsLC(TInt &aValueStart, TInt &aNameLen);
|
|
protected: IMPORT_C void MakePropertyL(TPtr8 &aPropName, TInt aValueStart);
Ignore junk lines between vCal entities. Skip readMultiLine within ParseBegin.
|
protected: IMPORT_C CArrayPtr< CParserParam > *GetPropertyParamsLC(TPtr8 aParams);
|
|
protected: IMPORT_C void ParseParamL(CArrayPtr< CParserParam > *aArray, TPtr8 aParam);
|
protected: IMPORT_C void AnalysesEncodingCharset(CArrayPtr< CParserParam > *aArrayOfParams);
|
protected: IMPORT_C void ReadMultiLineValueL(TPtr8 &aValue, TInt aValueStart, TBool aBinaryData);
|
protected: IMPORT_C void DoAddPropertyL(CParserProperty *aProperty);
|
protected: virtual IMPORT_C CVersitParser *MakeEntityL(TInt aEntityUid, HBufC *aEntityName);
|
|
protected: virtual IMPORT_C CParserPropertyValue *MakePropertyValueL(const TUid &aPropertyUid, HBufC16 *&aValue);
|
|
protected: IMPORT_C HBufC *DecodePropertyValueL(const TDesC8 &aValue);
|
|
protected: IMPORT_C void DecodePropertyValueL(const TDesC8 &aValue, const TUid &aEncodingUid);
|
protected: IMPORT_C CDesCArray *MakePropertyValueCDesCArrayL(TPtr16 aStringValue);
Parses a compound property value string.
The sub-values found are appended to an array, after removal of escape characters. The array is returned, and ownership is transferred to the caller.
|
|
protected: IMPORT_C CArrayPtr< TVersitDateTime > *MakePropertyValueMultiDateTimeL(TPtr16 aDateTimeGroup);
|
|
protected: IMPORT_C CVersitDaylight *MakePropertyValueDaylightL(TPtr16 aDaylightValue);
|
|
protected: IMPORT_C TBool FindFirstField(TPtr16 &aField, TPtr16 &aRemaining, TBool aTrimSpace=ETrue);
|
|
protected: IMPORT_C void FindRemainingField(TPtr16 &aField, TPtr16 &aRemaining);
Sets up a pointer to the remaining field. Sets the original remaining field pointers length to 0.
|
protected: IMPORT_C TTimeIntervalSeconds DecodeTimeZoneL(const TDesC &aToken) const;
|
|
protected: IMPORT_C TTime *DecodeTimePeriodL(const TDesC &aToken) const;
|
|
protected: IMPORT_C TInt GetNumberL(const TDesC &aToken, TInt &aNumChars) const;
|
|
virtual IMPORT_C TUid RecognizeToken(const TDesC8 &aToken) const;
Returns a UID for the specified token.
This function only recognizes generic Versit
tokens. For example, if aToken contains the property name KVersitTokenBEGIN, the function returns KVersitTokenBeginUid. More
specific recognition should occur in derived classes which implement this function, using this as the base recogniser.
|
|
virtual IMPORT_C TInt RecognizeEntityName() const;
Tests the current entity to see if it is a vEvent or vTodo.
This function is virtual. Actual testing only occurs in derived classes which implement this function.
|
static IMPORT_C void ResetAndDestroyArrayOfParams(TAny *aObject);
Destroys an array of parameters.
|
static IMPORT_C void ResetAndDestroyArrayOfProperties(TAny *aObject);
Destroys an array of properties.
|
static IMPORT_C void ResetAndDestroyArrayOfEntities(TAny *aObject);
Destroys an array of entities.
|
static IMPORT_C void ResetAndDestroyArrayOfDateTimes(TAny *aObject);
Destroys an array of Versit
dates and times.
|
protected: IMPORT_C void SetLineEncoding(Versit::TVersitEncoding aLineEncoding);
|
protected: IMPORT_C void SetLineEncoding(TUint aVersitEncodingUid);
|
protected: IMPORT_C void SetLineCharacterSet(Versit::TVersitCharSet aLineCharSet);
|
protected: IMPORT_C void SetLineCharacterSetId(TUint aLineCharSetId);
|
protected: IMPORT_C void SetLineCoding(Versit::TVersitCharSet aLineCharSet, Versit::TVersitEncoding aLineEncoding);
|
protected: IMPORT_C Versit::TVersitEncoding LineEncoding() const;
|
protected: IMPORT_C Versit::TVersitCharSet LineCharSet() const;
|
protected: inline CVersitUnicodeUtils &UnicodeUtils();
|
protected: struct TParserCodingDetails;
Defined in CVersitParser::TParserCodingDetails
:
iCharSet
, iCharSetUid
, iEncoding
, iEncodingUid
iEncoding
Versit::TVersitEncoding iEncoding;
iEncodingUid
TUint iEncodingUid;
iCharSet
Versit::TVersitCharSet iCharSet;
iCharSetUid
TUint iCharSetUid;
TCharCodes
White space character codes: used while analysing the syntax of the received data and while externalising data.
|
TVersitParserFlags
Flags that can be specified on construction.
|
protected: const CArrayFix< CCnvCharacterSetConverter::SCharacterSet > * iAutoDetectCharSets;
protected: MVersitPlugIn * iPlugIn;