csBox3 Class Reference
[Geometry utilities]
A bounding box in 3D space.
More...
#include <csgeom/box.h>
Inheritance diagram for csBox3:
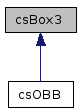
Public Member Functions | |
void | AddBoundingBox (const csBox3 &box) |
Add another bounding box into this one, combining the two of them. | |
void | AddBoundingVertex (const csVector3 &v) |
Add a new vertex and recalculate the bounding box. | |
void | AddBoundingVertex (float x, float y, float z) |
Add a new vertex and recalculate the bounding box. | |
void | AddBoundingVertexSmart (const csVector3 &v) |
Add a new vertex and recalculate the bounding box. | |
void | AddBoundingVertexSmart (float x, float y, float z) |
Add a new vertex and recalculate the bounding box. | |
bool | AddBoundingVertexSmartTest (const csVector3 &v) |
Add a new vertex and recalculate the bounding box. | |
bool | AddBoundingVertexSmartTest (float x, float y, float z) |
Add a new vertex and recalculate the bounding box. | |
bool | AddBoundingVertexTest (const csVector3 &v) |
Add a new vertex and recalculate the bounding box. | |
bool | AddBoundingVertexTest (float x, float y, float z) |
Add a new vertex and recalculate the bounding box. | |
int | Adjacent (const csBox3 &other, float epsilon=SMALL_EPSILON) const |
Test if this box is adjacent to the other one. | |
bool | AdjacentX (const csBox3 &other, float epsilon=SMALL_EPSILON) const |
Test if this box is adjacent to the other on the X side. | |
bool | AdjacentY (const csBox3 &other, float epsilon=SMALL_EPSILON) const |
Test if this box is adjacent to the other on the Y side. | |
bool | AdjacentZ (const csBox3 &other, float epsilon=SMALL_EPSILON) const |
Test if this box is adjacent to the other on the Z side. | |
float | Area () const |
Compute area of box. | |
bool | Between (const csBox3 &box1, const csBox3 &box2) const |
Test if this box is between two others. | |
int | CalculatePointSegment (const csVector3 &pos) const |
Assume that 3D space is divided into 27 areas. | |
bool | Contains (const csBox3 &box) const |
Test if this box contains the other box. | |
csBox3 (float x1, float y1, float z1, float x2, float y2, float z2) | |
Initialize this box with the given values. | |
csBox3 (const csVector3 &v1, const csVector3 &v2) | |
Initialize this box with two points. | |
csBox3 (const csVector3 &v) | |
Initialize this box with one point. | |
csBox3 () | |
Initialize this box to empty. | |
csString | Description () const |
Return a textual representation of the box in the form "(minx,miny,minz)-(maxx,maxy,maxz)". | |
bool | Empty () const |
Test if this box is empty. | |
void | GetAxisPlane (int side, int &axis, float &where) const |
Get axis aligned plane information from a side of this box. | |
csVector3 | GetCenter () const |
Get the center of this box. | |
void | GetConvexOutline (const csVector3 &pos, csVector3 *array, int &num_array, bool bVisible=false) const |
Get a convex outline (not a polygon unless projected to 2D) for for this box as seen from the given position. | |
csVector3 | GetCorner (int corner) const |
Return every corner of this bounding box from 0 to 7. | |
void | GetEdge (int edge, csSegment3 &e) const |
Return every edge (segment) of this bounding box from 0 to 23 (use one of the CS_BOX_EDGE_Xyz_xyz etc. | |
csSegment3 | GetEdge (int edge) const |
Return every edge (segment) of this bounding box from 0 to 23 (use one of the CS_BOX_EDGE_Xyz_xyz etc. | |
void | GetEdgeInfo (int edge, int &v1, int &v2, int &fleft, int &fright) const |
Given an edge index (CS_BOX_EDGE_Xyz_xyz etc. | |
const uint8 * | GetFaceEdges (int face) const |
Given a face index (CS_BOX_SIDE_x etc. | |
csBox2 | GetSide (int side) const |
Get a side of this box as a 2D box. | |
csVector3 | GetSize () const |
Get the size of the box. | |
int | GetVisibleSides (const csVector3 &pos, int *visible_sides) const |
Fill the array (which should be three long at least) with all visible sides (CS_BOX_SIDE_x etc. | |
bool | In (const csVector3 &v) const |
Test if the given coordinate is in this box. | |
bool | In (float x, float y, float z) const |
Test if the given coordinate is in this box. | |
void | ManhattanDistance (const csBox3 &other, csVector3 &dist) const |
Calculate the minimum manhattan distance between this box and another one. | |
const csVector3 & | Max () const |
Get the 3d vector of maximum (x, y, z) values. | |
float | Max (int idx) const |
Get Max component for 0 (x), 1 (y), or 2 (z). | |
float | MaxX () const |
Get the maximum X value of the box. | |
float | MaxY () const |
Get the maximum Y value of the box. | |
float | MaxZ () const |
Get the maximum Z value of the box. | |
const csVector3 & | Min () const |
Get the 3d vector of minimum (x, y, z) values. | |
float | Min (int idx) const |
Get Min component for 0 (x), 1 (y), or 2 (z). | |
float | MinX () const |
Get the minimum X value of the box. | |
float | MinY () const |
Get the minimum Y value of the box. | |
float | MinZ () const |
Get the minimum Z value of the box. | |
csBox3 & | operator *= (const csBox3 &box) |
Compute the intersection of two bounding boxes. | |
csBox3 & | operator+= (const csVector3 &point) |
Compute the union of a point with this bounding box. | |
csBox3 & | operator+= (const csBox3 &box) |
Compute the union of two bounding boxes. | |
bool | Overlap (const csBox3 &box) const |
Test if this box overlaps with the given box. | |
bool | ProjectBox (const csTransform &trans, float fov, float sx, float sy, csBox2 &sbox, float &min_z, float &max_z) const |
Project this box to a 2D bounding box given the view point transformation and also the field-of-view and shift values (for perspective projection). | |
bool | ProjectBoxAndOutline (const csTransform &trans, float fov, float sx, float sy, csBox2 &sbox, csPoly2D &poly, float &min_z, float &max_z) const |
Project this box to the 2D outline given the view point transformation and also the field-of-view and shift values (for perspective correction). | |
bool | ProjectOutline (const csVector3 &origin, int axis, float where, csPoly2D &poly) const |
Project this box to the 2D outline given the origin and an axis aligned plane. | |
bool | ProjectOutline (const csVector3 &origin, int axis, float where, csArray< csVector2 > &poly) const |
Project this box to the 2D outline given the origin and an axis aligned plane. | |
bool | ProjectOutline (const csTransform &trans, float fov, float sx, float sy, csPoly2D &poly, float &min_z, float &max_z) const |
Project this box to the 2D outline given the view point transformation and also the field-of-view and shift values (for perspective correction). | |
void | Set (float x1, float y1, float z1, float x2, float y2, float z2) |
Sets the bounds of the box with the given values. | |
void | Set (const csVector3 &bmin, const csVector3 &bmax) |
Sets the bounds of the box with the given values. | |
void | SetCenter (const csVector3 &c) |
Set the center of this box. | |
void | SetMax (int idx, float val) |
Set Max component for 0 (x), 1 (y), or 2 (z). | |
void | SetMin (int idx, float val) |
Set Min component for 0 (x), 1 (y), or 2 (z). | |
void | SetSize (const csVector3 &s) |
Set the size of the box but keep the center intact. | |
void | Split (int axis, float where, csBox3 &bl, csBox3 &br) const |
Split this box along an axis and construct two new boxes. | |
float | SquaredOriginDist () const |
Calculate the squared distance between (0,0,0) and the box This routine is extremely efficient. | |
float | SquaredOriginMaxDist () const |
Calculate the squared distance between (0,0,0) and the point on the box which is furthest away from (0,0,0). | |
float | SquaredPosDist (const csVector3 &pos) const |
Calculate the squared distance between pos and the box This routine is extremely efficient. | |
float | SquaredPosMaxDist (const csVector3 &pos) const |
Calculate the squared distance between pos and the point on the box which is furthest away from pos. | |
void | StartBoundingBox (const csVector3 &v) |
Initialize this box to one vertex. | |
void | StartBoundingBox () |
Initialize this box to empty. | |
bool | TestIntersect (const csBox3 &box) const |
Test if the two boxes have an intersection. | |
int | TestSplit (int axis, float where) const |
Test if this box intersects with the given axis aligned plane. | |
float | Volume () const |
Compute volume of box. | |
Static Public Member Functions | |
static int | OtherSide (int side) |
Static function to get the 'other' side (i.e. | |
Protected Types | |
typedef uint8 | bFace [4] |
Indices of four clock-wise edges (0..23). | |
Protected Attributes | |
csVector3 | maxbox |
The bottom-right. | |
csVector3 | minbox |
The top-left of this bounding box. | |
Static Protected Attributes | |
static const bEdge | edges [24] |
Index by edge number. | |
static const bFace | faces [6] |
Index by CS_BOX_SIDE_? number. | |
static const Outline | outlines [27] |
Outline lookup table. | |
Friends | |
csBox3 | operator * (const csBox3 &box1, const csBox3 &box2) |
Compute the intersection of two bounding boxes. | |
bool | operator!= (const csBox3 &box1, const csBox3 &box2) |
Tests if two bounding boxes are unequal. | |
csBox3 | operator+ (const csBox3 &box, const csVector3 &point) |
Compute the union of a bounding box and a point. | |
csBox3 | operator+ (const csBox3 &box1, const csBox3 &box2) |
Compute the union of two bounding boxes. | |
bool | operator< (const csVector3 &point, const csBox3 &box) |
Tests if a point is contained in a box. | |
bool | operator< (const csBox3 &box1, const csBox3 &box2) |
Tests if box1 is a subset of box2. | |
bool | operator== (const csBox3 &box1, const csBox3 &box2) |
Tests if two bounding boxes are equal. | |
bool | operator> (const csBox3 &box1, const csBox3 &box2) |
Tests if box1 is a superset of box2. | |
Classes | |
struct | bEdge |
struct | Outline |
This table also contains an array of sides visible from that region. More... |
Detailed Description
A bounding box in 3D space.In order to operate correctly, this bounding box assumes that all values entered or compared against lie within the range (-CS_BOUNDINGBOX_MAXVALUE, CS_BOUNDINGBOX_MAXVALUE). It is not recommended to use points outside of this range.
Definition at line 595 of file box.h.
Member Typedef Documentation
typedef uint8 csBox3::bFace[4] [protected] |
Constructor & Destructor Documentation
csBox3::csBox3 | ( | const csVector3 & | v | ) | [inline] |
csBox3::csBox3 | ( | float | x1, | |
float | y1, | |||
float | z1, | |||
float | x2, | |||
float | y2, | |||
float | z2 | |||
) | [inline] |
Member Function Documentation
void csBox3::AddBoundingBox | ( | const csBox3 & | box | ) | [inline] |
Add another bounding box into this one, combining the two of them.
Definition at line 920 of file box.h.
References csMax(), csMin(), maxbox, minbox, csVector3::x, csVector3::y, and csVector3::z.
void csBox3::AddBoundingVertex | ( | const csVector3 & | v | ) | [inline] |
Add a new vertex and recalculate the bounding box.
Reimplemented in csOBB.
Definition at line 836 of file box.h.
References csVector3::x, csVector3::y, and csVector3::z.
void csBox3::AddBoundingVertex | ( | float | x, | |
float | y, | |||
float | z | |||
) | [inline] |
Add a new vertex and recalculate the bounding box.
Definition at line 828 of file box.h.
References csVector3::x.
void csBox3::AddBoundingVertexSmart | ( | const csVector3 & | v | ) | [inline] |
Add a new vertex and recalculate the bounding box.
This version is a little more optimal. It assumes however that at least one point has been added to the bounding box.
Definition at line 858 of file box.h.
References csVector3::x, csVector3::y, and csVector3::z.
void csBox3::AddBoundingVertexSmart | ( | float | x, | |
float | y, | |||
float | z | |||
) | [inline] |
bool csBox3::AddBoundingVertexSmartTest | ( | const csVector3 & | v | ) | [inline] |
Add a new vertex and recalculate the bounding box.
This version is a little more optimal. It assumes however that at least one point has been added to the bounding box. Returns true if box was modified.
Definition at line 912 of file box.h.
References csVector3::x, csVector3::y, and csVector3::z.
bool csBox3::AddBoundingVertexSmartTest | ( | float | x, | |
float | y, | |||
float | z | |||
) | [inline] |
bool csBox3::AddBoundingVertexTest | ( | const csVector3 & | v | ) | [inline] |
Add a new vertex and recalculate the bounding box.
Returns true if box was modified.
Definition at line 883 of file box.h.
References csVector3::x, csVector3::y, and csVector3::z.
bool csBox3::AddBoundingVertexTest | ( | float | x, | |
float | y, | |||
float | z | |||
) | [inline] |
int csBox3::Adjacent | ( | const csBox3 & | other, | |
float | epsilon = SMALL_EPSILON | |||
) | const |
Test if this box is adjacent to the other one.
Return -1 if not adjacent or else any of the CS_BOX_SIDE_x etc. flags to indicate the side of this box that the other box is adjacent with. The epsilon value is used to decide when adjacency is ok.
bool csBox3::AdjacentX | ( | const csBox3 & | other, | |
float | epsilon = SMALL_EPSILON | |||
) | const |
Test if this box is adjacent to the other on the X side.
bool csBox3::AdjacentY | ( | const csBox3 & | other, | |
float | epsilon = SMALL_EPSILON | |||
) | const |
Test if this box is adjacent to the other on the Y side.
bool csBox3::AdjacentZ | ( | const csBox3 & | other, | |
float | epsilon = SMALL_EPSILON | |||
) | const |
Test if this box is adjacent to the other on the Z side.
Test if this box is between two others.
int csBox3::CalculatePointSegment | ( | const csVector3 & | pos | ) | const |
Assume that 3D space is divided into 27 areas.
One is inside the box. The other 26 are rectangular segments around the box. This function will calculate the right segment for a given point and return that.
bool csBox3::Contains | ( | const csBox3 & | box | ) | const [inline] |
Test if this box contains the other box.
Definition at line 794 of file box.h.
References maxbox, minbox, csVector3::x, csVector3::y, and csVector3::z.
csString csBox3::Description | ( | ) | const |
Return a textual representation of the box in the form "(minx,miny,minz)-(maxx,maxy,maxz)".
bool csBox3::Empty | ( | ) | const [inline] |
void csBox3::GetAxisPlane | ( | int | side, | |
int & | axis, | |||
float & | where | |||
) | const |
Get axis aligned plane information from a side of this box.
Side is one of CS_BOX_SIDE_x. Axis will be one of CS_AXIS_X, CS_AXIS_Y or CS_AXIS_Z.
csVector3 csBox3::GetCenter | ( | ) | const [inline] |
void csBox3::GetConvexOutline | ( | const csVector3 & | pos, | |
csVector3 * | array, | |||
int & | num_array, | |||
bool | bVisible = false | |||
) | const |
Get a convex outline (not a polygon unless projected to 2D) for for this box as seen from the given position.
The coordinates returned are world space coordinates. Note that you need place for at least six vectors in the array. If you set bVisible true, you will get all visible corners - this could be up to 7.
csVector3 csBox3::GetCorner | ( | int | corner | ) | const |
Return every corner of this bounding box from 0 to 7.
This contrasts with Min() and Max() because those are only the min and max corners. Corner 0 = xyz, 1 = xyZ, 2 = xYz, 3 = xYZ, 4 = Xyz, 5 = XyZ, 6 = XYz, 7 = XYZ. Use CS_BOX_CORNER_xyz etc. defines. CS_BOX_CENTER3 also works.
Reimplemented in csOBB.
Referenced by csPolygonMeshBox::SetBox().
void csBox3::GetEdge | ( | int | edge, | |
csSegment3 & | e | |||
) | const [inline] |
Return every edge (segment) of this bounding box from 0 to 23 (use one of the CS_BOX_EDGE_Xyz_xyz etc.
indices). The returned edge is undefined for any other index.
Definition at line 763 of file box.h.
References csSegment3::SetEnd(), and csSegment3::SetStart().
csSegment3 csBox3::GetEdge | ( | int | edge | ) | const [inline] |
Return every edge (segment) of this bounding box from 0 to 23 (use one of the CS_BOX_EDGE_Xyz_xyz etc.
indices). The returned edge is undefined for any other index.
void csBox3::GetEdgeInfo | ( | int | edge, | |
int & | v1, | |||
int & | v2, | |||
int & | fleft, | |||
int & | fright | |||
) | const [inline] |
Given an edge index (CS_BOX_EDGE_Xyz_xyz etc.
) return the two vertices (index CS_BOX_CORNER_xyz, etc.) and left/right faces (CS_BOX_SIDE_x, etc.).
const uint8* csBox3::GetFaceEdges | ( | int | face | ) | const [inline] |
Given a face index (CS_BOX_SIDE_x etc.
) return the four edges oriented clockwise around this face (CS_BOX_EDGE_Xyz_xyz etc.).
csBox2 csBox3::GetSide | ( | int | side | ) | const |
Get a side of this box as a 2D box.
Use CS_BOX_SIDE_x etc. defines.
csVector3 csBox3::GetSize | ( | ) | const [inline] |
int csBox3::GetVisibleSides | ( | const csVector3 & | pos, | |
int * | visible_sides | |||
) | const |
Fill the array (which should be three long at least) with all visible sides (CS_BOX_SIDE_x etc.
defines) as seen from the given point. Returns the number of visible sides.
bool csBox3::In | ( | const csVector3 & | v | ) | const [inline] |
Test if the given coordinate is in this box.
Definition at line 779 of file box.h.
References csVector3::x, csVector3::y, and csVector3::z.
bool csBox3::In | ( | float | x, | |
float | y, | |||
float | z | |||
) | const [inline] |
Calculate the minimum manhattan distance between this box and another one.
const csVector3& csBox3::Max | ( | ) | const [inline] |
float csBox3::Max | ( | int | idx | ) | const [inline] |
float csBox3::MaxX | ( | ) | const [inline] |
float csBox3::MaxY | ( | ) | const [inline] |
float csBox3::MaxZ | ( | ) | const [inline] |
const csVector3& csBox3::Min | ( | ) | const [inline] |
float csBox3::Min | ( | int | idx | ) | const [inline] |
float csBox3::MinX | ( | ) | const [inline] |
float csBox3::MinY | ( | ) | const [inline] |
float csBox3::MinZ | ( | ) | const [inline] |
Compute the union of a point with this bounding box.
static int csBox3::OtherSide | ( | int | side | ) | [inline, static] |
Static function to get the 'other' side (i.e.
CS_BOX_SIDE_X to CS_BOX_SIDE_x, ...).
bool csBox3::Overlap | ( | const csBox3 & | box | ) | const [inline] |
Test if this box overlaps with the given box.
Definition at line 785 of file box.h.
References maxbox, minbox, csVector3::x, csVector3::y, and csVector3::z.
bool csBox3::ProjectBox | ( | const csTransform & | trans, | |
float | fov, | |||
float | sx, | |||
float | sy, | |||
csBox2 & | sbox, | |||
float & | min_z, | |||
float & | max_z | |||
) | const |
Project this box to a 2D bounding box given the view point transformation and also the field-of-view and shift values (for perspective projection).
The transform should transform from world to camera space (using Other2This). The minimum and maximum z are also calculated. If the bounding box is behind the camera then the 'sbox' will not be calculated (min_z and max_z are still calculated) and the function will return false. If the camera is inside the transformed box then this function will return true and a conservative screen space bounding box is returned.
bool csBox3::ProjectBoxAndOutline | ( | const csTransform & | trans, | |
float | fov, | |||
float | sx, | |||
float | sy, | |||
csBox2 & | sbox, | |||
csPoly2D & | poly, | |||
float & | min_z, | |||
float & | max_z | |||
) | const |
Project this box to the 2D outline given the view point transformation and also the field-of-view and shift values (for perspective correction).
The minimum and maximum z are also calculated. If the box is fully behind the camera then false is returned and this function will not do anything. If the box is partially behind the camera you will get an outline that is conservatively correct (i.e. it will overestimate the box). In addition to the outline this function also returns the projected screen-space box. So it is a combination of ProjectBox() and ProjectOutline().
bool csBox3::ProjectOutline | ( | const csVector3 & | origin, | |
int | axis, | |||
float | where, | |||
csPoly2D & | poly | |||
) | const |
Project this box to the 2D outline given the origin and an axis aligned plane.
If this fails (because some of the points cannot be projected) then it will return false.
bool csBox3::ProjectOutline | ( | const csVector3 & | origin, | |
int | axis, | |||
float | where, | |||
csArray< csVector2 > & | poly | |||
) | const |
Project this box to the 2D outline given the origin and an axis aligned plane.
If this fails (because some of the points cannot be projected) then it will return false. Note that this function will NOT clear the input array. So it will add the projected vertices after the vertices that may already be there.
bool csBox3::ProjectOutline | ( | const csTransform & | trans, | |
float | fov, | |||
float | sx, | |||
float | sy, | |||
csPoly2D & | poly, | |||
float & | min_z, | |||
float & | max_z | |||
) | const |
Project this box to the 2D outline given the view point transformation and also the field-of-view and shift values (for perspective correction).
The minimum and maximum z are also calculated. If the box is fully behind the camera then false is returned and this function will not do anything. If the box is partially behind the camera you will get an outline that is conservatively correct (i.e. it will overestimate the box).
void csBox3::Set | ( | float | x1, | |
float | y1, | |||
float | z1, | |||
float | x2, | |||
float | y2, | |||
float | z2 | |||
) | [inline] |
void csBox3::SetCenter | ( | const csVector3 & | c | ) |
Set the center of this box.
This will not change the size of the box but just relocate the center.
void csBox3::SetMax | ( | int | idx, | |
float | val | |||
) | [inline] |
void csBox3::SetMin | ( | int | idx, | |
float | val | |||
) | [inline] |
void csBox3::SetSize | ( | const csVector3 & | s | ) |
Set the size of the box but keep the center intact.
float csBox3::SquaredOriginDist | ( | ) | const |
Calculate the squared distance between (0,0,0) and the box This routine is extremely efficient.
float csBox3::SquaredOriginMaxDist | ( | ) | const |
Calculate the squared distance between (0,0,0) and the point on the box which is furthest away from (0,0,0).
This routine is extremely efficient.
float csBox3::SquaredPosDist | ( | const csVector3 & | pos | ) | const |
Calculate the squared distance between pos and the box This routine is extremely efficient.
float csBox3::SquaredPosMaxDist | ( | const csVector3 & | pos | ) | const |
Calculate the squared distance between pos and the point on the box which is furthest away from pos.
This routine is extremely efficient.
void csBox3::StartBoundingBox | ( | const csVector3 & | v | ) | [inline] |
void csBox3::StartBoundingBox | ( | ) | [inline] |
Initialize this box to empty.
Definition at line 811 of file box.h.
References CS_BOUNDINGBOX_MAXVALUE.
bool csBox3::TestIntersect | ( | const csBox3 & | box | ) | const |
int csBox3::TestSplit | ( | int | axis, | |
float | where | |||
) | const [inline] |
float csBox3::Volume | ( | ) | const [inline] |
Friends And Related Function Documentation
Compute the intersection of two bounding boxes.
Tests if two bounding boxes are unequal.
Compute the union of a bounding box and a point.
Compute the union of two bounding boxes.
Tests if a point is contained in a box.
Tests if box1 is a subset of box2.
Tests if two bounding boxes are equal.
Tests if box1 is a superset of box2.
Member Data Documentation
const bEdge csBox3::edges[24] [static, protected] |
const bFace csBox3::faces[6] [static, protected] |
csVector3 csBox3::maxbox [protected] |
The bottom-right.
Definition at line 601 of file box.h.
Referenced by AddBoundingBox(), Contains(), and Overlap().
csVector3 csBox3::minbox [protected] |
The top-left of this bounding box.
Definition at line 599 of file box.h.
Referenced by AddBoundingBox(), Contains(), and Overlap().
const Outline csBox3::outlines[27] [static, protected] |
The documentation for this class was generated from the following file:
- csgeom/box.h
Generated for Crystal Space by doxygen 1.4.7